Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / Util / Misc.cs / 1305376 / Misc.cs
using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Data; using System.Diagnostics; using System.Globalization; using System.Linq; using System.Linq.Expressions; using System.Text; using System.Web.DynamicData.Util; using System.Web.Resources; using System.Web.Routing; using System.Web.UI; using System.Web.UI.WebControls; namespace System.Web.DynamicData { internal static class Misc { public static HttpContextWrapper ToWrapper(this HttpContext context) { return new HttpContextWrapper(context); } public static object GetRealDataItem(object dataItem) { if (dataItem is ICustomTypeDescriptor) { // Unwrap EF object dataItem = ((ICustomTypeDescriptor)dataItem).GetPropertyOwner(null); } return dataItem; } // Walks the type hierachy up to endingType (assuming startingType is a subtype of starting type) // trying to find a meta table. public static MetaTable GetTableFromTypeHierarchy(Type entityType) { if (entityType == null) { throw new ArgumentNullException("entityType"); } Type type = entityType; while (type != null) { MetaTable table; if (MetaTable.TryGetTable(type, out table)) { return table; } type = type.BaseType; } return null; } public static Type RemoveNullableFromType(Type type) { return Nullable.GetUnderlyingType(type) ?? type; } // Copied from System.Web.UI.WebControls.DataBoundControlHelper.IsBindableType // and extended to support enums. public static bool IsBindableType(Type type) { if (type == null) { return false; } // HACK: We're excluding this type since objects generated by ef's designer (excluding poco) // derive from EntityObject which has this "EntityState" enum that the user may not have indended to show in their UI. if (type == typeof(EntityState)) { return false; } // If the type is Nullable then it has an underlying type, in which case // we want to check the underlying type for bindability. type = RemoveNullableFromType(type); return (type.IsPrimitive || type.IsEnum || (type == typeof(string)) || (type == typeof(DateTime)) || (type == typeof(Decimal)) || (type == typeof(Guid)) || (type == typeof(DateTimeOffset)) || (type == typeof(TimeSpan))); } internal static bool IsColumnInDictionary(IMetaColumn column, IDictionaryvalues) { if (column == null) { throw new ArgumentNullException("column"); } if (values == null) { throw new ArgumentNullException("values"); } IMetaForeignKeyColumn foreignKeyColumn = column as IMetaForeignKeyColumn; if (foreignKeyColumn != null) { return foreignKeyColumn.ForeignKeyNames.All(fkName => values.ContainsKey(fkName)); } return values.ContainsKey(column.Name); } internal static IDictionary ConvertObjectToDictionary(object instance) { if (instance == null) { throw new ArgumentNullException("instance"); } Dictionary values = new Dictionary (); var props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor p in props) { values[p.Name] = p.GetValue(instance); } return values; } public static T ChangeType (object value) { return (T)ChangeType(value, typeof(T)); } public static object ChangeType(object value, Type type) { if (type == null) { throw new ArgumentNullException("type"); } if (value == null) { if (TypeAllowsNull(type)) { return null; } return Convert.ChangeType(value, type, CultureInfo.CurrentCulture); } type = RemoveNullableFromType(type); if (value.GetType() == type) { return value; } TypeConverter converter = TypeDescriptor.GetConverter(type); if (converter.CanConvertFrom(value.GetType())) { return converter.ConvertFrom(value); } TypeConverter otherConverter = TypeDescriptor.GetConverter(value.GetType()); if (otherConverter.CanConvertTo(type)) { return otherConverter.ConvertTo(value, type); } throw new InvalidOperationException(String.Format( CultureInfo.CurrentCulture, DynamicDataResources.Misc_CannotConvertType, value.GetType(), type)); } internal static bool TypeAllowsNull(Type type) { return Nullable.GetUnderlyingType(type) != null || !type.IsValueType; } public static ContainerType FindContainerType(Control control) { if (control == null) { throw new ArgumentNullException("control"); } Control container = control; // Walk up NamingContainers until we find one of the DataBound control interfaces while (container != null) { #if !ORYX_VNEXT if (container is IDataBoundItemControl) { return ContainerType.Item; } else if (container is IDataBoundListControl || container is Repeater) { return ContainerType.List; } #else if (container is GridView || container is ListView || container is Repeater) { return ContainerType.List; } if (container is FormView || container is DetailsView) { return ContainerType.Item; } #endif container = container.NamingContainer; } // Default container type is a list if none of the known // interfaces are found return ContainerType.List; } public static IOrderedDictionary GetEnumNamesAndValues(Type enumType) { Debug.Assert(enumType != null); Debug.Assert(enumType.IsEnum); OrderedDictionary result = new OrderedDictionary(); var enumEntries = from e in Enum.GetValues(enumType).OfType
Link Menu
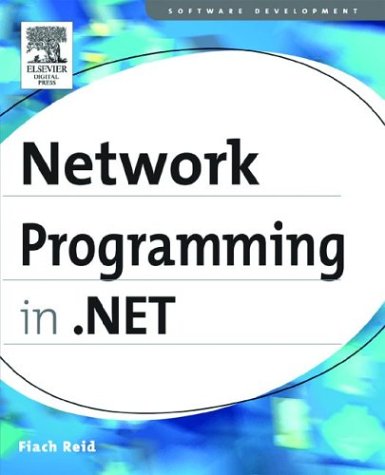
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageParser.cs
- XmlComplianceUtil.cs
- ConfigXmlSignificantWhitespace.cs
- SqlInternalConnectionSmi.cs
- _Connection.cs
- ValueChangedEventManager.cs
- PropertyPathWorker.cs
- arc.cs
- Table.cs
- BindToObject.cs
- DescendantOverDescendantQuery.cs
- ToolboxItem.cs
- ProcessInputEventArgs.cs
- ToolStripInSituService.cs
- WindowCollection.cs
- SpecialFolderEnumConverter.cs
- TextBlockAutomationPeer.cs
- SQLRoleProvider.cs
- AttributeUsageAttribute.cs
- XPathAncestorQuery.cs
- SByteStorage.cs
- DetailsViewRowCollection.cs
- FreeFormDesigner.cs
- KnownBoxes.cs
- DataBoundControlAdapter.cs
- GenericIdentity.cs
- FastEncoderWindow.cs
- DelegateBodyWriter.cs
- ExportOptions.cs
- SqlWorkflowInstanceStore.cs
- ServiceDebugElement.cs
- OleDbConnection.cs
- ControlTemplate.cs
- XmlILTrace.cs
- SrgsRulesCollection.cs
- ResourceExpressionBuilder.cs
- shaperfactoryquerycachekey.cs
- Utils.cs
- CacheOutputQuery.cs
- BaseDataBoundControlDesigner.cs
- RootProfilePropertySettingsCollection.cs
- SqlDataSourceView.cs
- SystemPens.cs
- ConfigurationPermission.cs
- Exceptions.cs
- RequestNavigateEventArgs.cs
- Padding.cs
- TheQuery.cs
- FilterQuery.cs
- RelationshipEndCollection.cs
- NeutralResourcesLanguageAttribute.cs
- DoubleUtil.cs
- ManagementDateTime.cs
- TraceContext.cs
- InboundActivityHelper.cs
- PersonalizationStateInfoCollection.cs
- TextEditorMouse.cs
- CodeDelegateCreateExpression.cs
- CodeCompiler.cs
- ZoneIdentityPermission.cs
- TypeSystemProvider.cs
- SourceInterpreter.cs
- HtmlInputHidden.cs
- keycontainerpermission.cs
- StringDictionary.cs
- TextRangeBase.cs
- StreamGeometry.cs
- CopyOfAction.cs
- DragDropHelper.cs
- HtmlControlPersistable.cs
- ExplicitDiscriminatorMap.cs
- Compress.cs
- SimpleWebHandlerParser.cs
- ChameleonKey.cs
- HtmlInputRadioButton.cs
- RIPEMD160.cs
- _NtlmClient.cs
- LabelLiteral.cs
- XmlSchemaAnyAttribute.cs
- WebPartCancelEventArgs.cs
- IntranetCredentialPolicy.cs
- CompilerScopeManager.cs
- Table.cs
- shaper.cs
- MasterPage.cs
- JsonDeserializer.cs
- EntityContainer.cs
- RequestQueryParser.cs
- Environment.cs
- ByteStack.cs
- WebBrowserBase.cs
- EnumBuilder.cs
- SqlDataSource.cs
- ListItemCollection.cs
- WrappedIUnknown.cs
- SystemResources.cs
- BaseComponentEditor.cs
- ConnectionProviderAttribute.cs
- ImmutablePropertyDescriptorGridEntry.cs
- CodeSubDirectoriesCollection.cs