Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Net / System / Net / Sockets / TCPListener.cs / 1 / TCPListener.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Sockets { using System; using System.Net; using System.Security.Permissions; ////// public class TcpListener { IPEndPoint m_ServerSocketEP; Socket m_ServerSocket; bool m_Active; bool m_ExclusiveAddressUse; ///The ///class provide TCP services at a higher level of abstraction than the /// class. is used to create a host process that /// listens for connections from TCP clients. /// public TcpListener(IPEndPoint localEP) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "TcpListener", localEP); if (localEP == null) { throw new ArgumentNullException("localEP"); } m_ServerSocketEP = localEP; m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if(Logging.On)Logging.Exit(Logging.Sockets, this, "TcpListener", null); } ////// Initializes a new instance of the TcpListener class with the specified local /// end point. /// ////// public TcpListener(IPAddress localaddr, int port) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "TcpListener", localaddr); if (localaddr == null) { throw new ArgumentNullException("localaddr"); } if (!ValidationHelper.ValidateTcpPort(port)) { throw new ArgumentOutOfRangeException("port"); } m_ServerSocketEP = new IPEndPoint(localaddr, port); m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if(Logging.On)Logging.Exit(Logging.Sockets, this, "TcpListener", null); } // implementation picks an address for client ////// Initializes a new instance of the TcpListener class that listens to the /// specified IP address and port. /// ////// /// [Obsolete("This method has been deprecated. Please use TcpListener(IPAddress localaddr, int port) instead. http://go.microsoft.com/fwlink/?linkid=14202")] public TcpListener(int port){ if (!ValidationHelper.ValidateTcpPort(port)) throw new ArgumentOutOfRangeException("port"); m_ServerSocketEP = new IPEndPoint(IPAddress.Any, port); m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); } ////// Initiailizes a new instance of the TcpListener class /// that listens on the specified /// port. /// ////// public Socket Server { get { return m_ServerSocket; } } ////// Used by the class to provide the underlying network socket. /// ////// protected bool Active { get { return m_Active; } } ////// Used /// by the class to indicate that the listener's socket has been bound to a port /// and started listening. /// ////// public EndPoint LocalEndpoint { get { return m_Active ? m_ServerSocket.LocalEndPoint : m_ServerSocketEP; } } public bool ExclusiveAddressUse { get { return m_ServerSocket.ExclusiveAddressUse; } set{ if (m_Active) { throw new InvalidOperationException(SR.GetString(SR.net_tcplistener_mustbestopped)); } m_ServerSocket.ExclusiveAddressUse = value; m_ExclusiveAddressUse = value; } } // Start/stop the listener ////// Gets the m_Active EndPoint for the local listener socket. /// ////// public void Start() { Start((int)SocketOptionName.MaxConnections); } public void Start(int backlog) { if (backlog > (int)SocketOptionName.MaxConnections || backlog < 0) { throw new ArgumentOutOfRangeException("backlog"); } if(Logging.On)Logging.Enter(Logging.Sockets, this, "Start", null); GlobalLog.Print("TCPListener::Start()"); if (m_ServerSocket == null) throw new InvalidOperationException(SR.GetString(SR.net_InvalidSocketHandle)); //already listening if (m_Active) { if(Logging.On)Logging.Exit(Logging.Sockets, this, "Start", null); return; } m_ServerSocket.Bind(m_ServerSocketEP); m_ServerSocket.Listen(backlog); m_Active = true; if(Logging.On)Logging.Exit(Logging.Sockets, this, "Start", null); } ////// Starts listening to network requests. /// ////// public void Stop() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "Stop", null); GlobalLog.Print("TCPListener::Stop()"); if (m_ServerSocket != null) { m_ServerSocket.Close(); m_ServerSocket = null; } m_Active = false; m_ServerSocket = new Socket(m_ServerSocketEP.AddressFamily, SocketType.Stream, ProtocolType.Tcp); if (m_ExclusiveAddressUse) { m_ServerSocket.ExclusiveAddressUse = true; } if(Logging.On)Logging.Exit(Logging.Sockets, this, "Stop", null); } // Determine if there are pending connections ////// Closes the network connection. /// ////// public bool Pending() { if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); return m_ServerSocket.Poll(0, SelectMode.SelectRead); } // Accept the first pending connection ////// Determine if there are pending connection requests. /// ////// public Socket AcceptSocket() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "AcceptSocket", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); Socket socket = m_ServerSocket.Accept(); if(Logging.On)Logging.Exit(Logging.Sockets, this, "AcceptSocket", socket); return socket; } // UEUE ////// Accepts a pending connection request. /// ////// public TcpClient AcceptTcpClient() { if(Logging.On)Logging.Enter(Logging.Sockets, this, "AcceptTcpClient", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); Socket acceptedSocket = m_ServerSocket.Accept(); TcpClient returnValue = new TcpClient(acceptedSocket); if(Logging.On)Logging.Exit(Logging.Sockets, this, "AcceptTcpClient", returnValue); return returnValue; } //methods [HostProtection(ExternalThreading=true)] public IAsyncResult BeginAcceptSocket(AsyncCallback callback, object state) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "BeginAcceptSocket", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); IAsyncResult result = m_ServerSocket.BeginAccept(callback,state); if(Logging.On)Logging.Exit(Logging.Sockets, this, "BeginAcceptSocket", null); return result; } public Socket EndAcceptSocket(IAsyncResult asyncResult){ if(Logging.On)Logging.Enter(Logging.Sockets, this, "EndAcceptSocket", null); if (asyncResult == null) { throw new ArgumentNullException("asyncResult"); } LazyAsyncResult lazyResult = asyncResult as LazyAsyncResult; Socket asyncSocket = lazyResult == null ? null : lazyResult.AsyncObject as Socket; if (asyncSocket == null) { throw new ArgumentException(SR.GetString(SR.net_io_invalidasyncresult), "asyncResult"); } // This will throw ObjectDisposedException if Stop() has been called. Socket socket = asyncSocket.EndAccept(asyncResult); if(Logging.On)Logging.Exit(Logging.Sockets, this, "EndAcceptSocket", socket); return socket; } [HostProtection(ExternalThreading=true)] public IAsyncResult BeginAcceptTcpClient(AsyncCallback callback, object state) { if(Logging.On)Logging.Enter(Logging.Sockets, this, "BeginAcceptTcpClient", null); if (!m_Active) throw new InvalidOperationException(SR.GetString(SR.net_stopped)); IAsyncResult result = m_ServerSocket.BeginAccept(callback,state); if(Logging.On)Logging.Exit(Logging.Sockets, this, "BeginAcceptTcpClient", null); return result; } public TcpClient EndAcceptTcpClient(IAsyncResult asyncResult){ if(Logging.On)Logging.Enter(Logging.Sockets, this, "EndAcceptTcpClient", null); if (asyncResult == null) { throw new ArgumentNullException("asyncResult"); } LazyAsyncResult lazyResult = asyncResult as LazyAsyncResult; Socket asyncSocket = lazyResult == null ? null : lazyResult.AsyncObject as Socket; if (asyncSocket == null) { throw new ArgumentException(SR.GetString(SR.net_io_invalidasyncresult), "asyncResult"); } // This will throw ObjectDisposedException if Stop() has been called. Socket socket = asyncSocket.EndAccept(asyncResult); if(Logging.On)Logging.Exit(Logging.Sockets, this, "EndAcceptTcpClient", socket); return new TcpClient(socket); } }; // class TcpListener } // namespace System.Net.Sockets[To be supplied.] ///
Link Menu
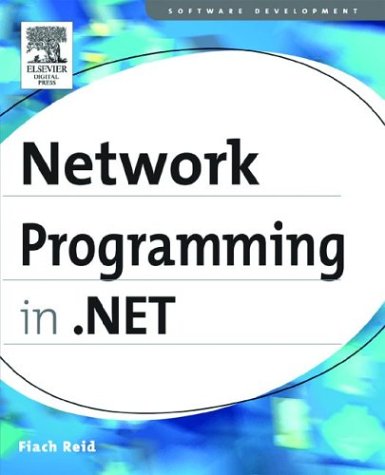
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Vector3DConverter.cs
- WebChannelFactory.cs
- EnumerableRowCollectionExtensions.cs
- TraceSection.cs
- LinqDataSource.cs
- FontNameEditor.cs
- DbFunctionCommandTree.cs
- DataGridViewComboBoxColumn.cs
- OutputChannelBinder.cs
- WindowsToolbar.cs
- InternalBufferOverflowException.cs
- AliasedSlot.cs
- DSASignatureDeformatter.cs
- PrivilegedConfigurationManager.cs
- NodeLabelEditEvent.cs
- DependencyPropertyDescriptor.cs
- DesignRelation.cs
- SecurityResources.cs
- MetadataProperty.cs
- SymLanguageType.cs
- WindowHideOrCloseTracker.cs
- OneOfElement.cs
- PrePrepareMethodAttribute.cs
- StylusDownEventArgs.cs
- Rotation3D.cs
- PageTheme.cs
- LinqDataSourceEditData.cs
- SqlConnectionFactory.cs
- ExternalException.cs
- XPathCompiler.cs
- IfAction.cs
- TablePattern.cs
- JsonQNameDataContract.cs
- Utility.cs
- WebSysDescriptionAttribute.cs
- CryptoKeySecurity.cs
- GridPattern.cs
- ConfigXmlReader.cs
- AgileSafeNativeMemoryHandle.cs
- KeyInstance.cs
- MetadataProperty.cs
- UdpMessageProperty.cs
- AutoResizedEvent.cs
- StreamUpdate.cs
- xmlfixedPageInfo.cs
- InstanceDataCollection.cs
- ActivationArguments.cs
- CoTaskMemHandle.cs
- PropertyPathConverter.cs
- DrawingContextDrawingContextWalker.cs
- HMACSHA384.cs
- RegexCaptureCollection.cs
- RijndaelCryptoServiceProvider.cs
- ObjectQueryProvider.cs
- ScrollChrome.cs
- AxImporter.cs
- DataControlButton.cs
- ThumbButtonInfo.cs
- IIS7WorkerRequest.cs
- HashCodeCombiner.cs
- VersionedStreamOwner.cs
- ParserOptions.cs
- CallbackValidatorAttribute.cs
- altserialization.cs
- storepermissionattribute.cs
- CssTextWriter.cs
- CreateUserWizard.cs
- tibetanshape.cs
- printdlgexmarshaler.cs
- StructuralObject.cs
- SoapIgnoreAttribute.cs
- WindowsTreeView.cs
- HttpCacheParams.cs
- FormViewInsertEventArgs.cs
- RepeaterItemCollection.cs
- DesignerSerializationOptionsAttribute.cs
- SqlClientWrapperSmiStream.cs
- Message.cs
- _Win32.cs
- XmlParser.cs
- RemoteWebConfigurationHost.cs
- Rijndael.cs
- VectorCollectionValueSerializer.cs
- SchemaImporterExtensionElement.cs
- Memoizer.cs
- LocalizableResourceBuilder.cs
- PresentationTraceSources.cs
- MailHeaderInfo.cs
- PatternMatcher.cs
- TdsEnums.cs
- WindowsPrincipal.cs
- TextModifierScope.cs
- DataGridViewImageColumn.cs
- HtmlFormParameterReader.cs
- BulletedList.cs
- _LoggingObject.cs
- EventLevel.cs
- Stroke.cs
- SelectionProcessor.cs
- XmlSecureResolver.cs