Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / BufferedGraphics.cs / 1 / BufferedGraphics.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.ComponentModel; using System.Collections; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Text; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; ////// /// The BufferedGraphics class can be thought of as a "Token" or "Reference" to the /// buffer that a BufferedGraphicsContext creates. While a BufferedGraphics is /// outstanding, the memory associated with the buffer is locked. The general design /// is such that under normal conditions a single BufferedGraphics will be in use at /// one time for a given BufferedGraphicsContext. /// [SuppressMessage("Microsoft.Usage", "CA2216:DisposableTypesShouldDeclareFinalizer")] public sealed class BufferedGraphics : IDisposable { private Graphics bufferedGraphicsSurface; private Graphics targetGraphics; private BufferedGraphicsContext context; private IntPtr targetDC; private Point targetLoc; private Size virtualSize; private bool disposeContext; private static int rop = 0xcc0020; // RasterOp.SOURCE.GetRop(); ////// /// Internal constructor, this class is created by the BufferedGraphicsContext. /// internal BufferedGraphics(Graphics bufferedGraphicsSurface, BufferedGraphicsContext context, Graphics targetGraphics, IntPtr targetDC, Point targetLoc, Size virtualSize) { this.context = context; this.bufferedGraphicsSurface = bufferedGraphicsSurface; this.targetDC = targetDC; this.targetGraphics = targetGraphics; this.targetLoc = targetLoc; this.virtualSize = virtualSize; } ~BufferedGraphics() { Dispose(false); } ////// /// Disposes the object and releases the lock on the memory. /// public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { if (context != null) { context.ReleaseBuffer(this); if (DisposeContext) { context.Dispose(); context = null; } } if (bufferedGraphicsSurface != null) { bufferedGraphicsSurface.Dispose(); bufferedGraphicsSurface = null; } } } ////// /// Internal property - determines if we need to dispose of the Context when this is disposed /// internal bool DisposeContext { get { return disposeContext; } set { disposeContext = value; } } ////// /// Allows access to the Graphics wrapper for the buffer. /// public Graphics Graphics { get { Debug.Assert(bufferedGraphicsSurface != null, "The BufferedGraphicsSurface is null!"); return bufferedGraphicsSurface; } } ////// /// Renders the buffer to the original graphics used to allocate the buffer. /// public void Render() { if (targetGraphics != null) { Render(targetGraphics); } else { RenderInternal(new HandleRef(Graphics, targetDC), this); } } ////// /// Renders the buffer to the specified target graphics. /// public void Render(Graphics target) { if (target != null) { IntPtr targetDC = target.GetHdc(); try { RenderInternal(new HandleRef(target, targetDC), this); } finally { target.ReleaseHdcInternal(targetDC); } } } ////// /// Renders the buffer to the specified target HDC. /// public void Render(IntPtr targetDC) { IntSecurity.UnmanagedCode.Demand(); RenderInternal(new HandleRef(null, targetDC), this); } ////// /// Internal method that renders the specified buffer into the target. /// private void RenderInternal(HandleRef refTargetDC, BufferedGraphics buffer) { IntPtr sourceDC = buffer.Graphics.GetHdc(); try { SafeNativeMethods.BitBlt(refTargetDC, targetLoc.X, targetLoc.Y, virtualSize.Width, virtualSize.Height, new HandleRef(buffer.Graphics, sourceDC), 0, 0, rop); } finally { buffer.Graphics.ReleaseHdcInternal(sourceDC); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.ComponentModel; using System.Collections; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Text; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; ////// /// The BufferedGraphics class can be thought of as a "Token" or "Reference" to the /// buffer that a BufferedGraphicsContext creates. While a BufferedGraphics is /// outstanding, the memory associated with the buffer is locked. The general design /// is such that under normal conditions a single BufferedGraphics will be in use at /// one time for a given BufferedGraphicsContext. /// [SuppressMessage("Microsoft.Usage", "CA2216:DisposableTypesShouldDeclareFinalizer")] public sealed class BufferedGraphics : IDisposable { private Graphics bufferedGraphicsSurface; private Graphics targetGraphics; private BufferedGraphicsContext context; private IntPtr targetDC; private Point targetLoc; private Size virtualSize; private bool disposeContext; private static int rop = 0xcc0020; // RasterOp.SOURCE.GetRop(); ////// /// Internal constructor, this class is created by the BufferedGraphicsContext. /// internal BufferedGraphics(Graphics bufferedGraphicsSurface, BufferedGraphicsContext context, Graphics targetGraphics, IntPtr targetDC, Point targetLoc, Size virtualSize) { this.context = context; this.bufferedGraphicsSurface = bufferedGraphicsSurface; this.targetDC = targetDC; this.targetGraphics = targetGraphics; this.targetLoc = targetLoc; this.virtualSize = virtualSize; } ~BufferedGraphics() { Dispose(false); } ////// /// Disposes the object and releases the lock on the memory. /// public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { if (context != null) { context.ReleaseBuffer(this); if (DisposeContext) { context.Dispose(); context = null; } } if (bufferedGraphicsSurface != null) { bufferedGraphicsSurface.Dispose(); bufferedGraphicsSurface = null; } } } ////// /// Internal property - determines if we need to dispose of the Context when this is disposed /// internal bool DisposeContext { get { return disposeContext; } set { disposeContext = value; } } ////// /// Allows access to the Graphics wrapper for the buffer. /// public Graphics Graphics { get { Debug.Assert(bufferedGraphicsSurface != null, "The BufferedGraphicsSurface is null!"); return bufferedGraphicsSurface; } } ////// /// Renders the buffer to the original graphics used to allocate the buffer. /// public void Render() { if (targetGraphics != null) { Render(targetGraphics); } else { RenderInternal(new HandleRef(Graphics, targetDC), this); } } ////// /// Renders the buffer to the specified target graphics. /// public void Render(Graphics target) { if (target != null) { IntPtr targetDC = target.GetHdc(); try { RenderInternal(new HandleRef(target, targetDC), this); } finally { target.ReleaseHdcInternal(targetDC); } } } ////// /// Renders the buffer to the specified target HDC. /// public void Render(IntPtr targetDC) { IntSecurity.UnmanagedCode.Demand(); RenderInternal(new HandleRef(null, targetDC), this); } ////// /// Internal method that renders the specified buffer into the target. /// private void RenderInternal(HandleRef refTargetDC, BufferedGraphics buffer) { IntPtr sourceDC = buffer.Graphics.GetHdc(); try { SafeNativeMethods.BitBlt(refTargetDC, targetLoc.X, targetLoc.Y, virtualSize.Width, virtualSize.Height, new HandleRef(buffer.Graphics, sourceDC), 0, 0, rop); } finally { buffer.Graphics.ReleaseHdcInternal(sourceDC); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
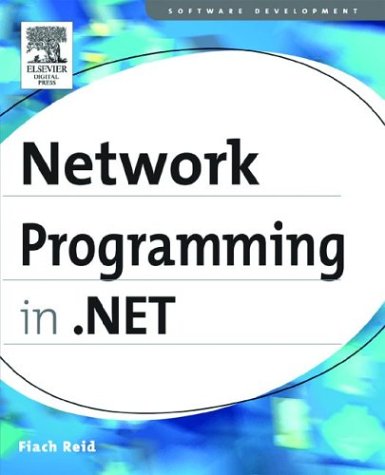
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogExtentCollection.cs
- RegexReplacement.cs
- VarRemapper.cs
- SyndicationElementExtension.cs
- MappedMetaModel.cs
- DoubleStorage.cs
- FormViewRow.cs
- SingleAnimation.cs
- InvalidOperationException.cs
- SemanticResultValue.cs
- LiteralControl.cs
- Crc32Helper.cs
- TextViewSelectionProcessor.cs
- PolicyValidationException.cs
- UIntPtr.cs
- SimpleHandlerFactory.cs
- BitmapCodecInfoInternal.cs
- AppDomainFactory.cs
- IPPacketInformation.cs
- ConfigurationSection.cs
- SqlProcedureAttribute.cs
- DependsOnAttribute.cs
- TextParagraph.cs
- RowBinding.cs
- DbExpressionRules.cs
- StructureChangedEventArgs.cs
- TextSelectionProcessor.cs
- SerializationInfo.cs
- RectKeyFrameCollection.cs
- ViewGenerator.cs
- DataGridViewCellValidatingEventArgs.cs
- InputLanguageManager.cs
- MissingSatelliteAssemblyException.cs
- LoadMessageLogger.cs
- ModuleConfigurationInfo.cs
- SocketException.cs
- X509Certificate2.cs
- CultureNotFoundException.cs
- AppDomainManager.cs
- DataSvcMapFile.cs
- XmlDictionaryReaderQuotas.cs
- DataServiceQuery.cs
- EntityDataSourceStatementEditor.cs
- NameTable.cs
- SubqueryRules.cs
- ClientSideProviderDescription.cs
- SoapIncludeAttribute.cs
- ZipPackagePart.cs
- PreviewPrintController.cs
- CommonRemoteMemoryBlock.cs
- RepeaterItemEventArgs.cs
- ButtonChrome.cs
- AssemblyInfo.cs
- NamedPermissionSet.cs
- TextEditorParagraphs.cs
- LingerOption.cs
- ReflectionUtil.cs
- ListItemCollection.cs
- XmlSchemaSubstitutionGroup.cs
- IsolationInterop.cs
- TrackingProfileDeserializationException.cs
- ValidationEventArgs.cs
- LinqMaximalSubtreeNominator.cs
- URI.cs
- ListControlDataBindingHandler.cs
- TableStyle.cs
- CfgParser.cs
- MachineKeySection.cs
- PixelFormats.cs
- XmlSiteMapProvider.cs
- CodeCastExpression.cs
- ProtocolViolationException.cs
- DecoderFallback.cs
- RawStylusInput.cs
- ImageSourceConverter.cs
- JsonFaultDetail.cs
- ReservationNotFoundException.cs
- VisualProxy.cs
- LowerCaseStringConverter.cs
- ClientRuntimeConfig.cs
- ReliableSessionElement.cs
- TreeBuilder.cs
- ADMembershipProvider.cs
- InitialServerConnectionReader.cs
- ResponseBodyWriter.cs
- HybridDictionary.cs
- DictionaryEntry.cs
- PageFunction.cs
- ContentTextAutomationPeer.cs
- WebPartDescription.cs
- XmlWhitespace.cs
- SafeMarshalContext.cs
- Propagator.Evaluator.cs
- WmlValidationSummaryAdapter.cs
- DbParameterHelper.cs
- XmlCountingReader.cs
- UserNameSecurityTokenAuthenticator.cs
- _ProxyRegBlob.cs
- InputBinding.cs
- ToolboxDataAttribute.cs