Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Effects / BitmapEffectvisualstate.cs / 1 / BitmapEffectvisualstate.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectVisualState.cs //----------------------------------------------------------------------------- using System; using System.Windows.Threading; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Resources; using MS.Utility; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Effects { ////// This class is used to realize the content of the /// visual if a bitmap effect is applied to it /// internal class BitmapEffectContent : IRealizationContextClient { Visual _visual; DUCE.Channel _channel; public BitmapEffectContent(Visual v, RealizationContext ctx) { _visual = v; _channel = ctx.Channel; } public void ExecuteRealizationsUpdate() { Visual.BitmapEffectStateField.GetValue(_visual).RenderBitmapEffect(_visual, _channel); } } ////// /// internal class BitmapEffectVisualState : BitmapEffectState { public BitmapEffectVisualState() { } public BitmapEffectDrawing BitmapEffectDrawing { get { if (_bitmapEffectDrawing == null) { _bitmapEffectDrawing = new BitmapEffectDrawing(); } return _bitmapEffectDrawing; } set { _bitmapEffectDrawing = null; } } ////// Remove the content from the composition node /// /// /// internal void FreeContent(Visual visual, DUCE.Channel channel) { SetContent(null, visual, channel); _bitmapEffectDrawing = null; } ////// This sets the content of the visual if there is a /// bitmap effect applied to it /// /// drawing content /// The visual /// The channel internal void SetContent(IDrawingContent content, Visual visual, DUCE.Channel channel) { DUCE.ResourceHandle visualHandle = ((DUCE.IResource)visual).GetHandle(channel); if (content != null) { content.AddRefOnChannel(channel); DUCE.CompositionNode.SetContent( visualHandle, content.GetHandle(channel), channel); // it is ok to release here, because the unmanaged code // holds a reference to the content and will release it // when the parent object is released or the content // is reset to null content.ReleaseOnChannel(channel); } else { Debug.Assert(visual.IsOnChannel(channel)); DUCE.CompositionNode.SetContent( visualHandle, DUCE.ResourceHandle.Null, channel); } } ////// This methods renders the visual to a bitmap, then applies /// the effect to the rendered bitmap, and sets the content /// of the visual to be the resulting bitmap /// /// The visual to apply the effect to /// The current channels internal void RenderBitmapEffect(Visual visual, DUCE.Channel channel) { if (_bitmapEffect == null) throw new InvalidOperationException(SR.Get(SRID.Visual_No_Effect, null)); Debug.Assert(channel != null); BitmapEffectDrawing.UpdateTransformAndDrawingLists(true); bool effectRendered = false; int drawingsCount = BitmapEffectDrawing.Drawings.Count; for (int iTransform = BitmapEffectDrawing.WorldTransforms.Count - 1; iTransform >= drawingsCount; iTransform--) { Matrix finalTransform = BitmapEffectDrawing.WorldTransforms[iTransform].Value; BitmapSource outputImage = GetEffectOutput(visual, ref _renderBitmap, finalTransform, BitmapEffectDrawing.WindowClip, out finalTransform); if (outputImage != null) { // if there was no group for that transform create one // and update it, otherwise we don't need to do anything it // NOTE: this is ok to update here since it is not // connected to the visual tree DrawingGroup effectGroup = new DrawingGroup(); effectGroup.Children.Add( new ImageDrawing(outputImage, new Rect(0, 0, outputImage.Width, outputImage.Height))); effectGroup.Transform = new MatrixTransform(finalTransform); BitmapEffectDrawing.Drawings.Insert(drawingsCount, effectGroup); effectRendered = true; } else { BitmapEffectDrawing.WorldTransforms.RemoveAt(iTransform); } } if (BitmapEffectDrawing.Drawings.Count > 0 && effectRendered == true) { SetContent(BitmapEffectDrawing, visual, channel); } visual.SetFlags(channel, false, VisualProxyFlags.IsBitmapEffectDirty); BitmapEffectDrawing.ScheduleForUpdates = true; } #region Private Fields BitmapEffectDrawing _bitmapEffectDrawing; RenderTargetBitmap _renderBitmap; #endregion } ////// This class is used to store the user provided bitmap effect data on Visual. /// It is necessary to implement the emulation layer for some legacy effects on top /// of the new pipline. /// internal class BitmapEffectData { private BitmapEffect _bitmapEffect; private BitmapEffectInput _bitmapEffectInput; public BitmapEffectData() {} public BitmapEffect BitmapEffect { get { return _bitmapEffect; } set { _bitmapEffect = value; } } public BitmapEffectInput BitmapEffectInput { get { return _bitmapEffectInput; } set { _bitmapEffectInput = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectVisualState.cs //----------------------------------------------------------------------------- using System; using System.Windows.Threading; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Diagnostics; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Resources; using MS.Utility; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Effects { ////// This class is used to realize the content of the /// visual if a bitmap effect is applied to it /// internal class BitmapEffectContent : IRealizationContextClient { Visual _visual; DUCE.Channel _channel; public BitmapEffectContent(Visual v, RealizationContext ctx) { _visual = v; _channel = ctx.Channel; } public void ExecuteRealizationsUpdate() { Visual.BitmapEffectStateField.GetValue(_visual).RenderBitmapEffect(_visual, _channel); } } ////// /// internal class BitmapEffectVisualState : BitmapEffectState { public BitmapEffectVisualState() { } public BitmapEffectDrawing BitmapEffectDrawing { get { if (_bitmapEffectDrawing == null) { _bitmapEffectDrawing = new BitmapEffectDrawing(); } return _bitmapEffectDrawing; } set { _bitmapEffectDrawing = null; } } ////// Remove the content from the composition node /// /// /// internal void FreeContent(Visual visual, DUCE.Channel channel) { SetContent(null, visual, channel); _bitmapEffectDrawing = null; } ////// This sets the content of the visual if there is a /// bitmap effect applied to it /// /// drawing content /// The visual /// The channel internal void SetContent(IDrawingContent content, Visual visual, DUCE.Channel channel) { DUCE.ResourceHandle visualHandle = ((DUCE.IResource)visual).GetHandle(channel); if (content != null) { content.AddRefOnChannel(channel); DUCE.CompositionNode.SetContent( visualHandle, content.GetHandle(channel), channel); // it is ok to release here, because the unmanaged code // holds a reference to the content and will release it // when the parent object is released or the content // is reset to null content.ReleaseOnChannel(channel); } else { Debug.Assert(visual.IsOnChannel(channel)); DUCE.CompositionNode.SetContent( visualHandle, DUCE.ResourceHandle.Null, channel); } } ////// This methods renders the visual to a bitmap, then applies /// the effect to the rendered bitmap, and sets the content /// of the visual to be the resulting bitmap /// /// The visual to apply the effect to /// The current channels internal void RenderBitmapEffect(Visual visual, DUCE.Channel channel) { if (_bitmapEffect == null) throw new InvalidOperationException(SR.Get(SRID.Visual_No_Effect, null)); Debug.Assert(channel != null); BitmapEffectDrawing.UpdateTransformAndDrawingLists(true); bool effectRendered = false; int drawingsCount = BitmapEffectDrawing.Drawings.Count; for (int iTransform = BitmapEffectDrawing.WorldTransforms.Count - 1; iTransform >= drawingsCount; iTransform--) { Matrix finalTransform = BitmapEffectDrawing.WorldTransforms[iTransform].Value; BitmapSource outputImage = GetEffectOutput(visual, ref _renderBitmap, finalTransform, BitmapEffectDrawing.WindowClip, out finalTransform); if (outputImage != null) { // if there was no group for that transform create one // and update it, otherwise we don't need to do anything it // NOTE: this is ok to update here since it is not // connected to the visual tree DrawingGroup effectGroup = new DrawingGroup(); effectGroup.Children.Add( new ImageDrawing(outputImage, new Rect(0, 0, outputImage.Width, outputImage.Height))); effectGroup.Transform = new MatrixTransform(finalTransform); BitmapEffectDrawing.Drawings.Insert(drawingsCount, effectGroup); effectRendered = true; } else { BitmapEffectDrawing.WorldTransforms.RemoveAt(iTransform); } } if (BitmapEffectDrawing.Drawings.Count > 0 && effectRendered == true) { SetContent(BitmapEffectDrawing, visual, channel); } visual.SetFlags(channel, false, VisualProxyFlags.IsBitmapEffectDirty); BitmapEffectDrawing.ScheduleForUpdates = true; } #region Private Fields BitmapEffectDrawing _bitmapEffectDrawing; RenderTargetBitmap _renderBitmap; #endregion } ////// This class is used to store the user provided bitmap effect data on Visual. /// It is necessary to implement the emulation layer for some legacy effects on top /// of the new pipline. /// internal class BitmapEffectData { private BitmapEffect _bitmapEffect; private BitmapEffectInput _bitmapEffectInput; public BitmapEffectData() {} public BitmapEffect BitmapEffect { get { return _bitmapEffect; } set { _bitmapEffect = value; } } public BitmapEffectInput BitmapEffectInput { get { return _bitmapEffectInput; } set { _bitmapEffectInput = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
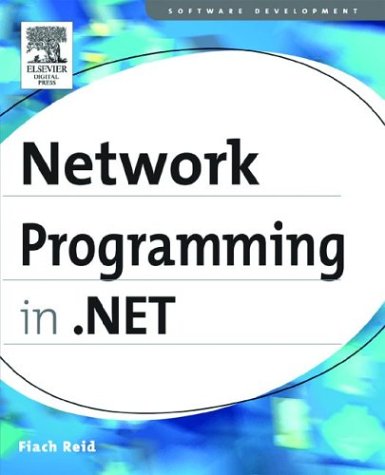
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputButton.cs
- TrackBar.cs
- RoleManagerEventArgs.cs
- TextDecorationUnitValidation.cs
- ContextStack.cs
- XNodeValidator.cs
- MembershipValidatePasswordEventArgs.cs
- PanelStyle.cs
- ResourceProviderFactory.cs
- SoapCodeExporter.cs
- AssemblyInfo.cs
- translator.cs
- LinkClickEvent.cs
- baseaxisquery.cs
- ImageFormatConverter.cs
- PropertySegmentSerializer.cs
- Soap.cs
- Storyboard.cs
- DelayedRegex.cs
- RuntimeConfigurationRecord.cs
- Header.cs
- XmlSchemaNotation.cs
- MultipleViewProviderWrapper.cs
- ColorAnimationBase.cs
- MILUtilities.cs
- LocalizabilityAttribute.cs
- SerializableReadOnlyDictionary.cs
- InvalidWMPVersionException.cs
- ImageListUtils.cs
- Module.cs
- RuntimeConfigurationRecord.cs
- DataGridViewRow.cs
- OutputCacheSection.cs
- XmlQueryType.cs
- ReadOnlyAttribute.cs
- TextEndOfSegment.cs
- WSDualHttpBindingElement.cs
- MetadataAssemblyHelper.cs
- GlyphElement.cs
- ObjectCacheHost.cs
- TreeViewHitTestInfo.cs
- CompilerScope.Storage.cs
- IdentityHolder.cs
- SystemNetworkInterface.cs
- XmlHierarchicalDataSourceView.cs
- PngBitmapEncoder.cs
- BitHelper.cs
- webproxy.cs
- ParamArrayAttribute.cs
- ProcessManager.cs
- CodeTypeOfExpression.cs
- ZipIOExtraFieldElement.cs
- AnnotationHelper.cs
- ArrayElementGridEntry.cs
- DBCSCodePageEncoding.cs
- XPathNavigator.cs
- CqlLexerHelpers.cs
- sqlnorm.cs
- HandleRef.cs
- WeakHashtable.cs
- XmlWriterSettings.cs
- ListDictionaryInternal.cs
- XmlAtomErrorReader.cs
- CustomCategoryAttribute.cs
- DesignTimeParseData.cs
- ButtonPopupAdapter.cs
- EmptyEnumerable.cs
- ViewStateException.cs
- ObjectTypeMapping.cs
- ToggleButton.cs
- ByteStreamMessageEncoder.cs
- DesignerForm.cs
- NativeCompoundFileAPIs.cs
- SkipStoryboardToFill.cs
- BooleanAnimationBase.cs
- ProfilePropertyNameValidator.cs
- UIAgentMonitor.cs
- ResumeStoryboard.cs
- BevelBitmapEffect.cs
- SafeReversePInvokeHandle.cs
- ExtendLockCommand.cs
- SpecialNameAttribute.cs
- securitymgrsite.cs
- SqlProvider.cs
- CompilationRelaxations.cs
- ExternalException.cs
- ChannelServices.cs
- PieceNameHelper.cs
- ListViewSelectEventArgs.cs
- FastEncoderWindow.cs
- InputReferenceExpression.cs
- BitArray.cs
- CommentEmitter.cs
- Typeface.cs
- DataKey.cs
- WebPartExportVerb.cs
- TypeUtil.cs
- ReplacementText.cs
- SqlAliaser.cs
- UIPermission.cs