Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / ScalarType.cs / 3 / ScalarType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// This is an adapter to make PrimitiveTypeKindData fit in the Schema Object Model tree /// internal sealed class ScalarType : SchemaType { internal const string DateTimeFormat = @"yyyy-MM-dd HH\:mm\:ss.fffZ"; internal const string TimeFormat = @"HH\:mm\:ss.fffffffZ"; internal const string DateTimeOffsetFormat = @"yyyy-MM-dd HH\:mm\:ss.fffffffz"; private readonly static System.Text.RegularExpressions.Regex _BinaryValueValidator = new System.Text.RegularExpressions.Regex("^0[xX][0-9a-fA-F]+$", System.Text.RegularExpressions.RegexOptions.Compiled); private readonly static System.Text.RegularExpressions.Regex _GuidValueValidator = new System.Text.RegularExpressions.Regex("[0-9a-fA-F]{8,8}(-[0-9a-fA-F]{4,4}){3,3}-[0-9a-fA-F]{12,12}", System.Text.RegularExpressions.RegexOptions.Compiled); private PrimitiveType _primitiveType = null; ////// Construct an internal (not from schema) CDM scalar type /// /// the owning schema /// the naem of the type /// the PrimitiveTypeKind of the type internal ScalarType(Schema parentElement, string typeName, PrimitiveType primitiveType) : base(parentElement) { Name = typeName; _primitiveType = primitiveType; } ////// try to parse a string /// /// the string to parse /// the value of the string ///true if the value is a valid value, false otherwise public bool TryParse(string text, out object value) { switch(_primitiveType.PrimitiveTypeKind) { case PrimitiveTypeKind.Binary: return TryParseBinary(text, out value); case PrimitiveTypeKind.Boolean: return TryParseBoolean(text, out value); case PrimitiveTypeKind.Byte: return TryParseByte(text, out value); case PrimitiveTypeKind.DateTime: return TryParseDateTime(text, out value); case PrimitiveTypeKind.Time: return TryParseTime(text, out value); case PrimitiveTypeKind.DateTimeOffset: return TryParseDateTimeOffset(text, out value); case PrimitiveTypeKind.Decimal: return TryParseDecimal(text, out value); case PrimitiveTypeKind.Double: return TryParseDouble(text, out value); case PrimitiveTypeKind.Guid: return TryParseGuid(text, out value); case PrimitiveTypeKind.Int16: return TryParseInt16(text, out value); case PrimitiveTypeKind.Int32: return TryParseInt32(text, out value); case PrimitiveTypeKind.Int64: return TryParseInt64(text, out value); case PrimitiveTypeKind.Single: return TryParseSingle(text, out value); case PrimitiveTypeKind.String: return TryParseString(text, out value); case PrimitiveTypeKind.SByte: return TryParseSByte(text, out value); default: throw EntityUtil.NotSupported(_primitiveType.FullName); } } ////// The type kind of this type. /// public PrimitiveTypeKind TypeKind { get { return _primitiveType.PrimitiveTypeKind; } } ////// Returns the PrimitiveType of the scalar type. /// public PrimitiveType Type { get { return _primitiveType; } } private static bool TryParseBoolean(string text, out object value) { Boolean temp; if (!Boolean.TryParse(text, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseByte(string text, out object value) { Byte temp; if (!Byte.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseSByte(string text, out object value) { SByte temp; if (!SByte.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseInt16(string text, out object value) { Int16 temp; if (!Int16.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseInt32(string text, out object value) { Int32 temp; if (!Int32.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseInt64(string text, out object value) { Int64 temp; if (!Int64.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseDouble(string text, out object value) { Double temp; if (!Double.TryParse(text, System.Globalization.NumberStyles.Float, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseDecimal(string text, out object value) { Decimal temp; if (!Decimal.TryParse(text, System.Globalization.NumberStyles.Float, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseDateTime(string text, out object value) { DateTime temp; if (!DateTime.TryParseExact(text, DateTimeFormat, CultureInfo.InvariantCulture, DateTimeStyles.AssumeUniversal | DateTimeStyles.AdjustToUniversal, out temp)) { value = null; return false; } value = temp; return true; } ////// Parses the default value for Edm Type Time based on the DateTime format "HH:mm:ss.fffffffz". /// The value is first converted to DateTime value and then converted to TimeSpan. /// /// /// ///private static bool TryParseTime(string text, out object value) { DateTime temp; if (!DateTime.TryParseExact(text, TimeFormat, CultureInfo.InvariantCulture, DateTimeStyles.AssumeUniversal | DateTimeStyles.AdjustToUniversal, out temp)) { value = null; return false; } value = new TimeSpan(temp.Ticks); return true; } private static bool TryParseDateTimeOffset(string text, out object value) { DateTimeOffset temp; if (!DateTimeOffset.TryParse(text, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseGuid(string text, out object value) { if (!_GuidValueValidator.IsMatch(text)) { value = null; return false; } value = new Guid(text); return true; } private static bool TryParseString(string text, out object value) { value = text; return true; } private static bool TryParseBinary(string text, out object value) { //value must look like 0xddddd... if (!_BinaryValueValidator.IsMatch(text)) { value = null; return false; } int inc = 2; if (text.Length % 2 == 1) inc = 1; int numBytes = (text.Length - 1) / 2; byte[] bytes = new byte[numBytes]; for (int index = 2, iByte = 0; index < text.Length; index += inc, inc = 2, ++iByte) { bytes[iByte] = byte.Parse(text.Substring(index, inc), System.Globalization.NumberStyles.HexNumber, System.Globalization.CultureInfo.InvariantCulture); } value = bytes; return true; } private static bool TryParseSingle(string text, out object value) { Single temp; if (!Single.TryParse(text, System.Globalization.NumberStyles.Float, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// This is an adapter to make PrimitiveTypeKindData fit in the Schema Object Model tree /// internal sealed class ScalarType : SchemaType { internal const string DateTimeFormat = @"yyyy-MM-dd HH\:mm\:ss.fffZ"; internal const string TimeFormat = @"HH\:mm\:ss.fffffffZ"; internal const string DateTimeOffsetFormat = @"yyyy-MM-dd HH\:mm\:ss.fffffffz"; private readonly static System.Text.RegularExpressions.Regex _BinaryValueValidator = new System.Text.RegularExpressions.Regex("^0[xX][0-9a-fA-F]+$", System.Text.RegularExpressions.RegexOptions.Compiled); private readonly static System.Text.RegularExpressions.Regex _GuidValueValidator = new System.Text.RegularExpressions.Regex("[0-9a-fA-F]{8,8}(-[0-9a-fA-F]{4,4}){3,3}-[0-9a-fA-F]{12,12}", System.Text.RegularExpressions.RegexOptions.Compiled); private PrimitiveType _primitiveType = null; ////// Construct an internal (not from schema) CDM scalar type /// /// the owning schema /// the naem of the type /// the PrimitiveTypeKind of the type internal ScalarType(Schema parentElement, string typeName, PrimitiveType primitiveType) : base(parentElement) { Name = typeName; _primitiveType = primitiveType; } ////// try to parse a string /// /// the string to parse /// the value of the string ///true if the value is a valid value, false otherwise public bool TryParse(string text, out object value) { switch(_primitiveType.PrimitiveTypeKind) { case PrimitiveTypeKind.Binary: return TryParseBinary(text, out value); case PrimitiveTypeKind.Boolean: return TryParseBoolean(text, out value); case PrimitiveTypeKind.Byte: return TryParseByte(text, out value); case PrimitiveTypeKind.DateTime: return TryParseDateTime(text, out value); case PrimitiveTypeKind.Time: return TryParseTime(text, out value); case PrimitiveTypeKind.DateTimeOffset: return TryParseDateTimeOffset(text, out value); case PrimitiveTypeKind.Decimal: return TryParseDecimal(text, out value); case PrimitiveTypeKind.Double: return TryParseDouble(text, out value); case PrimitiveTypeKind.Guid: return TryParseGuid(text, out value); case PrimitiveTypeKind.Int16: return TryParseInt16(text, out value); case PrimitiveTypeKind.Int32: return TryParseInt32(text, out value); case PrimitiveTypeKind.Int64: return TryParseInt64(text, out value); case PrimitiveTypeKind.Single: return TryParseSingle(text, out value); case PrimitiveTypeKind.String: return TryParseString(text, out value); case PrimitiveTypeKind.SByte: return TryParseSByte(text, out value); default: throw EntityUtil.NotSupported(_primitiveType.FullName); } } ////// The type kind of this type. /// public PrimitiveTypeKind TypeKind { get { return _primitiveType.PrimitiveTypeKind; } } ////// Returns the PrimitiveType of the scalar type. /// public PrimitiveType Type { get { return _primitiveType; } } private static bool TryParseBoolean(string text, out object value) { Boolean temp; if (!Boolean.TryParse(text, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseByte(string text, out object value) { Byte temp; if (!Byte.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseSByte(string text, out object value) { SByte temp; if (!SByte.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseInt16(string text, out object value) { Int16 temp; if (!Int16.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseInt32(string text, out object value) { Int32 temp; if (!Int32.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseInt64(string text, out object value) { Int64 temp; if (!Int64.TryParse(text, System.Globalization.NumberStyles.Integer, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseDouble(string text, out object value) { Double temp; if (!Double.TryParse(text, System.Globalization.NumberStyles.Float, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseDecimal(string text, out object value) { Decimal temp; if (!Decimal.TryParse(text, System.Globalization.NumberStyles.Float, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseDateTime(string text, out object value) { DateTime temp; if (!DateTime.TryParseExact(text, DateTimeFormat, CultureInfo.InvariantCulture, DateTimeStyles.AssumeUniversal | DateTimeStyles.AdjustToUniversal, out temp)) { value = null; return false; } value = temp; return true; } ////// Parses the default value for Edm Type Time based on the DateTime format "HH:mm:ss.fffffffz". /// The value is first converted to DateTime value and then converted to TimeSpan. /// /// /// ///private static bool TryParseTime(string text, out object value) { DateTime temp; if (!DateTime.TryParseExact(text, TimeFormat, CultureInfo.InvariantCulture, DateTimeStyles.AssumeUniversal | DateTimeStyles.AdjustToUniversal, out temp)) { value = null; return false; } value = new TimeSpan(temp.Ticks); return true; } private static bool TryParseDateTimeOffset(string text, out object value) { DateTimeOffset temp; if (!DateTimeOffset.TryParse(text, out temp)) { value = null; return false; } value = temp; return true; } private static bool TryParseGuid(string text, out object value) { if (!_GuidValueValidator.IsMatch(text)) { value = null; return false; } value = new Guid(text); return true; } private static bool TryParseString(string text, out object value) { value = text; return true; } private static bool TryParseBinary(string text, out object value) { //value must look like 0xddddd... if (!_BinaryValueValidator.IsMatch(text)) { value = null; return false; } int inc = 2; if (text.Length % 2 == 1) inc = 1; int numBytes = (text.Length - 1) / 2; byte[] bytes = new byte[numBytes]; for (int index = 2, iByte = 0; index < text.Length; index += inc, inc = 2, ++iByte) { bytes[iByte] = byte.Parse(text.Substring(index, inc), System.Globalization.NumberStyles.HexNumber, System.Globalization.CultureInfo.InvariantCulture); } value = bytes; return true; } private static bool TryParseSingle(string text, out object value) { Single temp; if (!Single.TryParse(text, System.Globalization.NumberStyles.Float, System.Globalization.CultureInfo.InvariantCulture, out temp)) { value = null; return false; } value = temp; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
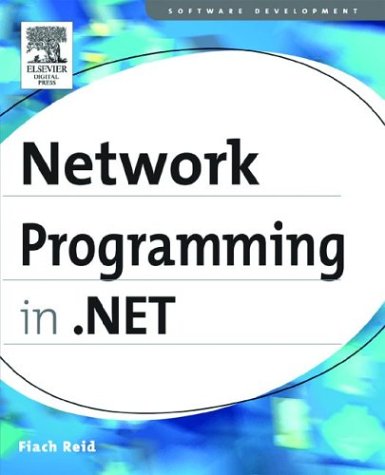
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DBBindings.cs
- NativeMethods.cs
- TraceListeners.cs
- SizeF.cs
- ValidationError.cs
- ChannelReliableSession.cs
- TransformCollection.cs
- CustomLineCap.cs
- DeclaredTypeElementCollection.cs
- ToolStripItemGlyph.cs
- XmlMtomReader.cs
- TextElementAutomationPeer.cs
- PeerInvitationResponse.cs
- ReadOnlyDictionary.cs
- RectKeyFrameCollection.cs
- EntityDataSourceChangingEventArgs.cs
- ToolStripSplitStackLayout.cs
- SystemPens.cs
- RowToParametersTransformer.cs
- JoinCqlBlock.cs
- HwndProxyElementProvider.cs
- HttpCachePolicyElement.cs
- PageWrapper.cs
- DotExpr.cs
- ManagementClass.cs
- SignatureHelper.cs
- SortFieldComparer.cs
- CodeDefaultValueExpression.cs
- FirewallWrapper.cs
- TemplatedAdorner.cs
- SmiContextFactory.cs
- Win32NamedPipes.cs
- XmlHierarchicalEnumerable.cs
- UndoEngine.cs
- HashAlgorithm.cs
- Queue.cs
- XmlArrayItemAttributes.cs
- InternalControlCollection.cs
- ToolStripItemEventArgs.cs
- Operators.cs
- GAC.cs
- FrameworkTemplate.cs
- RenderData.cs
- HttpWebResponse.cs
- SqlNodeAnnotation.cs
- ExitEventArgs.cs
- ListBoxItem.cs
- QilTargetType.cs
- HttpListener.cs
- ControlBindingsCollection.cs
- UnsafeNativeMethodsCLR.cs
- BaseUriHelper.cs
- ConnectionPoolManager.cs
- WinInet.cs
- LingerOption.cs
- ResponseStream.cs
- PriorityItem.cs
- TTSEngineProxy.cs
- MailWebEventProvider.cs
- FixedLineResult.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- HiddenField.cs
- SubtreeProcessor.cs
- PrimitiveType.cs
- DataSourceProvider.cs
- HMACRIPEMD160.cs
- Floater.cs
- Clock.cs
- ECDiffieHellmanCng.cs
- ToolboxCategory.cs
- QuaternionAnimation.cs
- GlyphRun.cs
- HtmlInputRadioButton.cs
- UriExt.cs
- AuthorizationRule.cs
- SplineKeyFrames.cs
- MetabaseServerConfig.cs
- NativeMethodsOther.cs
- GlyphElement.cs
- EntityContainerEmitter.cs
- DateTimeParse.cs
- shaperfactoryquerycachekey.cs
- SubqueryTrackingVisitor.cs
- AssemblyEvidenceFactory.cs
- Themes.cs
- MailAddressParser.cs
- XmlSequenceWriter.cs
- PermissionListSet.cs
- _AcceptOverlappedAsyncResult.cs
- DataGridViewColumnConverter.cs
- SocketInformation.cs
- StateMachineSubscriptionManager.cs
- PropertyTabChangedEvent.cs
- QueryAsyncResult.cs
- ImmutablePropertyDescriptorGridEntry.cs
- BooleanExpr.cs
- DeferredReference.cs
- SmiEventSink.cs
- XmlSchemaImport.cs
- AssemblyHelper.cs