Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWebControls / System / Data / WebControls / EntityDataSourceWrapperCollection.cs / 1305376 / EntityDataSourceWrapperCollection.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Data; using System.Configuration; using System.Linq; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.HtmlControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Collections; using System.ComponentModel; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Mapping; using System.Data.Objects; using System.Reflection; using System.Diagnostics; using System.Data.Common; using System.Data.Objects.DataClasses; using System.Globalization; using System.Data.EntityClient; using System.Collections.ObjectModel; namespace System.Web.UI.WebControls { ////// Summary description for EntityDataSourceWrapperCollection /// internal class EntityDataSourceWrapperCollection : IEnumerable, ICollection, ITypedList { private readonly ObjectContext _context; private readonly List_wrapperList; /// /// Gets the property descriptors exposed to the user. /// private readonly PropertyDescriptorCollection _visiblePropertyDescriptors; ////// Gets all property descriptors. /// internal readonly ReadOnlyCollectionAllPropertyDescriptors; private readonly bool _isReadOnly; private readonly Type _clrEntityType; internal EntityDataSourceWrapperCollection(ObjectContext context, EntitySet entitySet, EntityType restrictedEntityType) { EntityDataSourceUtil.CheckArgumentNull(context, "context"); EntityDataSourceUtil.CheckArgumentNull(entitySet, "entitySet"); _context = context; _wrapperList = new List (); // get handles on the relevant workspaces MetadataWorkspace csWorkspace = ((EntityConnection)context.Connection).GetMetadataWorkspace(); MetadataWorkspace ocWorkspace = context.MetadataWorkspace; // if no restricted type is given, we assume the entity set element type is exposed EntityType entityType = restrictedEntityType ?? entitySet.ElementType; _clrEntityType = EntityDataSourceUtil.GetClrType(ocWorkspace, entityType); // if no restricted type is given and the set is polymorphic, make the collection readonly if (null == restrictedEntityType && 1 < EntityDataSourceUtil.GetTypeAndSubtypesOf(entityType, csWorkspace.GetItemCollection(DataSpace.CSpace), true).Count()) { _isReadOnly = true; } // gather the properties Type componentType = EntityDataSourceUtil.GetClrType(context.MetadataWorkspace, entityType); ReadOnlyCollection columns = EntityDataSourceUtil.GetNamedColumns(csWorkspace, ocWorkspace, entitySet, entityType); List visiblePropertyDescriptors = new List (columns.Count); List propertyDescriptors = new List (columns.Count); foreach (EntityDataSourceColumn column in columns) { var descriptor = new EntityDataSourceWrapperPropertyDescriptor(this, column); propertyDescriptors.Add(descriptor); // if the descriptor does not have a dependent, it is exposed to the user if (!descriptor.Column.IsHidden) { visiblePropertyDescriptors.Add(descriptor); } } _visiblePropertyDescriptors = new PropertyDescriptorCollection(visiblePropertyDescriptors.ToArray(), true); AllPropertyDescriptors = propertyDescriptors.AsReadOnly(); } internal EntityDataSourceWrapper AddWrappedEntity(object entity) { EntityDataSourceWrapper wrapper = new EntityDataSourceWrapper(this, entity); this._wrapperList.Add(wrapper); return wrapper; } #region IEnumerable Implementation IEnumerator IEnumerable.GetEnumerator() { return this._wrapperList.GetEnumerator(); } #endregion IEnumerable Implementation #region ICollection Implementation public int Count { get { return this._wrapperList.Count; } } public void CopyTo(Array array, int index) { ((ICollection)this._wrapperList).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return null; } } #endregion #region ITypedList Implementation public PropertyDescriptorCollection GetItemProperties(PropertyDescriptor[] listAccessors) { if (null == listAccessors) { return this._visiblePropertyDescriptors ; } else { return null;// Implement this feature when we support traversing collections. } } string ITypedList.GetListName(PropertyDescriptor[] listAccessors) { return null; } #endregion #region Internal properties /// /// Gets CLR type or base type for entities exposed in this collection. /// internal Type ClrEntityType { get { return _clrEntityType; } } ////// Indicates whether this configuration supports modifications. /// internal bool IsReadOnly { get { return _isReadOnly; } } ////// Gets object context tracking the contents of this collection. /// internal ObjectContext Context { get { return _context; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Data; using System.Configuration; using System.Linq; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.HtmlControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Collections; using System.ComponentModel; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Mapping; using System.Data.Objects; using System.Reflection; using System.Diagnostics; using System.Data.Common; using System.Data.Objects.DataClasses; using System.Globalization; using System.Data.EntityClient; using System.Collections.ObjectModel; namespace System.Web.UI.WebControls { ////// Summary description for EntityDataSourceWrapperCollection /// internal class EntityDataSourceWrapperCollection : IEnumerable, ICollection, ITypedList { private readonly ObjectContext _context; private readonly List_wrapperList; /// /// Gets the property descriptors exposed to the user. /// private readonly PropertyDescriptorCollection _visiblePropertyDescriptors; ////// Gets all property descriptors. /// internal readonly ReadOnlyCollectionAllPropertyDescriptors; private readonly bool _isReadOnly; private readonly Type _clrEntityType; internal EntityDataSourceWrapperCollection(ObjectContext context, EntitySet entitySet, EntityType restrictedEntityType) { EntityDataSourceUtil.CheckArgumentNull(context, "context"); EntityDataSourceUtil.CheckArgumentNull(entitySet, "entitySet"); _context = context; _wrapperList = new List (); // get handles on the relevant workspaces MetadataWorkspace csWorkspace = ((EntityConnection)context.Connection).GetMetadataWorkspace(); MetadataWorkspace ocWorkspace = context.MetadataWorkspace; // if no restricted type is given, we assume the entity set element type is exposed EntityType entityType = restrictedEntityType ?? entitySet.ElementType; _clrEntityType = EntityDataSourceUtil.GetClrType(ocWorkspace, entityType); // if no restricted type is given and the set is polymorphic, make the collection readonly if (null == restrictedEntityType && 1 < EntityDataSourceUtil.GetTypeAndSubtypesOf(entityType, csWorkspace.GetItemCollection(DataSpace.CSpace), true).Count()) { _isReadOnly = true; } // gather the properties Type componentType = EntityDataSourceUtil.GetClrType(context.MetadataWorkspace, entityType); ReadOnlyCollection columns = EntityDataSourceUtil.GetNamedColumns(csWorkspace, ocWorkspace, entitySet, entityType); List visiblePropertyDescriptors = new List (columns.Count); List propertyDescriptors = new List (columns.Count); foreach (EntityDataSourceColumn column in columns) { var descriptor = new EntityDataSourceWrapperPropertyDescriptor(this, column); propertyDescriptors.Add(descriptor); // if the descriptor does not have a dependent, it is exposed to the user if (!descriptor.Column.IsHidden) { visiblePropertyDescriptors.Add(descriptor); } } _visiblePropertyDescriptors = new PropertyDescriptorCollection(visiblePropertyDescriptors.ToArray(), true); AllPropertyDescriptors = propertyDescriptors.AsReadOnly(); } internal EntityDataSourceWrapper AddWrappedEntity(object entity) { EntityDataSourceWrapper wrapper = new EntityDataSourceWrapper(this, entity); this._wrapperList.Add(wrapper); return wrapper; } #region IEnumerable Implementation IEnumerator IEnumerable.GetEnumerator() { return this._wrapperList.GetEnumerator(); } #endregion IEnumerable Implementation #region ICollection Implementation public int Count { get { return this._wrapperList.Count; } } public void CopyTo(Array array, int index) { ((ICollection)this._wrapperList).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return null; } } #endregion #region ITypedList Implementation public PropertyDescriptorCollection GetItemProperties(PropertyDescriptor[] listAccessors) { if (null == listAccessors) { return this._visiblePropertyDescriptors ; } else { return null;// Implement this feature when we support traversing collections. } } string ITypedList.GetListName(PropertyDescriptor[] listAccessors) { return null; } #endregion #region Internal properties /// /// Gets CLR type or base type for entities exposed in this collection. /// internal Type ClrEntityType { get { return _clrEntityType; } } ////// Indicates whether this configuration supports modifications. /// internal bool IsReadOnly { get { return _isReadOnly; } } ////// Gets object context tracking the contents of this collection. /// internal ObjectContext Context { get { return _context; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
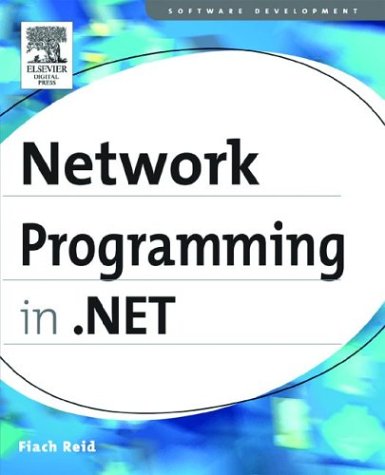
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ALinqExpressionVisitor.cs
- unitconverter.cs
- BidirectionalDictionary.cs
- DirectoryNotFoundException.cs
- ValueSerializer.cs
- ComAwareEventInfo.cs
- TextServicesLoader.cs
- WebPartAuthorizationEventArgs.cs
- NCryptNative.cs
- TransactionScope.cs
- RelatedCurrencyManager.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- CompilationPass2Task.cs
- InstanceKeyCompleteException.cs
- DesignerProperties.cs
- ArithmeticException.cs
- WindowsToolbar.cs
- ThreadExceptionDialog.cs
- WebChannelFactory.cs
- WorkflowCreationContext.cs
- UnitControl.cs
- SecurityDescriptor.cs
- GetTokenRequest.cs
- Size.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- Style.cs
- HostProtectionException.cs
- HttpContextServiceHost.cs
- HwndHostAutomationPeer.cs
- ToolStripLabel.cs
- SessionStateModule.cs
- DaylightTime.cs
- Scripts.cs
- PositiveTimeSpanValidatorAttribute.cs
- EarlyBoundInfo.cs
- DataSetViewSchema.cs
- WasEndpointConfigContainer.cs
- IntranetCredentialPolicy.cs
- HttpResponseBase.cs
- TableLayoutColumnStyleCollection.cs
- Figure.cs
- GlobalEventManager.cs
- DbConnectionFactory.cs
- TextServicesPropertyRanges.cs
- HtmlInputText.cs
- connectionpool.cs
- BuildProviderCollection.cs
- ValidationRuleCollection.cs
- AlignmentXValidation.cs
- IResourceProvider.cs
- ListBoxItemAutomationPeer.cs
- ScrollChrome.cs
- TextDecoration.cs
- Matrix.cs
- RewritingValidator.cs
- InvalidPropValue.cs
- HiddenFieldPageStatePersister.cs
- ObjectFullSpanRewriter.cs
- UnsafeNativeMethods.cs
- CodeStatementCollection.cs
- SubMenuStyleCollection.cs
- WsdlBuildProvider.cs
- TemplatedWizardStep.cs
- WindowsListViewSubItem.cs
- ValidatingReaderNodeData.cs
- DataGridTable.cs
- ConfigurationValue.cs
- UpdateProgress.cs
- DataGridTablesFactory.cs
- SqlExpressionNullability.cs
- DrawingImage.cs
- CodeBinaryOperatorExpression.cs
- Merger.cs
- ColorInterpolationModeValidation.cs
- WbmpConverter.cs
- WizardForm.cs
- TrustManagerPromptUI.cs
- UnaryQueryOperator.cs
- XLinq.cs
- SqlClientWrapperSmiStreamChars.cs
- HyperLinkStyle.cs
- DesignerCategoryAttribute.cs
- ClientScriptManager.cs
- JsonObjectDataContract.cs
- ToolStripSettings.cs
- FtpCachePolicyElement.cs
- Scanner.cs
- BinaryCommonClasses.cs
- NameSpaceEvent.cs
- Imaging.cs
- IOThreadScheduler.cs
- TokenBasedSetEnumerator.cs
- _UriSyntax.cs
- ResourceExpressionBuilder.cs
- _SSPISessionCache.cs
- ParameterBuilder.cs
- KoreanLunisolarCalendar.cs
- ReachFixedDocumentSerializer.cs
- _AutoWebProxyScriptHelper.cs
- PropertyPathWorker.cs