Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / DateTimeEditor.cs / 1 / DateTimeEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using System.Drawing; using System.Drawing.Design; using System.Windows.Forms; using System.Windows.Forms.Design; ////// /// /// public class DateTimeEditor : UITypeEditor { ////// This date/time editor is a UITypeEditor suitable for /// visually editing DateTime objects. /// ////// /// Edits the given object value using the editor style provided by /// GetEditorStyle. A service provider is provided so that any /// required editing services can be obtained. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { object returnValue = value; if (provider != null) { IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); if (edSvc != null) { using (DateTimeUI dateTimeUI = new DateTimeUI()) { dateTimeUI.Start(edSvc, value); edSvc.DropDownControl(dateTimeUI); value = dateTimeUI.Value; dateTimeUI.End(); } } } return value; } ////// /// Retrieves the editing style of the Edit method. If the method /// is not supported, this will return None. /// [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] // everything in this assembly is full trust. public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.DropDown; } ////// /// UI we drop down to pick dates. /// private class DateTimeUI : Control { private MonthCalendar monthCalendar = new DateTimeMonthCalendar(); private object value; private IWindowsFormsEditorService edSvc; ////// /// public DateTimeUI() { InitializeComponent(); Size = monthCalendar.SingleMonthSize; monthCalendar.Resize += new EventHandler(this.MonthCalResize); } ////// /// public object Value { get { return value; } } ////// /// public void End() { edSvc = null; value = null; } private void MonthCalKeyDown(object sender, KeyEventArgs e) { switch (e.KeyCode) { case Keys.Enter: OnDateSelected(sender, null); break; } } ////// /// private void InitializeComponent() { monthCalendar.DateSelected += new DateRangeEventHandler(this.OnDateSelected); monthCalendar.KeyDown += new KeyEventHandler(this.MonthCalKeyDown); this.Controls.Add(monthCalendar); } private void MonthCalResize(object sender, EventArgs e) { this.Size = monthCalendar.Size; } ////// /// private void OnDateSelected(object sender, DateRangeEventArgs e) { value = monthCalendar.SelectionStart; edSvc.CloseDropDown(); } protected override void OnGotFocus(EventArgs e) { base.OnGotFocus(e); monthCalendar.Focus(); } ////// /// public void Start(IWindowsFormsEditorService edSvc, object value) { this.edSvc = edSvc; this.value = value; if (value != null) { DateTime dt = (DateTime) value; monthCalendar.SetDate((dt.Equals(DateTime.MinValue)) ? DateTime.Today : dt); } } class DateTimeMonthCalendar : MonthCalendar { protected override bool IsInputKey(System.Windows.Forms.Keys keyData) { switch (keyData) { case Keys.Enter: return true; } return base.IsInputKey(keyData); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
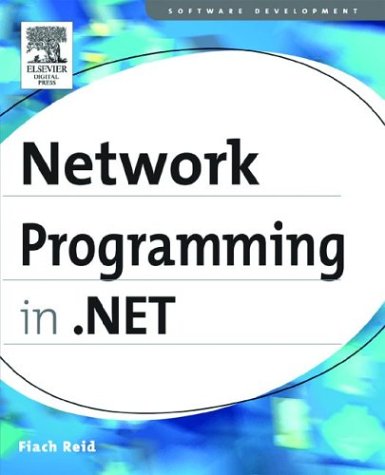
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsPrincipal.cs
- EraserBehavior.cs
- WmfPlaceableFileHeader.cs
- Transform3DGroup.cs
- UpdateTranslator.cs
- XPathQueryGenerator.cs
- TranslateTransform.cs
- QueryStoreStatusRequest.cs
- SchemaSetCompiler.cs
- ColorAnimation.cs
- ThreadStaticAttribute.cs
- StringResourceManager.cs
- DataGridViewRowsAddedEventArgs.cs
- DataSourceCache.cs
- PointLightBase.cs
- SettingsAttributes.cs
- WebPartEditorApplyVerb.cs
- HtmlMeta.cs
- cryptoapiTransform.cs
- DropDownButton.cs
- FixedSOMContainer.cs
- TakeQueryOptionExpression.cs
- EventProviderTraceListener.cs
- HttpCapabilitiesBase.cs
- TemplateBindingExpression.cs
- ControlEvent.cs
- Int16KeyFrameCollection.cs
- AutomationElementCollection.cs
- BuildResult.cs
- LogEntryHeaderDeserializer.cs
- ColorTransformHelper.cs
- TreeViewItem.cs
- DataQuery.cs
- HybridDictionary.cs
- ListViewItemSelectionChangedEvent.cs
- FolderBrowserDialog.cs
- TextSyndicationContentKindHelper.cs
- LoginUtil.cs
- MetadataItemSerializer.cs
- ListViewTableCell.cs
- ImportContext.cs
- Int64Animation.cs
- InvalidCardException.cs
- ElementAtQueryOperator.cs
- ModuleConfigurationInfo.cs
- SettingsPropertyWrongTypeException.cs
- ToolStripArrowRenderEventArgs.cs
- ProjectedSlot.cs
- TemplateControlBuildProvider.cs
- RecordBuilder.cs
- OptimalBreakSession.cs
- DeferredSelectedIndexReference.cs
- ResourceDefaultValueAttribute.cs
- FamilyTypeface.cs
- DtrList.cs
- TypeReference.cs
- Funcletizer.cs
- XmlWrappingWriter.cs
- Effect.cs
- WebPermission.cs
- PermissionSetTriple.cs
- EventLogPermissionEntry.cs
- TargetInvocationException.cs
- SymbolTable.cs
- ProcessManager.cs
- CompilerInfo.cs
- XmlAttributeCollection.cs
- StreamGeometry.cs
- ProxyGenerationError.cs
- XmlSchemaException.cs
- RegexCharClass.cs
- ActionItem.cs
- HealthMonitoringSection.cs
- RawAppCommandInputReport.cs
- HandlerBase.cs
- MexTcpBindingElement.cs
- CqlWriter.cs
- ProxyWebPartManager.cs
- Typeface.cs
- DataTableTypeConverter.cs
- ToolBarPanel.cs
- UshortList2.cs
- CategoryNameCollection.cs
- DataGridColumn.cs
- PageCache.cs
- DesignerAttribute.cs
- DocumentViewer.cs
- RectKeyFrameCollection.cs
- Matrix.cs
- FixedPageStructure.cs
- FrameworkTemplate.cs
- __Filters.cs
- SQLGuid.cs
- CompressStream.cs
- ThumbButtonInfo.cs
- StringArrayConverter.cs
- ClientSettingsSection.cs
- ImageSource.cs
- CheckBoxDesigner.cs
- CodeMemberEvent.cs