Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / Point4D.cs / 1305600 / Point4D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 4D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point4D - 4D point representation. /// Defaults to (0,0,0,0). /// public partial struct Point4D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. /// Value of the W coordinate of the new point. public Point4D(double x, double y, double z, double w) { _x = x; _y = y; _z = z; _w = w; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding deltaX to X, deltaY to Y, deltaZ to Z, and deltaW to W. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. /// Offset in the W direction. public void Offset(double deltaX, double deltaY, double deltaZ, double deltaW) { _x += deltaX; _y += deltaY; _z += deltaZ; _w += deltaW; } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D operator +(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D Add(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D operator -(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D Subtract(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D operator *(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D Multiply(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 4D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/04/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point4D - 4D point representation. /// Defaults to (0,0,0,0). /// public partial struct Point4D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. /// Value of the W coordinate of the new point. public Point4D(double x, double y, double z, double w) { _x = x; _y = y; _z = z; _w = w; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding deltaX to X, deltaY to Y, deltaZ to Z, and deltaW to W. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. /// Offset in the W direction. public void Offset(double deltaX, double deltaY, double deltaZ, double deltaW) { _x += deltaX; _y += deltaY; _z += deltaZ; _w += deltaW; } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D operator +(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Addition. /// /// First point being added. /// Second point being added. ///Result of addition. public static Point4D Add(Point4D point1, Point4D point2) { return new Point4D(point1._x + point2._x, point1._y + point2._y, point1._z + point2._z, point1._w + point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D operator -(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Point4D Subtract(Point4D point1, Point4D point2) { return new Point4D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z, point1._w - point2._w); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D operator *(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point4D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point4D Multiply(Point4D point, Matrix3D matrix) { return matrix.Transform(point); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
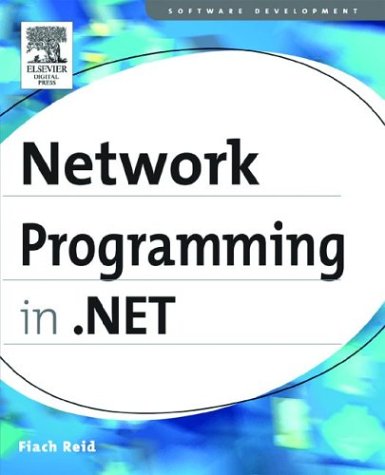
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutSettings.cs
- RijndaelManagedTransform.cs
- DoubleUtil.cs
- TransactionFlowElement.cs
- TemplateComponentConnector.cs
- BindingNavigator.cs
- HelloOperationCD1AsyncResult.cs
- HierarchicalDataBoundControlAdapter.cs
- HwndAppCommandInputProvider.cs
- CaseStatement.cs
- DetailsViewRowCollection.cs
- EventMappingSettingsCollection.cs
- ProvidePropertyAttribute.cs
- Queue.cs
- ServiceDefaults.cs
- DataTableReaderListener.cs
- SqlConnectionStringBuilder.cs
- SetStoryboardSpeedRatio.cs
- SmtpNtlmAuthenticationModule.cs
- XsltArgumentList.cs
- JavascriptCallbackResponseProperty.cs
- TransformerInfoCollection.cs
- SvcMapFileLoader.cs
- FramingChannels.cs
- Model3D.cs
- XmlCodeExporter.cs
- AxisAngleRotation3D.cs
- WSTrust.cs
- XmlNamespaceManager.cs
- TransactionBridgeSection.cs
- _ScatterGatherBuffers.cs
- UInt32Converter.cs
- ColumnCollection.cs
- WindowPattern.cs
- DnsEndPoint.cs
- oledbmetadatacolumnnames.cs
- RemotingAttributes.cs
- EventOpcode.cs
- HttpBindingExtension.cs
- VectorAnimationBase.cs
- IndexerNameAttribute.cs
- WindowsFormsLinkLabel.cs
- AttachmentCollection.cs
- Verify.cs
- TransformConverter.cs
- MouseGestureConverter.cs
- ScriptBehaviorDescriptor.cs
- SerTrace.cs
- GroupBox.cs
- HotSpotCollectionEditor.cs
- StrokeCollection2.cs
- CreatingCookieEventArgs.cs
- FrugalList.cs
- FontEditor.cs
- StopStoryboard.cs
- BitmapDownload.cs
- EventLogTraceListener.cs
- XamlClipboardData.cs
- ImplicitInputBrush.cs
- Point.cs
- GotoExpression.cs
- PtsHelper.cs
- NamedServiceModelExtensionCollectionElement.cs
- Image.cs
- ContextProperty.cs
- ByteArrayHelperWithString.cs
- MimeParameterWriter.cs
- RevocationPoint.cs
- AuthenticationManager.cs
- UrlMappingCollection.cs
- BinaryUtilClasses.cs
- ColorTranslator.cs
- PointAnimationUsingPath.cs
- SqlDataSourceConfigureFilterForm.cs
- Signature.cs
- AstNode.cs
- UserControl.cs
- HttpPostClientProtocol.cs
- StylusPointPropertyUnit.cs
- ListViewDeleteEventArgs.cs
- SqlDependencyListener.cs
- CustomAttributeFormatException.cs
- DataGridViewTextBoxColumn.cs
- InputLanguageSource.cs
- EntityParameterCollection.cs
- FlowLayoutPanel.cs
- ConstraintConverter.cs
- WebPartCatalogCloseVerb.cs
- BamlRecordHelper.cs
- SeekableReadStream.cs
- DeviceContext.cs
- MatchSingleFxEngineOpcode.cs
- WinFormsUtils.cs
- XmlUrlResolver.cs
- RootBrowserWindow.cs
- wgx_sdk_version.cs
- AnimatedTypeHelpers.cs
- ServerIdentity.cs
- DelimitedListTraceListener.cs
- XmlSchemaSequence.cs