Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Runtime / Serialization / SerializationInfo.cs / 1 / SerializationInfo.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SerializationInfo ** ** ** Purpose: The structure for holding all of the data needed ** for object serialization and deserialization. ** ** ===========================================================*/ namespace System.Runtime.Serialization { using System; using System.Reflection; using System.Runtime.Remoting; using System.Runtime.Remoting.Proxies; using System.Globalization; [System.Runtime.InteropServices.ComVisible(true)] public sealed class SerializationInfo { private const int defaultSize = 4; internal String[] m_members; internal Object[] m_data; internal Type[] m_types; internal String m_fullTypeName; internal int m_currMember; internal String m_assemName; internal IFormatterConverter m_converter; [CLSCompliant(false)] public SerializationInfo(Type type, IFormatterConverter converter) { if (null==type) { throw new ArgumentNullException("type"); } if (converter==null) { throw new ArgumentNullException("converter"); } m_fullTypeName = type.FullName; m_assemName = type.Module.Assembly.FullName; BCLDebug.Assert(m_fullTypeName!=null, "[SerializationInfo.ctor]m_fullTypeName!=null"); BCLDebug.Assert(m_assemName!=null, "[SerializationInfo.ctor]m_assemName!=null"); m_members= new String[defaultSize]; m_data = new Object[defaultSize]; m_types = new Type[defaultSize]; m_converter = converter; m_currMember = 0; } public String FullTypeName { get { return m_fullTypeName; } set { if (null==value) { throw new ArgumentNullException("value"); } m_fullTypeName = value; } } public String AssemblyName { get { return m_assemName; } set { if (null==value) { throw new ArgumentNullException("value"); } m_assemName = value; } } public void SetType(Type type) { if (type==null) { throw new ArgumentNullException("type"); } m_fullTypeName = type.FullName; m_assemName = type.Module.Assembly.FullName; BCLDebug.Assert(m_fullTypeName!=null, "[SerializationInfo.ctor]m_fullTypeName!=null"); BCLDebug.Assert(m_assemName!=null, "[SerializationInfo.ctor]m_assemName!=null"); } public int MemberCount { get { return m_currMember; } } public SerializationInfoEnumerator GetEnumerator() { return new SerializationInfoEnumerator(m_members, m_data, m_types, m_currMember); } private void ExpandArrays() { int newSize; BCLDebug.Assert(m_members.Length == m_currMember, "[SerializationInfo.ExpandArrays]m_members.Length == m_currMember"); newSize = (m_currMember * 2); // // In the pathological case, we may wrap // if (newSizem_currMember) { newSize = Int32.MaxValue; } } // // Allocate more space and copy the data // String[] newMembers = new String[newSize]; Object[] newData = new Object[newSize]; Type[] newTypes = new Type[newSize]; Array.Copy(m_members, newMembers, m_currMember); Array.Copy(m_data, newData, m_currMember); Array.Copy(m_types, newTypes, m_currMember); // // Assign the new arrys back to the member vars. // m_members = newMembers; m_data = newData; m_types = newTypes; } public void AddValue(String name, Object value, Type type) { if (null==name) { throw new ArgumentNullException("name"); } if (null==type) { throw new ArgumentNullException("type"); } // // Walk until we find a member by the same name or until // we reach the end. If we find a member by the same name, // throw. for (int i=0; i =m_members.Length) { ExpandArrays(); } // // Add the data and then advance the counter. // m_members[index] = name; m_data[index] = value; m_types[index] = type; m_currMember++; } /*=================================UpdateValue================================== **Action: Finds the value if it exists in the current data. If it does, we replace ** the values, if not, we append it to the end. This is useful to the ** ObjectManager when it's performing fixups, but shouldn't be used by ** clients. Exposing out this functionality would allow children to overwrite ** their parent's values. **Returns: void **Arguments: name -- the name of the data to be updated. ** value -- the new value. ** type -- the type of the data being added. **Exceptions: None. All error checking is done with asserts. ==============================================================================*/ internal void UpdateValue(String name, Object value, Type type) { BCLDebug.Assert(null!=name, "[SerializationInfo.UpdateValue]name!=null"); BCLDebug.Assert(null!=value, "[SerializationInfo.UpdateValue]value!=null"); BCLDebug.Assert(null!=type, "[SerializationInfo.UpdateValue]type!=null"); int index = FindElement(name); if (index<0) { AddValue(name, value, type, m_currMember); } else { m_members[index] = name; m_data[index] = value; m_types[index] = type; } } private int FindElement (String name) { if (null==name) { throw new ArgumentNullException("name"); } BCLDebug.Trace("SER", "[SerializationInfo.FindElement]Looking for ", name, " CurrMember is: ", m_currMember); for (int i=0; i
Link Menu
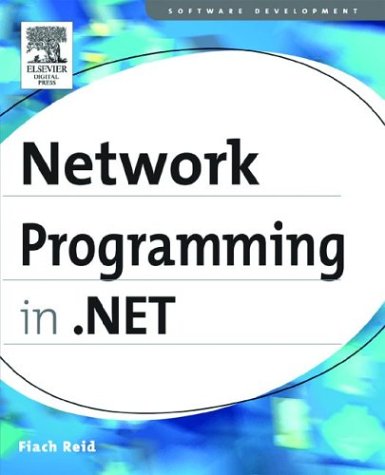
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnSafeCharBuffer.cs
- HtmlInputButton.cs
- WindowsProgressbar.cs
- EntityDataSourceWrapperCollection.cs
- LinqDataView.cs
- HttpFileCollection.cs
- COM2PropertyBuilderUITypeEditor.cs
- ItemList.cs
- BehaviorEditorPart.cs
- CounterCreationDataCollection.cs
- PrintPreviewDialog.cs
- ReflectionUtil.cs
- HtmlEmptyTagControlBuilder.cs
- MenuEventArgs.cs
- BaseProcessor.cs
- DocumentGridPage.cs
- PartialCachingControl.cs
- Environment.cs
- EnumBuilder.cs
- TrackingParameters.cs
- ObfuscateAssemblyAttribute.cs
- BigInt.cs
- IssuedTokenClientBehaviorsElementCollection.cs
- DataControlFieldCollection.cs
- LoadedOrUnloadedOperation.cs
- EntityRecordInfo.cs
- QueryStringConverter.cs
- XmlQualifiedNameTest.cs
- DecoderReplacementFallback.cs
- CalendarDay.cs
- PropertyEmitter.cs
- QuaternionRotation3D.cs
- IBuiltInEvidence.cs
- FontDialog.cs
- XmlChildNodes.cs
- HtmlControl.cs
- XpsFontSubsetter.cs
- FlagsAttribute.cs
- ViewStateModeByIdAttribute.cs
- ToolStripContentPanelRenderEventArgs.cs
- DataSourceExpression.cs
- InkPresenterAutomationPeer.cs
- SrgsElementFactory.cs
- ErrorLog.cs
- Internal.cs
- LocalizationParserHooks.cs
- InstanceDataCollection.cs
- CredentialCache.cs
- HtmlInputText.cs
- BaseCodeDomTreeGenerator.cs
- RuleValidation.cs
- PngBitmapDecoder.cs
- BinaryVersion.cs
- ForEachDesigner.xaml.cs
- ToolStripDropDownButton.cs
- State.cs
- DataGridViewRowPrePaintEventArgs.cs
- DirectoryNotFoundException.cs
- DefaultParameterValueAttribute.cs
- ViewPort3D.cs
- CatalogPartDesigner.cs
- DefaultValueMapping.cs
- BoolExpr.cs
- Funcletizer.cs
- ControlParameter.cs
- Exception.cs
- RangeValidator.cs
- MachineKeySection.cs
- EndpointAddress.cs
- SoapProtocolReflector.cs
- MouseButtonEventArgs.cs
- CustomAttributeBuilder.cs
- ByteStorage.cs
- SafeNativeMethods.cs
- Transform.cs
- InstanceContextManager.cs
- MenuBase.cs
- HtmlControl.cs
- DockingAttribute.cs
- ComplexBindingPropertiesAttribute.cs
- ListBoxItem.cs
- TickBar.cs
- HttpValueCollection.cs
- SQLDecimal.cs
- SymmetricKey.cs
- VirtualPathProvider.cs
- ApplicationCommands.cs
- PriorityBindingExpression.cs
- CompoundFileIOPermission.cs
- ArithmeticException.cs
- ComplexBindingPropertiesAttribute.cs
- Image.cs
- SettingsPropertyNotFoundException.cs
- SoapAttributeOverrides.cs
- CustomBindingCollectionElement.cs
- fixedPageContentExtractor.cs
- ClientTargetCollection.cs
- ObjectTag.cs
- PenContext.cs
- WebHttpBehavior.cs