Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / SystemKeyConverter.cs / 1 / SystemKeyConverter.cs
//---------------------------------------------------------------------------- // // File: SystemKeyConverter.cs // // Description: // TypeConverter for SystemResourceKey and SystemThemeKey. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; using System.Windows.Controls; using System.Windows.Controls.Primitives; namespace System.Windows.Markup { ////// Common TypeConverter functionality SystemThemeKey and SystemResourceKey; each /// is an internal type, so gets serialized as an {x:Static} reference. /// internal class SystemKeyConverter : TypeConverter { ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert from /// ////// true if conversion is possible /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == null) { throw new ArgumentNullException("sourceType"); } return base.CanConvertFrom(context, sourceType); } ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert to /// ////// true if conversion is possible /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // Validate Input Arguments if (destinationType == null) { throw new ArgumentNullException("destinationType"); } else if( destinationType == typeof(MarkupExtension) && context is IValueSerializerContext ) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// ////// value that is result of conversion /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { return base.ConvertFrom(context, culture, value); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// /// /// Type to convert to /// ////// converted value /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { // Input validation if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(MarkupExtension) && CanConvertTo(context, destinationType) ) { SystemResourceKeyID keyId; // Get the SystemResourceKeyID if( value is SystemResourceKey ) { keyId = (value as SystemResourceKey).InternalKey; } else if( value is SystemThemeKey ) { keyId = (value as SystemThemeKey).InternalKey; } else { throw new ArgumentException(SR.Get(SRID.MustBeOfType, "value", "SystemResourceKey or SystemThemeKey")); } // System resource keys can be converted into a MarkupExtension (StaticExtension) Type keyType = SystemKeyConverter.GetSystemClassType(keyId); // Get the value serialization context IValueSerializerContext valueSerializerContext = context as IValueSerializerContext; if( valueSerializerContext != null ) { // And from that get a System.Type serializer ValueSerializer typeSerializer = valueSerializerContext.GetValueSerializerFor(typeof(Type)); if( typeSerializer != null ) { // And use that to create the string-ized class name string systemClassName = typeSerializer.ConvertToString(keyType, valueSerializerContext); // And finally create the StaticExtension. return new StaticExtension( systemClassName + "." + GetSystemKeyName(keyId) ); } } } return base.CanConvertTo(context, destinationType); } internal static Type GetSystemClassType(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemColorsEnd)) { return typeof(SystemColors); } else if ((SystemResourceKeyID.InternalSystemFontsStart < id) && (id < SystemResourceKeyID.InternalSystemFontsEnd)) { return typeof(SystemFonts); } else if ((SystemResourceKeyID.InternalSystemParametersStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return typeof(SystemParameters); } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id) { return typeof(MenuItem); } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { return typeof(ToolBar); } else if (SystemResourceKeyID.StatusBarSeparatorStyle == id) { return typeof(StatusBar); } else if ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle)) { return typeof(GridView); } return null; } internal static string GetSystemClassName(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemColorsEnd)) { return "SystemColors"; } else if ((SystemResourceKeyID.InternalSystemFontsStart < id) && (id < SystemResourceKeyID.InternalSystemFontsEnd)) { return "SystemFonts"; } else if ((SystemResourceKeyID.InternalSystemParametersStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return "SystemParameters"; } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id) { return "MenuItem"; } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { return "ToolBar"; } else if (SystemResourceKeyID.StatusBarSeparatorStyle == id) { return "StatusBar"; } else if ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle)) { return "GridView"; } return String.Empty; } internal static string GetSystemKeyName(SystemResourceKeyID id) { if (((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) || ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle))) { return Enum.GetName(typeof(SystemResourceKeyID), id) + "Key"; } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id || SystemResourceKeyID.StatusBarSeparatorStyle == id) { return "SeparatorStyleKey"; } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { string propName = Enum.GetName(typeof(SystemResourceKeyID), id) + "Key"; return propName.Remove(0, 7); // Remove the "ToolBar" prefix } return String.Empty; } internal static string GetSystemPropertyName(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return Enum.GetName(typeof(SystemResourceKeyID), id); } return String.Empty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: SystemKeyConverter.cs // // Description: // TypeConverter for SystemResourceKey and SystemThemeKey. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; using System.Windows.Controls; using System.Windows.Controls.Primitives; namespace System.Windows.Markup { ////// Common TypeConverter functionality SystemThemeKey and SystemResourceKey; each /// is an internal type, so gets serialized as an {x:Static} reference. /// internal class SystemKeyConverter : TypeConverter { ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert from /// ////// true if conversion is possible /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == null) { throw new ArgumentNullException("sourceType"); } return base.CanConvertFrom(context, sourceType); } ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert to /// ////// true if conversion is possible /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // Validate Input Arguments if (destinationType == null) { throw new ArgumentNullException("destinationType"); } else if( destinationType == typeof(MarkupExtension) && context is IValueSerializerContext ) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// ////// value that is result of conversion /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { return base.ConvertFrom(context, culture, value); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// /// /// Type to convert to /// ////// converted value /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { // Input validation if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(MarkupExtension) && CanConvertTo(context, destinationType) ) { SystemResourceKeyID keyId; // Get the SystemResourceKeyID if( value is SystemResourceKey ) { keyId = (value as SystemResourceKey).InternalKey; } else if( value is SystemThemeKey ) { keyId = (value as SystemThemeKey).InternalKey; } else { throw new ArgumentException(SR.Get(SRID.MustBeOfType, "value", "SystemResourceKey or SystemThemeKey")); } // System resource keys can be converted into a MarkupExtension (StaticExtension) Type keyType = SystemKeyConverter.GetSystemClassType(keyId); // Get the value serialization context IValueSerializerContext valueSerializerContext = context as IValueSerializerContext; if( valueSerializerContext != null ) { // And from that get a System.Type serializer ValueSerializer typeSerializer = valueSerializerContext.GetValueSerializerFor(typeof(Type)); if( typeSerializer != null ) { // And use that to create the string-ized class name string systemClassName = typeSerializer.ConvertToString(keyType, valueSerializerContext); // And finally create the StaticExtension. return new StaticExtension( systemClassName + "." + GetSystemKeyName(keyId) ); } } } return base.CanConvertTo(context, destinationType); } internal static Type GetSystemClassType(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemColorsEnd)) { return typeof(SystemColors); } else if ((SystemResourceKeyID.InternalSystemFontsStart < id) && (id < SystemResourceKeyID.InternalSystemFontsEnd)) { return typeof(SystemFonts); } else if ((SystemResourceKeyID.InternalSystemParametersStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return typeof(SystemParameters); } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id) { return typeof(MenuItem); } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { return typeof(ToolBar); } else if (SystemResourceKeyID.StatusBarSeparatorStyle == id) { return typeof(StatusBar); } else if ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle)) { return typeof(GridView); } return null; } internal static string GetSystemClassName(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemColorsEnd)) { return "SystemColors"; } else if ((SystemResourceKeyID.InternalSystemFontsStart < id) && (id < SystemResourceKeyID.InternalSystemFontsEnd)) { return "SystemFonts"; } else if ((SystemResourceKeyID.InternalSystemParametersStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return "SystemParameters"; } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id) { return "MenuItem"; } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { return "ToolBar"; } else if (SystemResourceKeyID.StatusBarSeparatorStyle == id) { return "StatusBar"; } else if ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle)) { return "GridView"; } return String.Empty; } internal static string GetSystemKeyName(SystemResourceKeyID id) { if (((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) || ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle))) { return Enum.GetName(typeof(SystemResourceKeyID), id) + "Key"; } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id || SystemResourceKeyID.StatusBarSeparatorStyle == id) { return "SeparatorStyleKey"; } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { string propName = Enum.GetName(typeof(SystemResourceKeyID), id) + "Key"; return propName.Remove(0, 7); // Remove the "ToolBar" prefix } return String.Empty; } internal static string GetSystemPropertyName(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return Enum.GetName(typeof(SystemResourceKeyID), id); } return String.Empty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
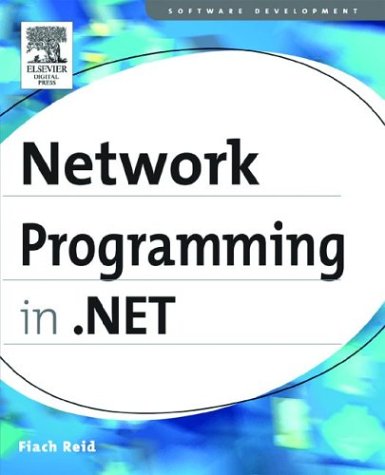
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BooleanAnimationUsingKeyFrames.cs
- TreeViewImageKeyConverter.cs
- XamlInt32CollectionSerializer.cs
- DictionaryGlobals.cs
- securitycriticaldataformultiplegetandset.cs
- TcpSocketManager.cs
- SplineQuaternionKeyFrame.cs
- ToolStripHighContrastRenderer.cs
- CurrentChangedEventManager.cs
- DynamicResourceExtensionConverter.cs
- MenuCommand.cs
- CodeRegionDirective.cs
- SingleResultAttribute.cs
- FunctionImportElement.cs
- DataObjectSettingDataEventArgs.cs
- AnimationClockResource.cs
- DesignTimeTemplateParser.cs
- OleDbRowUpdatingEvent.cs
- Matrix3DStack.cs
- SqlCachedBuffer.cs
- CompilerCollection.cs
- EntityDataSourceConfigureObjectContext.cs
- SoapSchemaMember.cs
- Latin1Encoding.cs
- Misc.cs
- HtmlImage.cs
- WebHttpDispatchOperationSelector.cs
- PageRanges.cs
- ManagedIStream.cs
- LocatorPartList.cs
- VirtualPathUtility.cs
- CngProvider.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- FontUnit.cs
- Int32RectValueSerializer.cs
- PrivilegedConfigurationManager.cs
- Hashtable.cs
- InstanceCreationEditor.cs
- StylusEditingBehavior.cs
- StateInitializationDesigner.cs
- HtmlAnchor.cs
- OdbcUtils.cs
- XmlObjectSerializerWriteContextComplex.cs
- XmlILOptimizerVisitor.cs
- WebEncodingValidatorAttribute.cs
- ToolStripMenuItem.cs
- CreateUserWizardStep.cs
- SourceSwitch.cs
- CodeObject.cs
- WindowsPen.cs
- LoginUtil.cs
- DetailsViewModeEventArgs.cs
- XsltQilFactory.cs
- HostingMessageProperty.cs
- EntityViewGenerationAttribute.cs
- DataGridViewRowPostPaintEventArgs.cs
- GridSplitterAutomationPeer.cs
- State.cs
- DataGridBoolColumn.cs
- EUCJPEncoding.cs
- HttpRuntime.cs
- ReflectionServiceProvider.cs
- EventLogReader.cs
- SmtpFailedRecipientException.cs
- OutArgumentConverter.cs
- DataGridLinkButton.cs
- MenuAdapter.cs
- MembershipUser.cs
- StyleSheetDesigner.cs
- ActivityDesignerResources.cs
- WebServicesSection.cs
- DataMemberConverter.cs
- PartialClassGenerationTask.cs
- PrimitiveXmlSerializers.cs
- NullRuntimeConfig.cs
- ShowExpandedMultiValueConverter.cs
- CellQuery.cs
- MinimizableAttributeTypeConverter.cs
- SecurityRuntime.cs
- DeflateStream.cs
- TreeNodeBindingCollection.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- NewItemsContextMenuStrip.cs
- SQLDecimalStorage.cs
- ActivityValidator.cs
- Utils.cs
- StructuredTypeEmitter.cs
- NativeRecognizer.cs
- WorkflowRuntimeServicesBehavior.cs
- DataGridViewSelectedColumnCollection.cs
- WebPartManager.cs
- XmlQualifiedNameTest.cs
- ReaderWriterLockWrapper.cs
- SettingsPropertyWrongTypeException.cs
- TableColumnCollectionInternal.cs
- ValidationVisibilityAttribute.cs
- ProtocolsConfigurationEntry.cs
- ListViewGroup.cs
- DoubleConverter.cs
- NativeMethodsOther.cs