Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / TokenFactoryCredential.cs / 1 / TokenFactoryCredential.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.IO; using System.Security.Cryptography; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; internal enum TokenFactoryCredentialType { Invalid = 0, LocalCredential = -1, UserNamePasswordCredential = 1, SelfIssuedCredential = 2, X509CertificateCredential = 3, KerberosCredential = 4, } // // Summary: // Class to managed and protect credentials collected for access // to token factories. // abstract internal class TokenFactoryCredential : IDisposable { public const int NoCredential = (int)TokenFactoryCredentialType.LocalCredential; TokenFactoryCredentialType m_type; Uri m_cardId; int m_paramIndex; int m_lcid; // // Summary: // Creates an emtpy credential. // protected TokenFactoryCredential( TokenFactoryCredentialType type ) { m_type = type; } public void Dispose() { Dispose( true ); } public virtual void Dispose( bool disposing ) { } // // Summary: // Create a new instance of a TokenFactoryCredential using the specified reader. // public static TokenFactoryCredential CreateFrom( BinaryReader reader, UIAgentRequest request, int lcid ) { TokenFactoryCredential cred; TokenFactoryCredentialType type = (TokenFactoryCredentialType)reader.ReadInt32(); switch( type ) { case TokenFactoryCredentialType.LocalCredential: cred = null; break; case TokenFactoryCredentialType.UserNamePasswordCredential: cred = new UserNameTokenFactoryCredential( ); cred.Deserialize( reader ); break; case TokenFactoryCredentialType.SelfIssuedCredential: cred = new SelfIssuedTokenFactoryCredential( ); cred.Deserialize( reader ); break; case TokenFactoryCredentialType.X509CertificateCredential: cred = new X509CertificateTokenFactoryCredential( request ); cred.Deserialize( reader ); break; case TokenFactoryCredentialType.KerberosCredential: cred = new KerberosTokenFactoryCredential( ); cred.Deserialize( reader ); break; default: IDT.ThrowInvalidArgumentConditional( true, "CredentialType" ); cred = null; break; } if( null != cred ) { cred.LCID = lcid; } return cred; } // // Gets the type of credentials // public int ParameterIndex { get{ return m_paramIndex; } } // // Gets the marshaled TokenFactoryCredentialType value // public TokenFactoryCredentialType CredentialType { get{ return m_type; } } // // LCID for the language that the display token should be in // public int LCID { get { return m_lcid; } set { m_lcid = value; } } // // Get the cardid of the selected card. // public Uri CardId { get{ return m_cardId; } } // // Summary: // Populates the class data from binary form // // Arguments: // reader: The reader that contains the data to populate the class // private void Deserialize( BinaryReader reader ) { // // Byte this point, we have already read the type info, so we skip it here. // m_cardId = new Uri( Utility.DeserializeString( reader ) ); m_paramIndex = reader.ReadInt32(); DeserializeData( reader ); } protected abstract void DeserializeData( BinaryReader reader ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
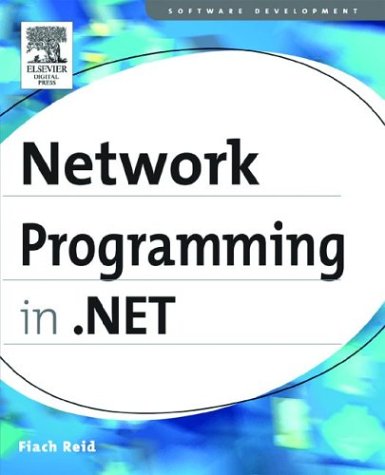
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SynchronizationLockException.cs
- PropertyToken.cs
- ResponseStream.cs
- LayoutSettings.cs
- Assert.cs
- WindowsSecurityToken.cs
- WindowsListViewItemCheckBox.cs
- DataColumnChangeEvent.cs
- MarkupCompilePass2.cs
- DomainConstraint.cs
- CallTemplateAction.cs
- PolyLineSegmentFigureLogic.cs
- safePerfProviderHandle.cs
- InputScopeManager.cs
- PrivacyNoticeElement.cs
- Cursor.cs
- VirtualPathUtility.cs
- NativeMethods.cs
- HasCopySemanticsAttribute.cs
- DataGridLength.cs
- HttpServerVarsCollection.cs
- StreamWriter.cs
- FormView.cs
- OperationCanceledException.cs
- TimeSpanStorage.cs
- Section.cs
- SafeBitVector32.cs
- RoleManagerSection.cs
- IsolatedStorageFile.cs
- BufferedGraphicsManager.cs
- PropertyPathConverter.cs
- WindowsRegion.cs
- WmlImageAdapter.cs
- WebAdminConfigurationHelper.cs
- CodeTypeOfExpression.cs
- XmlCharCheckingWriter.cs
- ResourceDictionary.cs
- CharConverter.cs
- IUnknownConstantAttribute.cs
- HeaderedItemsControl.cs
- FunctionUpdateCommand.cs
- XmlConverter.cs
- RTLAwareMessageBox.cs
- EndOfStreamException.cs
- XmlSchemaImport.cs
- TextEditor.cs
- SafeHandles.cs
- ProcessModule.cs
- NetNamedPipeBindingCollectionElement.cs
- DockAndAnchorLayout.cs
- AnnotationResource.cs
- PopupRootAutomationPeer.cs
- UInt32Storage.cs
- AutomationFocusChangedEventArgs.cs
- OleDbMetaDataFactory.cs
- RectValueSerializer.cs
- BindableTemplateBuilder.cs
- SmtpCommands.cs
- Pen.cs
- ArgIterator.cs
- Frame.cs
- XPathNodeList.cs
- DecimalConstantAttribute.cs
- PolicyStatement.cs
- DataGridCaption.cs
- EntityParameterCollection.cs
- ImageInfo.cs
- WebServicesSection.cs
- Model3DGroup.cs
- CompositeFontInfo.cs
- IsolatedStoragePermission.cs
- TransactionFilter.cs
- ServiceContractListItemList.cs
- TextTreeTextNode.cs
- TextServicesPropertyRanges.cs
- XmlCodeExporter.cs
- TemplateField.cs
- Odbc32.cs
- DataGridViewAutoSizeModeEventArgs.cs
- ReflectionHelper.cs
- NetworkAddressChange.cs
- CompilationSection.cs
- ToolStripButton.cs
- BinHexDecoder.cs
- DataObjectAttribute.cs
- PersonalizationAdministration.cs
- PrintEvent.cs
- ImageSource.cs
- ButtonBaseAutomationPeer.cs
- SetIterators.cs
- FtpWebRequest.cs
- _Semaphore.cs
- JsonCollectionDataContract.cs
- ChtmlPageAdapter.cs
- ClientData.cs
- RewritingProcessor.cs
- SessionEndingCancelEventArgs.cs
- URLMembershipCondition.cs
- UnsafeCollabNativeMethods.cs
- RMEnrollmentPage1.cs