Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Shell / ThumbButtonInfo.cs / 1305600 / ThumbButtonInfo.cs
/**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace System.Windows.Shell { using System; using System.Diagnostics; using System.Windows; using System.Windows.Input; using System.Windows.Media; public sealed class ThumbButtonInfo : Freezable, ICommandSource { // The CommandManager keeps weak references on delegates // hooked for CanExecuteChanged. We need to explicitly keep a // strong reference, because just adding an instance method causes // the auto-generated handler to be GC'd. private EventHandler _commandEvent; protected override Freezable CreateInstanceCore() { return new ThumbButtonInfo(); } #region Dependency Properties and support methods public static readonly DependencyProperty VisibilityProperty = DependencyProperty.Register( "Visibility", typeof(Visibility), typeof(ThumbButtonInfo), new PropertyMetadata(Visibility.Visible)); ////// Gets or sets the whether this should be visible in the UI. /// public Visibility Visibility { get { return (Visibility)GetValue(VisibilityProperty); } set { SetValue(VisibilityProperty, value); } } public static readonly DependencyProperty DismissWhenClickedProperty = DependencyProperty.Register( "DismissWhenClicked", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata(false)); ////// Gets or sets the DismissWhenClicked property. This dependency property /// indicates whether the thumbnail window should disappear as a result /// of the user clicking this button. /// public bool DismissWhenClicked { get { return (bool)GetValue(DismissWhenClickedProperty); } set { SetValue(DismissWhenClickedProperty, value); } } public static readonly DependencyProperty ImageSourceProperty = DependencyProperty.Register( "ImageSource", typeof(ImageSource), typeof(ThumbButtonInfo), new PropertyMetadata(null)); ////// Gets or sets the ImageSource property. This dependency property /// indicates the ImageSource to use for this button's display. /// public ImageSource ImageSource { get { return (ImageSource)GetValue(ImageSourceProperty); } set { SetValue(ImageSourceProperty, value); } } public static readonly DependencyProperty IsBackgroundVisibleProperty = DependencyProperty.Register( "IsBackgroundVisible", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata(true)); ////// Gets or sets the IsBackgroundVisible property. This dependency property /// indicates whether the default background should be shown. /// public bool IsBackgroundVisible { get { return (bool)GetValue(IsBackgroundVisibleProperty); } set { SetValue(IsBackgroundVisibleProperty, value); } } public static readonly DependencyProperty DescriptionProperty = DependencyProperty.Register( "Description", typeof(string), typeof(ThumbButtonInfo), new PropertyMetadata( string.Empty, null, CoerceDescription)); ////// Gets or sets the Description property. This dependency property /// indicates the text to display in the tooltip for this button. /// public string Description { get { return (string)GetValue(DescriptionProperty); } set { SetValue(DescriptionProperty, value); } } // The THUMBBUTTON struct has a hard-coded length for this field of 260. private static object CoerceDescription(DependencyObject d, object value) { var text = (string)value; if (text != null && text.Length >= 260) { // Account for the NULL in native LPWSTRs text = text.Substring(0, 259); } return text; } public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.Register( "IsEnabled", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata( true, null, (d, e) => ((ThumbButtonInfo)d).CoerceIsEnabledValue(e))); private object CoerceIsEnabledValue(object value) { var enabled = (bool)value; return enabled && CanExecute; } ////// Gets or sets the IsEnabled property. /// ////// This dependency property /// indicates whether the button is receptive to user interaction and /// should appear as such. The button will not raise click events from /// the user when this property is false. /// See also IsInteractive. /// public bool IsEnabled { get { return (bool)GetValue(IsEnabledProperty); } set { SetValue(IsEnabledProperty, value); } } public static readonly DependencyProperty IsInteractiveProperty = DependencyProperty.Register( "IsInteractive", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata(true)); ////// Gets or sets the IsInteractive property. /// ////// This dependency property allows an enabled button, as determined /// by the IsEnabled property, to not raise click events. Buttons that /// have IsInteractive=false can be used to indicate status. /// IsEnabled=false takes precedence over IsInteractive=false. /// public bool IsInteractive { get { return (bool)GetValue(IsInteractiveProperty); } set { SetValue(IsInteractiveProperty, value); } } public static readonly DependencyProperty CommandProperty = DependencyProperty.Register( "Command", typeof(ICommand), typeof(ThumbButtonInfo), new PropertyMetadata( null, (d, e) => ((ThumbButtonInfo)d).OnCommandChanged(e))); private void OnCommandChanged(DependencyPropertyChangedEventArgs e) { var oldCommand = (ICommand)e.OldValue; var newCommand = (ICommand)e.NewValue; if (oldCommand == newCommand) { return; } if (oldCommand != null) { UnhookCommand(oldCommand); } if (newCommand != null) { HookCommand(newCommand); } } ////// CommandParameter Dependency Property /// public static readonly DependencyProperty CommandParameterProperty = DependencyProperty.Register( "CommandParameter", typeof(object), typeof(ThumbButtonInfo), new PropertyMetadata( null, (d, e) => ((ThumbButtonInfo)d).UpdateCanExecute())); // .Net property deferred to ICommandSource region. ////// CommandTarget Dependency Property /// public static readonly DependencyProperty CommandTargetProperty = DependencyProperty.Register( "CommandTarget", typeof(IInputElement), typeof(ThumbButtonInfo), new PropertyMetadata( null, (d, e) => ((ThumbButtonInfo)d).UpdateCanExecute())); // .Net property deferred to ICommandSource region. private static readonly DependencyProperty _CanExecuteProperty = DependencyProperty.Register( "_CanExecute", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata( true, (d, e) => d.CoerceValue(IsEnabledProperty))); private bool CanExecute { get { return (bool)GetValue(_CanExecuteProperty); } set { SetValue(_CanExecuteProperty, value); } } #endregion public event EventHandler Click; internal void InvokeClick() { EventHandler local = Click; if (local != null) { local(this, EventArgs.Empty); } _InvokeCommand(); } private void _InvokeCommand() { ICommand command = Command; if (command != null) { object parameter = CommandParameter; IInputElement target = CommandTarget; RoutedCommand routedCommand = command as RoutedCommand; if (routedCommand != null) { if (routedCommand.CanExecute(parameter, target)) { routedCommand.Execute(parameter, target); } } else if (command.CanExecute(parameter)) { command.Execute(parameter); } } } private void UnhookCommand(ICommand command) { Debug.Assert(command != null); command.CanExecuteChanged -= _commandEvent; _commandEvent = null; UpdateCanExecute(); } private void HookCommand(ICommand command) { _commandEvent = new EventHandler(OnCanExecuteChanged); command.CanExecuteChanged += _commandEvent; UpdateCanExecute(); } private void OnCanExecuteChanged(object sender, EventArgs e) { UpdateCanExecute(); } private void UpdateCanExecute() { if (Command != null) { object parameter = CommandParameter; IInputElement target = CommandTarget; RoutedCommand routed = Command as RoutedCommand; if (routed != null) { CanExecute = routed.CanExecute(parameter, target); } else { CanExecute = Command.CanExecute(parameter); } } else { CanExecute = true; } } #region ICommandSource Members public ICommand Command { get { return (ICommand)GetValue(CommandProperty); } set { SetValue(CommandProperty, value); } } public object CommandParameter { get { return (object)GetValue(CommandParameterProperty); } set { SetValue(CommandParameterProperty, value); } } public IInputElement CommandTarget { get { return (IInputElement)GetValue(CommandTargetProperty); } set { SetValue(CommandTargetProperty, value); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace System.Windows.Shell { using System; using System.Diagnostics; using System.Windows; using System.Windows.Input; using System.Windows.Media; public sealed class ThumbButtonInfo : Freezable, ICommandSource { // The CommandManager keeps weak references on delegates // hooked for CanExecuteChanged. We need to explicitly keep a // strong reference, because just adding an instance method causes // the auto-generated handler to be GC'd. private EventHandler _commandEvent; protected override Freezable CreateInstanceCore() { return new ThumbButtonInfo(); } #region Dependency Properties and support methods public static readonly DependencyProperty VisibilityProperty = DependencyProperty.Register( "Visibility", typeof(Visibility), typeof(ThumbButtonInfo), new PropertyMetadata(Visibility.Visible)); ////// Gets or sets the whether this should be visible in the UI. /// public Visibility Visibility { get { return (Visibility)GetValue(VisibilityProperty); } set { SetValue(VisibilityProperty, value); } } public static readonly DependencyProperty DismissWhenClickedProperty = DependencyProperty.Register( "DismissWhenClicked", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata(false)); ////// Gets or sets the DismissWhenClicked property. This dependency property /// indicates whether the thumbnail window should disappear as a result /// of the user clicking this button. /// public bool DismissWhenClicked { get { return (bool)GetValue(DismissWhenClickedProperty); } set { SetValue(DismissWhenClickedProperty, value); } } public static readonly DependencyProperty ImageSourceProperty = DependencyProperty.Register( "ImageSource", typeof(ImageSource), typeof(ThumbButtonInfo), new PropertyMetadata(null)); ////// Gets or sets the ImageSource property. This dependency property /// indicates the ImageSource to use for this button's display. /// public ImageSource ImageSource { get { return (ImageSource)GetValue(ImageSourceProperty); } set { SetValue(ImageSourceProperty, value); } } public static readonly DependencyProperty IsBackgroundVisibleProperty = DependencyProperty.Register( "IsBackgroundVisible", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata(true)); ////// Gets or sets the IsBackgroundVisible property. This dependency property /// indicates whether the default background should be shown. /// public bool IsBackgroundVisible { get { return (bool)GetValue(IsBackgroundVisibleProperty); } set { SetValue(IsBackgroundVisibleProperty, value); } } public static readonly DependencyProperty DescriptionProperty = DependencyProperty.Register( "Description", typeof(string), typeof(ThumbButtonInfo), new PropertyMetadata( string.Empty, null, CoerceDescription)); ////// Gets or sets the Description property. This dependency property /// indicates the text to display in the tooltip for this button. /// public string Description { get { return (string)GetValue(DescriptionProperty); } set { SetValue(DescriptionProperty, value); } } // The THUMBBUTTON struct has a hard-coded length for this field of 260. private static object CoerceDescription(DependencyObject d, object value) { var text = (string)value; if (text != null && text.Length >= 260) { // Account for the NULL in native LPWSTRs text = text.Substring(0, 259); } return text; } public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.Register( "IsEnabled", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata( true, null, (d, e) => ((ThumbButtonInfo)d).CoerceIsEnabledValue(e))); private object CoerceIsEnabledValue(object value) { var enabled = (bool)value; return enabled && CanExecute; } ////// Gets or sets the IsEnabled property. /// ////// This dependency property /// indicates whether the button is receptive to user interaction and /// should appear as such. The button will not raise click events from /// the user when this property is false. /// See also IsInteractive. /// public bool IsEnabled { get { return (bool)GetValue(IsEnabledProperty); } set { SetValue(IsEnabledProperty, value); } } public static readonly DependencyProperty IsInteractiveProperty = DependencyProperty.Register( "IsInteractive", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata(true)); ////// Gets or sets the IsInteractive property. /// ////// This dependency property allows an enabled button, as determined /// by the IsEnabled property, to not raise click events. Buttons that /// have IsInteractive=false can be used to indicate status. /// IsEnabled=false takes precedence over IsInteractive=false. /// public bool IsInteractive { get { return (bool)GetValue(IsInteractiveProperty); } set { SetValue(IsInteractiveProperty, value); } } public static readonly DependencyProperty CommandProperty = DependencyProperty.Register( "Command", typeof(ICommand), typeof(ThumbButtonInfo), new PropertyMetadata( null, (d, e) => ((ThumbButtonInfo)d).OnCommandChanged(e))); private void OnCommandChanged(DependencyPropertyChangedEventArgs e) { var oldCommand = (ICommand)e.OldValue; var newCommand = (ICommand)e.NewValue; if (oldCommand == newCommand) { return; } if (oldCommand != null) { UnhookCommand(oldCommand); } if (newCommand != null) { HookCommand(newCommand); } } ////// CommandParameter Dependency Property /// public static readonly DependencyProperty CommandParameterProperty = DependencyProperty.Register( "CommandParameter", typeof(object), typeof(ThumbButtonInfo), new PropertyMetadata( null, (d, e) => ((ThumbButtonInfo)d).UpdateCanExecute())); // .Net property deferred to ICommandSource region. ////// CommandTarget Dependency Property /// public static readonly DependencyProperty CommandTargetProperty = DependencyProperty.Register( "CommandTarget", typeof(IInputElement), typeof(ThumbButtonInfo), new PropertyMetadata( null, (d, e) => ((ThumbButtonInfo)d).UpdateCanExecute())); // .Net property deferred to ICommandSource region. private static readonly DependencyProperty _CanExecuteProperty = DependencyProperty.Register( "_CanExecute", typeof(bool), typeof(ThumbButtonInfo), new PropertyMetadata( true, (d, e) => d.CoerceValue(IsEnabledProperty))); private bool CanExecute { get { return (bool)GetValue(_CanExecuteProperty); } set { SetValue(_CanExecuteProperty, value); } } #endregion public event EventHandler Click; internal void InvokeClick() { EventHandler local = Click; if (local != null) { local(this, EventArgs.Empty); } _InvokeCommand(); } private void _InvokeCommand() { ICommand command = Command; if (command != null) { object parameter = CommandParameter; IInputElement target = CommandTarget; RoutedCommand routedCommand = command as RoutedCommand; if (routedCommand != null) { if (routedCommand.CanExecute(parameter, target)) { routedCommand.Execute(parameter, target); } } else if (command.CanExecute(parameter)) { command.Execute(parameter); } } } private void UnhookCommand(ICommand command) { Debug.Assert(command != null); command.CanExecuteChanged -= _commandEvent; _commandEvent = null; UpdateCanExecute(); } private void HookCommand(ICommand command) { _commandEvent = new EventHandler(OnCanExecuteChanged); command.CanExecuteChanged += _commandEvent; UpdateCanExecute(); } private void OnCanExecuteChanged(object sender, EventArgs e) { UpdateCanExecute(); } private void UpdateCanExecute() { if (Command != null) { object parameter = CommandParameter; IInputElement target = CommandTarget; RoutedCommand routed = Command as RoutedCommand; if (routed != null) { CanExecute = routed.CanExecute(parameter, target); } else { CanExecute = Command.CanExecute(parameter); } } else { CanExecute = true; } } #region ICommandSource Members public ICommand Command { get { return (ICommand)GetValue(CommandProperty); } set { SetValue(CommandProperty, value); } } public object CommandParameter { get { return (object)GetValue(CommandParameterProperty); } set { SetValue(CommandParameterProperty, value); } } public IInputElement CommandTarget { get { return (IInputElement)GetValue(CommandTargetProperty); } set { SetValue(CommandTargetProperty, value); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
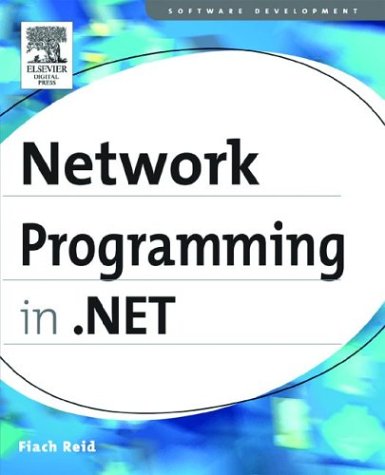
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlToClrEventProxy.cs
- EdmConstants.cs
- CellTreeNodeVisitors.cs
- DeobfuscatingStream.cs
- HtmlTitle.cs
- XsltLibrary.cs
- IndexingContentUnit.cs
- CFStream.cs
- DocumentSchemaValidator.cs
- EventProxy.cs
- SemanticBasicElement.cs
- SiteMap.cs
- CellRelation.cs
- _AutoWebProxyScriptHelper.cs
- OutputCacheSection.cs
- Compiler.cs
- DescendantOverDescendantQuery.cs
- TrustManager.cs
- ColumnClickEvent.cs
- ApplyImportsAction.cs
- MetabaseServerConfig.cs
- TextBreakpoint.cs
- Simplifier.cs
- StdValidatorsAndConverters.cs
- SymmetricAlgorithm.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- SettingsSection.cs
- wmiprovider.cs
- ItemContainerPattern.cs
- DataExchangeServiceBinder.cs
- CodeCommentStatementCollection.cs
- VariableBinder.cs
- BinaryVersion.cs
- ImageFormat.cs
- XmlSchemaImport.cs
- FixUp.cs
- CharEnumerator.cs
- SetterBase.cs
- MarshalByValueComponent.cs
- ThemeConfigurationDialog.cs
- ExecutionScope.cs
- EmbeddedMailObject.cs
- ColorMatrix.cs
- NaturalLanguageHyphenator.cs
- UserControlBuildProvider.cs
- UpdatePanelTrigger.cs
- AssemblyAssociatedContentFileAttribute.cs
- ParseChildrenAsPropertiesAttribute.cs
- EntityDataSourceWrapper.cs
- CqlBlock.cs
- SystemResourceHost.cs
- TreeViewEvent.cs
- TypeNameConverter.cs
- DataError.cs
- XmlArrayItemAttribute.cs
- NamespaceCollection.cs
- PropertiesTab.cs
- PrivilegedConfigurationManager.cs
- FixedSOMTable.cs
- TemplateControlBuildProvider.cs
- FontStyleConverter.cs
- SqlUserDefinedAggregateAttribute.cs
- RealProxy.cs
- RefreshEventArgs.cs
- UnaryNode.cs
- CurrentTimeZone.cs
- XmlSchemaSimpleTypeUnion.cs
- MemberHolder.cs
- FormsAuthenticationConfiguration.cs
- MaterializeFromAtom.cs
- SQLDateTimeStorage.cs
- TemplateField.cs
- WasNotInstalledException.cs
- TabControlAutomationPeer.cs
- RectAnimation.cs
- StrokeNodeOperations2.cs
- ExpressionVisitor.cs
- SchemaNames.cs
- BitmapPalettes.cs
- AuthStoreRoleProvider.cs
- DecoderBestFitFallback.cs
- WebResourceAttribute.cs
- BasePropertyDescriptor.cs
- ObjectListComponentEditor.cs
- XmlUtil.cs
- UnmanagedMarshal.cs
- PagesSection.cs
- RadioButtonPopupAdapter.cs
- WebPartConnectionsCancelEventArgs.cs
- ContentPathSegment.cs
- AssociationTypeEmitter.cs
- GeneratedCodeAttribute.cs
- InfoCardCryptoHelper.cs
- XmlUtilWriter.cs
- PackUriHelper.cs
- OptimizedTemplateContentHelper.cs
- ToolStripSeparatorRenderEventArgs.cs
- DetailsViewCommandEventArgs.cs
- CommandDesigner.cs
- BamlTreeNode.cs