Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Discovery / System / ServiceModel / Discovery / AsyncOperationContext.cs / 1305376 / AsyncOperationContext.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Discovery { using System; using System.ComponentModel; using System.Runtime; using System.Threading; using System.Xml; // WARNING: This object is not thread safe. // Use SyncRoot to protect access to methods and properties as required. abstract class AsyncOperationContext { AsyncOperation asyncOperation; TimeSpan duration; bool isCompleted; int maxResults; UniqueId operationId; NullablestartTime; [Fx.Tag.SynchronizationObject()] object syncRoot; IOThreadTimer timer; object userState; internal AsyncOperationContext(UniqueId operationId, int maxResults, TimeSpan duration, object userState) { Fx.Assert(operationId != null, "The operation id must be non null."); Fx.Assert(maxResults > 0, "The maxResults parameter must be positive."); Fx.Assert(duration > TimeSpan.Zero, "The duration parameter must be positive."); this.maxResults = maxResults; this.duration = duration; this.userState = userState; this.operationId = operationId; this.syncRoot = new object(); } public AsyncOperation AsyncOperation { get { return this.asyncOperation; } set { this.asyncOperation = value; } } public TimeSpan Duration { get { return this.duration; } } public bool IsCompleted { get { return this.isCompleted; } } public bool IsSyncOperation { get { return (UserState is SyncOperationState); } } public int MaxResults { get { return this.maxResults; } } public UniqueId OperationId { get { return this.operationId; } } public object SyncRoot { get { return syncRoot; } } public object UserState { get { return this.userState; } } public Nullable StartedAt { get { return this.startTime; } } public void Complete() { this.StopTimer(); this.isCompleted = true; } public void StartTimer(Action
Link Menu
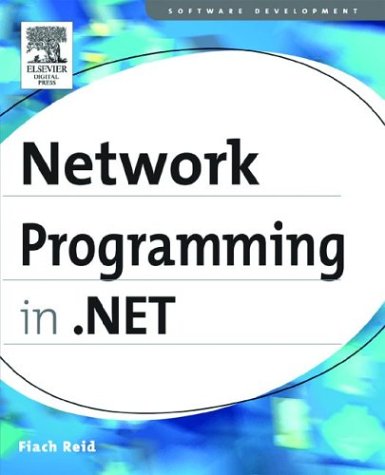
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapInteropTypes.cs
- XamlWrappingReader.cs
- FileUtil.cs
- WriteStateInfoBase.cs
- StaticTextPointer.cs
- ExplicitDiscriminatorMap.cs
- EmptyControlCollection.cs
- SmtpDateTime.cs
- BaseTemplateCodeDomTreeGenerator.cs
- NavigationFailedEventArgs.cs
- DataRelation.cs
- MulticastIPAddressInformationCollection.cs
- DetailsViewUpdatedEventArgs.cs
- NodeFunctions.cs
- ShaderRenderModeValidation.cs
- DBSchemaRow.cs
- DescendantBaseQuery.cs
- DataComponentNameHandler.cs
- AttributeXamlType.cs
- AssertFilter.cs
- FixedBufferAttribute.cs
- WebReferencesBuildProvider.cs
- IdlingCommunicationPool.cs
- ComplexTypeEmitter.cs
- SoapInteropTypes.cs
- WebPartZoneAutoFormat.cs
- Point3D.cs
- TableRow.cs
- ApplicationGesture.cs
- SourceFileBuildProvider.cs
- PropertyMetadata.cs
- DesignerListAdapter.cs
- IChannel.cs
- Column.cs
- TableProviderWrapper.cs
- NavigatorInput.cs
- BindingCollectionElement.cs
- OLEDB_Util.cs
- InvalidOperationException.cs
- SiteOfOriginContainer.cs
- OdbcHandle.cs
- PathGradientBrush.cs
- CalloutQueueItem.cs
- Rules.cs
- ArglessEventHandlerProxy.cs
- TextSegment.cs
- HttpListenerResponse.cs
- __ComObject.cs
- KerberosRequestorSecurityTokenAuthenticator.cs
- HttpConfigurationContext.cs
- XmlReflectionMember.cs
- MenuTracker.cs
- ExpressionBindings.cs
- NestedContainer.cs
- OdbcConnectionPoolProviderInfo.cs
- AutomationElement.cs
- SaveWorkflowCommand.cs
- PerformanceCounter.cs
- ProfileParameter.cs
- AuthenticationService.cs
- HttpCachePolicy.cs
- EmbeddedMailObject.cs
- OleDbErrorCollection.cs
- PerformanceCounter.cs
- RoleManagerModule.cs
- Formatter.cs
- ReadContentAsBinaryHelper.cs
- dataprotectionpermissionattribute.cs
- DataGridViewLayoutData.cs
- TreeNodeBindingDepthConverter.cs
- CompilerGlobalScopeAttribute.cs
- UrlAuthFailedErrorFormatter.cs
- ShimAsPublicXamlType.cs
- BufferedGraphics.cs
- UIElementHelper.cs
- PropertyConverter.cs
- MultipleViewPattern.cs
- BooleanConverter.cs
- xml.cs
- QilPatternVisitor.cs
- ResourceKey.cs
- ListManagerBindingsCollection.cs
- ResXResourceReader.cs
- ProxyManager.cs
- WindowProviderWrapper.cs
- NamedElement.cs
- DiffuseMaterial.cs
- ScrollPattern.cs
- PresentationTraceSources.cs
- _NestedSingleAsyncResult.cs
- WindowsToolbar.cs
- MimeImporter.cs
- GeneralTransformGroup.cs
- AdornedElementPlaceholder.cs
- PermissionListSet.cs
- AlternateView.cs
- Transform3DGroup.cs
- ProfileSettings.cs
- CryptoApi.cs
- XmlDeclaration.cs