Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / FamilyMapCollection.cs / 1 / FamilyMapCollection.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: FamilyMapCollection.cs // // Contents: FontFamilyMapCollection // // Created: 2-5-05 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Globalization; using SC=System.Collections; using System.Collections.Generic; using MS.Internal.FontFace; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// List of FontFamilyMap objects in a FontFamily, in lookup order. /// public sealed class FontFamilyMapCollection : IList, SC.IList { private const int InitialCapacity = 8; private CompositeFontInfo _fontInfo; private FontFamilyMap[] _items; private int _count; internal FontFamilyMapCollection(CompositeFontInfo fontInfo) { _fontInfo = fontInfo; _items = null; _count = 0; } #region IEnumerable members /// /// Returns an enumerator for iterating through the list. /// public IEnumeratorGetEnumerator() { return new Enumerator(_items, _count); } SC.IEnumerator SC.IEnumerable.GetEnumerator() { return new Enumerator(_items, _count); } #endregion #region ICollection methods /// /// Adds a FontFamilyMap to the font family. /// public void Add(FontFamilyMap item) { InsertItem(_count, item); } ////// Removes all FontFamilyMap objects from the FontFamily. /// public void Clear() { ClearItems(); } ////// Determines whether the FontFamily contains the specified FontFamilyMap. /// public bool Contains(FontFamilyMap item) { return FindItem(item) >= 0; } ////// Copies the contents of the list to the specified array. /// public void CopyTo(FontFamilyMap[] array, int index) { if (array == null) throw new ArgumentNullException("array"); if (index >= array.Length) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "index", "array")); if (_count > array.Length - index) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, index, "array")); if (_count != 0) Array.Copy(_items, 0, array, index, _count); } void SC.ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional)); Type elementType = array.GetType().GetElementType(); if (!elementType.IsAssignableFrom(typeof(FamilyTypeface))) throw new ArgumentException(SR.Get(SRID.CannotConvertType, typeof(FamilyTypeface), elementType)); if (index >= array.Length) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "index", "array")); if (_count > array.Length - index) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, index, "array")); if (_count != 0) Array.Copy(_items, 0, array, index, _count); } bool SC.ICollection.IsSynchronized { get { return false; } } object SC.ICollection.SyncRoot { get { return (_fontInfo != null) ? (object)_fontInfo : (object)this; } } ////// Removes the specified FontFamilyMap. /// public bool Remove(FontFamilyMap item) { VerifyChangeable(); int i = FindItem(item); if (i >= 0) { RemoveAt(i); return true; } return false; } ////// Gets the number of items in the list. /// public int Count { get { return _count; } } ////// Gets a value indicating whether the FontFamilyMap list can be changed. /// public bool IsReadOnly { get { return _fontInfo == null; } } #endregion #region IList members ////// Gets the index of the specified FontFamilyMap. /// public int IndexOf(FontFamilyMap item) { return FindItem(item); } ////// Inserts a FontFamilyMap into the list. /// public void Insert(int index, FontFamilyMap item) { InsertItem(index, item); } ////// Removes the FontFamilyMap at the specified index. /// public void RemoveAt(int index) { RemoveItem(index); } ////// Gets or sets the FontFamilyMap at the specified index. /// public FontFamilyMap this[int index] { get { RangeCheck(index); return _items[index]; } set { SetItem(index, value); } } int SC.IList.Add(object value) { return InsertItem(_count, ConvertValue(value)); } bool SC.IList.Contains(object value) { return FindItem(value as FontFamilyMap) >= 0; } int SC.IList.IndexOf(object value) { return FindItem(value as FontFamilyMap); } void SC.IList.Insert(int index, object item) { InsertItem(index, ConvertValue(item)); } void SC.IList.Remove(object value) { VerifyChangeable(); int i = FindItem(value as FontFamilyMap); if (i >= 0) RemoveItem(i); } bool SC.IList.IsFixedSize { get { return IsReadOnly; } } object SC.IList.this[int index] { get { RangeCheck(index); return _items[index]; } set { SetItem(index, ConvertValue(value)); } } #endregion #region Internal implementation private int InsertItem(int index, FontFamilyMap item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); // Limit the number of family maps because we use ushort indexes in the skip lists. // To exceed this limit a user would have to have a separate family maps for almost // every Unicode value, in which case (since we search sequentially) performance // would become a problem. if (_count + 1 >= ushort.MaxValue) throw new InvalidOperationException(SR.Get(SRID.CompositeFont_TooManyFamilyMaps)); // Validate the index. if (index < 0 || index > Count) throw new ArgumentOutOfRangeException("index"); // PrepareToAddFamilyMap validates the familyName and updates the internal state // of the CompositeFontInfo object. _fontInfo.PrepareToAddFamilyMap(item); // Make room for the new item. if (_items == null) { _items = new FontFamilyMap[InitialCapacity]; } else if (_count == _items.Length) { FontFamilyMap[] items = new FontFamilyMap[_count * 2]; for (int i = 0; i < index; ++i) items[i] = _items[i]; for (int i = index; i < _count; ++i) items[i + 1] = _items[i]; _items = items; } else if (index < _count) { for (int i = _count - 1; i >= index; --i) _items[i + 1] = _items[i]; } // Add the item. _items[index] = item; _count++; return index; } private void SetItem(int index, FontFamilyMap item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); RangeCheck(index); // PrepareToAddFamilyMap validates the familyName and updates the internal state // of the CompositeFontInfo object. _fontInfo.PrepareToAddFamilyMap(item); if (item.Language != _items[index].Language) { _fontInfo.InvalidateFamilyMapRanges(); } _items[index] = item; } private void ClearItems() { VerifyChangeable(); _fontInfo.InvalidateFamilyMapRanges(); _count = 0; _items = null; } private void RemoveItem(int index) { VerifyChangeable(); RangeCheck(index); _fontInfo.InvalidateFamilyMapRanges(); _count--; for (int i = index; i < _count; ++i) { _items[i] = _items[i + 1]; } _items[_count] = null; } private int FindItem(FontFamilyMap item) { if (_count != 0 && item != null) { for (int i = 0; i < _count; ++i) { if (_items[i].Equals(item)) return i; } } return -1; } private void RangeCheck(int index) { if (index < 0 || index >= _count) throw new ArgumentOutOfRangeException("index"); } private void VerifyChangeable() { if (_fontInfo == null) throw new NotSupportedException(SR.Get(SRID.General_ObjectIsReadOnly)); } private FontFamilyMap ConvertValue(object obj) { if (obj == null) throw new ArgumentNullException(); FontFamilyMap familyMap = obj as FontFamilyMap; if (familyMap == null) throw new ArgumentException(SR.Get(SRID.CannotConvertType, obj.GetType(), typeof(FontFamilyMap))); return familyMap; } private class Enumerator : IEnumerator, SC.IEnumerator { FontFamilyMap[] _items; int _count; int _index; FontFamilyMap _current; internal Enumerator(FontFamilyMap[] items, int count) { _items = items; _count = count; _index = -1; _current = null; } public bool MoveNext() { if (_index < _count) { _index++; if (_index < _count) { _current = _items[_index]; return true; } } _current = null; return false; } void SC.IEnumerator.Reset() { _index = -1; } public FontFamilyMap Current { get { return _current; } } object SC.IEnumerator.Current { get { // If there is no current item a non-generic IEnumerator should throw an exception, // but a generic IEnumerator is not required to. if (_current == null) throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); return _current; } } public void Dispose() { } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: FamilyMapCollection.cs // // Contents: FontFamilyMapCollection // // Created: 2-5-05 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Globalization; using SC=System.Collections; using System.Collections.Generic; using MS.Internal.FontFace; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { /// /// List of FontFamilyMap objects in a FontFamily, in lookup order. /// public sealed class FontFamilyMapCollection : IList, SC.IList { private const int InitialCapacity = 8; private CompositeFontInfo _fontInfo; private FontFamilyMap[] _items; private int _count; internal FontFamilyMapCollection(CompositeFontInfo fontInfo) { _fontInfo = fontInfo; _items = null; _count = 0; } #region IEnumerable members /// /// Returns an enumerator for iterating through the list. /// public IEnumeratorGetEnumerator() { return new Enumerator(_items, _count); } SC.IEnumerator SC.IEnumerable.GetEnumerator() { return new Enumerator(_items, _count); } #endregion #region ICollection methods /// /// Adds a FontFamilyMap to the font family. /// public void Add(FontFamilyMap item) { InsertItem(_count, item); } ////// Removes all FontFamilyMap objects from the FontFamily. /// public void Clear() { ClearItems(); } ////// Determines whether the FontFamily contains the specified FontFamilyMap. /// public bool Contains(FontFamilyMap item) { return FindItem(item) >= 0; } ////// Copies the contents of the list to the specified array. /// public void CopyTo(FontFamilyMap[] array, int index) { if (array == null) throw new ArgumentNullException("array"); if (index >= array.Length) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "index", "array")); if (_count > array.Length - index) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, index, "array")); if (_count != 0) Array.Copy(_items, 0, array, index, _count); } void SC.ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional)); Type elementType = array.GetType().GetElementType(); if (!elementType.IsAssignableFrom(typeof(FamilyTypeface))) throw new ArgumentException(SR.Get(SRID.CannotConvertType, typeof(FamilyTypeface), elementType)); if (index >= array.Length) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "index", "array")); if (_count > array.Length - index) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, index, "array")); if (_count != 0) Array.Copy(_items, 0, array, index, _count); } bool SC.ICollection.IsSynchronized { get { return false; } } object SC.ICollection.SyncRoot { get { return (_fontInfo != null) ? (object)_fontInfo : (object)this; } } ////// Removes the specified FontFamilyMap. /// public bool Remove(FontFamilyMap item) { VerifyChangeable(); int i = FindItem(item); if (i >= 0) { RemoveAt(i); return true; } return false; } ////// Gets the number of items in the list. /// public int Count { get { return _count; } } ////// Gets a value indicating whether the FontFamilyMap list can be changed. /// public bool IsReadOnly { get { return _fontInfo == null; } } #endregion #region IList members ////// Gets the index of the specified FontFamilyMap. /// public int IndexOf(FontFamilyMap item) { return FindItem(item); } ////// Inserts a FontFamilyMap into the list. /// public void Insert(int index, FontFamilyMap item) { InsertItem(index, item); } ////// Removes the FontFamilyMap at the specified index. /// public void RemoveAt(int index) { RemoveItem(index); } ////// Gets or sets the FontFamilyMap at the specified index. /// public FontFamilyMap this[int index] { get { RangeCheck(index); return _items[index]; } set { SetItem(index, value); } } int SC.IList.Add(object value) { return InsertItem(_count, ConvertValue(value)); } bool SC.IList.Contains(object value) { return FindItem(value as FontFamilyMap) >= 0; } int SC.IList.IndexOf(object value) { return FindItem(value as FontFamilyMap); } void SC.IList.Insert(int index, object item) { InsertItem(index, ConvertValue(item)); } void SC.IList.Remove(object value) { VerifyChangeable(); int i = FindItem(value as FontFamilyMap); if (i >= 0) RemoveItem(i); } bool SC.IList.IsFixedSize { get { return IsReadOnly; } } object SC.IList.this[int index] { get { RangeCheck(index); return _items[index]; } set { SetItem(index, ConvertValue(value)); } } #endregion #region Internal implementation private int InsertItem(int index, FontFamilyMap item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); // Limit the number of family maps because we use ushort indexes in the skip lists. // To exceed this limit a user would have to have a separate family maps for almost // every Unicode value, in which case (since we search sequentially) performance // would become a problem. if (_count + 1 >= ushort.MaxValue) throw new InvalidOperationException(SR.Get(SRID.CompositeFont_TooManyFamilyMaps)); // Validate the index. if (index < 0 || index > Count) throw new ArgumentOutOfRangeException("index"); // PrepareToAddFamilyMap validates the familyName and updates the internal state // of the CompositeFontInfo object. _fontInfo.PrepareToAddFamilyMap(item); // Make room for the new item. if (_items == null) { _items = new FontFamilyMap[InitialCapacity]; } else if (_count == _items.Length) { FontFamilyMap[] items = new FontFamilyMap[_count * 2]; for (int i = 0; i < index; ++i) items[i] = _items[i]; for (int i = index; i < _count; ++i) items[i + 1] = _items[i]; _items = items; } else if (index < _count) { for (int i = _count - 1; i >= index; --i) _items[i + 1] = _items[i]; } // Add the item. _items[index] = item; _count++; return index; } private void SetItem(int index, FontFamilyMap item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); RangeCheck(index); // PrepareToAddFamilyMap validates the familyName and updates the internal state // of the CompositeFontInfo object. _fontInfo.PrepareToAddFamilyMap(item); if (item.Language != _items[index].Language) { _fontInfo.InvalidateFamilyMapRanges(); } _items[index] = item; } private void ClearItems() { VerifyChangeable(); _fontInfo.InvalidateFamilyMapRanges(); _count = 0; _items = null; } private void RemoveItem(int index) { VerifyChangeable(); RangeCheck(index); _fontInfo.InvalidateFamilyMapRanges(); _count--; for (int i = index; i < _count; ++i) { _items[i] = _items[i + 1]; } _items[_count] = null; } private int FindItem(FontFamilyMap item) { if (_count != 0 && item != null) { for (int i = 0; i < _count; ++i) { if (_items[i].Equals(item)) return i; } } return -1; } private void RangeCheck(int index) { if (index < 0 || index >= _count) throw new ArgumentOutOfRangeException("index"); } private void VerifyChangeable() { if (_fontInfo == null) throw new NotSupportedException(SR.Get(SRID.General_ObjectIsReadOnly)); } private FontFamilyMap ConvertValue(object obj) { if (obj == null) throw new ArgumentNullException(); FontFamilyMap familyMap = obj as FontFamilyMap; if (familyMap == null) throw new ArgumentException(SR.Get(SRID.CannotConvertType, obj.GetType(), typeof(FontFamilyMap))); return familyMap; } private class Enumerator : IEnumerator, SC.IEnumerator { FontFamilyMap[] _items; int _count; int _index; FontFamilyMap _current; internal Enumerator(FontFamilyMap[] items, int count) { _items = items; _count = count; _index = -1; _current = null; } public bool MoveNext() { if (_index < _count) { _index++; if (_index < _count) { _current = _items[_index]; return true; } } _current = null; return false; } void SC.IEnumerator.Reset() { _index = -1; } public FontFamilyMap Current { get { return _current; } } object SC.IEnumerator.Current { get { // If there is no current item a non-generic IEnumerator should throw an exception, // but a generic IEnumerator is not required to. if (_current == null) throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); return _current; } } public void Dispose() { } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
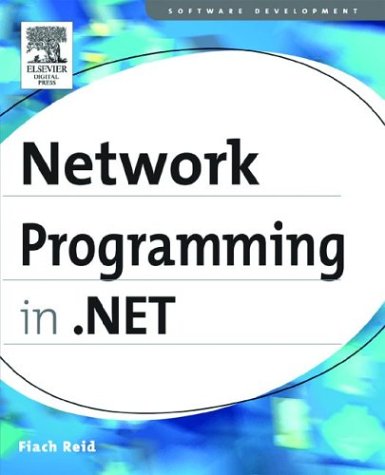
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InteropAutomationProvider.cs
- TabItem.cs
- XamlTemplateSerializer.cs
- ObjectQuery_EntitySqlExtensions.cs
- ListParaClient.cs
- TypeUtil.cs
- BindingsCollection.cs
- CodeConditionStatement.cs
- XmlSchemaObject.cs
- _HelperAsyncResults.cs
- SequentialWorkflowRootDesigner.cs
- TransformedBitmap.cs
- InfoCardX509Validator.cs
- Funcletizer.cs
- TopClause.cs
- XmlParserContext.cs
- StorageEntityTypeMapping.cs
- ImportOptions.cs
- StoreAnnotationsMap.cs
- SudsParser.cs
- AutomationProperty.cs
- ProfileParameter.cs
- IgnoreFlushAndCloseStream.cs
- StringArrayEditor.cs
- ReferencedType.cs
- Triplet.cs
- Rotation3DAnimationUsingKeyFrames.cs
- AndMessageFilter.cs
- XamlHostingSection.cs
- RegexFCD.cs
- ServiceBuildProvider.cs
- ConfigurationException.cs
- CatalogZone.cs
- QuaternionRotation3D.cs
- WinFormsSpinner.cs
- SmiGettersStream.cs
- FontStretch.cs
- OletxDependentTransaction.cs
- SqlGenerator.cs
- ServiceDebugBehavior.cs
- UserControl.cs
- ServiceHostFactory.cs
- RoleGroup.cs
- DataGridViewImageColumn.cs
- TagPrefixInfo.cs
- UdpContractFilterBehavior.cs
- CustomSignedXml.cs
- InkPresenter.cs
- CodeCompileUnit.cs
- WebPermission.cs
- FormViewDeletedEventArgs.cs
- WeakReferenceEnumerator.cs
- CombinedGeometry.cs
- XmlSchemaComplexContent.cs
- TemplateModeChangedEventArgs.cs
- SqlCachedBuffer.cs
- Process.cs
- ErrorInfoXmlDocument.cs
- HijriCalendar.cs
- ProfessionalColorTable.cs
- CodeExpressionCollection.cs
- SQLDoubleStorage.cs
- TraceData.cs
- DataGridViewColumnEventArgs.cs
- Metadata.cs
- ToolStripPanelRow.cs
- RectIndependentAnimationStorage.cs
- AvTraceFormat.cs
- XhtmlTextWriter.cs
- PrintingPermission.cs
- RouteValueExpressionBuilder.cs
- NullableConverter.cs
- Matrix3D.cs
- ResourcePool.cs
- DependencyObjectProvider.cs
- TypedElement.cs
- DiagnosticsConfigurationHandler.cs
- TypeConverterValueSerializer.cs
- SqlOuterApplyReducer.cs
- LineBreak.cs
- FontDriver.cs
- QueryContinueDragEvent.cs
- BuilderPropertyEntry.cs
- DeviceContexts.cs
- AutomationElementCollection.cs
- UserControl.cs
- HierarchicalDataBoundControl.cs
- BoolLiteral.cs
- HatchBrush.cs
- TreeNodeStyleCollection.cs
- EpmTargetPathSegment.cs
- RectangleHotSpot.cs
- NavigationHelper.cs
- CryptoApi.cs
- TypeDescriptor.cs
- ExtensionFile.cs
- X509IssuerSerialKeyIdentifierClause.cs
- NotificationContext.cs
- OleCmdHelper.cs
- SqlTransaction.cs