Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / WebControls / ControlParameter.cs / 1 / ControlParameter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Data; using System.Web.UI.WebControls; using System.Security.Permissions; ////// Represents a Parameter that gets its value from a control's property. /// [ DefaultProperty("ControlID"), ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ControlParameter : Parameter { ////// Creates an instance of the ControlParameter class. /// public ControlParameter() { } ////// Creates an instance of the ControlParameter class with the specified parameter name and control ID. /// public ControlParameter(string name, string controlID) : base(name) { ControlID = controlID; } ////// Creates an instance of the ControlParameter class with the specified parameter name, control ID, and property name. /// public ControlParameter(string name, string controlID, string propertyName) : base(name) { ControlID = controlID; PropertyName = propertyName; } ////// Creates an instance of the ControlParameter class with the specified parameter name, database type, /// control ID, and property name. /// public ControlParameter(string name, DbType dbType, string controlID, string propertyName) : base(name, dbType) { ControlID = controlID; PropertyName = propertyName; } ////// Creates an instance of the ControlParameter class with the specified parameter name, type, control ID, and property name. /// public ControlParameter(string name, TypeCode type, string controlID, string propertyName) : base(name, type) { ControlID = controlID; PropertyName = propertyName; } ////// Used to clone a parameter. /// protected ControlParameter(ControlParameter original) : base(original) { ControlID = original.ControlID; PropertyName = original.PropertyName; } ////// The ID of the control to get the value from. /// [ DefaultValue(""), IDReferenceProperty(), RefreshProperties(RefreshProperties.All), TypeConverter(typeof(ControlIDConverter)), WebCategory("Control"), WebSysDescription(SR.ControlParameter_ControlID), ] public string ControlID { get { object o = ViewState["ControlID"]; if (o == null) return String.Empty; return (string)o; } set { if (ControlID != value) { ViewState["ControlID"] = value; OnParameterChanged(); } } } ////// The name of the control's property to get the value from. /// If none is specified, the ControlValueProperty attribute of the control will be examined to determine the default property name. /// [ DefaultValue(""), TypeConverter(typeof(ControlPropertyNameConverter)), WebCategory("Control"), WebSysDescription(SR.ControlParameter_PropertyName), ] public string PropertyName { get { object o = ViewState["PropertyName"]; if (o == null) return String.Empty; return (string)o; } set { if (PropertyName != value) { ViewState["PropertyName"] = value; OnParameterChanged(); } } } ////// Creates a new ControlParameter that is a copy of this ControlParameter. /// protected override Parameter Clone() { return new ControlParameter(this); } ////// Returns the updated value of the parameter. /// protected override object Evaluate(HttpContext context, Control control) { if (control == null) { return null; } string controlID = ControlID; string propertyName = PropertyName; if (controlID.Length == 0) { throw new ArgumentException(SR.GetString(SR.ControlParameter_ControlIDNotSpecified, Name)); } Control foundControl = DataBoundControlHelper.FindControl(control, controlID); if (foundControl == null) { throw new InvalidOperationException(SR.GetString(SR.ControlParameter_CouldNotFindControl, controlID, Name)); } ControlValuePropertyAttribute controlValueProp = (ControlValuePropertyAttribute)TypeDescriptor.GetAttributes(foundControl)[typeof(ControlValuePropertyAttribute)]; // If no property name is specified, use the ControlValuePropertyAttribute to determine which property to use. if (propertyName.Length == 0) { if ((controlValueProp != null) && (!String.IsNullOrEmpty(controlValueProp.Name))) { propertyName = controlValueProp.Name; } else { throw new InvalidOperationException(SR.GetString(SR.ControlParameter_PropertyNameNotSpecified, controlID, Name)); } } // Get the value of the property object value = DataBinder.Eval(foundControl, propertyName); // Convert the value to null if this is the default property and the value is the property's default value if (controlValueProp != null && String.Equals(controlValueProp.Name, propertyName, StringComparison.OrdinalIgnoreCase) && controlValueProp.DefaultValue != null && controlValueProp.DefaultValue.Equals(value)) { return null; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.Data; using System.Web.UI.WebControls; using System.Security.Permissions; ////// Represents a Parameter that gets its value from a control's property. /// [ DefaultProperty("ControlID"), ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ControlParameter : Parameter { ////// Creates an instance of the ControlParameter class. /// public ControlParameter() { } ////// Creates an instance of the ControlParameter class with the specified parameter name and control ID. /// public ControlParameter(string name, string controlID) : base(name) { ControlID = controlID; } ////// Creates an instance of the ControlParameter class with the specified parameter name, control ID, and property name. /// public ControlParameter(string name, string controlID, string propertyName) : base(name) { ControlID = controlID; PropertyName = propertyName; } ////// Creates an instance of the ControlParameter class with the specified parameter name, database type, /// control ID, and property name. /// public ControlParameter(string name, DbType dbType, string controlID, string propertyName) : base(name, dbType) { ControlID = controlID; PropertyName = propertyName; } ////// Creates an instance of the ControlParameter class with the specified parameter name, type, control ID, and property name. /// public ControlParameter(string name, TypeCode type, string controlID, string propertyName) : base(name, type) { ControlID = controlID; PropertyName = propertyName; } ////// Used to clone a parameter. /// protected ControlParameter(ControlParameter original) : base(original) { ControlID = original.ControlID; PropertyName = original.PropertyName; } ////// The ID of the control to get the value from. /// [ DefaultValue(""), IDReferenceProperty(), RefreshProperties(RefreshProperties.All), TypeConverter(typeof(ControlIDConverter)), WebCategory("Control"), WebSysDescription(SR.ControlParameter_ControlID), ] public string ControlID { get { object o = ViewState["ControlID"]; if (o == null) return String.Empty; return (string)o; } set { if (ControlID != value) { ViewState["ControlID"] = value; OnParameterChanged(); } } } ////// The name of the control's property to get the value from. /// If none is specified, the ControlValueProperty attribute of the control will be examined to determine the default property name. /// [ DefaultValue(""), TypeConverter(typeof(ControlPropertyNameConverter)), WebCategory("Control"), WebSysDescription(SR.ControlParameter_PropertyName), ] public string PropertyName { get { object o = ViewState["PropertyName"]; if (o == null) return String.Empty; return (string)o; } set { if (PropertyName != value) { ViewState["PropertyName"] = value; OnParameterChanged(); } } } ////// Creates a new ControlParameter that is a copy of this ControlParameter. /// protected override Parameter Clone() { return new ControlParameter(this); } ////// Returns the updated value of the parameter. /// protected override object Evaluate(HttpContext context, Control control) { if (control == null) { return null; } string controlID = ControlID; string propertyName = PropertyName; if (controlID.Length == 0) { throw new ArgumentException(SR.GetString(SR.ControlParameter_ControlIDNotSpecified, Name)); } Control foundControl = DataBoundControlHelper.FindControl(control, controlID); if (foundControl == null) { throw new InvalidOperationException(SR.GetString(SR.ControlParameter_CouldNotFindControl, controlID, Name)); } ControlValuePropertyAttribute controlValueProp = (ControlValuePropertyAttribute)TypeDescriptor.GetAttributes(foundControl)[typeof(ControlValuePropertyAttribute)]; // If no property name is specified, use the ControlValuePropertyAttribute to determine which property to use. if (propertyName.Length == 0) { if ((controlValueProp != null) && (!String.IsNullOrEmpty(controlValueProp.Name))) { propertyName = controlValueProp.Name; } else { throw new InvalidOperationException(SR.GetString(SR.ControlParameter_PropertyNameNotSpecified, controlID, Name)); } } // Get the value of the property object value = DataBinder.Eval(foundControl, propertyName); // Convert the value to null if this is the default property and the value is the property's default value if (controlValueProp != null && String.Equals(controlValueProp.Name, propertyName, StringComparison.OrdinalIgnoreCase) && controlValueProp.DefaultValue != null && controlValueProp.DefaultValue.Equals(value)) { return null; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
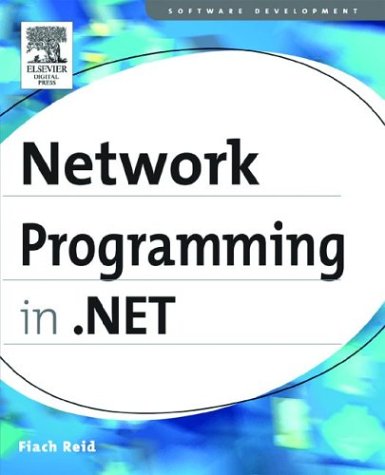
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _SingleItemRequestCache.cs
- ImpersonationContext.cs
- MarkupExtensionReturnTypeAttribute.cs
- CatalogZoneBase.cs
- DataGridSortCommandEventArgs.cs
- X509Chain.cs
- ModelService.cs
- XmlSiteMapProvider.cs
- ApplicationFileParser.cs
- WorkflowServiceHostFactory.cs
- PointCollectionConverter.cs
- WindowsStreamSecurityBindingElement.cs
- CroppedBitmap.cs
- WSDualHttpSecurity.cs
- SplineKeyFrames.cs
- EntitySetDataBindingList.cs
- xmlsaver.cs
- CustomValidator.cs
- SHA512.cs
- TypeListConverter.cs
- MenuEventArgs.cs
- XmlSchemaElement.cs
- SafeSystemMetrics.cs
- TargetPerspective.cs
- COAUTHINFO.cs
- SQLInt32Storage.cs
- _TimerThread.cs
- MenuRendererStandards.cs
- MarshalByValueComponent.cs
- EdmEntityTypeAttribute.cs
- GZipStream.cs
- FragmentNavigationEventArgs.cs
- EventProxy.cs
- JoinElimination.cs
- RequestCachePolicyConverter.cs
- JoinElimination.cs
- MemberInfoSerializationHolder.cs
- XmlSerializableWriter.cs
- XmlNodeChangedEventArgs.cs
- FormClosedEvent.cs
- PtsHelper.cs
- HttpModuleActionCollection.cs
- SessionMode.cs
- MethodCallExpression.cs
- WindowsServiceElement.cs
- MouseOverProperty.cs
- SchemaTableColumn.cs
- SizeFConverter.cs
- ClientTargetSection.cs
- AppSettingsReader.cs
- RenderDataDrawingContext.cs
- ColorConvertedBitmapExtension.cs
- WorkflowMessageEventArgs.cs
- SystemParameters.cs
- storepermission.cs
- WorkflowValidationFailedException.cs
- FlowLayout.cs
- SystemPens.cs
- TypeSystemHelpers.cs
- Model3DGroup.cs
- XmlValidatingReaderImpl.cs
- GraphicsContext.cs
- WebMessageEncodingElement.cs
- ExtractCollection.cs
- AddInProcess.cs
- LineInfo.cs
- RotateTransform.cs
- MarshalDirectiveException.cs
- ProgressBarAutomationPeer.cs
- DateTimeValueSerializerContext.cs
- XmlSchemaComplexContent.cs
- DoWorkEventArgs.cs
- UnhandledExceptionEventArgs.cs
- DataGridViewTextBoxEditingControl.cs
- SyndicationDeserializer.cs
- SpecialTypeDataContract.cs
- CodeGeneratorOptions.cs
- TextFormatterContext.cs
- HandlerMappingMemo.cs
- XmlCharacterData.cs
- EnlistmentTraceIdentifier.cs
- Lasso.cs
- ExpressionVisitorHelpers.cs
- IApplicationTrustManager.cs
- InternalControlCollection.cs
- XslAst.cs
- ChildTable.cs
- SystemSounds.cs
- GroupBoxAutomationPeer.cs
- ToolStripItemRenderEventArgs.cs
- ImageResources.Designer.cs
- AssemblyGen.cs
- DataSourceBooleanViewSchemaConverter.cs
- IndicCharClassifier.cs
- BitmapEffectvisualstate.cs
- FileDialog_Vista_Interop.cs
- WindowsGraphicsWrapper.cs
- ConfigurationManagerHelper.cs
- TrustLevelCollection.cs
- FontCacheUtil.cs