Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EdmType.cs / 3 / EdmType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Globalization; using System.Diagnostics; using System.Collections.Generic; using System.Text; using System.Threading; namespace System.Data.Metadata.Edm { ////// Base EdmType class for all the model types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract class EdmType : GlobalItem { #region Constructors ////// Initializes a new instance of EdmType /// internal EdmType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } ////// Constructs a new instance of EdmType with the given name, namespace and version /// /// name of the type /// namespace of the type /// version of the type /// dataSpace in which this edmtype belongs to ///Thrown if either the name, namespace or version arguments are null internal EdmType(string name, string namespaceName, DataSpace dataSpace) { EntityUtil.GenericCheckArgumentNull(name, "name"); EntityUtil.GenericCheckArgumentNull(namespaceName, "namespaceName"); // Initialize the item attributes EdmType.Initialize(this, name, namespaceName, dataSpace, false, null); } #endregion #region Fields private CollectionType _collectionType; private string _identity; private string _name; private string _namespace; private EdmType _baseType; #endregion #region Properties ////// Direct accessor for the field Identity. The reason we need to do this is that for derived class, /// they want to cache things only when they are readonly. Plus they want to check for null before /// updating the value /// internal string CacheIdentity { get { return _identity; } private set { _identity = value; } } ////// Returns the identity of the edm type /// internal override string Identity { get { if (this.CacheIdentity == null) { StringBuilder builder = new StringBuilder(50); BuildIdentity(builder); this.CacheIdentity = builder.ToString(); } return this.CacheIdentity; } } ////// Returns the name of the EdmType /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } internal set { Debug.Assert(value != null, "The name should never be set to null"); _name = value; } } ////// Returns the namespace of the EdmType /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String NamespaceName { get { return _namespace; } internal set { Debug.Assert(value != null, "Namespace should never be set to null"); _namespace = value; } } ////// Returns true if the EdmType is abstract /// ///Thrown if the setter is called on instance that is in ReadOnly state [MetadataProperty(PrimitiveTypeKind.Boolean, false)] public bool Abstract { get { return GetFlag(MetadataFlags.IsAbstract); } internal set { SetFlag(MetadataFlags.IsAbstract, value); } } ////// Returns the base type of the EdmType /// ///Thrown if the setter is called on instance that is in ReadOnly state ///Thrown if the value passed in for setter will create a loop in the inheritance chain [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType BaseType { get { return _baseType; } internal set { Util.ThrowIfReadOnly(this); Debug.Assert(_baseType == null, "BaseType can't be set multiple times"); // Check to make sure there won't be a loop in the inheritance EdmType type = value; while (type != null) { if (type == this) { throw EntityUtil.InvalidBaseTypeLoop("value"); } type = type.BaseType; } // Also if the base type is EntityTypeBase, make sure it doesn't have keys Debug.Assert(!Helper.IsEntityTypeBase(this) || ((EntityTypeBase)this).KeyMembers.Count == 0 || ((EntityTypeBase)value).KeyMembers.Count == 0, " For EntityTypeBase, both base type and derived types cannot have keys defined"); _baseType = value; } } ////// Returns the full name of this type, which is namespace + "." + name. /// Since the identity of all EdmTypes, except EdmFunction, is same as of that /// of the full name, FullName just returns the identity. This property is /// over-ridden in EdmFunctin, just to return NamespaceName + "." + Name /// public virtual string FullName { get { return this.Identity; } } ////// If OSpace, return the CLR Type else null /// ///Thrown if the setter is called on instance that is in ReadOnly state internal virtual System.Type ClrType { get { return null; } } internal override void BuildIdentity(StringBuilder builder) { // if we already know the identity, simply append it if (null != this.CacheIdentity) { builder.Append(this.CacheIdentity); return; } builder.Append(CreateEdmTypeIdentity(NamespaceName, Name)); } internal static string CreateEdmTypeIdentity(string namespaceName, string name) { string identity = string.Empty; if (string.Empty != namespaceName) { identity = namespaceName + "."; } identity += name; return identity; } #endregion #region Methods ////// Initialize the type. This method must be called since for bootstraping we only call the constructor. /// This method will help us initialize the type /// /// The edm type to initialize with item attributes /// The name of this type /// The namespace of this type /// The version of this type /// dataSpace in which this edmtype belongs to /// If the type is abstract /// If the type is sealed /// The base type for this type internal static void Initialize(EdmType edmType, string name, string namespaceName, DataSpace dataSpace, bool isAbstract, EdmType baseType) { edmType._baseType = baseType; edmType._name = name; edmType._namespace = namespaceName; edmType.DataSpace = dataSpace; edmType.Abstract = isAbstract; } ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.FullName; } ////// Returns the collection type whose element type is this edm type /// public CollectionType GetCollectionType() { if (_collectionType == null) { Interlocked.CompareExchange(ref _collectionType, new CollectionType(this), null); } return _collectionType; } /// /// check to see if otherType is among the base types, /// /// ////// if otherType is among the base types, return true, /// otherwise returns false. /// when othertype is same as the current type, return false. /// internal virtual bool IsSubtypeOf(EdmType otherType) { return Helper.IsSubtypeOf(this, otherType); } ////// check to see if otherType is among the sub-types, /// /// ////// if otherType is among the sub-types, returns true, /// otherwise returns false. /// when othertype is same as the current type, return false. /// internal virtual bool IsBaseTypeOf(EdmType otherType) { if (otherType == null) return false; return otherType.IsSubtypeOf(this); } ////// Check if this type is assignable from otherType /// /// ///internal virtual bool IsAssignableFrom(EdmType otherType) { return Helper.IsAssignableFrom(this, otherType); } /// /// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); EdmType baseType = BaseType; if (baseType != null) { baseType.SetReadOnly(); } } } ////// Returns all facet descriptions associated with this type. /// ///Descriptions for all built-in facets for this type. internal virtual IEnumerableGetAssociatedFacetDescriptions() { return MetadataItem.GetGeneralFacetDescriptions(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Globalization; using System.Diagnostics; using System.Collections.Generic; using System.Text; using System.Threading; namespace System.Data.Metadata.Edm { ////// Base EdmType class for all the model types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract class EdmType : GlobalItem { #region Constructors ////// Initializes a new instance of EdmType /// internal EdmType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } ////// Constructs a new instance of EdmType with the given name, namespace and version /// /// name of the type /// namespace of the type /// version of the type /// dataSpace in which this edmtype belongs to ///Thrown if either the name, namespace or version arguments are null internal EdmType(string name, string namespaceName, DataSpace dataSpace) { EntityUtil.GenericCheckArgumentNull(name, "name"); EntityUtil.GenericCheckArgumentNull(namespaceName, "namespaceName"); // Initialize the item attributes EdmType.Initialize(this, name, namespaceName, dataSpace, false, null); } #endregion #region Fields private CollectionType _collectionType; private string _identity; private string _name; private string _namespace; private EdmType _baseType; #endregion #region Properties ////// Direct accessor for the field Identity. The reason we need to do this is that for derived class, /// they want to cache things only when they are readonly. Plus they want to check for null before /// updating the value /// internal string CacheIdentity { get { return _identity; } private set { _identity = value; } } ////// Returns the identity of the edm type /// internal override string Identity { get { if (this.CacheIdentity == null) { StringBuilder builder = new StringBuilder(50); BuildIdentity(builder); this.CacheIdentity = builder.ToString(); } return this.CacheIdentity; } } ////// Returns the name of the EdmType /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } internal set { Debug.Assert(value != null, "The name should never be set to null"); _name = value; } } ////// Returns the namespace of the EdmType /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String NamespaceName { get { return _namespace; } internal set { Debug.Assert(value != null, "Namespace should never be set to null"); _namespace = value; } } ////// Returns true if the EdmType is abstract /// ///Thrown if the setter is called on instance that is in ReadOnly state [MetadataProperty(PrimitiveTypeKind.Boolean, false)] public bool Abstract { get { return GetFlag(MetadataFlags.IsAbstract); } internal set { SetFlag(MetadataFlags.IsAbstract, value); } } ////// Returns the base type of the EdmType /// ///Thrown if the setter is called on instance that is in ReadOnly state ///Thrown if the value passed in for setter will create a loop in the inheritance chain [MetadataProperty(BuiltInTypeKind.EdmType, false)] public EdmType BaseType { get { return _baseType; } internal set { Util.ThrowIfReadOnly(this); Debug.Assert(_baseType == null, "BaseType can't be set multiple times"); // Check to make sure there won't be a loop in the inheritance EdmType type = value; while (type != null) { if (type == this) { throw EntityUtil.InvalidBaseTypeLoop("value"); } type = type.BaseType; } // Also if the base type is EntityTypeBase, make sure it doesn't have keys Debug.Assert(!Helper.IsEntityTypeBase(this) || ((EntityTypeBase)this).KeyMembers.Count == 0 || ((EntityTypeBase)value).KeyMembers.Count == 0, " For EntityTypeBase, both base type and derived types cannot have keys defined"); _baseType = value; } } ////// Returns the full name of this type, which is namespace + "." + name. /// Since the identity of all EdmTypes, except EdmFunction, is same as of that /// of the full name, FullName just returns the identity. This property is /// over-ridden in EdmFunctin, just to return NamespaceName + "." + Name /// public virtual string FullName { get { return this.Identity; } } ////// If OSpace, return the CLR Type else null /// ///Thrown if the setter is called on instance that is in ReadOnly state internal virtual System.Type ClrType { get { return null; } } internal override void BuildIdentity(StringBuilder builder) { // if we already know the identity, simply append it if (null != this.CacheIdentity) { builder.Append(this.CacheIdentity); return; } builder.Append(CreateEdmTypeIdentity(NamespaceName, Name)); } internal static string CreateEdmTypeIdentity(string namespaceName, string name) { string identity = string.Empty; if (string.Empty != namespaceName) { identity = namespaceName + "."; } identity += name; return identity; } #endregion #region Methods ////// Initialize the type. This method must be called since for bootstraping we only call the constructor. /// This method will help us initialize the type /// /// The edm type to initialize with item attributes /// The name of this type /// The namespace of this type /// The version of this type /// dataSpace in which this edmtype belongs to /// If the type is abstract /// If the type is sealed /// The base type for this type internal static void Initialize(EdmType edmType, string name, string namespaceName, DataSpace dataSpace, bool isAbstract, EdmType baseType) { edmType._baseType = baseType; edmType._name = name; edmType._namespace = namespaceName; edmType.DataSpace = dataSpace; edmType.Abstract = isAbstract; } ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return this.FullName; } ////// Returns the collection type whose element type is this edm type /// public CollectionType GetCollectionType() { if (_collectionType == null) { Interlocked.CompareExchange(ref _collectionType, new CollectionType(this), null); } return _collectionType; } /// /// check to see if otherType is among the base types, /// /// ////// if otherType is among the base types, return true, /// otherwise returns false. /// when othertype is same as the current type, return false. /// internal virtual bool IsSubtypeOf(EdmType otherType) { return Helper.IsSubtypeOf(this, otherType); } ////// check to see if otherType is among the sub-types, /// /// ////// if otherType is among the sub-types, returns true, /// otherwise returns false. /// when othertype is same as the current type, return false. /// internal virtual bool IsBaseTypeOf(EdmType otherType) { if (otherType == null) return false; return otherType.IsSubtypeOf(this); } ////// Check if this type is assignable from otherType /// /// ///internal virtual bool IsAssignableFrom(EdmType otherType) { return Helper.IsAssignableFrom(this, otherType); } /// /// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); EdmType baseType = BaseType; if (baseType != null) { baseType.SetReadOnly(); } } } ////// Returns all facet descriptions associated with this type. /// ///Descriptions for all built-in facets for this type. internal virtual IEnumerableGetAssociatedFacetDescriptions() { return MetadataItem.GetGeneralFacetDescriptions(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
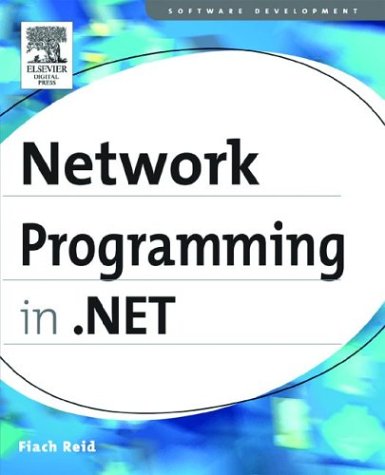
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Function.cs
- SerializationSectionGroup.cs
- CacheHelper.cs
- IsolationInterop.cs
- ReverseQueryOperator.cs
- UpdatePanelTrigger.cs
- DynamicQueryableWrapper.cs
- UnaryNode.cs
- FormViewDeleteEventArgs.cs
- MetadataItemEmitter.cs
- BitmapEffectInput.cs
- SymDocumentType.cs
- Token.cs
- SelectedCellsChangedEventArgs.cs
- EventLogStatus.cs
- SafeNativeMethodsOther.cs
- MimeBasePart.cs
- ManagedIStream.cs
- TypefaceMetricsCache.cs
- GenericWebPart.cs
- Rotation3D.cs
- MobileTextWriter.cs
- CachedPathData.cs
- BamlReader.cs
- TextBoxDesigner.cs
- MenuItem.cs
- SqlLiftIndependentRowExpressions.cs
- InvokeSchedule.cs
- XmlDataSourceView.cs
- BamlResourceContent.cs
- TreeNodeStyle.cs
- XPathChildIterator.cs
- CryptographicAttribute.cs
- PropertyValueChangedEvent.cs
- Quaternion.cs
- Boolean.cs
- ScrollEvent.cs
- HostedImpersonationContext.cs
- RegexRunner.cs
- SegmentTree.cs
- HttpCapabilitiesEvaluator.cs
- EntityPropertyMappingAttribute.cs
- UpdatableGenericsFeature.cs
- ImmutableClientRuntime.cs
- CompressedStack.cs
- PartitionedStreamMerger.cs
- EventMap.cs
- HtmlTitle.cs
- ProcessModule.cs
- NativeStructs.cs
- RoutedCommand.cs
- ContentControl.cs
- BinaryNode.cs
- DecimalKeyFrameCollection.cs
- LogReserveAndAppendState.cs
- ColumnCollection.cs
- OpenTypeLayout.cs
- DrawingVisualDrawingContext.cs
- SiteMapHierarchicalDataSourceView.cs
- SQLBytesStorage.cs
- Propagator.cs
- IntPtr.cs
- ParseChildrenAsPropertiesAttribute.cs
- GetPageCompletedEventArgs.cs
- BevelBitmapEffect.cs
- ComplexBindingPropertiesAttribute.cs
- SiteMap.cs
- _HTTPDateParse.cs
- SessionStateItemCollection.cs
- CompilerErrorCollection.cs
- clipboard.cs
- ItemsControlAutomationPeer.cs
- TimersDescriptionAttribute.cs
- DataGridViewBindingCompleteEventArgs.cs
- TextTrailingWordEllipsis.cs
- ConfigurationPropertyCollection.cs
- SafeUserTokenHandle.cs
- SubordinateTransaction.cs
- SubstitutionList.cs
- DefaultConfirmation.cs
- ResolvedKeyFrameEntry.cs
- DataTableReader.cs
- IgnoreDeviceFilterElement.cs
- SafeRightsManagementSessionHandle.cs
- RawStylusInputReport.cs
- SynchronizingStream.cs
- FormViewDeleteEventArgs.cs
- SizeKeyFrameCollection.cs
- FilterException.cs
- QueueNameHelper.cs
- DataGridColumnCollectionEditor.cs
- EncryptedXml.cs
- DrawingBrush.cs
- DoubleAnimationUsingPath.cs
- ValidatorCollection.cs
- ComPlusInstanceProvider.cs
- Triangle.cs
- WindowsSlider.cs
- ColumnResizeAdorner.cs
- Vector3DAnimationUsingKeyFrames.cs