Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / InterOp / HwndAppCommandInputProvider.cs / 1 / HwndAppCommandInputProvider.cs
using System.Windows.Input; using System.Windows.Media; using System.Windows.Threading; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Utility; using MS.Internal; using MS.Win32; namespace System.Windows.Interop { internal sealed class HwndAppCommandInputProvider : DispatcherObject, IInputProvider, IDisposable { ////// Accesses and store critical data. This class is also critical (_site and _source) /// [SecurityCritical] internal HwndAppCommandInputProvider( HwndSource source ) { (new UIPermission(PermissionState.Unrestricted)).Assert(); try { _site = new SecurityCriticalDataClass(InputManager.Current.RegisterInputProvider(this)); } finally { UIPermission.RevertAssert(); } _source = new SecurityCriticalDataClass (source); } /// /// Critical:This class accesses critical data, _site. /// TreatAsSafe: This class does not expose the critical data /// [SecurityCritical, SecurityTreatAsSafe] public void Dispose( ) { if (_site != null) { _site.Value.Dispose(); _site = null; } _source = null; } ////// Critical: As this accesses critical data HwndSource /// TreatAsSafe:Information about whether a given input provider services /// a visual is safe to expose. This method does not expose the critical data either. /// [SecurityCritical, SecurityTreatAsSafe] bool IInputProvider.ProvidesInputForRootVisual( Visual v ) { Debug.Assert(null != _source); return _source.Value.RootVisual == v; } void IInputProvider.NotifyDeactivate() {} ////// Critical: As this accesses critical data HwndSource /// [SecurityCritical] internal IntPtr FilterMessage( IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled ) { // It is possible to be re-entered during disposal. Just return. if(null == _source || null == _source.Value) { return IntPtr.Zero; } if (msg == NativeMethods.WM_APPCOMMAND) { // WM_APPCOMMAND message notifies a window that the user generated an application command event, // for example, by clicking an application command button using the mouse or typing an application command // key on the keyboard. RawAppCommandInputReport report = new RawAppCommandInputReport( _source.Value, InputMode.Foreground, SafeNativeMethods.GetMessageTime(), GetAppCommand(lParam), GetDevice(lParam), InputType.Command); handled = _site.Value.ReportInput(report); } return handled ? new IntPtr(1) : IntPtr.Zero ; } ////// Implementation of the GET_APPCOMMAND_LPARAM macro defined in Winuser.h /// /// ///private static int GetAppCommand( IntPtr lParam ) { return ((short)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & ~NativeMethods.FAPPCOMMAND_MASK)); } /// /// Returns the input device that originated this app command. /// InputType.Hid represents an unspecified device that is neither the /// keyboard nor the mouse. /// /// ///private static InputType GetDevice(IntPtr lParam) { InputType inputType = InputType.Hid; // Implementation of the GET_DEVICE_LPARAM macro defined in Winuser.h // Returns either FAPPCOMMAND_KEY (the user pressed a key), FAPPCOMMAND_MOUSE // (the user clicked a mouse button) or FAPPCOMMAND_OEM (unknown device) ushort deviceId = (ushort)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & NativeMethods.FAPPCOMMAND_MASK); switch (deviceId) { case NativeMethods.FAPPCOMMAND_MOUSE: inputType = InputType.Mouse; break; case NativeMethods.FAPPCOMMAND_KEY: inputType = InputType.Keyboard; break; case NativeMethods.FAPPCOMMAND_OEM: default: // Unknown device id or FAPPCOMMAND_OEM. // In either cases we set it to the generic human interface device. inputType=InputType.Hid; break; } return inputType; } /// /// This is got under an elevation and is hence critical. This data is not ok to expose. /// private SecurityCriticalDataClass_source; /// /// This is got under an elevation and is hence critical.This data is not ok to expose. /// private SecurityCriticalDataClass_site; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Windows.Input; using System.Windows.Media; using System.Windows.Threading; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Utility; using MS.Internal; using MS.Win32; namespace System.Windows.Interop { internal sealed class HwndAppCommandInputProvider : DispatcherObject, IInputProvider, IDisposable { /// /// Accesses and store critical data. This class is also critical (_site and _source) /// [SecurityCritical] internal HwndAppCommandInputProvider( HwndSource source ) { (new UIPermission(PermissionState.Unrestricted)).Assert(); try { _site = new SecurityCriticalDataClass(InputManager.Current.RegisterInputProvider(this)); } finally { UIPermission.RevertAssert(); } _source = new SecurityCriticalDataClass (source); } /// /// Critical:This class accesses critical data, _site. /// TreatAsSafe: This class does not expose the critical data /// [SecurityCritical, SecurityTreatAsSafe] public void Dispose( ) { if (_site != null) { _site.Value.Dispose(); _site = null; } _source = null; } ////// Critical: As this accesses critical data HwndSource /// TreatAsSafe:Information about whether a given input provider services /// a visual is safe to expose. This method does not expose the critical data either. /// [SecurityCritical, SecurityTreatAsSafe] bool IInputProvider.ProvidesInputForRootVisual( Visual v ) { Debug.Assert(null != _source); return _source.Value.RootVisual == v; } void IInputProvider.NotifyDeactivate() {} ////// Critical: As this accesses critical data HwndSource /// [SecurityCritical] internal IntPtr FilterMessage( IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled ) { // It is possible to be re-entered during disposal. Just return. if(null == _source || null == _source.Value) { return IntPtr.Zero; } if (msg == NativeMethods.WM_APPCOMMAND) { // WM_APPCOMMAND message notifies a window that the user generated an application command event, // for example, by clicking an application command button using the mouse or typing an application command // key on the keyboard. RawAppCommandInputReport report = new RawAppCommandInputReport( _source.Value, InputMode.Foreground, SafeNativeMethods.GetMessageTime(), GetAppCommand(lParam), GetDevice(lParam), InputType.Command); handled = _site.Value.ReportInput(report); } return handled ? new IntPtr(1) : IntPtr.Zero ; } ////// Implementation of the GET_APPCOMMAND_LPARAM macro defined in Winuser.h /// /// ///private static int GetAppCommand( IntPtr lParam ) { return ((short)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & ~NativeMethods.FAPPCOMMAND_MASK)); } /// /// Returns the input device that originated this app command. /// InputType.Hid represents an unspecified device that is neither the /// keyboard nor the mouse. /// /// ///private static InputType GetDevice(IntPtr lParam) { InputType inputType = InputType.Hid; // Implementation of the GET_DEVICE_LPARAM macro defined in Winuser.h // Returns either FAPPCOMMAND_KEY (the user pressed a key), FAPPCOMMAND_MOUSE // (the user clicked a mouse button) or FAPPCOMMAND_OEM (unknown device) ushort deviceId = (ushort)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & NativeMethods.FAPPCOMMAND_MASK); switch (deviceId) { case NativeMethods.FAPPCOMMAND_MOUSE: inputType = InputType.Mouse; break; case NativeMethods.FAPPCOMMAND_KEY: inputType = InputType.Keyboard; break; case NativeMethods.FAPPCOMMAND_OEM: default: // Unknown device id or FAPPCOMMAND_OEM. // In either cases we set it to the generic human interface device. inputType=InputType.Hid; break; } return inputType; } /// /// This is got under an elevation and is hence critical. This data is not ok to expose. /// private SecurityCriticalDataClass_source; /// /// This is got under an elevation and is hence critical.This data is not ok to expose. /// private SecurityCriticalDataClass_site; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
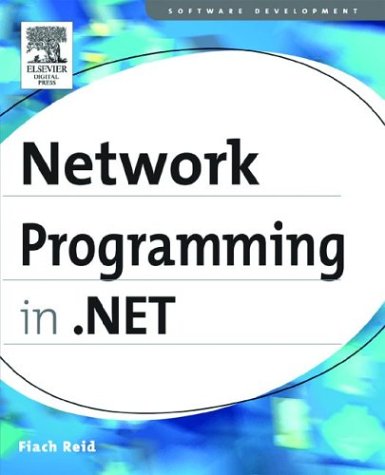
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageRequestManager.cs
- SystemIPInterfaceStatistics.cs
- StyleXamlTreeBuilder.cs
- DataPager.cs
- MetadataSource.cs
- HMACSHA384.cs
- RecordBuilder.cs
- XmlAttribute.cs
- XXXOnTypeBuilderInstantiation.cs
- DependentList.cs
- DataTableExtensions.cs
- AmbientProperties.cs
- TypeInitializationException.cs
- StorageAssociationSetMapping.cs
- PointAnimationUsingKeyFrames.cs
- TimeSpan.cs
- FloaterParagraph.cs
- ServiceOperationInfoTypeConverter.cs
- MetadataLocation.cs
- RowUpdatedEventArgs.cs
- SecurityContext.cs
- ListBoxItemAutomationPeer.cs
- backend.cs
- HtmlInputImage.cs
- DivideByZeroException.cs
- ResolveCriteriaApril2005.cs
- InvokeProviderWrapper.cs
- OdbcParameterCollection.cs
- PLINQETWProvider.cs
- DoubleLinkListEnumerator.cs
- SplineKeyFrames.cs
- MexHttpBindingElement.cs
- EventLogPermission.cs
- SqlTypeConverter.cs
- AsyncCompletedEventArgs.cs
- SqlAliasesReferenced.cs
- SafeRightsManagementQueryHandle.cs
- QilSortKey.cs
- PropertyDescriptor.cs
- XmlSchemaSimpleContent.cs
- SystemNetworkInterface.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- DodSequenceMerge.cs
- EntityConnection.cs
- HostedNamedPipeTransportManager.cs
- ProxyAttribute.cs
- SqlBuffer.cs
- ASCIIEncoding.cs
- FloatUtil.cs
- TableCell.cs
- DataSet.cs
- NoPersistScope.cs
- relpropertyhelper.cs
- RoutedCommand.cs
- ScriptingProfileServiceSection.cs
- ConcurrencyMode.cs
- _KerberosClient.cs
- Aes.cs
- assemblycache.cs
- DataMemberConverter.cs
- CommandExpr.cs
- ComponentChangedEvent.cs
- SelectionEditingBehavior.cs
- dataobject.cs
- SHA256Managed.cs
- NullableDecimalAverageAggregationOperator.cs
- SyndicationLink.cs
- ImageFormat.cs
- WebPartHelpVerb.cs
- ReadOnlyHierarchicalDataSource.cs
- MarkedHighlightComponent.cs
- BooleanProjectedSlot.cs
- ListControl.cs
- DictionaryGlobals.cs
- SortDescription.cs
- DataGridItemAutomationPeer.cs
- InternalsVisibleToAttribute.cs
- StateDesigner.Helpers.cs
- XPathAncestorQuery.cs
- PixelFormat.cs
- Expr.cs
- dbdatarecord.cs
- SspiSecurityTokenProvider.cs
- Attributes.cs
- Double.cs
- EpmTargetPathSegment.cs
- RegexWorker.cs
- SessionPageStateSection.cs
- PhonemeConverter.cs
- Stacktrace.cs
- DataGridToolTip.cs
- CodeRegionDirective.cs
- Bezier.cs
- X500Name.cs
- PathFigureCollectionConverter.cs
- PropertyIdentifier.cs
- Calendar.cs
- IndexExpression.cs
- BypassElementCollection.cs
- ClickablePoint.cs