Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / Context.cs / 1 / Context.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the wire representation of a WS-Coordination coordination context using System; using System.ServiceModel.Channels; using System.Collections.Generic; using System.Diagnostics; using System.Runtime.Serialization; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Transactions; using System.Threading; using System.Transactions; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; using Microsoft.Transactions.Bridge; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.Messaging { class CoordinationContext : IXmlSerializable { CoordinationStrings coordinationStrings; CoordinationXmlDictionaryStrings coordinationXmlDictionaryStrings; AtomicTransactionXmlDictionaryStrings atomicTransactionXmlDictionaryStrings; ProtocolVersion protocolVersion; public CoordinationContext(ProtocolVersion protocolVersion) { this.coordinationStrings = CoordinationStrings.Version(protocolVersion); this.coordinationXmlDictionaryStrings = CoordinationXmlDictionaryStrings.Version(protocolVersion); this.atomicTransactionXmlDictionaryStrings = AtomicTransactionXmlDictionaryStrings.Version(protocolVersion); this.protocolVersion = protocolVersion; } // We restrict WS-AT transaction identifiers to 256 characters // This is to protect MSDTC from very large messages, as it does // not handle them very well (see MB 32423) // // For what it's worth, the TIP protocol has the same limit on its URLs. public const int MaxIdentifierLength = 256; string contextId; public string Identifier { get { return contextId; } set { contextId = value; } } // Unrecognized attributes ListunknownIdentifierAttributes; bool expiresPresent; public bool ExpiresPresent { get { return this.expiresPresent; } } uint expiration; public uint Expires { get { return expiration; } set { this.expiration = value; this.expiresPresent = true; } } // Unrecognized attributes List unknownExpiresAttributes; EndpointAddress registrationRef; public EndpointAddress RegistrationService { get { return registrationRef; } set { registrationRef = value; } } // // Any elements // IsolationLevel isoLevel = IsolationLevel.Unspecified; public IsolationLevel IsolationLevel { get { return isoLevel; } set { isoLevel = value; } } IsolationFlags isoFlags = 0; public IsolationFlags IsolationFlags { get { return isoFlags; } set { isoFlags = value; } } string description; public string Description { get { return description; } set { description = value; } } Guid localTxId = Guid.Empty; public Guid LocalTransactionId { get { return localTxId; } set { localTxId = value; } } byte[] propToken; public byte[] PropagationToken { get { return this.propToken; } set { this.propToken = value; } } public ProtocolVersion ProtocolVersion { get { return this.protocolVersion; } } // Unrecognized elements List unknownData; public void WriteTo(XmlDictionaryWriter writer, XmlDictionaryString localName, XmlDictionaryString ns) { writer.WriteStartElement(this.coordinationStrings.Prefix, localName, ns); WriteContent(writer); writer.WriteEndElement(); } public void WriteContent(XmlDictionaryWriter writer) { // Hoist the mstx namespace if necessary if (this.isoLevel != IsolationLevel.Unspecified || this.localTxId != Guid.Empty) { writer.WriteXmlnsAttribute(DotNetAtomicTransactionExternalStrings.Prefix, XD.DotNetAtomicTransactionExternalDictionary.Namespace); } // Identifier writer.WriteStartElement(this.coordinationStrings.Prefix, this.coordinationXmlDictionaryStrings.Identifier, this.coordinationXmlDictionaryStrings.Namespace); if (this.unknownIdentifierAttributes != null) { foreach (XmlNode attr in this.unknownIdentifierAttributes) { attr.WriteTo(writer); } } writer.WriteString(this.contextId); writer.WriteEndElement(); // Expires if (this.expiresPresent) { writer.WriteStartElement(this.coordinationXmlDictionaryStrings.Expires, this.coordinationXmlDictionaryStrings.Namespace); if (this.unknownExpiresAttributes != null) { foreach (XmlNode attr in this.unknownExpiresAttributes) { attr.WriteTo(writer); } } writer.WriteValue(this.expiration); writer.WriteEndElement(); } // Coordination Type writer.WriteStartElement(this.coordinationXmlDictionaryStrings.CoordinationType, this.coordinationXmlDictionaryStrings.Namespace); writer.WriteString(this.atomicTransactionXmlDictionaryStrings.Namespace); writer.WriteEndElement(); // Registration Service this.registrationRef.WriteTo(MessagingVersionHelper.AddressingVersion(protocolVersion), writer, this.coordinationXmlDictionaryStrings.RegistrationService, this.coordinationXmlDictionaryStrings.Namespace); // // Any elements // // Isolation level if (this.isoLevel != IsolationLevel.Unspecified) { writer.WriteStartElement(XD.DotNetAtomicTransactionExternalDictionary.IsolationLevel, XD.DotNetAtomicTransactionExternalDictionary.Namespace); writer.WriteValue((int) isoLevel); writer.WriteEndElement(); } // IsolationFlags if (this.isoFlags != 0) { writer.WriteStartElement(XD.DotNetAtomicTransactionExternalDictionary.IsolationFlags, XD.DotNetAtomicTransactionExternalDictionary.Namespace); writer.WriteValue((int) this.isoFlags); writer.WriteEndElement(); } // Description if (!String.IsNullOrEmpty(this.description)) { writer.WriteStartElement(XD.DotNetAtomicTransactionExternalDictionary.Description, XD.DotNetAtomicTransactionExternalDictionary.Namespace); writer.WriteValue(this.description); writer.WriteEndElement(); } // Local transaction id if (this.localTxId != Guid.Empty) { writer.WriteStartElement(XD.DotNetAtomicTransactionExternalDictionary.LocalTransactionId, XD.DotNetAtomicTransactionExternalDictionary.Namespace); writer.WriteValue(localTxId); writer.WriteEndElement(); } if (this.propToken != null) { OleTxTransactionHeader.WritePropagationTokenElement(writer, this.propToken); } // Unknown data if (this.unknownData != null) { int count = this.unknownData.Count; for (int i = 0; i < count; i ++) { this.unknownData[i].WriteTo (writer); } } } public static CoordinationContext ReadFrom(XmlDictionaryReader reader, XmlDictionaryString localName, XmlDictionaryString ns, ProtocolVersion protocolVersion) { CoordinationContext that = new CoordinationContext(protocolVersion); ReadFrom (that, reader, localName, ns, protocolVersion); return that; } static void ReadFrom(CoordinationContext that, XmlDictionaryReader reader, XmlDictionaryString localName, XmlDictionaryString ns, ProtocolVersion protocolVersion) { try { CoordinationXmlDictionaryStrings coordinationXmlDictionaryStrings = CoordinationXmlDictionaryStrings.Version(protocolVersion); AtomicTransactionStrings atomicTransactionStrings = AtomicTransactionStrings.Version(protocolVersion); reader.ReadFullStartElement(localName, coordinationXmlDictionaryStrings.Namespace); // Identifier reader.MoveToStartElement(coordinationXmlDictionaryStrings.Identifier, coordinationXmlDictionaryStrings.Namespace); that.unknownIdentifierAttributes = ReadOtherAttributes(reader, coordinationXmlDictionaryStrings.Namespace); that.contextId = reader.ReadElementContentAsString().Trim(); if (that.contextId.Length == 0 || that.contextId.Length > MaxIdentifierLength) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext))); } // Verify identifier is really a URI Uri uri; if (!Uri.TryCreate (that.contextId, UriKind.Absolute, out uri)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext))); } // Expires if (reader.IsStartElement(coordinationXmlDictionaryStrings.Expires, coordinationXmlDictionaryStrings.Namespace)) { that.unknownExpiresAttributes = ReadOtherAttributes(reader, coordinationXmlDictionaryStrings.Namespace); int expires = reader.ReadElementContentAsInt(); if (expires < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext))); } that.expiration = (uint) expires; that.expiresPresent = true; } // Coordination Type reader.MoveToStartElement(coordinationXmlDictionaryStrings.CoordinationType, coordinationXmlDictionaryStrings.Namespace); string coordinationType = reader.ReadElementContentAsString().Trim(); if (coordinationType != atomicTransactionStrings.Namespace) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext))); } // Registration Service that.registrationRef = EndpointAddress.ReadFrom(MessagingVersionHelper.AddressingVersion(protocolVersion), reader, coordinationXmlDictionaryStrings.RegistrationService, coordinationXmlDictionaryStrings.Namespace); // // Any elements // // Isolation Level if (reader.IsStartElement(XD.DotNetAtomicTransactionExternalDictionary.IsolationLevel, XD.DotNetAtomicTransactionExternalDictionary.Namespace)) { that.isoLevel = (IsolationLevel) reader.ReadElementContentAsInt(); if (that.IsolationLevel < IsolationLevel.Serializable || that.IsolationLevel > IsolationLevel.Unspecified || that.IsolationLevel == IsolationLevel.Snapshot) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext))); } } // Isolation Flags if (reader.IsStartElement(XD.DotNetAtomicTransactionExternalDictionary.IsolationFlags, XD.DotNetAtomicTransactionExternalDictionary.Namespace)) { that.isoFlags = (IsolationFlags)reader.ReadElementContentAsInt(); // DTC does not verify isolation flags. Neither do we. } // Description if (reader.IsStartElement(XD.DotNetAtomicTransactionExternalDictionary.Description, XD.DotNetAtomicTransactionExternalDictionary.Namespace)) { that.description = reader.ReadElementContentAsString().Trim(); } // Local transaction id if (reader.IsStartElement(XD.DotNetAtomicTransactionExternalDictionary.LocalTransactionId, XD.DotNetAtomicTransactionExternalDictionary.Namespace)) { that.localTxId = reader.ReadElementContentAsGuid(); } if (OleTxTransactionHeader.IsStartPropagationTokenElement(reader)) { that.propToken = OleTxTransactionHeader.ReadPropagationTokenElement(reader); } // Unknown data if (reader.IsStartElement()) { // There are unrecognized elements in this coordination context // The context might have belong to a foreign WS-AT node or a newer .NET-AT node. XmlDocument document = new XmlDocument(); that.unknownData = new List (5); while (reader.IsStartElement()) { XmlNode node = document.ReadNode(reader); that.unknownData.Add(node); } } // End of the envelope reader.ReadEndElement(); } catch (XmlException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext), e)); } } static List ReadOtherAttributes(XmlDictionaryReader reader, XmlDictionaryString ns) { DiagnosticUtility.DebugAssert(reader.IsStartElement(), "The XmlReader must be positioned at a start element"); int attrCount = reader.AttributeCount; if (attrCount == 0) { return null; } XmlDocument doc = new XmlDocument(); List attrList = new List (attrCount); reader.MoveToFirstAttribute(); do { XmlNode attr = doc.ReadNode(reader); if (attr == null || attr.NamespaceURI == ns.Value) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCoordinationContextException(SR.GetString(SR.InvalidCoordinationContext))); } attrList.Add(attr); } while (reader.MoveToNextAttribute()); reader.MoveToElement(); return attrList; } // // IXmlSerializable // // This interface is implemented for tracing purposes, so that a // CoordinationContextRecordSchema can be serialized easily. XmlSchema IXmlSerializable.GetSchema() { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } void IXmlSerializable.ReadXml (XmlReader reader) { XmlDictionaryReader dictReader = XmlDictionaryReader.CreateDictionaryReader(reader); ReadFrom(this, dictReader, this.coordinationXmlDictionaryStrings.CoordinationContext, this.coordinationXmlDictionaryStrings.Namespace, this.protocolVersion); } void IXmlSerializable.WriteXml (XmlWriter writer) { XmlDictionaryWriter dictWriter = XmlDictionaryWriter.CreateDictionaryWriter(writer); this.WriteTo(dictWriter, this.coordinationXmlDictionaryStrings.CoordinationContext, this.coordinationXmlDictionaryStrings.Namespace); } // // Helpers // public const string UuidScheme = "urn:uuid:"; public static string CreateNativeIdentifier(Guid transactionId) { return UuidScheme + transactionId.ToString("D"); } public static bool IsNativeIdentifier(string identifier, Guid transactionId) { return string.Compare(identifier, CreateNativeIdentifier(transactionId), StringComparison.Ordinal) == 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
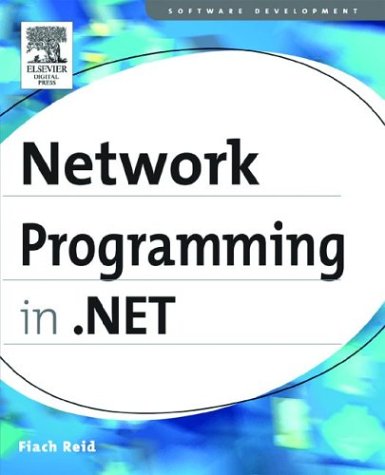
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeViewDesigner.cs
- SingleAnimationUsingKeyFrames.cs
- FrameworkPropertyMetadata.cs
- xmlglyphRunInfo.cs
- TraceSource.cs
- LabelAutomationPeer.cs
- MSG.cs
- Win32Exception.cs
- DocumentAutomationPeer.cs
- ComponentGlyph.cs
- DesignerVerbCollection.cs
- DataGrid.cs
- ListViewUpdateEventArgs.cs
- ConfigurationStrings.cs
- MailAddress.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- PnrpPermission.cs
- SqlConnectionManager.cs
- SinglePhaseEnlistment.cs
- Int32Animation.cs
- SignatureConfirmations.cs
- DataTrigger.cs
- LocalTransaction.cs
- DataConnectionHelper.cs
- WrappingXamlSchemaContext.cs
- PrintingPermissionAttribute.cs
- QueryStringParameter.cs
- SqlTypeConverter.cs
- InstanceLockLostException.cs
- DataGridTable.cs
- CodePageEncoding.cs
- RoleManagerEventArgs.cs
- LinkConverter.cs
- UIElementParagraph.cs
- Content.cs
- PathHelper.cs
- Control.cs
- WindowsTokenRoleProvider.cs
- FileUpload.cs
- _ScatterGatherBuffers.cs
- UIElementAutomationPeer.cs
- UIElementParaClient.cs
- BitmapMetadata.cs
- HostedBindingBehavior.cs
- CachedFontFace.cs
- ToolStripTextBox.cs
- SamlAuthorizationDecisionStatement.cs
- TraceFilter.cs
- TransformCryptoHandle.cs
- HttpException.cs
- OperationSelectorBehavior.cs
- DSACryptoServiceProvider.cs
- SortedList.cs
- DialogResultConverter.cs
- DependencyObject.cs
- HttpWebRequest.cs
- PeerToPeerException.cs
- WsdlBuildProvider.cs
- InvalidCommandTreeException.cs
- FreeIndexList.cs
- Filter.cs
- ProtocolsConfiguration.cs
- FloaterBaseParagraph.cs
- FixedBufferAttribute.cs
- HandoffBehavior.cs
- OleDbReferenceCollection.cs
- NamespaceList.cs
- PropertyEmitterBase.cs
- XmlSchemaGroup.cs
- EventProvider.cs
- SingleSelectRootGridEntry.cs
- DataGridColumnCollection.cs
- AutomationProperties.cs
- PolygonHotSpot.cs
- DataGridTable.cs
- Win32PrintDialog.cs
- VisualTreeFlattener.cs
- ExceptionValidationRule.cs
- Stackframe.cs
- ToolStripPanelRow.cs
- ObjectSerializerFactory.cs
- StyleHelper.cs
- EntityStoreSchemaGenerator.cs
- GeometryConverter.cs
- ConstraintEnumerator.cs
- PrtCap_Public_Simple.cs
- AutomationPatternInfo.cs
- Message.cs
- ServiceMetadataContractBehavior.cs
- InvalidDataContractException.cs
- ParamArrayAttribute.cs
- TextTreeRootNode.cs
- UIElementParagraph.cs
- IntSecurity.cs
- TransactionFilter.cs
- TargetConverter.cs
- TextEffect.cs
- ModuleBuilderData.cs
- IUnknownConstantAttribute.cs
- BuildDependencySet.cs