Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / ListItem.cs / 1 / ListItem.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: ListItem acts by default like a Paragraph, but with // different margins/padding and features to support markers // such as bullets and numbering. // // History: // 06/06/2003 : [....] - created. // 10/28/2004 : [....] - ContentElements refactoring. // //--------------------------------------------------------------------------- using MS.Internal; // Invariant using System.Windows.Markup; // ContentProperty using System.ComponentModel; // TypeConverter using System.Windows.Media; // Brush namespace System.Windows.Documents { ////// ListItem acts by default like a Paragraph, but with different /// margins/padding and features to support markers such as bullets and /// numbering. /// [ContentProperty("Blocks")] public class ListItem : TextElement { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of a ListItem element. /// public ListItem() : base() { } ////// Initializes a new instance of a ListItem element specifying a Paragraph element as its initial child. /// /// /// Paragraph added as a single child of a ListItem /// public ListItem(Paragraph paragraph) : base() { if (paragraph == null) { throw new ArgumentNullException("paragraph"); } this.Blocks.Add(paragraph); } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Parent element as IBlockItemParent through which a Children collection /// containing this BlockItem is available for Adding/Removing purposes. /// public List List { get { Invariant.Assert(this.Parent == null || this.Parent is List); return (List)this.Parent; } } ////// Collection of BlockItems contained in this ListItem. /// Usually this collection contains only one Paragraph element. /// In case of nested lists it can contain List element as a second /// item of the collection. /// More Paragraphs can be added to a collection as well. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public BlockCollection Blocks { get { return new BlockCollection(this, /*isOwnerParent*/true); } } ////// A collection of ListItems containing this one in its sequential tree. /// May return null if an element is not inserted into any tree. /// public ListItemCollection SiblingListItems { get { if (this.Parent == null) { return null; } return new ListItemCollection(this, /*isOwnerParent*/false); } } ////// Returns a ListItem immediately following this one /// public ListItem NextListItem { get { return this.NextElement as ListItem; } } ////// Returns a block immediately preceding this one /// on the same level of siblings /// public ListItem PreviousListItem { get { return this.PreviousElement as ListItem; } } ////// DependencyProperty for public static readonly DependencyProperty MarginProperty = Block.MarginProperty.AddOwner( typeof(ListItem), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Margin property specifies the margin of the element. /// public Thickness Margin { get { return (Thickness)GetValue(MarginProperty); } set { SetValue(MarginProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty PaddingProperty = Block.PaddingProperty.AddOwner( typeof(ListItem), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = Block.BorderThicknessProperty.AddOwner( typeof(ListItem), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = Block.BorderBrushProperty.AddOwner( typeof(ListItem), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = Block.TextAlignmentProperty.AddOwner(typeof(ListItem)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = Block.FlowDirectionProperty.AddOwner(typeof(ListItem)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = Block.LineHeightProperty.AddOwner(typeof(ListItem)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = Block.LineStackingStrategyProperty.AddOwner(typeof(ListItem)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } #endregion Public Properties #region Internal Methods ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeBlocks(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
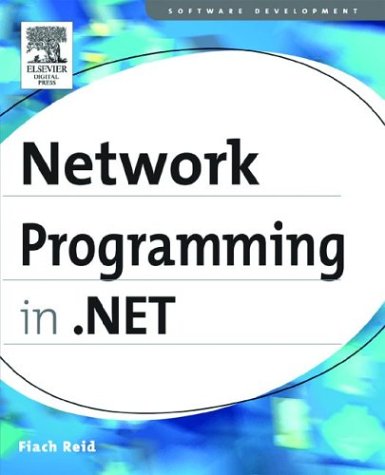
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityRuntime.cs
- ContextToken.cs
- WindowsTitleBar.cs
- IteratorDescriptor.cs
- XPathNodeIterator.cs
- StringComparer.cs
- ServiceObjectContainer.cs
- KeyValuePairs.cs
- StringArrayConverter.cs
- ListParaClient.cs
- PowerEase.cs
- HttpModuleActionCollection.cs
- _ListenerResponseStream.cs
- SafeCertificateStore.cs
- AspProxy.cs
- ItemsChangedEventArgs.cs
- InternalConfigEventArgs.cs
- DateTimeStorage.cs
- GenericTextProperties.cs
- WrappedDispatcherException.cs
- OutputScopeManager.cs
- RegexCharClass.cs
- CompositeKey.cs
- XsltQilFactory.cs
- HttpListenerRequest.cs
- GridSplitter.cs
- DbDataAdapter.cs
- WebSysDisplayNameAttribute.cs
- HttpUnhandledOperationInvoker.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- NonClientArea.cs
- BitmapMetadataBlob.cs
- ValidationErrorEventArgs.cs
- MenuItemCollection.cs
- HtmlValidatorAdapter.cs
- SerializationException.cs
- ElementAction.cs
- SystemUnicastIPAddressInformation.cs
- SQLByteStorage.cs
- SafeCloseHandleCritical.cs
- OpenTypeCommon.cs
- DeclarativeCatalogPart.cs
- DrawingDrawingContext.cs
- SuspendDesigner.cs
- IisTraceWebEventProvider.cs
- WmlSelectionListAdapter.cs
- LineServicesRun.cs
- TraceInternal.cs
- RuntimeConfigLKG.cs
- DataTableNewRowEvent.cs
- ColorKeyFrameCollection.cs
- XslNumber.cs
- WpfSharedBamlSchemaContext.cs
- ExpressionQuoter.cs
- ApplicationActivator.cs
- Duration.cs
- CustomError.cs
- EmptyStringExpandableObjectConverter.cs
- ServiceDebugElement.cs
- SemaphoreSlim.cs
- XmlLoader.cs
- InputDevice.cs
- ISFClipboardData.cs
- TagPrefixInfo.cs
- OpenFileDialog.cs
- FragmentQueryKB.cs
- HMACSHA1.cs
- isolationinterop.cs
- ObjectPersistData.cs
- XamlHostingSection.cs
- Pointer.cs
- TextSelectionHelper.cs
- CacheHelper.cs
- ComboBoxRenderer.cs
- WebEventCodes.cs
- WriteStateInfoBase.cs
- CurrentTimeZone.cs
- DataPagerFieldCollection.cs
- SafeRightsManagementEnvironmentHandle.cs
- BindingListCollectionView.cs
- EntityTemplateFactory.cs
- TailPinnedEventArgs.cs
- EntityDesignerDataSourceView.cs
- UInt64.cs
- ContentDesigner.cs
- Knowncolors.cs
- DataServiceQueryProvider.cs
- Attribute.cs
- DataGridColumnFloatingHeader.cs
- MetadataArtifactLoaderCompositeFile.cs
- OptimizerPatterns.cs
- PropertyReferenceSerializer.cs
- _LocalDataStore.cs
- ZipIOExtraFieldZip64Element.cs
- BamlStream.cs
- BaseAsyncResult.cs
- CultureInfo.cs
- XmlJsonWriter.cs
- StorageAssociationTypeMapping.cs
- IRCollection.cs