Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / InstallHelper.cs / 2 / InstallHelper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using Microsoft.Win32; using System; using System.Diagnostics; using System.DirectoryServices; using System.Globalization; using System.IO; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Security.Principal; using System.Threading; using System.ComponentModel; internal static class InstallHelper { const string AspNetIsapiDllName = @"aspnet_isapi.dll"; static string frameworkInstallPath = null; static string highestOtherWcfRuntimeInstallPath = null; static string nativeIsapiFilterFileName = null; static string wow64IsapiFilterFileName = null; static string nativeWcfRuntimeInstallPath = null; static string wcfRuntimeInstallPath = null; const string webEngineDllName = @"webengine.dll"; static string wow64MachineConfigFileName = null; static string wow64WebConfigFileName = null; static string wow64FrameworkInstallPath = null; static Nullableenable32BitAppOnWin64; static DataReceivedEventHandler errorDataReceivedCallback = new DataReceivedEventHandler(OnErrorDataReceived); static DataReceivedEventHandler outputDataReceivedCallback = new DataReceivedEventHandler(OnOutputDataReceived); static internal string GetFrameworkInstallPath() { if (String.IsNullOrEmpty(InstallHelper.frameworkInstallPath)) { InstallHelper.frameworkInstallPath = InstallHelper.InstallDirectory(typeof(object)); } return InstallHelper.frameworkInstallPath; } static string GetWow64FrameworkInstallPath() { if (!Is64BitMachine()) { return String.Empty; } if (String.IsNullOrEmpty(InstallHelper.wow64FrameworkInstallPath)) { // Lookup in 32 bit hive: Assembly assembly = typeof(InstallHelper).Assembly; Version maxVersion = new Version(assembly.ImageRuntimeVersion.TrimStart('v')); Version version = maxVersion; Version minPolicy = null; Version maxPolicy = null; string maxVersionName = null; RegistryKey key = Registry.LocalMachine.OpenSubKey(ServiceModelInstallStrings.Wow64FrameworkPolicyKey); if (key != null) { foreach (string name in key.GetValueNames()) { string data = key.GetValue(name) as string; if (!String.IsNullOrEmpty(data)) { string[] vals = data.Split('-'); if (vals.Length == 2) { minPolicy = new Version(vals[0]); maxPolicy = new Version(vals[1]); // Value is in range if ((minPolicy <= version) && (maxPolicy >= maxVersion)) { maxVersion = maxPolicy; maxVersionName = name; } } } } if (!String.IsNullOrEmpty(maxVersionName)) { key = Registry.LocalMachine.OpenSubKey(ServiceModelInstallStrings.Wow64FrameworkKey); string installRoot = key.GetValue("InstallRoot") as string; if (!String.IsNullOrEmpty(installRoot)) { InstallHelper.wow64FrameworkInstallPath = String.Format(CultureInfo.InvariantCulture, @"{0}v{1}", installRoot, maxVersionName); } } } } return InstallHelper.wow64FrameworkInstallPath; } internal static string GetNativeWcfRuntimeInstallPath() { if (InstallHelper.nativeWcfRuntimeInstallPath == null) { using (RegistryHandle regKey = RegistryHandle.OpenNativeHKLMSubkey( ServiceModelInstallStrings.WcfRegistryKey)) { InstallHelper.nativeWcfRuntimeInstallPath = regKey.QueryStringValue( ServiceModelInstallStrings.RuntimeInstallPathName); } } return InstallHelper.nativeWcfRuntimeInstallPath; } internal static string GetWcfRuntimeInstallPath() { if (String.IsNullOrEmpty(InstallHelper.wcfRuntimeInstallPath)) { using (RegistryKey wcfRegKey = Registry.LocalMachine.OpenSubKey(ServiceModelInstallStrings.WcfRegistryKey)) { if (null != wcfRegKey) { InstallHelper.wcfRuntimeInstallPath = (string)wcfRegKey.GetValue(ServiceModelInstallStrings.RuntimeInstallPathName, String.Empty); if (String.IsNullOrEmpty(InstallHelper.wcfRuntimeInstallPath)) { throw new InvalidOperationException(SR.GetString(SR.RegistryKeyDoesNotContainValue, ServiceModelInstallStrings.WcfRegistryKey, ServiceModelInstallStrings.RuntimeInstallPathName)); } } else { throw new InvalidOperationException(SR.GetString(SR.RegistryKeyDoesNotExist, ServiceModelInstallStrings.WcfRegistryKey)); } } } return InstallHelper.wcfRuntimeInstallPath; } internal static bool ShouldInstallToWebConfig { get { return File.Exists(Path.Combine(GetFrameworkInstallPath(), webEngineDllName)); } } internal static string Wow64MachineConfigFileName { get { if (String.IsNullOrEmpty(InstallHelper.wow64MachineConfigFileName)) { string wow64InstallPath = InstallHelper.GetWow64FrameworkInstallPath(); if (!String.IsNullOrEmpty(wow64InstallPath)) { InstallHelper.wow64MachineConfigFileName = Path.Combine(wow64InstallPath, @"config\machine.config"); } } return InstallHelper.wow64MachineConfigFileName; } } internal static string Wow64WebConfigFileName { get { if (String.IsNullOrEmpty(InstallHelper.wow64WebConfigFileName)) { string wow64InstallPath = InstallHelper.GetWow64FrameworkInstallPath(); if (!String.IsNullOrEmpty(wow64InstallPath)) { InstallHelper.wow64WebConfigFileName = Path.Combine(wow64InstallPath, @"config\web.config"); } } return InstallHelper.wow64WebConfigFileName; } } internal static string GetHighestOtherWcfRuntimeInstallPath() { if (String.IsNullOrEmpty(InstallHelper.highestOtherWcfRuntimeInstallPath)) { using (RegistryKey winFxRegKey = Registry.LocalMachine.OpenSubKey(ServiceModelInstallStrings.WinFXRegistryKey)) { if (null != winFxRegKey) { double currentVersion = -1.0; double currentHighVersion = -1.0; foreach (string subKeyName in winFxRegKey.GetSubKeyNames()) { if (string.IsNullOrEmpty(subKeyName)) { continue; } string versionString = subKeyName.Substring(1); if (ServiceModelInstallStrings.WcfRegistryKey.StartsWith(ServiceModelInstallStrings.WinFXRegistryKey + @"\" + subKeyName, StringComparison.OrdinalIgnoreCase)) { currentVersion = double.Parse(versionString, NumberStyles.AllowDecimalPoint, NumberFormatInfo.InvariantInfo); } else { double version = 0.0; if (!double.TryParse(versionString, NumberStyles.AllowDecimalPoint, NumberFormatInfo.InvariantInfo, out version)) { continue; } if (version <= currentHighVersion) { continue; } using (RegistryKey alternateWinFxRegKey = winFxRegKey.OpenSubKey(subKeyName)) { if (alternateWinFxRegKey != null) { using (RegistryKey alternateWcfRegKey = alternateWinFxRegKey.OpenSubKey(ServiceModelInstallStrings.WcfRegistrySubKey)) { if (alternateWcfRegKey != null) { string alternateWcfRuntimeInstallPath = alternateWcfRegKey.GetValue(ServiceModelInstallStrings.RuntimeInstallPathName) as string; if (alternateWcfRuntimeInstallPath != null) { currentHighVersion = version; InstallHelper.highestOtherWcfRuntimeInstallPath = alternateWcfRuntimeInstallPath; } } } } } } } } else { throw new InvalidOperationException(SR.GetString(SR.RegistryKeyDoesNotExist, ServiceModelInstallStrings.WinFXRegistryKey)); } } } return InstallHelper.highestOtherWcfRuntimeInstallPath; } internal static bool Is64BitMachine() { bool retval = false; if (8 == IntPtr.Size) { retval = true; } else { if (!NativeMethods.IsWow64Process(Process.GetCurrentProcess().Handle, out retval)) { throw new Win32Exception(); } } return retval; } internal static string GetNativeIsapiFilter(bool useEnvironmentVariable) { if (String.IsNullOrEmpty(InstallHelper.nativeIsapiFilterFileName)) { string frameworkInstallPath = InstallHelper.GetFrameworkInstallPath(); if (!String.IsNullOrEmpty(frameworkInstallPath)) { InstallHelper.nativeIsapiFilterFileName = Path.Combine(frameworkInstallPath, AspNetIsapiDllName); } } if (!useEnvironmentVariable) { return InstallHelper.nativeIsapiFilterFileName; } return NormalizePathForSystemRoot(InstallHelper.nativeIsapiFilterFileName); } static string NormalizePathForSystemRoot(string path) { string fullPath = Path.GetFullPath(path); string rootPath = Environment.GetEnvironmentVariable("SystemRoot"); if (fullPath.StartsWith(rootPath, StringComparison.OrdinalIgnoreCase)) { path = string.Format(CultureInfo.InvariantCulture, "%SystemRoot%{0}", fullPath.Substring(rootPath.Length)); } return path; } internal static string GetWow64IsapiFilter(bool useEnvironmentVariable) { if (!Is64BitMachine()) { return null; } if (String.IsNullOrEmpty(InstallHelper.wow64IsapiFilterFileName)) { string wow64InstallPath = InstallHelper.GetWow64FrameworkInstallPath(); if (!String.IsNullOrEmpty(wow64InstallPath)) { InstallHelper.wow64IsapiFilterFileName = Path.Combine(wow64InstallPath, AspNetIsapiDllName); } } if (!useEnvironmentVariable) { return InstallHelper.wow64IsapiFilterFileName; } return NormalizePathForSystemRoot(InstallHelper.wow64IsapiFilterFileName); } internal static string IsapiFilter { get { if (!enable32BitAppOnWin64.HasValue) { enable32BitAppOnWin64 = false; if (Is64BitMachine()) { using (DirectoryEntry entry = new DirectoryEntry(ServiceModelInstallStrings.IISAdsRoot + ServiceModelInstallStrings.AppPoolsAdsPath)) { IisHelper.ValidateDirectoryEntry(entry); PropertyValueCollection enable32BitAppOnWin64Collection = entry.Properties["Enable32BitAppOnWin64"]; if ((bool)enable32BitAppOnWin64Collection[0]) { enable32BitAppOnWin64 = true; } } } } if (!enable32BitAppOnWin64.Value) { return InstallHelper.GetNativeIsapiFilter(false); } return InstallHelper.GetWow64IsapiFilter(false); } } internal static string GetVersionStringFromTypeString(string typeString) { Type type = Type.GetType(typeString); return type.Assembly.GetName().Version.ToString(); } internal static string InstallDirectory(Type type) { string filename = Assembly.GetAssembly(type).Location.Replace('/', '\\'); return Path.GetDirectoryName(filename); } internal static bool IsExitCodeAllowed(int[] allowedExitCodes, int exitCode) { if (exitCode == 0) { return true; } foreach (int element in allowedExitCodes) { if (element == exitCode) { return true; } } return false; } static int ExecuteWaitHelper(string program, string parameters) { if (!File.Exists(program)) { // Perform this check so that we can throw something more informative than Win32Exception. throw new FileNotFoundException(null, program); } using (Process execProcess = new Process()) { execProcess.StartInfo.FileName = program; execProcess.StartInfo.Arguments = parameters; execProcess.StartInfo.CreateNoWindow = true; execProcess.StartInfo.UseShellExecute = false; execProcess.StartInfo.RedirectStandardError = true; execProcess.ErrorDataReceived += errorDataReceivedCallback; execProcess.StartInfo.RedirectStandardOutput = true; execProcess.OutputDataReceived += outputDataReceivedCallback; EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.ToolStarting, program, parameters)); execProcess.Start(); execProcess.BeginErrorReadLine(); execProcess.BeginOutputReadLine(); execProcess.WaitForExit(); execProcess.Refresh(); int exitCode = execProcess.ExitCode; EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.ToolExited, program, parameters, exitCode)); return exitCode; } } static void OnErrorDataReceived(object sender, DataReceivedEventArgs args) { EventLogger.WriteMsiStyleLogEntry(args.Data); } static void OnOutputDataReceived(object sender, DataReceivedEventArgs args) { EventLogger.WriteMsiStyleLogEntry(args.Data); } internal static bool TryExecuteWait(OutputLevel outputLevel, string program, string parameters, params int[] allowedExitCodes) { int exitCode; try { exitCode = ExecuteWaitHelper(program, parameters); } catch (Win32Exception exception) { EventLogger.LogWarning(SR.GetString(SR.ProgramExecutionFailed, program, parameters, exception), (OutputLevel.Normal == outputLevel)); return false; } return IsExitCodeAllowed(allowedExitCodes, exitCode); } internal static void ExecuteWait(string program, string parameters, params int[] allowedExitCodes) { int exitCode = ExecuteWaitHelper(program, parameters); if (!IsExitCodeAllowed(allowedExitCodes, exitCode)) { throw new ApplicationException(SR.GetString(SR.InstallIssueExecuteUnexpected, exitCode, program, parameters)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
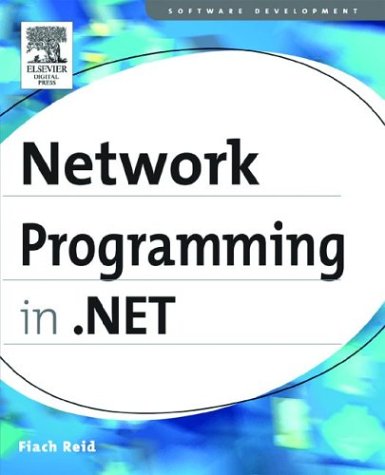
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DSASignatureFormatter.cs
- AnnotationAdorner.cs
- TextTreeDeleteContentUndoUnit.cs
- ComponentSerializationService.cs
- XPathPatternBuilder.cs
- TextDecorations.cs
- PageAction.cs
- Stroke2.cs
- XmlChoiceIdentifierAttribute.cs
- COM2Properties.cs
- PropertyPushdownHelper.cs
- BitmapEffectState.cs
- AnchoredBlock.cs
- Exceptions.cs
- ToolStripItemCollection.cs
- UIntPtr.cs
- BufferedStream.cs
- Style.cs
- DiffuseMaterial.cs
- MenuItemCollection.cs
- PackageFilter.cs
- DataBoundControlAdapter.cs
- StructuredTypeEmitter.cs
- InputLanguageCollection.cs
- EmbeddedMailObjectsCollection.cs
- RegisteredDisposeScript.cs
- ConstructorArgumentAttribute.cs
- BindValidator.cs
- NativeMethods.cs
- BamlResourceDeserializer.cs
- FormattedText.cs
- Version.cs
- WebDescriptionAttribute.cs
- ConfigurationStrings.cs
- HandleCollector.cs
- ActivationServices.cs
- EdmValidator.cs
- SigningCredentials.cs
- SecurityDocument.cs
- TableLayoutPanelDesigner.cs
- ColorPalette.cs
- XslVisitor.cs
- ConfigurationElement.cs
- FormClosedEvent.cs
- SetStoryboardSpeedRatio.cs
- __ConsoleStream.cs
- TimeoutConverter.cs
- ObjectToIdCache.cs
- ListenerHandler.cs
- WebBrowsableAttribute.cs
- Attributes.cs
- TemplateBindingExtensionConverter.cs
- ExternalCalls.cs
- MemoryRecordBuffer.cs
- HttpException.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- XmlSchemaIdentityConstraint.cs
- PartManifestEntry.cs
- PkcsUtils.cs
- BlockUIContainer.cs
- SqlInfoMessageEvent.cs
- DomainConstraint.cs
- NavigationWindowAutomationPeer.cs
- BaseTemplateCodeDomTreeGenerator.cs
- ScriptControl.cs
- ServiceAuthorizationManager.cs
- XmlSchemaProviderAttribute.cs
- ObjectAnimationUsingKeyFrames.cs
- OrderPreservingMergeHelper.cs
- SelectionUIHandler.cs
- ContextMenuAutomationPeer.cs
- WebExceptionStatus.cs
- XmlCodeExporter.cs
- documentsequencetextpointer.cs
- CodeTypeReference.cs
- XmlSchemaElement.cs
- ComEventsMethod.cs
- FastEncoder.cs
- DataColumnChangeEvent.cs
- Predicate.cs
- InputManager.cs
- AtomServiceDocumentSerializer.cs
- RefreshInfo.cs
- NetworkInformationPermission.cs
- ModelTreeManager.cs
- MarkupObject.cs
- TextBox.cs
- TextInfo.cs
- TextElementEnumerator.cs
- ReflectionTypeLoadException.cs
- NativeMethodsCLR.cs
- CodeTypeParameterCollection.cs
- Splitter.cs
- TaiwanLunisolarCalendar.cs
- CheckBoxField.cs
- FrameworkTextComposition.cs
- DataTrigger.cs
- StringDictionary.cs
- MultiView.cs
- WpfWebRequestHelper.cs