Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / TreeViewImageIndexConverter.cs / 1 / TreeViewImageIndexConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Collections.Specialized; ////// /// TreeViewImageIndexConverter is a class that can be used to convert /// image index values one data type to another. /// public class TreeViewImageIndexConverter : ImageIndexConverter { ///protected override bool IncludeNoneAsStandardValue { get { return false; } } /// /// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { string strValue = value as string; if (strValue != null) { if (String.Compare(strValue, SR.GetString(SR.toStringDefault), true, culture) == 0) { return -1; } else if (String.Compare(strValue, SR.GetString(SR.toStringNone), true, culture) == 0) { return -2; } } return base.ConvertFrom(context, culture, value); } ////// Converts the given value object to a 32-bit signed integer object. /// ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string) && value is int) { int intValue = (int)value; if (intValue == -1) { return SR.GetString(SR.toStringDefault); } else if (intValue == -2) { return SR.GetString(SR.toStringNone); } } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null && context.Instance != null) { object instance = context.Instance; PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); while (instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { ImageList imageList = (ImageList)imageListProp.GetValue(instance); if (imageList != null) { // Create array to contain standard values // object[] values; int nImages = imageList.Images.Count+2; values = new object[nImages]; values[nImages-2] = -1; values[nImages-1] = -2; // Fill in the array // for (int i = 0; i < nImages-2; i++) { values[i] = i; } return new StandardValuesCollection(values); } } } return new StandardValuesCollection(new object[] { -1, -2 }); } } } // Namespace system.windows.forms // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Collections.Specialized; ////// /// TreeViewImageIndexConverter is a class that can be used to convert /// image index values one data type to another. /// public class TreeViewImageIndexConverter : ImageIndexConverter { ///protected override bool IncludeNoneAsStandardValue { get { return false; } } /// /// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { string strValue = value as string; if (strValue != null) { if (String.Compare(strValue, SR.GetString(SR.toStringDefault), true, culture) == 0) { return -1; } else if (String.Compare(strValue, SR.GetString(SR.toStringNone), true, culture) == 0) { return -2; } } return base.ConvertFrom(context, culture, value); } ////// Converts the given value object to a 32-bit signed integer object. /// ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string) && value is int) { int intValue = (int)value; if (intValue == -1) { return SR.GetString(SR.toStringDefault); } else if (intValue == -2) { return SR.GetString(SR.toStringNone); } } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null && context.Instance != null) { object instance = context.Instance; PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); while (instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { ImageList imageList = (ImageList)imageListProp.GetValue(instance); if (imageList != null) { // Create array to contain standard values // object[] values; int nImages = imageList.Images.Count+2; values = new object[nImages]; values[nImages-2] = -1; values[nImages-1] = -2; // Fill in the array // for (int i = 0; i < nImages-2; i++) { values[i] = i; } return new StandardValuesCollection(values); } } } return new StandardValuesCollection(new object[] { -1, -2 }); } } } // Namespace system.windows.forms // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
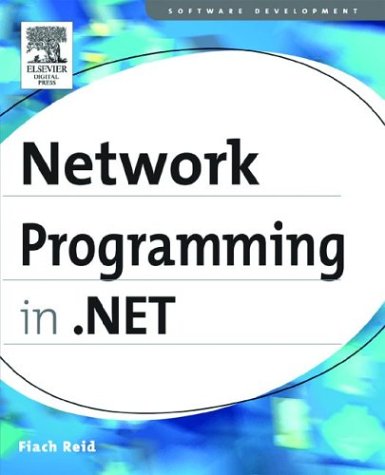
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProbeRequestResponseAsyncResult.cs
- MessageQueuePermission.cs
- PackageRelationship.cs
- SqlNotificationEventArgs.cs
- SplitterPanel.cs
- FirstMatchCodeGroup.cs
- SqlCrossApplyToCrossJoin.cs
- ListViewTableRow.cs
- LogicalMethodInfo.cs
- RequestCacheManager.cs
- PropertyPathConverter.cs
- HttpValueCollection.cs
- CallContext.cs
- EventLog.cs
- XMLSchema.cs
- PageHandlerFactory.cs
- ListContractAdapter.cs
- AnnotationHelper.cs
- SQLSingle.cs
- EditorBrowsableAttribute.cs
- TemplateControlCodeDomTreeGenerator.cs
- HyperLinkDesigner.cs
- hwndwrapper.cs
- DataServiceProviderWrapper.cs
- SymbolMethod.cs
- WindowsTitleBar.cs
- RenderData.cs
- ObjectCacheHost.cs
- ComplexType.cs
- DataSourceControlBuilder.cs
- XmlNodeReader.cs
- CodeExpressionCollection.cs
- RegexInterpreter.cs
- Types.cs
- CroppedBitmap.cs
- TextTreeTextNode.cs
- SqlDataSourceSelectingEventArgs.cs
- BuildProviderAppliesToAttribute.cs
- Visual3D.cs
- BatchStream.cs
- RectConverter.cs
- CompressionTransform.cs
- SerializationInfo.cs
- DeviceContext2.cs
- Model3DGroup.cs
- SuppressMessageAttribute.cs
- JobDuplex.cs
- SqlStream.cs
- ProcessThreadCollection.cs
- WebPart.cs
- TextElementEnumerator.cs
- ResourceDictionary.cs
- Privilege.cs
- DataServiceContext.cs
- DictionarySectionHandler.cs
- Clipboard.cs
- ItemsControlAutomationPeer.cs
- AffineTransform3D.cs
- WindowsStatusBar.cs
- MarkupExtensionParser.cs
- RuleSet.cs
- CheckBoxAutomationPeer.cs
- Mutex.cs
- ItemList.cs
- PasswordPropertyTextAttribute.cs
- CompiledAction.cs
- DeadLetterQueue.cs
- DynamicScriptObject.cs
- CommittableTransaction.cs
- EtwTrackingBehaviorElement.cs
- CodePageUtils.cs
- Random.cs
- ErrorLog.cs
- TrackBar.cs
- IDictionary.cs
- InputReport.cs
- InputLanguageManager.cs
- WorkItem.cs
- ChildTable.cs
- TextOnlyOutput.cs
- ToolStripContainer.cs
- HttpModuleCollection.cs
- TextServicesCompartmentContext.cs
- SecurityTokenAuthenticator.cs
- Subtree.cs
- TemplateBindingExpression.cs
- coordinatorfactory.cs
- ProjectionPathBuilder.cs
- DataGridAddNewRow.cs
- TriggerAction.cs
- TransformFinalBlockRequest.cs
- RedirectionProxy.cs
- Setter.cs
- LingerOption.cs
- WorkflowTransactionService.cs
- HyperLink.cs
- Encoder.cs
- ActivityWithResult.cs
- CodeBlockBuilder.cs
- ContractsBCL.cs