Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Ink / SelectionEditor.cs / 1305600 / SelectionEditor.cs
//---------------------------------------------------------------------------- // // File: SelectionEditor.cs // // Description: // Provides basic ink editing // Features: // // History: // 2/14/2003 samgeo: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- //#define TRACE_MAJOR // DO NOT LEAVE ENABLED IN CHECKED IN CODE //#define TRACE_ADDITIONAL // DO NOT LEAVE ENABLED IN CHECKED IN CODE using MS.Internal; using MS.Internal.Controls; using System; using System.Security; using System.Security.Permissions; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Controls; using System.Windows.Markup; using System.Threading; using System.Windows.Ink; namespace MS.Internal.Ink { ////// SelectionEditor /// internal class SelectionEditor : EditingBehavior { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// SelectionEditor constructor /// internal SelectionEditor(EditingCoordinator editingCoordinator, InkCanvas inkCanvas) : base (editingCoordinator, inkCanvas) { } #endregion Constructors //-------------------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------------------- #region Internal Methods ////// InkCanvas' Selection is changed /// internal void OnInkCanvasSelectionChanged() { Point currentPosition = Mouse.PrimaryDevice.GetPosition(InkCanvas.SelectionAdorner); UpdateSelectionCursor(currentPosition); } #endregion Internal Methods //-------------------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------------------- #region Protected Methods ////// Attach to new scope as defined by this element. /// protected override void OnActivate() { // Add mouse event handles to InkCanvas.SelectionAdorner InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseDownEvent, new MouseButtonEventHandler(OnAdornerMouseButtonDownEvent)); InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseMoveEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseEnterEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseLeaveEvent, new MouseEventHandler(OnAdornerMouseLeaveEvent)); Point currentPosition = Mouse.PrimaryDevice.GetPosition(InkCanvas.SelectionAdorner); UpdateSelectionCursor(currentPosition); } ////// Called when the SelectionEditor is detached /// protected override void OnDeactivate() { // Remove all event hanlders. InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseDownEvent, new MouseButtonEventHandler(OnAdornerMouseButtonDownEvent)); InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseMoveEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseEnterEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseLeaveEvent, new MouseEventHandler(OnAdornerMouseLeaveEvent)); } ////// OnCommit /// /// protected override void OnCommit(bool commit) { // Nothing to do. } ////// Retrieve the cursor /// ///protected override Cursor GetCurrentCursor() { // NTRAID:WINDOWSOS#1648430-2006/05/12-WAYNEZEN, // We only show the selection related cursor when the mouse is over the SelectionAdorner. // If mouse is outside of the SelectionAdorner, we let the system to pick up the default cursor. if ( InkCanvas.SelectionAdorner.IsMouseOver ) { return PenCursorManager.GetSelectionCursor(_hitResult, (this.InkCanvas.FlowDirection == FlowDirection.RightToLeft)); } else { return null; } } #endregion Protected Methods //------------------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------------------- #region Private Methods /// /// SelectionAdorner MouseButtonDown /// /// /// ////// Critical: Calls critical method EditingCoordinator.ActivateDynamicBehavior /// /// TreatAsSafe: Doesn't handle critical data. Also, this method is called by /// SecurityTransparent code in the input system and can not be marked SecurityCritical. /// [SecurityCritical, SecurityTreatAsSafe] private void OnAdornerMouseButtonDownEvent(object sender, MouseButtonEventArgs args) { // If the ButtonDown is raised by RightMouse, we should just bail out. if ( (args.StylusDevice == null && args.LeftButton != MouseButtonState.Pressed) ) { return; } Point pointOnSelectionAdorner = args.GetPosition(InkCanvas.SelectionAdorner); InkCanvasSelectionHitResult hitResult = InkCanvasSelectionHitResult.None; // Check if we should start resizing/moving hitResult = HitTestOnSelectionAdorner(pointOnSelectionAdorner); if ( hitResult != InkCanvasSelectionHitResult.None ) { // We always use MouseDevice for the selection editing. EditingCoordinator.ActivateDynamicBehavior(EditingCoordinator.SelectionEditingBehavior, args.Device); } else { // //push selection and we're done // // If the current captured device is Stylus, we should activate the LassoSelectionBehavior with // the Stylus. Otherwise, use mouse. EditingCoordinator.ActivateDynamicBehavior(EditingCoordinator.LassoSelectionBehavior, args.StylusDevice != null ? args.StylusDevice : args.Device); } } ////// Selection Adorner MouseMove /// /// /// ////// Critical: Calls critical method EditingCoordinator.ActivateDynamicBehavior /// /// TreatAsSafe: Doesn't handle critical data. Also, this method is called by /// SecurityTransparent code in the input system and can not be marked SecurityCritical. /// [SecurityCritical, SecurityTreatAsSafe] private void OnAdornerMouseMoveEvent(object sender, MouseEventArgs args) { Point pointOnSelectionAdorner = args.GetPosition(InkCanvas.SelectionAdorner); UpdateSelectionCursor(pointOnSelectionAdorner); } ////// Adorner MouseLeave Handler /// /// /// private void OnAdornerMouseLeaveEvent(object sender, MouseEventArgs args) { // We are leaving the adorner, update our cursor. EditingCoordinator.InvalidateBehaviorCursor(this); } ////// Hit test for the grab handles /// /// ///private InkCanvasSelectionHitResult HitTestOnSelectionAdorner(Point position) { InkCanvasSelectionHitResult hitResult = InkCanvasSelectionHitResult.None; if ( InkCanvas.InkCanvasSelection.HasSelection ) { // First, do hit test on the adorner hitResult = InkCanvas.SelectionAdorner.SelectionHandleHitTest(position); // Now, check if ResizeEnabled or MoveEnabled has been set. // If so, reset the grab handle. if ( hitResult >= InkCanvasSelectionHitResult.TopLeft && hitResult <= InkCanvasSelectionHitResult.Left ) { hitResult = InkCanvas.ResizeEnabled ? hitResult : InkCanvasSelectionHitResult.None; } else if ( hitResult == InkCanvasSelectionHitResult.Selection ) { hitResult = InkCanvas.MoveEnabled ? hitResult : InkCanvasSelectionHitResult.None; } } return hitResult; } /// /// This method updates the cursor when the mouse is hovering ont the selection adorner. /// It is called from /// OnAdornerMouseLeaveEvent /// OnAdornerMouseEvent /// /// the handle is being hit private void UpdateSelectionCursor(Point hitPoint) { InkCanvasSelectionHitResult hitResult = HitTestOnSelectionAdorner(hitPoint); if ( _hitResult != hitResult ) { // Keep the current handle _hitResult = hitResult; EditingCoordinator.InvalidateBehaviorCursor(this); } } #endregion Private Methods //------------------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------------------- #region Private Fields private InkCanvasSelectionHitResult _hitResult; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: SelectionEditor.cs // // Description: // Provides basic ink editing // Features: // // History: // 2/14/2003 samgeo: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- //#define TRACE_MAJOR // DO NOT LEAVE ENABLED IN CHECKED IN CODE //#define TRACE_ADDITIONAL // DO NOT LEAVE ENABLED IN CHECKED IN CODE using MS.Internal; using MS.Internal.Controls; using System; using System.Security; using System.Security.Permissions; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Controls; using System.Windows.Markup; using System.Threading; using System.Windows.Ink; namespace MS.Internal.Ink { ////// SelectionEditor /// internal class SelectionEditor : EditingBehavior { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// SelectionEditor constructor /// internal SelectionEditor(EditingCoordinator editingCoordinator, InkCanvas inkCanvas) : base (editingCoordinator, inkCanvas) { } #endregion Constructors //-------------------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------------------- #region Internal Methods ////// InkCanvas' Selection is changed /// internal void OnInkCanvasSelectionChanged() { Point currentPosition = Mouse.PrimaryDevice.GetPosition(InkCanvas.SelectionAdorner); UpdateSelectionCursor(currentPosition); } #endregion Internal Methods //-------------------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------------------- #region Protected Methods ////// Attach to new scope as defined by this element. /// protected override void OnActivate() { // Add mouse event handles to InkCanvas.SelectionAdorner InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseDownEvent, new MouseButtonEventHandler(OnAdornerMouseButtonDownEvent)); InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseMoveEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseEnterEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.AddHandler(Mouse.MouseLeaveEvent, new MouseEventHandler(OnAdornerMouseLeaveEvent)); Point currentPosition = Mouse.PrimaryDevice.GetPosition(InkCanvas.SelectionAdorner); UpdateSelectionCursor(currentPosition); } ////// Called when the SelectionEditor is detached /// protected override void OnDeactivate() { // Remove all event hanlders. InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseDownEvent, new MouseButtonEventHandler(OnAdornerMouseButtonDownEvent)); InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseMoveEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseEnterEvent, new MouseEventHandler(OnAdornerMouseMoveEvent)); InkCanvas.SelectionAdorner.RemoveHandler(Mouse.MouseLeaveEvent, new MouseEventHandler(OnAdornerMouseLeaveEvent)); } ////// OnCommit /// /// protected override void OnCommit(bool commit) { // Nothing to do. } ////// Retrieve the cursor /// ///protected override Cursor GetCurrentCursor() { // NTRAID:WINDOWSOS#1648430-2006/05/12-WAYNEZEN, // We only show the selection related cursor when the mouse is over the SelectionAdorner. // If mouse is outside of the SelectionAdorner, we let the system to pick up the default cursor. if ( InkCanvas.SelectionAdorner.IsMouseOver ) { return PenCursorManager.GetSelectionCursor(_hitResult, (this.InkCanvas.FlowDirection == FlowDirection.RightToLeft)); } else { return null; } } #endregion Protected Methods //------------------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------------------- #region Private Methods /// /// SelectionAdorner MouseButtonDown /// /// /// ////// Critical: Calls critical method EditingCoordinator.ActivateDynamicBehavior /// /// TreatAsSafe: Doesn't handle critical data. Also, this method is called by /// SecurityTransparent code in the input system and can not be marked SecurityCritical. /// [SecurityCritical, SecurityTreatAsSafe] private void OnAdornerMouseButtonDownEvent(object sender, MouseButtonEventArgs args) { // If the ButtonDown is raised by RightMouse, we should just bail out. if ( (args.StylusDevice == null && args.LeftButton != MouseButtonState.Pressed) ) { return; } Point pointOnSelectionAdorner = args.GetPosition(InkCanvas.SelectionAdorner); InkCanvasSelectionHitResult hitResult = InkCanvasSelectionHitResult.None; // Check if we should start resizing/moving hitResult = HitTestOnSelectionAdorner(pointOnSelectionAdorner); if ( hitResult != InkCanvasSelectionHitResult.None ) { // We always use MouseDevice for the selection editing. EditingCoordinator.ActivateDynamicBehavior(EditingCoordinator.SelectionEditingBehavior, args.Device); } else { // //push selection and we're done // // If the current captured device is Stylus, we should activate the LassoSelectionBehavior with // the Stylus. Otherwise, use mouse. EditingCoordinator.ActivateDynamicBehavior(EditingCoordinator.LassoSelectionBehavior, args.StylusDevice != null ? args.StylusDevice : args.Device); } } ////// Selection Adorner MouseMove /// /// /// ////// Critical: Calls critical method EditingCoordinator.ActivateDynamicBehavior /// /// TreatAsSafe: Doesn't handle critical data. Also, this method is called by /// SecurityTransparent code in the input system and can not be marked SecurityCritical. /// [SecurityCritical, SecurityTreatAsSafe] private void OnAdornerMouseMoveEvent(object sender, MouseEventArgs args) { Point pointOnSelectionAdorner = args.GetPosition(InkCanvas.SelectionAdorner); UpdateSelectionCursor(pointOnSelectionAdorner); } ////// Adorner MouseLeave Handler /// /// /// private void OnAdornerMouseLeaveEvent(object sender, MouseEventArgs args) { // We are leaving the adorner, update our cursor. EditingCoordinator.InvalidateBehaviorCursor(this); } ////// Hit test for the grab handles /// /// ///private InkCanvasSelectionHitResult HitTestOnSelectionAdorner(Point position) { InkCanvasSelectionHitResult hitResult = InkCanvasSelectionHitResult.None; if ( InkCanvas.InkCanvasSelection.HasSelection ) { // First, do hit test on the adorner hitResult = InkCanvas.SelectionAdorner.SelectionHandleHitTest(position); // Now, check if ResizeEnabled or MoveEnabled has been set. // If so, reset the grab handle. if ( hitResult >= InkCanvasSelectionHitResult.TopLeft && hitResult <= InkCanvasSelectionHitResult.Left ) { hitResult = InkCanvas.ResizeEnabled ? hitResult : InkCanvasSelectionHitResult.None; } else if ( hitResult == InkCanvasSelectionHitResult.Selection ) { hitResult = InkCanvas.MoveEnabled ? hitResult : InkCanvasSelectionHitResult.None; } } return hitResult; } /// /// This method updates the cursor when the mouse is hovering ont the selection adorner. /// It is called from /// OnAdornerMouseLeaveEvent /// OnAdornerMouseEvent /// /// the handle is being hit private void UpdateSelectionCursor(Point hitPoint) { InkCanvasSelectionHitResult hitResult = HitTestOnSelectionAdorner(hitPoint); if ( _hitResult != hitResult ) { // Keep the current handle _hitResult = hitResult; EditingCoordinator.InvalidateBehaviorCursor(this); } } #endregion Private Methods //------------------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------------------- #region Private Fields private InkCanvasSelectionHitResult _hitResult; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
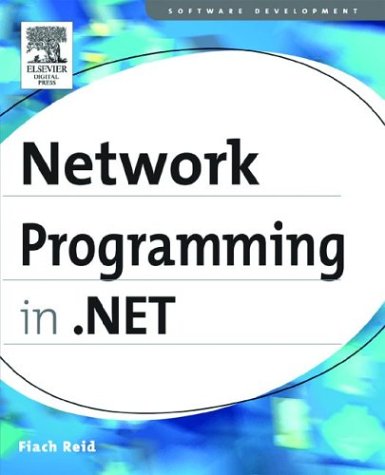
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlCommandBuilder.cs
- SizeValueSerializer.cs
- AbstractDataSvcMapFileLoader.cs
- FilterException.cs
- ServiceOperationHelpers.cs
- XPathNode.cs
- VirtualizingStackPanel.cs
- FramingFormat.cs
- UniqueConstraint.cs
- BufferAllocator.cs
- CodeTypeReferenceCollection.cs
- Command.cs
- TextEditorThreadLocalStore.cs
- ToolStripSeparatorRenderEventArgs.cs
- CompositionAdorner.cs
- NameValueFileSectionHandler.cs
- SynthesizerStateChangedEventArgs.cs
- StateChangeEvent.cs
- DbDataSourceEnumerator.cs
- AdornedElementPlaceholder.cs
- ResourceManagerWrapper.cs
- TableStyle.cs
- MatcherBuilder.cs
- DataConnectionHelper.cs
- IERequestCache.cs
- MasterPageCodeDomTreeGenerator.cs
- BasicAsyncResult.cs
- ByteStack.cs
- ToolStripPanelRenderEventArgs.cs
- Renderer.cs
- DateTimeFormat.cs
- BooleanExpr.cs
- DesignTimeParseData.cs
- XsltConvert.cs
- TransformGroup.cs
- TypeBuilder.cs
- OdbcDataAdapter.cs
- ConnectionManagementElementCollection.cs
- ApplicationSecurityManager.cs
- ChtmlCommandAdapter.cs
- NameValueConfigurationElement.cs
- OleTxTransaction.cs
- CompilerParameters.cs
- ObjectDataSourceChooseTypePanel.cs
- MutexSecurity.cs
- ClientTarget.cs
- DBPropSet.cs
- DataGridViewSelectedCellCollection.cs
- OutOfMemoryException.cs
- GradientBrush.cs
- WebMessageEncodingElement.cs
- CodeTypeMember.cs
- QueryLifecycle.cs
- WebPartManager.cs
- EndGetFileNameFromUserRequest.cs
- HttpWebResponse.cs
- BindingSourceDesigner.cs
- IisTraceListener.cs
- SerializationHelper.cs
- Semaphore.cs
- EntityViewGenerationConstants.cs
- ChangeConflicts.cs
- ExtenderProvidedPropertyAttribute.cs
- SpeechDetectedEventArgs.cs
- SparseMemoryStream.cs
- Opcode.cs
- TypeBuilder.cs
- ScrollItemPattern.cs
- ProxyElement.cs
- ToolStripManager.cs
- SqlTransaction.cs
- ObjectDataSourceView.cs
- LessThanOrEqual.cs
- BooleanToVisibilityConverter.cs
- CacheHelper.cs
- DoubleAnimation.cs
- DynamicResourceExtension.cs
- Stylesheet.cs
- AddToCollection.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- Bezier.cs
- GenericAuthenticationEventArgs.cs
- FixedPageAutomationPeer.cs
- DataColumnCollection.cs
- TransformedBitmap.cs
- AppSettingsReader.cs
- CatalogPart.cs
- RegexCapture.cs
- EmptyEnumerator.cs
- DesignerMetadata.cs
- DummyDataSource.cs
- CheckBoxPopupAdapter.cs
- WebPartEditorOkVerb.cs
- CommandDevice.cs
- PropertyChangingEventArgs.cs
- DataGridRowsPresenter.cs
- TextParaLineResult.cs
- TimeSpan.cs
- DispatcherHooks.cs
- X509CertificateValidator.cs