Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / _ShellExpression.cs / 1 / _ShellExpression.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; internal struct ShellExpression { private enum ShExpTokens { Asterisk = -1, Question = -2, AugmentedDot = -3, AugmentedAsterisk = -4, AugmentedQuestion = -5, Start = -6, End = -7 } private ShExpTokens[] pattern; private int[] match; // Normally would defer parsing, but we want to throw here if it's invalid. internal ShellExpression(string pattern) { this.pattern = null; this.match = null; GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::.ctor() pattern:" + ValidationHelper.ToString(pattern)); Parse(pattern); } /* // Consider removing. internal void SetPattern(string pattern) { GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::SetPattern() pattern:" + ValidationHelper.ToString(pattern)); Parse(pattern); } */ internal bool IsMatch(string target) { GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::IsMatch() target:" + ValidationHelper.ToString(target)); int i = 0; int j = 0; bool reverse = false; bool matched = false; while (true) { if (!reverse) { if (j > target.Length) { break; } switch (pattern[i]) { case ShExpTokens.Asterisk: match[i++] = j = target.Length; continue; case ShExpTokens.Question: if (j == target.Length) { reverse = true; } else { match[i++] = ++j; } continue; case ShExpTokens.AugmentedDot: if (j == target.Length) { match[i++] = j; } else if (target[j] == '.') { match[i++] = ++j; } else { reverse = true; } continue; case ShExpTokens.AugmentedAsterisk: if (j == target.Length || target[j] == '.') { reverse = true; } else { match[i++] = ++j; } continue; case ShExpTokens.AugmentedQuestion: if (j == target.Length || target[j] == '.') { match[i++] = j; } else { match[i++] = ++j; } continue; case ShExpTokens.Start: if (j != 0) { break; } match[i++] = 0; continue; case ShExpTokens.End: if (j == target.Length) { matched = true; break; } reverse = true; continue; default: if (j < target.Length && (int) pattern[i] == (int) char.ToLowerInvariant(target[j])) { match[i++] = ++j; } else { reverse = true; } continue; } } else { switch (pattern[--i]) { case ShExpTokens.Asterisk: case ShExpTokens.AugmentedQuestion: if (match[i] != match[i - 1]) { j = --match[i++]; reverse = false; } continue; case ShExpTokens.Start: case ShExpTokens.End: break; case ShExpTokens.Question: case ShExpTokens.AugmentedDot: case ShExpTokens.AugmentedAsterisk: default: continue; } } break; } GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::IsMatch() return:" + matched.ToString()); return matched; } private void Parse(string patString) { pattern = new ShExpTokens[patString.Length + 2]; // 2 for the start, end match = null; int i = 0; pattern[i++] = ShExpTokens.Start; for (int j = 0; j < patString.Length; j++) { switch (patString[j]) { case '?': pattern[i++] = ShExpTokens.Question; break; case '*': pattern[i++] = ShExpTokens.Asterisk; break; case '^': if (j < patString.Length - 1) { j++; } else { pattern = null; if (Logging.On) Logging.PrintWarning(Logging.Web, SR.GetString(SR.net_log_shell_expression_pattern_format_warning, patString)); throw new FormatException(SR.GetString(SR.net_format_shexp, patString)); } switch (patString[j]) { case '.': pattern[i++] = ShExpTokens.AugmentedDot; break; case '?': pattern[i++] = ShExpTokens.AugmentedQuestion; break; case '*': pattern[i++] = ShExpTokens.AugmentedAsterisk; break; default: pattern = null; if (Logging.On) Logging.PrintWarning(Logging.Web, SR.GetString(SR.net_log_shell_expression_pattern_format_warning, patString)); throw new FormatException(SR.GetString(SR.net_format_shexp, patString)); } break; default: pattern[i++] = (ShExpTokens) (int) char.ToLowerInvariant(patString[j]); break; } } pattern[i++] = ShExpTokens.End; match = new int[i]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; internal struct ShellExpression { private enum ShExpTokens { Asterisk = -1, Question = -2, AugmentedDot = -3, AugmentedAsterisk = -4, AugmentedQuestion = -5, Start = -6, End = -7 } private ShExpTokens[] pattern; private int[] match; // Normally would defer parsing, but we want to throw here if it's invalid. internal ShellExpression(string pattern) { this.pattern = null; this.match = null; GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::.ctor() pattern:" + ValidationHelper.ToString(pattern)); Parse(pattern); } /* // Consider removing. internal void SetPattern(string pattern) { GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::SetPattern() pattern:" + ValidationHelper.ToString(pattern)); Parse(pattern); } */ internal bool IsMatch(string target) { GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::IsMatch() target:" + ValidationHelper.ToString(target)); int i = 0; int j = 0; bool reverse = false; bool matched = false; while (true) { if (!reverse) { if (j > target.Length) { break; } switch (pattern[i]) { case ShExpTokens.Asterisk: match[i++] = j = target.Length; continue; case ShExpTokens.Question: if (j == target.Length) { reverse = true; } else { match[i++] = ++j; } continue; case ShExpTokens.AugmentedDot: if (j == target.Length) { match[i++] = j; } else if (target[j] == '.') { match[i++] = ++j; } else { reverse = true; } continue; case ShExpTokens.AugmentedAsterisk: if (j == target.Length || target[j] == '.') { reverse = true; } else { match[i++] = ++j; } continue; case ShExpTokens.AugmentedQuestion: if (j == target.Length || target[j] == '.') { match[i++] = j; } else { match[i++] = ++j; } continue; case ShExpTokens.Start: if (j != 0) { break; } match[i++] = 0; continue; case ShExpTokens.End: if (j == target.Length) { matched = true; break; } reverse = true; continue; default: if (j < target.Length && (int) pattern[i] == (int) char.ToLowerInvariant(target[j])) { match[i++] = ++j; } else { reverse = true; } continue; } } else { switch (pattern[--i]) { case ShExpTokens.Asterisk: case ShExpTokens.AugmentedQuestion: if (match[i] != match[i - 1]) { j = --match[i++]; reverse = false; } continue; case ShExpTokens.Start: case ShExpTokens.End: break; case ShExpTokens.Question: case ShExpTokens.AugmentedDot: case ShExpTokens.AugmentedAsterisk: default: continue; } } break; } GlobalLog.Print("ShellServices.ShellExpression#" + ValidationHelper.HashString(this) + "::IsMatch() return:" + matched.ToString()); return matched; } private void Parse(string patString) { pattern = new ShExpTokens[patString.Length + 2]; // 2 for the start, end match = null; int i = 0; pattern[i++] = ShExpTokens.Start; for (int j = 0; j < patString.Length; j++) { switch (patString[j]) { case '?': pattern[i++] = ShExpTokens.Question; break; case '*': pattern[i++] = ShExpTokens.Asterisk; break; case '^': if (j < patString.Length - 1) { j++; } else { pattern = null; if (Logging.On) Logging.PrintWarning(Logging.Web, SR.GetString(SR.net_log_shell_expression_pattern_format_warning, patString)); throw new FormatException(SR.GetString(SR.net_format_shexp, patString)); } switch (patString[j]) { case '.': pattern[i++] = ShExpTokens.AugmentedDot; break; case '?': pattern[i++] = ShExpTokens.AugmentedQuestion; break; case '*': pattern[i++] = ShExpTokens.AugmentedAsterisk; break; default: pattern = null; if (Logging.On) Logging.PrintWarning(Logging.Web, SR.GetString(SR.net_log_shell_expression_pattern_format_warning, patString)); throw new FormatException(SR.GetString(SR.net_format_shexp, patString)); } break; default: pattern[i++] = (ShExpTokens) (int) char.ToLowerInvariant(patString[j]); break; } } pattern[i++] = ShExpTokens.End; match = new int[i]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
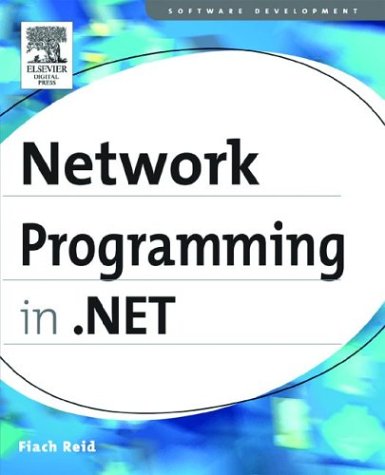
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewAutomationPeer.cs
- ValueQuery.cs
- MobileContainerDesigner.cs
- ClientFormsIdentity.cs
- XmlBinaryReader.cs
- BaseTemplateCodeDomTreeGenerator.cs
- KeyboardInputProviderAcquireFocusEventArgs.cs
- XmlSchemaDocumentation.cs
- PackagePart.cs
- InitiatorServiceModelSecurityTokenRequirement.cs
- Helpers.cs
- XmlDataProvider.cs
- SecurityHelper.cs
- EntityTypeEmitter.cs
- PermissionListSet.cs
- ObjectSecurity.cs
- SystemColorTracker.cs
- XmlNullResolver.cs
- CodeDOMProvider.cs
- PeerResolver.cs
- ObjectCache.cs
- __Error.cs
- RestHandler.cs
- XmlCharType.cs
- DbParameterHelper.cs
- PopupEventArgs.cs
- XmlImplementation.cs
- DeviceOverridableAttribute.cs
- MediaEntryAttribute.cs
- SizeConverter.cs
- AudioException.cs
- OverrideMode.cs
- __ConsoleStream.cs
- CodeIterationStatement.cs
- Int32Rect.cs
- GcSettings.cs
- Annotation.cs
- TextPenaltyModule.cs
- TrustLevelCollection.cs
- Axis.cs
- IpcChannelHelper.cs
- Scheduling.cs
- Comparer.cs
- ToolStripDropDownDesigner.cs
- TypeInformation.cs
- XmlQueryCardinality.cs
- GlobalizationAssembly.cs
- GridLength.cs
- DoubleConverter.cs
- RequestedSignatureDialog.cs
- TimeSpanStorage.cs
- Material.cs
- CustomError.cs
- TreeNodeCollection.cs
- CodeStatement.cs
- NameValueCollection.cs
- TransportContext.cs
- DiscoveryDocumentSerializer.cs
- Activator.cs
- MemberHolder.cs
- EditorZoneBase.cs
- BinaryUtilClasses.cs
- DropTarget.cs
- __Filters.cs
- CompilerParameters.cs
- MarkupObject.cs
- PageFunction.cs
- CommandDesigner.cs
- TagNameToTypeMapper.cs
- Stylesheet.cs
- VideoDrawing.cs
- ConnectionConsumerAttribute.cs
- StrokeCollectionConverter.cs
- SecurityPermission.cs
- SqlUdtInfo.cs
- KeyMatchBuilder.cs
- DataGridViewCellValueEventArgs.cs
- ReferenceEqualityComparer.cs
- Thread.cs
- TextFormatter.cs
- DataBoundControlAdapter.cs
- BamlRecordHelper.cs
- DesignObjectWrapper.cs
- SelectionPattern.cs
- ControlCachePolicy.cs
- SystemMulticastIPAddressInformation.cs
- SettingsPropertyValue.cs
- HtmlElementErrorEventArgs.cs
- WeakRefEnumerator.cs
- SqlNode.cs
- DispatcherHooks.cs
- EntityDataSourceDataSelection.cs
- HttpWebRequest.cs
- BitmapEffectInput.cs
- HttpsTransportElement.cs
- ScrollViewerAutomationPeer.cs
- HttpListenerContext.cs
- BitmapSizeOptions.cs
- Stackframe.cs
- CustomSignedXml.cs