Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Misc / GDI / WindowsBrush.cs / 1 / WindowsBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Internal.WindowsBrush.FromLogBrush(System.Windows.Forms.Internal.IntNativeMethods+LOGBRUSH):System.Windows.Forms.Internal.WindowsBrush")] [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Internal.WindowsBrush.FromHdc(System.IntPtr):System.Windows.Forms.Internal.WindowsBrush")] [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Internal.WindowsBrush.FromBrush(System.Drawing.Brush):System.Windows.Forms.Internal.WindowsBrush")] #if WINFORMS_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Drawing; ////// #if WINFORMS_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif abstract class WindowsBrush : MarshalByRefObject, ICloneable, IDisposable { // Handle to the native Windows brush object. // private DeviceContext dc; private IntPtr nativeHandle; // Cannot be protected because the class is internal (C# doesn't allow it). private Color color = Color.White; // GDI brushes have just one color as opposed to GDI+ that can have background color. // Note: We may need to implement background color too. #if WINGRAPHICS_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif public abstract object Clone(); // Declaration required by C# even though this is an abstract class. protected abstract void CreateBrush(); ////// Encapsulates a GDI Brush object. /// ////// Parameterless constructor to use default color. /// Notice that the actual object construction is done in the derived classes. /// public WindowsBrush(DeviceContext dc) { this.dc = dc; } public WindowsBrush(DeviceContext dc, Color color) { this.dc = dc; this.color = color; } ~WindowsBrush() { Dispose(false); } protected DeviceContext DC { get { return this.dc; } } public void Dispose() { Dispose(true); } protected virtual void Dispose(bool disposing) { if (dc != null && this.nativeHandle != IntPtr.Zero) { DbgUtil.AssertFinalization(this, disposing); dc.DeleteObject(this.nativeHandle, GdiObjectType.Brush); this.nativeHandle = IntPtr.Zero; } if (disposing) { GC.SuppressFinalize(this); } } public Color Color { get { return this.color; } } ////// Gets the native Win32 brush handle. It creates it on demand. /// protected IntPtr NativeHandle { get { if( this.nativeHandle == IntPtr.Zero ) { CreateBrush(); } return this.nativeHandle; } set { Debug.Assert(this.nativeHandle == IntPtr.Zero, "WindowsBrush object is immutable"); Debug.Assert(value != IntPtr.Zero, "WARNING: assigning IntPtr.Zero to the nativeHandle object."); this.nativeHandle = value; } } #if WINFORMS_PUBLIC_GRAPHICS_LIBRARY ////// Derived classes implement this method to get a native GDI brush wrapper with the same /// properties as this object. /// public static WindowsBrush FromBrush(DeviceContext dc, Brush originalBrush) { if(originalBrush is SolidBrush) { return new WindowsSolidBrush(dc, ((SolidBrush)originalBrush).Color); } if(originalBrush is System.Drawing.Drawing2D.HatchBrush) { System.Drawing.Drawing2D.HatchBrush hatchBrush = ((System.Drawing.Drawing2D.HatchBrush)originalBrush); return new WindowsHatchBrush(dc, (WindowsHatchStyle) hatchBrush.HatchStyle, hatchBrush.ForegroundColor, hatchBrush.BackgroundColor); } /* */ Debug.Fail("Don't know how to convert this brush!"); return null; } ////// Creates a WindowsBrush from the DC currently selected HBRUSH /// public static WindowsBrush FromDC(DeviceContext dc) { IntPtr hBrush = IntUnsafeNativeMethods.GetCurrentObject(new HandleRef(null, dc.Hdc), IntNativeMethods.OBJ_BRUSH); IntNativeMethods.LOGBRUSH logBrush = new IntNativeMethods.LOGBRUSH(); IntUnsafeNativeMethods.GetObject(new HandleRef(null, hBrush), logBrush); // don't call DeleteObject on handle from GetCurrentObject, it is the one selected in the hdc. return WindowsBrush.FromLogBrush(dc, logBrush ); } ////// Creates a WindowsBrush from a LOGBRUSH. /// public static WindowsBrush FromLogBrush( DeviceContext dc, IntNativeMethods.LOGBRUSH logBrush ) { Debug.Assert( logBrush != null, "logBrush is null" ); switch( logBrush.lbStyle ) { // currently supported brushes: case IntNativeMethods.BS_HATCHED: return new WindowsHatchBrush(dc, (WindowsHatchStyle) logBrush.lbHatch ); case IntNativeMethods.BS_SOLID: return new WindowsSolidBrush( dc, Color.FromArgb(logBrush.lbColor) ); default: Debug.Fail( "Don't know how to create WindowsBrush from specified logBrush" ); return null; } } #endif ////// public IntPtr HBrush { get { return this.NativeHandle; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Returns the native Win32 brush handle. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Internal.WindowsBrush.FromLogBrush(System.Windows.Forms.Internal.IntNativeMethods+LOGBRUSH):System.Windows.Forms.Internal.WindowsBrush")] [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Internal.WindowsBrush.FromHdc(System.IntPtr):System.Windows.Forms.Internal.WindowsBrush")] [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Internal.WindowsBrush.FromBrush(System.Drawing.Brush):System.Windows.Forms.Internal.WindowsBrush")] #if WINFORMS_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Drawing; ////// #if WINFORMS_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif abstract class WindowsBrush : MarshalByRefObject, ICloneable, IDisposable { // Handle to the native Windows brush object. // private DeviceContext dc; private IntPtr nativeHandle; // Cannot be protected because the class is internal (C# doesn't allow it). private Color color = Color.White; // GDI brushes have just one color as opposed to GDI+ that can have background color. // Note: We may need to implement background color too. #if WINGRAPHICS_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif public abstract object Clone(); // Declaration required by C# even though this is an abstract class. protected abstract void CreateBrush(); ////// Encapsulates a GDI Brush object. /// ////// Parameterless constructor to use default color. /// Notice that the actual object construction is done in the derived classes. /// public WindowsBrush(DeviceContext dc) { this.dc = dc; } public WindowsBrush(DeviceContext dc, Color color) { this.dc = dc; this.color = color; } ~WindowsBrush() { Dispose(false); } protected DeviceContext DC { get { return this.dc; } } public void Dispose() { Dispose(true); } protected virtual void Dispose(bool disposing) { if (dc != null && this.nativeHandle != IntPtr.Zero) { DbgUtil.AssertFinalization(this, disposing); dc.DeleteObject(this.nativeHandle, GdiObjectType.Brush); this.nativeHandle = IntPtr.Zero; } if (disposing) { GC.SuppressFinalize(this); } } public Color Color { get { return this.color; } } ////// Gets the native Win32 brush handle. It creates it on demand. /// protected IntPtr NativeHandle { get { if( this.nativeHandle == IntPtr.Zero ) { CreateBrush(); } return this.nativeHandle; } set { Debug.Assert(this.nativeHandle == IntPtr.Zero, "WindowsBrush object is immutable"); Debug.Assert(value != IntPtr.Zero, "WARNING: assigning IntPtr.Zero to the nativeHandle object."); this.nativeHandle = value; } } #if WINFORMS_PUBLIC_GRAPHICS_LIBRARY ////// Derived classes implement this method to get a native GDI brush wrapper with the same /// properties as this object. /// public static WindowsBrush FromBrush(DeviceContext dc, Brush originalBrush) { if(originalBrush is SolidBrush) { return new WindowsSolidBrush(dc, ((SolidBrush)originalBrush).Color); } if(originalBrush is System.Drawing.Drawing2D.HatchBrush) { System.Drawing.Drawing2D.HatchBrush hatchBrush = ((System.Drawing.Drawing2D.HatchBrush)originalBrush); return new WindowsHatchBrush(dc, (WindowsHatchStyle) hatchBrush.HatchStyle, hatchBrush.ForegroundColor, hatchBrush.BackgroundColor); } /* */ Debug.Fail("Don't know how to convert this brush!"); return null; } ////// Creates a WindowsBrush from the DC currently selected HBRUSH /// public static WindowsBrush FromDC(DeviceContext dc) { IntPtr hBrush = IntUnsafeNativeMethods.GetCurrentObject(new HandleRef(null, dc.Hdc), IntNativeMethods.OBJ_BRUSH); IntNativeMethods.LOGBRUSH logBrush = new IntNativeMethods.LOGBRUSH(); IntUnsafeNativeMethods.GetObject(new HandleRef(null, hBrush), logBrush); // don't call DeleteObject on handle from GetCurrentObject, it is the one selected in the hdc. return WindowsBrush.FromLogBrush(dc, logBrush ); } ////// Creates a WindowsBrush from a LOGBRUSH. /// public static WindowsBrush FromLogBrush( DeviceContext dc, IntNativeMethods.LOGBRUSH logBrush ) { Debug.Assert( logBrush != null, "logBrush is null" ); switch( logBrush.lbStyle ) { // currently supported brushes: case IntNativeMethods.BS_HATCHED: return new WindowsHatchBrush(dc, (WindowsHatchStyle) logBrush.lbHatch ); case IntNativeMethods.BS_SOLID: return new WindowsSolidBrush( dc, Color.FromArgb(logBrush.lbColor) ); default: Debug.Fail( "Don't know how to create WindowsBrush from specified logBrush" ); return null; } } #endif ////// public IntPtr HBrush { get { return this.NativeHandle; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns the native Win32 brush handle. /// ///
Link Menu
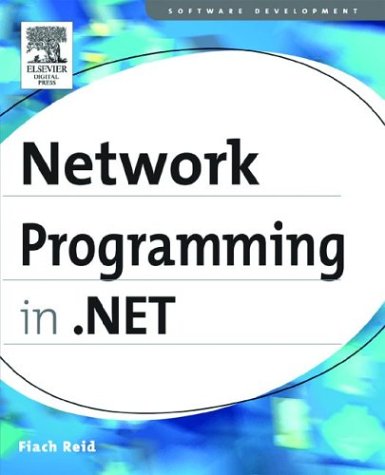
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewCellStyleConverter.cs
- DataGridViewElement.cs
- ObjectDataSourceDesigner.cs
- LineProperties.cs
- WebPartEditVerb.cs
- NativeMethodsCLR.cs
- ParagraphVisual.cs
- DecimalAnimation.cs
- Context.cs
- ValidatorCompatibilityHelper.cs
- Debug.cs
- ResourceReferenceExpression.cs
- PointHitTestResult.cs
- EntityConnection.cs
- DataServiceRequestOfT.cs
- StylusPointPropertyInfoDefaults.cs
- XPathDocumentNavigator.cs
- ArrayConverter.cs
- PrimitiveSchema.cs
- SchemaCollectionPreprocessor.cs
- XmlDictionaryWriter.cs
- KeyboardEventArgs.cs
- ExpressionBinding.cs
- TransactionOptions.cs
- DrawingContextDrawingContextWalker.cs
- VisualStyleRenderer.cs
- _FtpControlStream.cs
- PrintingPermissionAttribute.cs
- DockAndAnchorLayout.cs
- MachineSettingsSection.cs
- webeventbuffer.cs
- UnmanagedMemoryStreamWrapper.cs
- TextDocumentView.cs
- TextAutomationPeer.cs
- COMException.cs
- XsltConvert.cs
- FontUnit.cs
- Trace.cs
- LoadRetryHandler.cs
- IncrementalReadDecoders.cs
- DoubleAnimationUsingPath.cs
- TypeSystemProvider.cs
- DataBinder.cs
- DataGridViewCell.cs
- ConsoleEntryPoint.cs
- FieldMetadata.cs
- CompiledXpathExpr.cs
- DataGridViewCellValidatingEventArgs.cs
- InternalConfigRoot.cs
- ReadOnlyAttribute.cs
- FixedSOMTextRun.cs
- EntityTypeBase.cs
- BitmapFrame.cs
- DeclarativeConditionsCollection.cs
- SequentialUshortCollection.cs
- OdbcConnectionString.cs
- CodeIdentifier.cs
- ObjectRef.cs
- WindowsStartMenu.cs
- Attributes.cs
- DiffuseMaterial.cs
- BitmapEffectGroup.cs
- RepeatButton.cs
- CssStyleCollection.cs
- PlainXmlWriter.cs
- RC2CryptoServiceProvider.cs
- Emitter.cs
- DataBindingCollection.cs
- SerializationObjectManager.cs
- ProfileEventArgs.cs
- PKCS1MaskGenerationMethod.cs
- FrameworkContextData.cs
- DataTemplateKey.cs
- KeyGestureValueSerializer.cs
- CompilerResults.cs
- AsymmetricKeyExchangeDeformatter.cs
- XmlAttributeProperties.cs
- SqlNotificationEventArgs.cs
- ConfigurationValues.cs
- MenuCommand.cs
- StrokeRenderer.cs
- SettingsPropertyNotFoundException.cs
- SoapExtensionTypeElementCollection.cs
- MatrixTransform.cs
- TreeNodeSelectionProcessor.cs
- RemoteWebConfigurationHost.cs
- SecurityAppliedMessage.cs
- AdPostCacheSubstitution.cs
- SessionStateContainer.cs
- ClientSession.cs
- ConditionalAttribute.cs
- VectorAnimation.cs
- _LoggingObject.cs
- SpotLight.cs
- DocumentXPathNavigator.cs
- Application.cs
- UserInitiatedNavigationPermission.cs
- ContainsRowNumberChecker.cs
- GroupBoxDesigner.cs
- FamilyMap.cs