Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Internal / SequentialUshortCollection.cs / 1 / SequentialUshortCollection.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: A class that implements ICollectionfor a sequence of numbers [0..n-1]. // // // History: // 03/21/2005 : MLeonov - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal { internal class SequentialUshortCollection : ICollection { public SequentialUshortCollection(ushort count) { _count = count; } #region ICollection Members public void Add(ushort item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(ushort item) { return item < _count; } public void CopyTo(ushort[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } for (ushort i = 0; i < _count; ++i) array[arrayIndex + i] = i; } public int Count { get { return _count; } } public bool IsReadOnly { get { return true; } } public bool Remove(ushort item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { for (ushort i = 0; i < _count; ++i) yield return i; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion private ushort _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: A class that implements ICollection for a sequence of numbers [0..n-1]. // // // History: // 03/21/2005 : MLeonov - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal { internal class SequentialUshortCollection : ICollection { public SequentialUshortCollection(ushort count) { _count = count; } #region ICollection Members public void Add(ushort item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(ushort item) { return item < _count; } public void CopyTo(ushort[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } for (ushort i = 0; i < _count; ++i) array[arrayIndex + i] = i; } public int Count { get { return _count; } } public bool IsReadOnly { get { return true; } } public bool Remove(ushort item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { for (ushort i = 0; i < _count; ++i) yield return i; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion private ushort _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
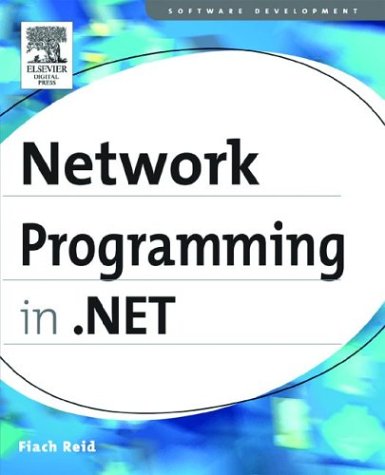
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Emitter.cs
- ListViewItem.cs
- Logging.cs
- BooleanConverter.cs
- OracleCommandSet.cs
- HtmlContainerControl.cs
- XmlDataImplementation.cs
- RegexFCD.cs
- ErrorWrapper.cs
- RequestSecurityTokenForGetBrowserToken.cs
- SQLByteStorage.cs
- Policy.cs
- OdbcConnectionFactory.cs
- AddInControllerImpl.cs
- DefaultPropertyAttribute.cs
- RuntimeUtils.cs
- DataSourceProvider.cs
- HttpChannelHelper.cs
- FixedPageProcessor.cs
- Effect.cs
- Substitution.cs
- BookmarkEventArgs.cs
- ParseChildrenAsPropertiesAttribute.cs
- ListItemCollection.cs
- BitConverter.cs
- RegionData.cs
- BamlVersionHeader.cs
- TypeExtensionConverter.cs
- StateMachineHelpers.cs
- StateMachine.cs
- UITypeEditor.cs
- ScriptingSectionGroup.cs
- XDeferredAxisSource.cs
- CalloutQueueItem.cs
- SemanticBasicElement.cs
- StreamInfo.cs
- OleDbMetaDataFactory.cs
- BookmarkScopeManager.cs
- CommonRemoteMemoryBlock.cs
- ReferencedAssembly.cs
- ErrorWebPart.cs
- EntityContainerAssociationSet.cs
- FilterQuery.cs
- ToolStripItemEventArgs.cs
- XmlRawWriterWrapper.cs
- Subset.cs
- EventBookmark.cs
- RecognizedPhrase.cs
- ArrayHelper.cs
- NamespaceCollection.cs
- SafeFileMappingHandle.cs
- DataControlPagerLinkButton.cs
- CoTaskMemUnicodeSafeHandle.cs
- CategoryNameCollection.cs
- XmlCharacterData.cs
- CachedTypeface.cs
- SerializationObjectManager.cs
- ScriptingRoleServiceSection.cs
- KnownBoxes.cs
- RequestReplyCorrelator.cs
- PersonalizationDictionary.cs
- _DigestClient.cs
- AttachedAnnotation.cs
- ObjectDataSourceStatusEventArgs.cs
- PropertyDescriptorGridEntry.cs
- OrderedDictionaryStateHelper.cs
- ConsumerConnectionPointCollection.cs
- MDIWindowDialog.cs
- columnmapkeybuilder.cs
- CryptoConfig.cs
- OleDbRowUpdatingEvent.cs
- MultilineStringConverter.cs
- ConditionedDesigner.cs
- MulticastOption.cs
- XmlSerializerImportOptions.cs
- HandleCollector.cs
- EventPropertyMap.cs
- CapabilitiesAssignment.cs
- CodeDirectoryCompiler.cs
- ToolStripControlHost.cs
- HtmlHead.cs
- DataBoundLiteralControl.cs
- FloaterParagraph.cs
- FixedPosition.cs
- ConfigurationValue.cs
- ConfigDefinitionUpdates.cs
- Literal.cs
- ListViewItemMouseHoverEvent.cs
- HttpConfigurationSystem.cs
- EncryptedData.cs
- DynamicDataResources.Designer.cs
- TokenBasedSet.cs
- DuplicateWaitObjectException.cs
- DocumentViewerBaseAutomationPeer.cs
- figurelengthconverter.cs
- PagerSettings.cs
- Deserializer.cs
- Debugger.cs
- DataGridPageChangedEventArgs.cs
- Scripts.cs