Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / ImageInfo.cs / 1305376 / ImageInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Threading; using System; using System.Diagnostics; using System.Drawing.Imaging; ////// Animates one or more images that have time-based frames. /// This file contains the nested ImageInfo class - See ImageAnimator.cs for the definition of the outer class. /// public sealed partial class ImageAnimator { ////// ImageAnimator nested helper class used to store extra image state info. /// private class ImageInfo { const int PropertyTagFrameDelay = 0x5100; Image image; int frame; int frameCount; bool frameDirty; bool animated; EventHandler onFrameChangedHandler; int[] frameDelay; int frameTimer; ////// public ImageInfo(Image image) { this.image = image; animated = ImageAnimator.CanAnimate(image); if (animated) { frameCount = image.GetFrameCount(FrameDimension.Time); PropertyItem frameDelayItem = image.GetPropertyItem(PropertyTagFrameDelay); // If the image does not have a frame delay, we just return 0. // if (frameDelayItem != null) { // Convert the frame delay from byte[] to int // byte[] values = frameDelayItem.Value; Debug.Assert(values.Length == 4 * FrameCount, "PropertyItem has invalid value byte array"); frameDelay = new int[FrameCount]; for (int i=0; i < FrameCount; ++i) { frameDelay[i] = values[i * 4] + 256 * values[i * 4 + 1] + 256 * 256 * values[i * 4 + 2] + 256 * 256 * 256 * values[i * 4 + 3]; } } } else { frameCount = 1; } if (frameDelay == null) { frameDelay = new int[FrameCount]; } } ////// Whether the image supports animation. /// public bool Animated { get { return animated; } } ////// The current frame. /// public int Frame { get { return frame; } set { if (frame != value) { if (value < 0 || value >= FrameCount) { throw new ArgumentException(SR.GetString(SR.InvalidFrame), "value"); } if (Animated) { frame = value; frameDirty = true; OnFrameChanged(EventArgs.Empty); } } } } ////// The current frame has not been updated. /// public bool FrameDirty { get { return frameDirty; } } ////// public EventHandler FrameChangedHandler { get { return onFrameChangedHandler; } set { onFrameChangedHandler = value; } } ////// The number of frames in the image. /// public int FrameCount { get { return frameCount; } } ////// The delay associated with the frame at the specified index. /// public int FrameDelay(int frame) { return frameDelay[frame]; } ////// internal int FrameTimer { get { return frameTimer; } set { frameTimer = value; } } ////// The image this object wraps. /// internal Image Image { get { return image; } } ////// Selects the current frame as the active frame in the image. /// internal void UpdateFrame() { if (frameDirty) { image.SelectActiveFrame(FrameDimension.Time, Frame); frameDirty = false; } } ////// Raises the FrameChanged event. /// protected void OnFrameChanged(EventArgs e) { if( this.onFrameChangedHandler != null ){ this.onFrameChangedHandler(image, e); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
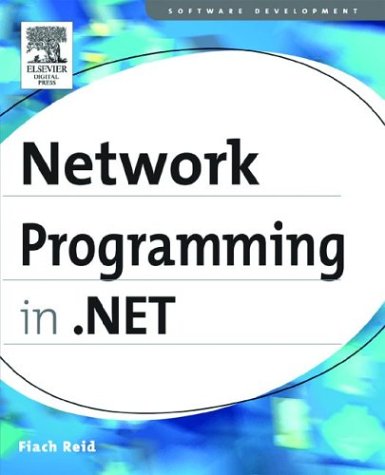
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ControlAdapter.cs
- PerformanceCounterManager.cs
- Mutex.cs
- EdmType.cs
- MarginsConverter.cs
- HttpsChannelListener.cs
- SerializationInfoEnumerator.cs
- DrawingGroupDrawingContext.cs
- ScriptManager.cs
- DataGridRowsPresenter.cs
- SqlParameterCollection.cs
- PackageFilter.cs
- AspNetSynchronizationContext.cs
- BigInt.cs
- ListChangedEventArgs.cs
- TreeChangeInfo.cs
- Point3DValueSerializer.cs
- ThemeableAttribute.cs
- ProfileParameter.cs
- SystemShuttingDownException.cs
- BitSet.cs
- ServicePointManagerElement.cs
- ExtensionSimplifierMarkupObject.cs
- Item.cs
- HttpCapabilitiesEvaluator.cs
- AppearanceEditorPart.cs
- HttpException.cs
- RangeContentEnumerator.cs
- SortExpressionBuilder.cs
- WorkItem.cs
- _AuthenticationState.cs
- CodeExporter.cs
- ClientRolePrincipal.cs
- Config.cs
- ApplicationManager.cs
- RequestCacheManager.cs
- NetCodeGroup.cs
- CatalogZone.cs
- DataPagerFieldCollection.cs
- ConnectionConsumerAttribute.cs
- SaveFileDialog.cs
- CSharpCodeProvider.cs
- TransactionTraceIdentifier.cs
- TextSpanModifier.cs
- FrameDimension.cs
- CodeDesigner.cs
- HtmlInputPassword.cs
- SimpleHandlerBuildProvider.cs
- CodeTypeReferenceCollection.cs
- RotationValidation.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- SynchronizedDispatch.cs
- MaterialGroup.cs
- EventLogEntry.cs
- MobileCategoryAttribute.cs
- CompleteWizardStep.cs
- RsaSecurityKey.cs
- FrameworkElementFactoryMarkupObject.cs
- StreamGeometryContext.cs
- LabelDesigner.cs
- BinaryCommonClasses.cs
- Formatter.cs
- SQLCharsStorage.cs
- MultipartIdentifier.cs
- ContentAlignmentEditor.cs
- DataGridDetailsPresenterAutomationPeer.cs
- BitmapMetadata.cs
- StorageRoot.cs
- BooleanExpr.cs
- GridViewCommandEventArgs.cs
- ConsoleTraceListener.cs
- HandledMouseEvent.cs
- SignatureHelper.cs
- CompilerScopeManager.cs
- FilteredReadOnlyMetadataCollection.cs
- LabelLiteral.cs
- regiisutil.cs
- ProviderCommandInfoUtils.cs
- MatrixUtil.cs
- StorageModelBuildProvider.cs
- SmtpNetworkElement.cs
- LookupNode.cs
- EntityClassGenerator.cs
- TargetControlTypeAttribute.cs
- RootNamespaceAttribute.cs
- FontStretches.cs
- httpapplicationstate.cs
- ObjectComplexPropertyMapping.cs
- ChtmlMobileTextWriter.cs
- FormViewInsertedEventArgs.cs
- Automation.cs
- StylusButtonCollection.cs
- SelectorAutomationPeer.cs
- SchemaImporter.cs
- SchemaElementLookUpTable.cs
- FontResourceCache.cs
- FrameworkElementFactory.cs
- WorkflowViewElement.cs
- ScriptIgnoreAttribute.cs
- UpdatePanel.cs