Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / GraphicsContext.cs / 1 / GraphicsContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.Drawing.Drawing2D; using System.Diagnostics; ////// Contains information about the context of a Graphics object. /// internal class GraphicsContext : IDisposable { ////// The state that identifies the context. /// private int contextState; ////// The context's translate transform. /// private PointF transformOffset; ////// The context's clip region. /// private Region clipRegion; ////// The next context up the stack. /// private GraphicsContext nextContext; ////// The previous context down the stack. /// private GraphicsContext prevContext; ////// Flags that determines whether the context was created for a Graphics.Save() operation. /// This kind of contexts are cumulative across subsequent Save() calls so the top context /// info is cumulative. This is not the same for contexts created for a Graphics.BeginContainer() /// operation, in this case the new context information is reset. See Graphics.BeginContainer() /// and Graphics.Save() for more information. /// bool isCumulative; ////// Private constructor disallowed. /// private GraphicsContext() { } public GraphicsContext(Graphics g) { Matrix transform = g.Transform; if (!transform.IsIdentity) { float[] elements = transform.Elements; this.transformOffset.X = elements[4]; this.transformOffset.Y = elements[5]; } transform.Dispose(); Region clip = g.Clip; if (clip.IsInfinite(g)) { clip.Dispose(); } else { this.clipRegion = clip; } } ////// Disposes this and all contexts up the stack. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes this and all contexts up the stack. /// public void Dispose(bool disposing) { if (this.nextContext != null) { // Dispose all contexts up the stack since they are relative to this one and its state will be invalid. this.nextContext.Dispose(); this.nextContext = null; } if (this.clipRegion != null) { this.clipRegion.Dispose(); this.clipRegion = null; } } ////// The state id representing the GraphicsContext. /// public int State { get { return this.contextState; } set { this.contextState = value; } } ////// The translate transform in the GraphicsContext. /// public PointF TransformOffset { get { return this.transformOffset; } } ////// The clipping region the GraphicsContext. /// public Region Clip { get { return this.clipRegion; } } ////// The next GraphicsContext object in the stack. /// public GraphicsContext Next { get { return this.nextContext; } set { this.nextContext = value; } } ////// The previous GraphicsContext object in the stack. /// public GraphicsContext Previous { get { return this.prevContext; } set { this.prevContext = value; } } ////// Determines whether this context is cumulative or not. See filed for more info. /// public bool IsCumulative { get { return this.isCumulative; } set { this.isCumulative = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.Drawing.Drawing2D; using System.Diagnostics; ////// Contains information about the context of a Graphics object. /// internal class GraphicsContext : IDisposable { ////// The state that identifies the context. /// private int contextState; ////// The context's translate transform. /// private PointF transformOffset; ////// The context's clip region. /// private Region clipRegion; ////// The next context up the stack. /// private GraphicsContext nextContext; ////// The previous context down the stack. /// private GraphicsContext prevContext; ////// Flags that determines whether the context was created for a Graphics.Save() operation. /// This kind of contexts are cumulative across subsequent Save() calls so the top context /// info is cumulative. This is not the same for contexts created for a Graphics.BeginContainer() /// operation, in this case the new context information is reset. See Graphics.BeginContainer() /// and Graphics.Save() for more information. /// bool isCumulative; ////// Private constructor disallowed. /// private GraphicsContext() { } public GraphicsContext(Graphics g) { Matrix transform = g.Transform; if (!transform.IsIdentity) { float[] elements = transform.Elements; this.transformOffset.X = elements[4]; this.transformOffset.Y = elements[5]; } transform.Dispose(); Region clip = g.Clip; if (clip.IsInfinite(g)) { clip.Dispose(); } else { this.clipRegion = clip; } } ////// Disposes this and all contexts up the stack. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes this and all contexts up the stack. /// public void Dispose(bool disposing) { if (this.nextContext != null) { // Dispose all contexts up the stack since they are relative to this one and its state will be invalid. this.nextContext.Dispose(); this.nextContext = null; } if (this.clipRegion != null) { this.clipRegion.Dispose(); this.clipRegion = null; } } ////// The state id representing the GraphicsContext. /// public int State { get { return this.contextState; } set { this.contextState = value; } } ////// The translate transform in the GraphicsContext. /// public PointF TransformOffset { get { return this.transformOffset; } } ////// The clipping region the GraphicsContext. /// public Region Clip { get { return this.clipRegion; } } ////// The next GraphicsContext object in the stack. /// public GraphicsContext Next { get { return this.nextContext; } set { this.nextContext = value; } } ////// The previous GraphicsContext object in the stack. /// public GraphicsContext Previous { get { return this.prevContext; } set { this.prevContext = value; } } ////// Determines whether this context is cumulative or not. See filed for more info. /// public bool IsCumulative { get { return this.isCumulative; } set { this.isCumulative = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
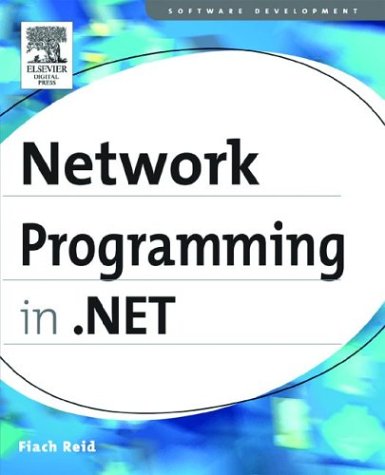
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostingEnvironmentSection.cs
- cache.cs
- NameValueSectionHandler.cs
- AnimationException.cs
- SqlCachedBuffer.cs
- WCFBuildProvider.cs
- DataObjectCopyingEventArgs.cs
- MsmqAppDomainProtocolHandler.cs
- HttpRequest.cs
- SplineQuaternionKeyFrame.cs
- MbpInfo.cs
- ResolveNameEventArgs.cs
- BindingManagerDataErrorEventArgs.cs
- ItemChangedEventArgs.cs
- HyperLinkStyle.cs
- LoginNameDesigner.cs
- QilInvokeLateBound.cs
- _Semaphore.cs
- UnsafeNativeMethods.cs
- TransferMode.cs
- FormsIdentity.cs
- FolderBrowserDialogDesigner.cs
- SoapMessage.cs
- BitmapDecoder.cs
- PaintValueEventArgs.cs
- MessageQueue.cs
- BasicHttpSecurityElement.cs
- TranslateTransform3D.cs
- WinFormsComponentEditor.cs
- ThreadSafeList.cs
- DiscoveryDocumentSearchPattern.cs
- ObjectDataSourceFilteringEventArgs.cs
- DocumentPage.cs
- DrawingContext.cs
- EqualityComparer.cs
- SqlBuffer.cs
- ResourceType.cs
- UpdatePanel.cs
- TPLETWProvider.cs
- LinkConverter.cs
- ProfileSettingsCollection.cs
- InputLanguageSource.cs
- DeliveryStrategy.cs
- CollectionViewGroupRoot.cs
- CompensationParticipant.cs
- ImmutablePropertyDescriptorGridEntry.cs
- InputEventArgs.cs
- NullReferenceException.cs
- PersistenceTypeAttribute.cs
- connectionpool.cs
- XmlWriterSettings.cs
- StreamResourceInfo.cs
- FamilyCollection.cs
- ObfuscationAttribute.cs
- SafeFileMappingHandle.cs
- DesigntimeLicenseContextSerializer.cs
- WindowsProgressbar.cs
- Function.cs
- IDReferencePropertyAttribute.cs
- IntPtr.cs
- DataGridParentRows.cs
- TextBoxAutoCompleteSourceConverter.cs
- RequestQueryProcessor.cs
- ManifestResourceInfo.cs
- EncodingDataItem.cs
- SerializationSectionGroup.cs
- Geometry.cs
- DataSourceProvider.cs
- ArgumentOutOfRangeException.cs
- ResourceReferenceExpressionConverter.cs
- HwndStylusInputProvider.cs
- IItemContainerGenerator.cs
- WorkflowTransactionOptions.cs
- StrokeSerializer.cs
- MouseBinding.cs
- DataGridItemCollection.cs
- NameNode.cs
- BufferedReceiveElement.cs
- GridView.cs
- ObjectItemCachedAssemblyLoader.cs
- BamlVersionHeader.cs
- ParallelTimeline.cs
- PreApplicationStartMethodAttribute.cs
- TiffBitmapEncoder.cs
- _WebProxyDataBuilder.cs
- ToolTipService.cs
- ConfigurationManagerHelperFactory.cs
- MenuItemBinding.cs
- SQLBytes.cs
- input.cs
- CryptoHelper.cs
- ThumbButtonInfo.cs
- BuildProviderAppliesToAttribute.cs
- ExtensionDataReader.cs
- ToolStripMenuItem.cs
- ParameterModifier.cs
- EventSetter.cs
- DataTableMapping.cs
- GridEntry.cs
- SafeRightsManagementQueryHandle.cs