Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Advanced / ImageFormatConverter.cs / 1 / ImageFormatConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Drawing.Imaging; ////// /// ImageFormatConverter is a class that can be used to convert /// colors from one data type to another. Access this /// class through the TypeDescriptor. /// public class ImageFormatConverter : TypeConverter { private StandardValuesCollection values; ////// /// public ImageFormatConverter() { } ///[To be supplied.] ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { string strValue = value as string; if (strValue != null) { string text = strValue.Trim(); PropertyInfo[] props = GetProperties(); for (int i = 0; i < props.Length; i++) { PropertyInfo prop = props[i]; if (string.Equals(prop.Name, text, StringComparison.OrdinalIgnoreCase)) { object[] tempIndex = null; return prop.GetValue(null, tempIndex); } } } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (value is ImageFormat) { PropertyInfo targetProp = null; PropertyInfo[] props = GetProperties(); foreach(PropertyInfo p in props) { if (p.GetValue(null, null).Equals(value)) { targetProp = p; break; } } if (targetProp != null) { if (destinationType == typeof(string)) { return targetProp.Name; } else if (destinationType == typeof(InstanceDescriptor)) { return new InstanceDescriptor(targetProp, null); } } } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves the properties for the available image formats. /// private PropertyInfo[] GetProperties() { return typeof(ImageFormat).GetProperties(BindingFlags.Static | BindingFlags.Public); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { ArrayList list = new ArrayList(); PropertyInfo[] props = GetProperties(); for (int i = 0; i < props.Length; i++) { PropertyInfo prop = props[i]; object[] tempIndex = null; Debug.Assert(prop.GetValue(null, tempIndex) != null, "Property " + prop.Name + " returned NULL"); list.Add(prop.GetValue(null, tempIndex)); } values = new StandardValuesCollection(list.ToArray()); } return values; } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
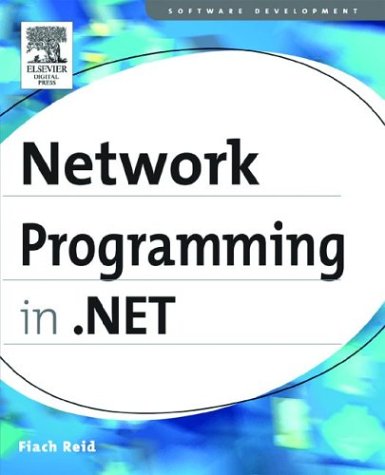
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorTranslator.cs
- ManipulationCompletedEventArgs.cs
- Accessible.cs
- ListBoxItem.cs
- TabletDeviceInfo.cs
- MessageAction.cs
- ArcSegment.cs
- HelpFileFileNameEditor.cs
- ObjectListItem.cs
- DataRelationPropertyDescriptor.cs
- HashLookup.cs
- Control.cs
- ProfileService.cs
- SchemaCollectionCompiler.cs
- ApplicationHost.cs
- ClientTargetCollection.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- XPathParser.cs
- SqlDataSourceView.cs
- WorkflowMarkupSerializationManager.cs
- Mutex.cs
- translator.cs
- PointHitTestResult.cs
- Converter.cs
- SpellerStatusTable.cs
- CodeDelegateInvokeExpression.cs
- TreePrinter.cs
- ContentFilePart.cs
- MessageLogTraceRecord.cs
- Operand.cs
- Activator.cs
- UInt32Converter.cs
- SqlTypeConverter.cs
- StoreAnnotationsMap.cs
- ColumnWidthChangedEvent.cs
- UInt64.cs
- UnsafeNativeMethods.cs
- SmiMetaData.cs
- HttpCapabilitiesBase.cs
- TableLayoutSettings.cs
- WebPartConnectVerb.cs
- PropertyInformation.cs
- SchemaImporter.cs
- DbProviderManifest.cs
- ExpressionBuilderCollection.cs
- ArgumentNullException.cs
- SynchronizedDispatch.cs
- MiniAssembly.cs
- Double.cs
- WorkflowItemPresenter.cs
- ImageSourceConverter.cs
- Span.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- RoleGroup.cs
- CacheHelper.cs
- XmlArrayItemAttribute.cs
- XPathNavigatorKeyComparer.cs
- SqlTrackingQuery.cs
- UnknownBitmapEncoder.cs
- EntitySqlQueryState.cs
- EdmType.cs
- DocumentApplicationJournalEntry.cs
- Variant.cs
- ObjectSecurity.cs
- httpapplicationstate.cs
- FontSizeConverter.cs
- AnimatedTypeHelpers.cs
- PriorityQueue.cs
- Int64Converter.cs
- CompositeCollection.cs
- DataConnectionHelper.cs
- DateTimeOffset.cs
- Rotation3DAnimation.cs
- ComponentResourceKeyConverter.cs
- LicenseContext.cs
- AutoResizedEvent.cs
- FontCacheUtil.cs
- SQLInt32.cs
- StackBuilderSink.cs
- BCryptSafeHandles.cs
- BitConverter.cs
- SettingsBindableAttribute.cs
- NumericUpDownAccelerationCollection.cs
- IImplicitResourceProvider.cs
- DoubleStorage.cs
- BmpBitmapDecoder.cs
- SecurityDescriptor.cs
- ClientViaElement.cs
- AtomContentProperty.cs
- _TLSstream.cs
- ProgressChangedEventArgs.cs
- ReadOnlyObservableCollection.cs
- MaskedTextProvider.cs
- DataControlPagerLinkButton.cs
- ListViewHitTestInfo.cs
- FileDataSourceCache.cs
- TableAutomationPeer.cs
- ManagementEventArgs.cs
- __FastResourceComparer.cs
- baseaxisquery.cs