Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Security / System / Security / Cryptography / Xml / Signature.cs / 1305376 / Signature.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // Signature.cs // // 21 [....] 2000 // namespace System.Security.Cryptography.Xml { using System; using System.Collections; using System.Xml; [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public class Signature { private string m_id; private SignedInfo m_signedInfo; private byte[] m_signatureValue; private string m_signatureValueId; private KeyInfo m_keyInfo; private IList m_embeddedObjects; private CanonicalXmlNodeList m_referencedItems; private SignedXml m_signedXml = null; internal SignedXml SignedXml { get { return m_signedXml; } set { m_signedXml = value; } } // // public constructors // public Signature() { m_embeddedObjects = new ArrayList(); m_referencedItems = new CanonicalXmlNodeList(); } // // public properties // public string Id { get { return m_id; } set { m_id = value; } } public SignedInfo SignedInfo { get { return m_signedInfo; } set { m_signedInfo = value; if (this.SignedXml != null && m_signedInfo != null) m_signedInfo.SignedXml = this.SignedXml; } } public byte[] SignatureValue { get { return m_signatureValue; } set { m_signatureValue = value; } } public KeyInfo KeyInfo { get { if (m_keyInfo == null) m_keyInfo = new KeyInfo(); return m_keyInfo; } set { m_keyInfo = value; } } public IList ObjectList { get { return m_embeddedObjects; } set { m_embeddedObjects = value; } } internal CanonicalXmlNodeList ReferencedItems { get { return m_referencedItems; } } // // public methods // public XmlElement GetXml() { XmlDocument document = new XmlDocument(); document.PreserveWhitespace = true; return GetXml(document); } internal XmlElement GetXml (XmlDocument document) { // Create the Signature XmlElement signatureElement = (XmlElement)document.CreateElement("Signature", SignedXml.XmlDsigNamespaceUrl); if (!String.IsNullOrEmpty(m_id)) signatureElement.SetAttribute("Id", m_id); // Add the SignedInfo if (m_signedInfo == null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_SignedInfoRequired")); signatureElement.AppendChild(m_signedInfo.GetXml(document)); // Add the SignatureValue if (m_signatureValue == null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_SignatureValueRequired")); XmlElement signatureValueElement = document.CreateElement("SignatureValue", SignedXml.XmlDsigNamespaceUrl); signatureValueElement.AppendChild(document.CreateTextNode(Convert.ToBase64String(m_signatureValue))); if (!String.IsNullOrEmpty(m_signatureValueId)) signatureValueElement.SetAttribute("Id", m_signatureValueId); signatureElement.AppendChild(signatureValueElement); // Add the KeyInfo if (this.KeyInfo.Count > 0) signatureElement.AppendChild(this.KeyInfo.GetXml(document)); // Add the Objects foreach (Object obj in m_embeddedObjects) { DataObject dataObj = obj as DataObject; if (dataObj != null) { signatureElement.AppendChild(dataObj.GetXml(document)); } } return signatureElement; } public void LoadXml(XmlElement value) { // Make sure we don't get passed null if (value == null) throw new ArgumentNullException("value"); // Signature XmlElement signatureElement = value; if (!signatureElement.LocalName.Equals("Signature")) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_InvalidElement"), "Signature"); // Id attribute -- optional m_id = Utils.GetAttribute(signatureElement, "Id", SignedXml.XmlDsigNamespaceUrl); XmlNamespaceManager nsm = new XmlNamespaceManager(value.OwnerDocument.NameTable); nsm.AddNamespace("ds", SignedXml.XmlDsigNamespaceUrl); // SignedInfo XmlElement signedInfoElement = signatureElement.SelectSingleNode("ds:SignedInfo", nsm) as XmlElement; if (signedInfoElement == null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_InvalidElement"),"SignedInfo"); this.SignedInfo = new SignedInfo(); this.SignedInfo.LoadXml(signedInfoElement); // SignatureValue XmlElement signatureValueElement = signatureElement.SelectSingleNode("ds:SignatureValue", nsm) as XmlElement; if (signatureValueElement == null) throw new CryptographicException(SecurityResources.GetResourceString("Cryptography_Xml_InvalidElement"),"SignedInfo/SignatureValue"); m_signatureValue = Convert.FromBase64String(Utils.DiscardWhiteSpaces(signatureValueElement.InnerText)); m_signatureValueId = Utils.GetAttribute(signatureValueElement, "Id", SignedXml.XmlDsigNamespaceUrl); XmlNodeList keyInfoNodes = signatureElement.SelectNodes("ds:KeyInfo", nsm); m_keyInfo = new KeyInfo(); if (keyInfoNodes != null) { foreach(XmlNode node in keyInfoNodes) { XmlElement keyInfoElement = node as XmlElement; if (keyInfoElement != null) m_keyInfo.LoadXml(keyInfoElement); } } XmlNodeList objectNodes = signatureElement.SelectNodes("ds:Object", nsm); m_embeddedObjects.Clear(); if (objectNodes != null) { foreach(XmlNode node in objectNodes) { XmlElement objectElement = node as XmlElement; if (objectElement != null) { DataObject dataObj = new DataObject(); dataObj.LoadXml(objectElement); m_embeddedObjects.Add(dataObj); } } } // Select all elements that have Id attributes XmlNodeList nodeList = signatureElement.SelectNodes("//*[@Id]", nsm); if (nodeList != null) { foreach (XmlNode node in nodeList) { m_referencedItems.Add(node); } } } public void AddObject(DataObject dataObject) { m_embeddedObjects.Add(dataObject); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
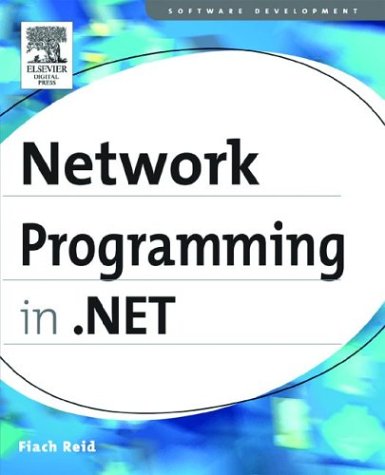
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RectangleF.cs
- FixedTextView.cs
- ControlIdConverter.cs
- ObjectComplexPropertyMapping.cs
- TrackingProvider.cs
- ManagementDateTime.cs
- TaskbarItemInfo.cs
- ObjectTag.cs
- AsymmetricSignatureDeformatter.cs
- SettingsPropertyValueCollection.cs
- HtmlControlPersistable.cs
- DbgCompiler.cs
- _UriSyntax.cs
- RegistrySecurity.cs
- ProxyHelper.cs
- ISFClipboardData.cs
- EntityDataSourceValidationException.cs
- SchemaEntity.cs
- AdapterUtil.cs
- _IPv4Address.cs
- XmlElementList.cs
- InstanceView.cs
- MetadataArtifactLoaderComposite.cs
- DictionaryChange.cs
- RegexCaptureCollection.cs
- IconConverter.cs
- TextTreeTextElementNode.cs
- SoapWriter.cs
- Message.cs
- CuspData.cs
- FrameworkElement.cs
- XsltInput.cs
- TransformPattern.cs
- DocumentReferenceCollection.cs
- EntryIndex.cs
- RefreshEventArgs.cs
- ZoneButton.cs
- ValidationError.cs
- DBConcurrencyException.cs
- BaseParser.cs
- GlyphElement.cs
- X509CertificateClaimSet.cs
- InstanceLockedException.cs
- GridViewColumnHeaderAutomationPeer.cs
- SqlTopReducer.cs
- CompModSwitches.cs
- Point4D.cs
- EventBuilder.cs
- DrawingState.cs
- FieldToken.cs
- DecoderNLS.cs
- ResourcesGenerator.cs
- SwitchLevelAttribute.cs
- TabControl.cs
- MetadataStore.cs
- FormattedText.cs
- FigureHelper.cs
- SafeHandles.cs
- CollectionViewProxy.cs
- XsltSettings.cs
- FormViewPageEventArgs.cs
- RelatedImageListAttribute.cs
- CssClassPropertyAttribute.cs
- Pts.cs
- ExpressionBinding.cs
- WmiEventSink.cs
- Regex.cs
- XmlSchemaSimpleTypeUnion.cs
- ItemDragEvent.cs
- DesignerProperties.cs
- RuntimeIdentifierPropertyAttribute.cs
- _CommandStream.cs
- QuaternionAnimation.cs
- DispatcherFrame.cs
- EmptyStringExpandableObjectConverter.cs
- GlobalItem.cs
- JsonReaderDelegator.cs
- StringUtil.cs
- TreeView.cs
- FrameworkElement.cs
- OracleColumn.cs
- CrossSiteScriptingValidation.cs
- SafeCertificateContext.cs
- HttpRequestWrapper.cs
- DBSchemaTable.cs
- UnknownMessageReceivedEventArgs.cs
- BamlTreeUpdater.cs
- OdbcInfoMessageEvent.cs
- ValuePattern.cs
- EventListenerClientSide.cs
- XmlIterators.cs
- TableStyle.cs
- NamespaceQuery.cs
- SqlPersonalizationProvider.cs
- ValueTable.cs
- Exceptions.cs
- PreviewPrintController.cs
- AlternationConverter.cs
- WebPartConnection.cs
- ToolStripHighContrastRenderer.cs