Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / ArraySegment.cs / 1 / ArraySegment.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ArraySegment** ** ** Purpose: Convenient wrapper for an array, an offset, and ** a count. Ideally used in streams & collections. ** Net Classes will consume an array of these. ** ** ===========================================================*/ using System.Runtime.InteropServices; namespace System { [Serializable] public struct ArraySegment { private T[] _array; private int _offset; private int _count; public ArraySegment(T[] array) { if (array == null) throw new ArgumentNullException("array"); _array = array; _offset = 0; _count = array.Length; } public ArraySegment(T[] array, int offset, int count) { if (array == null) throw new ArgumentNullException("array"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (array.Length - offset < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); _array = array; _offset = offset; _count = count; } public T[] Array { get { return _array; } } public int Offset { get { return _offset; } } public int Count { get { return _count; } } public override int GetHashCode() { return _array.GetHashCode() ^ _offset ^ _count; } public override bool Equals(Object obj) { if (obj is ArraySegment ) return Equals((ArraySegment )obj); else return false; } public bool Equals(ArraySegment obj) { return obj._array == _array && obj._offset == _offset && obj._count == _count; } public static bool operator ==(ArraySegment a, ArraySegment b) { return a.Equals(b); } public static bool operator !=(ArraySegment a, ArraySegment b) { return !(a == b); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ArraySegment ** ** ** Purpose: Convenient wrapper for an array, an offset, and ** a count. Ideally used in streams & collections. ** Net Classes will consume an array of these. ** ** ===========================================================*/ using System.Runtime.InteropServices; namespace System { [Serializable] public struct ArraySegment { private T[] _array; private int _offset; private int _count; public ArraySegment(T[] array) { if (array == null) throw new ArgumentNullException("array"); _array = array; _offset = 0; _count = array.Length; } public ArraySegment(T[] array, int offset, int count) { if (array == null) throw new ArgumentNullException("array"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (array.Length - offset < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); _array = array; _offset = offset; _count = count; } public T[] Array { get { return _array; } } public int Offset { get { return _offset; } } public int Count { get { return _count; } } public override int GetHashCode() { return _array.GetHashCode() ^ _offset ^ _count; } public override bool Equals(Object obj) { if (obj is ArraySegment ) return Equals((ArraySegment )obj); else return false; } public bool Equals(ArraySegment obj) { return obj._array == _array && obj._offset == _offset && obj._count == _count; } public static bool operator ==(ArraySegment a, ArraySegment b) { return a.Equals(b); } public static bool operator !=(ArraySegment a, ArraySegment b) { return !(a == b); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
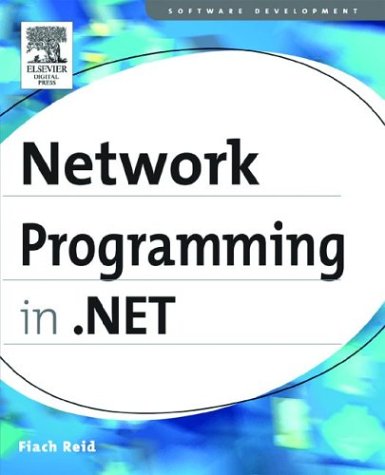
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompareValidator.cs
- Panel.cs
- FactoryMaker.cs
- AuthenticationService.cs
- sqlpipe.cs
- Int32AnimationUsingKeyFrames.cs
- UnionCqlBlock.cs
- RedistVersionInfo.cs
- TypeUnloadedException.cs
- TriState.cs
- InputProcessorProfilesLoader.cs
- _OverlappedAsyncResult.cs
- StickyNoteContentControl.cs
- RandomNumberGenerator.cs
- PseudoWebRequest.cs
- FixedPageAutomationPeer.cs
- PackUriHelper.cs
- Converter.cs
- KeyBinding.cs
- WindowsGrip.cs
- TrustVersion.cs
- FileRecordSequence.cs
- CmsInterop.cs
- MouseWheelEventArgs.cs
- StructuralType.cs
- DeviceContext2.cs
- SortedList.cs
- Animatable.cs
- Funcletizer.cs
- ColumnBinding.cs
- QilReference.cs
- TemplateManager.cs
- AttachInfo.cs
- ListenerConfig.cs
- SqlComparer.cs
- CompiledRegexRunner.cs
- Visual3D.cs
- DbProviderFactory.cs
- IndicShape.cs
- SinglePhaseEnlistment.cs
- Int32Rect.cs
- ShaderRenderModeValidation.cs
- MdiWindowListStrip.cs
- MultiViewDesigner.cs
- NamespaceList.cs
- EdmTypeAttribute.cs
- ManagedWndProcTracker.cs
- Light.cs
- ReferenceConverter.cs
- ComponentResourceKeyConverter.cs
- AspNetCacheProfileAttribute.cs
- StringComparer.cs
- DataTemplateSelector.cs
- HttpPostedFile.cs
- TraversalRequest.cs
- AnonymousIdentificationSection.cs
- ProcessModule.cs
- AdornerDecorator.cs
- Conditional.cs
- EndPoint.cs
- MulticastDelegate.cs
- exports.cs
- contentDescriptor.cs
- OutputCacheSection.cs
- SqlUtils.cs
- WindowsTreeView.cs
- FrugalList.cs
- AccessDataSource.cs
- Color.cs
- DefaultWorkflowSchedulerService.cs
- DiscriminatorMap.cs
- XmlElement.cs
- PropertyGridEditorPart.cs
- ListViewGroupConverter.cs
- ModelPropertyImpl.cs
- ISAPIWorkerRequest.cs
- SqlConnectionString.cs
- SafeLibraryHandle.cs
- Compilation.cs
- SqlTypeConverter.cs
- ObjectListSelectEventArgs.cs
- StringBlob.cs
- IdentityModelDictionary.cs
- CodePropertyReferenceExpression.cs
- TextServicesLoader.cs
- EntityDataSourceWrapper.cs
- EventItfInfo.cs
- RequestCacheEntry.cs
- StaticDataManager.cs
- BamlRecordHelper.cs
- SeekableReadStream.cs
- UriSection.cs
- BitmapMetadataBlob.cs
- HandlerFactoryCache.cs
- SerializableAttribute.cs
- Utils.cs
- ReliableSessionBindingElementImporter.cs
- CapabilitiesUse.cs
- TypefaceMap.cs
- RecognitionEventArgs.cs