Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / TextChangedEventArgs.cs / 1 / TextChangedEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextChanged event argument. // // History: // 09/27/2004 : psarrett - Created // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; namespace System.Windows.Controls { #region TextChangedEvent ////// How the undo stack caused or is affected by a text change. /// public enum UndoAction { ////// This change will not affect the undo stack at all /// None = 0, ////// This change will merge into the previous undo unit /// Merge = 1, ////// This change is the result of a call to Undo() /// Undo = 2, ////// This change is the result of a call to Redo() /// Redo = 3, ////// This change will clear the undo stack /// Clear = 4, ////// This change will create a new undo unit /// Create = 5 } ////// The delegate to use for handlers that receive TextChangedEventArgs /// public delegate void TextChangedEventHandler(object sender, TextChangedEventArgs e); ////// The TextChangedEventArgs class represents a type of RoutedEventArgs that /// are relevant to events raised by changing editable text. /// public class TextChangedEventArgs : RoutedEventArgs { ////// constructor /// /// event id /// UndoAction /// ReadOnlyCollection public TextChangedEventArgs(RoutedEvent id, UndoAction action, ICollectionchanges) : base() { if (id == null) { throw new ArgumentNullException("id"); } if (action < UndoAction.None || action > UndoAction.Create) { throw new InvalidEnumArgumentException("action", (int)action, typeof(UndoAction)); } RoutedEvent=id; _undoAction = action; _changes = changes; } /// /// constructor /// /// event id /// UndoAction public TextChangedEventArgs(RoutedEvent id, UndoAction action) : this(id, action, new ReadOnlyCollection(new List ())) { } /// /// How the undo stack caused or is affected by this text change /// ///UndoAction enum public UndoAction UndoAction { get { return _undoAction; } } ////// A readonly list of TextChanges, sorted by offset in order from the beginning to the end of /// the document, describing the dirty areas of the document and in what way(s) those /// areas differ from the pre-change document. For example, if content at offset 0 was: /// /// The quick brown fox jumps over the lazy dog. /// /// and changed to: /// /// The brown fox jumps over a dog. /// /// Changes would contain two TextChanges with the following information: /// /// Change 1 /// -------- /// Offset: 5 /// RemovedCount: 6 /// AddedCount: 0 /// PropertyCount: 0 /// /// Change 2 /// -------- /// Offset: 26 /// RemovedCount: 8 /// AddedCount: 1 /// PropertyCount: 0 /// /// If no changes were made, the collection will be empty. /// public ICollectionChanges { get { return _changes; } } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { TextChangedEventHandler handler; handler = (TextChangedEventHandler)genericHandler; handler(genericTarget, this); } private UndoAction _undoAction; private readonly ICollection_changes; } #endregion TextChangedEvent } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextChanged event argument. // // History: // 09/27/2004 : psarrett - Created // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Documents; using System.Collections.Generic; using System.Collections.ObjectModel; namespace System.Windows.Controls { #region TextChangedEvent ////// How the undo stack caused or is affected by a text change. /// public enum UndoAction { ////// This change will not affect the undo stack at all /// None = 0, ////// This change will merge into the previous undo unit /// Merge = 1, ////// This change is the result of a call to Undo() /// Undo = 2, ////// This change is the result of a call to Redo() /// Redo = 3, ////// This change will clear the undo stack /// Clear = 4, ////// This change will create a new undo unit /// Create = 5 } ////// The delegate to use for handlers that receive TextChangedEventArgs /// public delegate void TextChangedEventHandler(object sender, TextChangedEventArgs e); ////// The TextChangedEventArgs class represents a type of RoutedEventArgs that /// are relevant to events raised by changing editable text. /// public class TextChangedEventArgs : RoutedEventArgs { ////// constructor /// /// event id /// UndoAction /// ReadOnlyCollection public TextChangedEventArgs(RoutedEvent id, UndoAction action, ICollectionchanges) : base() { if (id == null) { throw new ArgumentNullException("id"); } if (action < UndoAction.None || action > UndoAction.Create) { throw new InvalidEnumArgumentException("action", (int)action, typeof(UndoAction)); } RoutedEvent=id; _undoAction = action; _changes = changes; } /// /// constructor /// /// event id /// UndoAction public TextChangedEventArgs(RoutedEvent id, UndoAction action) : this(id, action, new ReadOnlyCollection(new List ())) { } /// /// How the undo stack caused or is affected by this text change /// ///UndoAction enum public UndoAction UndoAction { get { return _undoAction; } } ////// A readonly list of TextChanges, sorted by offset in order from the beginning to the end of /// the document, describing the dirty areas of the document and in what way(s) those /// areas differ from the pre-change document. For example, if content at offset 0 was: /// /// The quick brown fox jumps over the lazy dog. /// /// and changed to: /// /// The brown fox jumps over a dog. /// /// Changes would contain two TextChanges with the following information: /// /// Change 1 /// -------- /// Offset: 5 /// RemovedCount: 6 /// AddedCount: 0 /// PropertyCount: 0 /// /// Change 2 /// -------- /// Offset: 26 /// RemovedCount: 8 /// AddedCount: 1 /// PropertyCount: 0 /// /// If no changes were made, the collection will be empty. /// public ICollectionChanges { get { return _changes; } } /// /// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { TextChangedEventHandler handler; handler = (TextChangedEventHandler)genericHandler; handler(genericTarget, this); } private UndoAction _undoAction; private readonly ICollection_changes; } #endregion TextChangedEvent } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
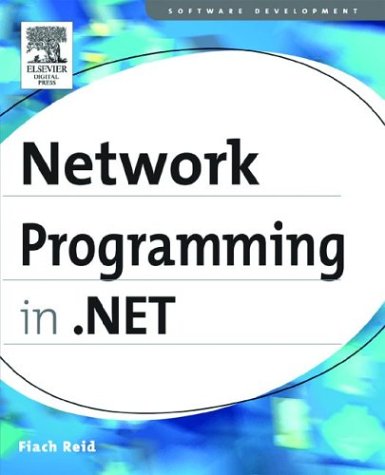
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReflectionServiceProvider.cs
- SafeLocalMemHandle.cs
- DictionaryKeyPropertyAttribute.cs
- XmlSerializerVersionAttribute.cs
- GridViewDeleteEventArgs.cs
- ResXBuildProvider.cs
- XmlArrayAttribute.cs
- ExtentKey.cs
- WebPartsPersonalizationAuthorization.cs
- MarginsConverter.cs
- DbMetaDataColumnNames.cs
- Pen.cs
- OdbcDataAdapter.cs
- UnsafeNativeMethods.cs
- CodeVariableDeclarationStatement.cs
- UpdateCompiler.cs
- PaintValueEventArgs.cs
- DefaultBinder.cs
- ActionNotSupportedException.cs
- MulticastOption.cs
- FastEncoderWindow.cs
- Composition.cs
- MissingManifestResourceException.cs
- ExceptQueryOperator.cs
- UnmanagedMemoryStream.cs
- DataListCommandEventArgs.cs
- Menu.cs
- TextDecorations.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- UIElementCollection.cs
- Viewport3DAutomationPeer.cs
- ScriptingRoleServiceSection.cs
- SQLByte.cs
- Debug.cs
- UnsafeNativeMethods.cs
- OdbcConnectionStringbuilder.cs
- XmlSchemaChoice.cs
- ScaleTransform3D.cs
- Enum.cs
- ExtenderControl.cs
- OneOfScalarConst.cs
- FormsAuthenticationUser.cs
- InternalControlCollection.cs
- Debug.cs
- Vector3DValueSerializer.cs
- MouseOverProperty.cs
- IconConverter.cs
- UIElement.cs
- ReceiveMessageAndVerifySecurityAsyncResultBase.cs
- XsltArgumentList.cs
- FilePresentation.cs
- OpCellTreeNode.cs
- WebPartsPersonalization.cs
- ServiceReference.cs
- ScrollBar.cs
- SQLMoney.cs
- ProcessProtocolHandler.cs
- HttpDictionary.cs
- DispatchChannelSink.cs
- PageCodeDomTreeGenerator.cs
- ObjectDisposedException.cs
- DataTableClearEvent.cs
- RequestCacheValidator.cs
- FixedSOMContainer.cs
- OleDbFactory.cs
- PrinterSettings.cs
- ImmutableObjectAttribute.cs
- XmlSchemaValidationException.cs
- _Win32.cs
- DataChangedEventManager.cs
- PropagatorResult.cs
- UnlockInstanceAsyncResult.cs
- DesignTimeTemplateParser.cs
- DetailsViewDeleteEventArgs.cs
- IsolationInterop.cs
- XsltException.cs
- COM2Enum.cs
- PlacementWorkspace.cs
- PreProcessInputEventArgs.cs
- ParagraphResult.cs
- SafeCertificateContext.cs
- ApplicationServicesHostFactory.cs
- Util.cs
- TreeViewHitTestInfo.cs
- ProcessHostConfigUtils.cs
- WhitespaceRule.cs
- IRCollection.cs
- SessionSwitchEventArgs.cs
- ByteAnimationBase.cs
- MULTI_QI.cs
- ListSortDescriptionCollection.cs
- TextBoxDesigner.cs
- ToolStripProgressBar.cs
- InvokeSchedule.cs
- ActivityWithResultWrapper.cs
- _Events.cs
- MsmqIntegrationBindingElement.cs
- DataGridColumnHeadersPresenter.cs
- Console.cs
- BreakSafeBase.cs