Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Generated / PolyLineSegment.cs / 1 / PolyLineSegment.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class PolyLineSegment : PathSegment { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PolyLineSegment Clone() { return (PolyLineSegment)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PolyLineSegment CloneCurrentValue() { return (PolyLineSegment)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Points - PointCollection. Default value is new FreezableDefaultValueFactory(PointCollection.Empty). /// public PointCollection Points { get { return (PointCollection) GetValue(PointsProperty); } set { SetValueInternal(PointsProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PolyLineSegment(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Points // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PolyLineSegment.Points property. /// public static readonly DependencyProperty PointsProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static PointCollection s_Points = PointCollection.Empty; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PolyLineSegment() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Points == null || s_Points.IsFrozen, "Detected context bound default value PolyLineSegment.s_Points (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(PolyLineSegment); PointsProperty = RegisterProperty("Points", typeof(PointCollection), typeofThis, new FreezableDefaultValueFactory(PointCollection.Empty), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class PolyLineSegment : PathSegment { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PolyLineSegment Clone() { return (PolyLineSegment)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PolyLineSegment CloneCurrentValue() { return (PolyLineSegment)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Points - PointCollection. Default value is new FreezableDefaultValueFactory(PointCollection.Empty). /// public PointCollection Points { get { return (PointCollection) GetValue(PointsProperty); } set { SetValueInternal(PointsProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PolyLineSegment(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Points // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PolyLineSegment.Points property. /// public static readonly DependencyProperty PointsProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal static PointCollection s_Points = PointCollection.Empty; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PolyLineSegment() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Points == null || s_Points.IsFrozen, "Detected context bound default value PolyLineSegment.s_Points (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(PolyLineSegment); PointsProperty = RegisterProperty("Points", typeof(PointCollection), typeofThis, new FreezableDefaultValueFactory(PointCollection.Empty), null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
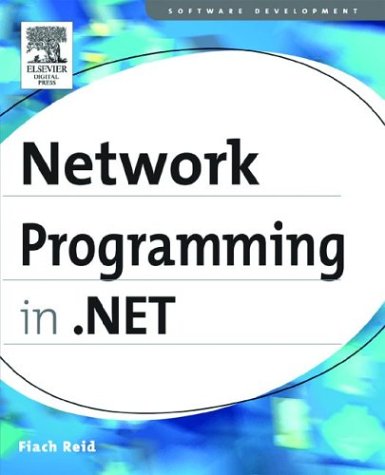
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Query.cs
- PtsContext.cs
- TreeNodeClickEventArgs.cs
- ObjectView.cs
- WebPartCatalogCloseVerb.cs
- Latin1Encoding.cs
- ViewStateException.cs
- DataBoundControl.cs
- EventListenerClientSide.cs
- IssuedTokenClientCredential.cs
- AssociationProvider.cs
- WebCategoryAttribute.cs
- EasingQuaternionKeyFrame.cs
- WindowsBrush.cs
- TrustSection.cs
- _NegoState.cs
- SqlUnionizer.cs
- GuidTagList.cs
- TimeZone.cs
- FontDifferentiator.cs
- CachedBitmap.cs
- DataGridViewImageCell.cs
- PropertyPathConverter.cs
- EntityDescriptor.cs
- LinkGrep.cs
- SessionPageStateSection.cs
- TCPClient.cs
- IProducerConsumerCollection.cs
- SimpleBitVector32.cs
- DataGridViewCellStateChangedEventArgs.cs
- HtmlShimManager.cs
- RightsManagementLicense.cs
- OrderingInfo.cs
- AttachedProperty.cs
- FileDataSourceCache.cs
- WebDescriptionAttribute.cs
- DiscoveryEndpointElement.cs
- QilValidationVisitor.cs
- WindowsTooltip.cs
- XmlChoiceIdentifierAttribute.cs
- TypeBuilder.cs
- CultureSpecificStringDictionary.cs
- PeerNodeTraceRecord.cs
- DoubleAnimationUsingPath.cs
- ScriptingAuthenticationServiceSection.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- HttpTransportBindingElement.cs
- CqlLexer.cs
- AppDomainProtocolHandler.cs
- TextBoxDesigner.cs
- PropertyChangedEventManager.cs
- ViewgenContext.cs
- BamlResourceDeserializer.cs
- PasswordTextNavigator.cs
- CacheVirtualItemsEvent.cs
- IIS7UserPrincipal.cs
- ProfileProvider.cs
- CurrentTimeZone.cs
- GridViewPageEventArgs.cs
- XmlQueryTypeFactory.cs
- BinHexEncoder.cs
- StringBuilder.cs
- CursorConverter.cs
- XmlNodeList.cs
- BindingMemberInfo.cs
- LOSFormatter.cs
- ClipboardData.cs
- XPathDescendantIterator.cs
- FrugalList.cs
- TraceSwitch.cs
- XamlPointCollectionSerializer.cs
- RotateTransform.cs
- ScriptManager.cs
- AesManaged.cs
- SimpleExpression.cs
- AlphaSortedEnumConverter.cs
- EpmContentDeSerializer.cs
- X509Certificate2Collection.cs
- GridViewDeleteEventArgs.cs
- Deflater.cs
- ToolStripDropDownClosedEventArgs.cs
- SqlDependencyUtils.cs
- QilParameter.cs
- ErrorTableItemStyle.cs
- InvariantComparer.cs
- EntityCommandCompilationException.cs
- ServiceCredentialsElement.cs
- WriterOutput.cs
- ModelTreeEnumerator.cs
- MiniMapControl.xaml.cs
- Lease.cs
- DataGridRowDetailsEventArgs.cs
- Encoder.cs
- Interlocked.cs
- TypeGeneratedEventArgs.cs
- AttributeUsageAttribute.cs
- WebResourceUtil.cs
- EnumValidator.cs
- Splitter.cs
- BitHelper.cs