Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Shared / MS / Win32 / SafeSystemMetrics.cs / 1 / SafeSystemMetrics.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2004 // // File: SafeSystemMetrics.cs // This class is copied from the system metrics class in frameworks. The // reason it exists is to consolidate all system metric calls through one layer // so that maintenance from a security stand point gets easier. We will add // mertrics on a need basis. The caching code is removed since the original calls // that were moved here do not rely on caching. If there is a percieved perf. problem // we can work on enabling this. //----------------------------------------------------------------------------- using System; using System.Collections; using System.Runtime.InteropServices; using System.Windows.Media; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; using MS.Internal.PresentationCore; namespace MS.Win32 { ////// Contains properties that are queries into the system's various settings. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal sealed class SafeSystemMetrics { private SafeSystemMetrics() { } #if !PRESENTATION_CORE ////// Maps to SM_CXVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenWidth { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXVIRTUALSCREEN); } } ////// Maps to SM_CYVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenHeight { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYVIRTUALSCREEN); } } #endif //end !PRESENTATIONCORE ////// Maps to SM_CXDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXDOUBLECLK); } } ////// Maps to SM_CYDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYDOUBLECLK); } } ////// Maps to SM_CXDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXDRAG); } } ////// Maps to SM_CYDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYDRAG); } } ////// Is an IMM enabled ? Maps to SM_IMMENABLED /// //////Critical - calls a method that performs an elevation. /// TreatAsSafe - data is considered safe to expose. /// internal static bool IsImmEnabled { [SecurityCritical, SecurityTreatAsSafe] get { return (UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_IMMENABLED) != 0); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2004 // // File: SafeSystemMetrics.cs // This class is copied from the system metrics class in frameworks. The // reason it exists is to consolidate all system metric calls through one layer // so that maintenance from a security stand point gets easier. We will add // mertrics on a need basis. The caching code is removed since the original calls // that were moved here do not rely on caching. If there is a percieved perf. problem // we can work on enabling this. //----------------------------------------------------------------------------- using System; using System.Collections; using System.Runtime.InteropServices; using System.Windows.Media; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; using MS.Internal.PresentationCore; namespace MS.Win32 { ////// Contains properties that are queries into the system's various settings. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal sealed class SafeSystemMetrics { private SafeSystemMetrics() { } #if !PRESENTATION_CORE ////// Maps to SM_CXVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenWidth { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXVIRTUALSCREEN); } } ////// Maps to SM_CYVIRTUALSCREEN /// ////// TreatAsSafe --There exists a demand /// Security Critical -- Calling UnsafeNativeMethods /// internal static int VirtualScreenHeight { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnmanagedCode(); return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYVIRTUALSCREEN); } } #endif //end !PRESENTATIONCORE ////// Maps to SM_CXDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXDOUBLECLK); } } ////// Maps to SM_CYDOUBLECLK /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DoubleClickDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYDOUBLECLK); } } ////// Maps to SM_CXDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaX { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CXDRAG); } } ////// Maps to SM_CYDRAG /// ////// TreatAsSafe --This data is safe to expose /// Security Critical -- Calling UnsafeNativeMethods /// internal static int DragDeltaY { [SecurityCritical, SecurityTreatAsSafe] get { return UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_CYDRAG); } } ////// Is an IMM enabled ? Maps to SM_IMMENABLED /// //////Critical - calls a method that performs an elevation. /// TreatAsSafe - data is considered safe to expose. /// internal static bool IsImmEnabled { [SecurityCritical, SecurityTreatAsSafe] get { return (UnsafeNativeMethods.GetSystemMetrics(NativeMethods.SM_IMMENABLED) != 0); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
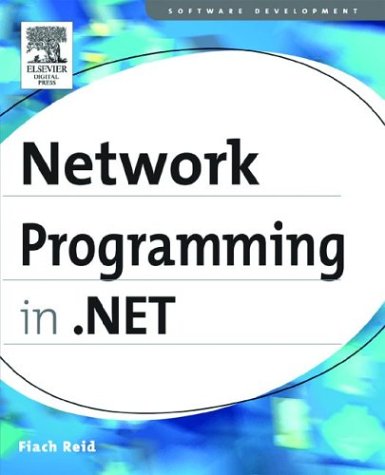
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeometryHitTestParameters.cs
- MD5.cs
- StorageEntitySetMapping.cs
- WorkflowService.cs
- PropertyGridEditorPart.cs
- InvalidateEvent.cs
- StatusBarAutomationPeer.cs
- ScriptResourceInfo.cs
- FrameworkElementFactoryMarkupObject.cs
- DbCommandTree.cs
- SqlCommandBuilder.cs
- DummyDataSource.cs
- ConfigXmlAttribute.cs
- DataGridTableStyleMappingNameEditor.cs
- PostBackTrigger.cs
- StrokeCollection2.cs
- DiscoveryRequestHandler.cs
- TypeSystem.cs
- ExtensionQuery.cs
- ScriptRef.cs
- TableDetailsCollection.cs
- PolygonHotSpot.cs
- NTAccount.cs
- AbsoluteQuery.cs
- URLAttribute.cs
- HostedHttpContext.cs
- WorkItem.cs
- _SafeNetHandles.cs
- KeyFrames.cs
- EmptyCollection.cs
- TableItemPatternIdentifiers.cs
- DataGridLength.cs
- MethodRental.cs
- SystemResourceKey.cs
- SecurityDocument.cs
- ReaderOutput.cs
- handlecollector.cs
- ExpressionValueEditor.cs
- AllMembershipCondition.cs
- MetricEntry.cs
- ValidationEventArgs.cs
- SymmetricKeyWrap.cs
- OleDbCommand.cs
- ToolStripItemEventArgs.cs
- WebPartCancelEventArgs.cs
- GradientStopCollection.cs
- TcpHostedTransportConfiguration.cs
- FrameworkRichTextComposition.cs
- TileModeValidation.cs
- MsmqIntegrationBindingElement.cs
- WebPartRestoreVerb.cs
- IconConverter.cs
- InstanceCreationEditor.cs
- ResourceProviderFactory.cs
- ResXResourceSet.cs
- path.cs
- SafeLocalMemHandle.cs
- FixedSOMFixedBlock.cs
- UserUseLicenseDictionaryLoader.cs
- PropertyNames.cs
- JoinGraph.cs
- DockingAttribute.cs
- ItemTypeToolStripMenuItem.cs
- ToolboxCategoryItems.cs
- UniqueConstraint.cs
- OrderedHashRepartitionEnumerator.cs
- DocumentPaginator.cs
- CfgArc.cs
- MouseWheelEventArgs.cs
- CodeTypeReferenceExpression.cs
- CompilerError.cs
- QueryOperator.cs
- TdsParserHelperClasses.cs
- DataGridCaption.cs
- SmtpNtlmAuthenticationModule.cs
- QueryTaskGroupState.cs
- FrugalMap.cs
- SoapMessage.cs
- ZoneIdentityPermission.cs
- EventProxy.cs
- ProviderUtil.cs
- AutoResizedEvent.cs
- ItemChangedEventArgs.cs
- BoolExpression.cs
- PrimaryKeyTypeConverter.cs
- ClosableStream.cs
- RegexTypeEditor.cs
- PriorityQueue.cs
- OracleBoolean.cs
- DataPagerFieldCommandEventArgs.cs
- EntityDataSourceValidationException.cs
- SelfIssuedAuthProofToken.cs
- LocalBuilder.cs
- RelationshipManager.cs
- WebConvert.cs
- XmlUrlResolver.cs
- HttpModuleAction.cs
- ProfilePropertySettingsCollection.cs
- BamlTreeNode.cs
- NetworkInterface.cs