Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Abstractions / HttpResponseBase.cs / 1503810 / HttpResponseBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections; using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Text; using System.Web.Caching; using System.Web.Routing; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public abstract class HttpResponseBase { public virtual bool Buffer { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool BufferOutput { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual HttpCachePolicyBase Cache { get { throw new NotImplementedException(); } } public virtual string CacheControl { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual String Charset { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Encoding ContentEncoding { set { throw new NotImplementedException(); } get { throw new NotImplementedException(); } } public virtual string ContentType { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual HttpCookieCollection Cookies { get { throw new NotImplementedException(); } } public virtual int Expires { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual DateTime ExpiresAbsolute { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Stream Filter { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual NameValueCollection Headers { get { throw new NotImplementedException(); } } public virtual Encoding HeaderEncoding { set { throw new NotImplementedException(); } get { throw new NotImplementedException(); } } public virtual bool IsClientConnected { get { throw new NotImplementedException(); } } public virtual bool IsRequestBeingRedirected { get { throw new NotImplementedException(); } } public virtual TextWriter Output { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Stream OutputStream { get { throw new NotImplementedException(); } } public virtual String RedirectLocation { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual string Status { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual int StatusCode { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual String StatusDescription { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual int SubStatusCode { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool SuppressContent { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool TrySkipIisCustomErrors { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void AddCacheItemDependency(string cacheKey) { throw new NotImplementedException(); } public virtual void AddCacheItemDependencies(ArrayList cacheKeys) { throw new NotImplementedException(); } public virtual void AddCacheItemDependencies(string[] cacheKeys) { throw new NotImplementedException(); } public virtual void AddCacheDependency(params CacheDependency[] dependencies) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void AddFileDependency(String filename) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void AddFileDependencies(ArrayList filenames) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void AddFileDependencies(string[] filenames) { throw new NotImplementedException(); } public virtual void AddHeader(String name, String value) { throw new NotImplementedException(); } public virtual void AppendCookie(HttpCookie cookie) { throw new NotImplementedException(); } public virtual void AppendHeader(String name, String value) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void AppendToLog(String param) { throw new NotImplementedException(); } public virtual string ApplyAppPathModifier(string virtualPath) { throw new NotImplementedException(); } public virtual void BinaryWrite(byte[] buffer) { throw new NotImplementedException(); } public virtual void Clear() { throw new NotImplementedException(); } public virtual void ClearContent() { throw new NotImplementedException(); } public virtual void ClearHeaders() { throw new NotImplementedException(); } public virtual void Close() { throw new NotImplementedException(); } public virtual void DisableKernelCache() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "End", Justification = "Matches HttpResponse class")] public virtual void End() { throw new NotImplementedException(); } public virtual void Flush() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void Pics(String value) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void Redirect(String url) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void Redirect(String url, bool endResponse) { throw new NotImplementedException(); } public virtual void RedirectToRoute(object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoute(string routeName) { throw new NotImplementedException(); } public virtual void RedirectToRoute(RouteValueDictionary routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoute(string routeName, object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoute(string routeName, RouteValueDictionary routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(string routeName) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(RouteValueDictionary routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(string routeName, object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(string routeName, RouteValueDictionary routeValues) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void RedirectPermanent(String url) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void RedirectPermanent(String url, bool endResponse) { throw new NotImplementedException(); } public virtual void RemoveOutputCacheItem(string path) { throw new NotImplementedException(); } public virtual void RemoveOutputCacheItem(string path, string providerName) { throw new NotImplementedException(); } public virtual void SetCookie(HttpCookie cookie) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void TransmitFile(string filename) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void TransmitFile(string filename, long offset, long length) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void Write(char ch) { throw new NotImplementedException(); } public virtual void Write(char[] buffer, int index, int count) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "obj", Justification = "Matches HttpResponse class")] public virtual void Write(Object obj) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void Write(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(String filename) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(String filename, bool readIntoMemory) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(String filename, long offset, long size) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(IntPtr fileHandle, long offset, long size) { throw new NotImplementedException(); } public virtual void WriteSubstitution(HttpResponseSubstitutionCallback callback) { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections; using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Text; using System.Web.Caching; using System.Web.Routing; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public abstract class HttpResponseBase { public virtual bool Buffer { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool BufferOutput { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual HttpCachePolicyBase Cache { get { throw new NotImplementedException(); } } public virtual string CacheControl { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual String Charset { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Encoding ContentEncoding { set { throw new NotImplementedException(); } get { throw new NotImplementedException(); } } public virtual string ContentType { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual HttpCookieCollection Cookies { get { throw new NotImplementedException(); } } public virtual int Expires { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual DateTime ExpiresAbsolute { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Stream Filter { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual NameValueCollection Headers { get { throw new NotImplementedException(); } } public virtual Encoding HeaderEncoding { set { throw new NotImplementedException(); } get { throw new NotImplementedException(); } } public virtual bool IsClientConnected { get { throw new NotImplementedException(); } } public virtual bool IsRequestBeingRedirected { get { throw new NotImplementedException(); } } public virtual TextWriter Output { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Stream OutputStream { get { throw new NotImplementedException(); } } public virtual String RedirectLocation { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual string Status { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual int StatusCode { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual String StatusDescription { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual int SubStatusCode { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool SuppressContent { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool TrySkipIisCustomErrors { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void AddCacheItemDependency(string cacheKey) { throw new NotImplementedException(); } public virtual void AddCacheItemDependencies(ArrayList cacheKeys) { throw new NotImplementedException(); } public virtual void AddCacheItemDependencies(string[] cacheKeys) { throw new NotImplementedException(); } public virtual void AddCacheDependency(params CacheDependency[] dependencies) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void AddFileDependency(String filename) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void AddFileDependencies(ArrayList filenames) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void AddFileDependencies(string[] filenames) { throw new NotImplementedException(); } public virtual void AddHeader(String name, String value) { throw new NotImplementedException(); } public virtual void AppendCookie(HttpCookie cookie) { throw new NotImplementedException(); } public virtual void AppendHeader(String name, String value) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void AppendToLog(String param) { throw new NotImplementedException(); } public virtual string ApplyAppPathModifier(string virtualPath) { throw new NotImplementedException(); } public virtual void BinaryWrite(byte[] buffer) { throw new NotImplementedException(); } public virtual void Clear() { throw new NotImplementedException(); } public virtual void ClearContent() { throw new NotImplementedException(); } public virtual void ClearHeaders() { throw new NotImplementedException(); } public virtual void Close() { throw new NotImplementedException(); } public virtual void DisableKernelCache() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "End", Justification = "Matches HttpResponse class")] public virtual void End() { throw new NotImplementedException(); } public virtual void Flush() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void Pics(String value) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void Redirect(String url) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void Redirect(String url, bool endResponse) { throw new NotImplementedException(); } public virtual void RedirectToRoute(object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoute(string routeName) { throw new NotImplementedException(); } public virtual void RedirectToRoute(RouteValueDictionary routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoute(string routeName, object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoute(string routeName, RouteValueDictionary routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(string routeName) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(RouteValueDictionary routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(string routeName, object routeValues) { throw new NotImplementedException(); } public virtual void RedirectToRoutePermanent(string routeName, RouteValueDictionary routeValues) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void RedirectPermanent(String url) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "Matches HttpResponse class")] public virtual void RedirectPermanent(String url, bool endResponse) { throw new NotImplementedException(); } public virtual void RemoveOutputCacheItem(string path) { throw new NotImplementedException(); } public virtual void RemoveOutputCacheItem(string path, string providerName) { throw new NotImplementedException(); } public virtual void SetCookie(HttpCookie cookie) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void TransmitFile(string filename) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void TransmitFile(string filename, long offset, long length) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void Write(char ch) { throw new NotImplementedException(); } public virtual void Write(char[] buffer, int index, int count) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:IdentifiersShouldNotContainTypeNames", MessageId = "obj", Justification = "Matches HttpResponse class")] public virtual void Write(Object obj) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpResponse class")] public virtual void Write(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(String filename) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(String filename, bool readIntoMemory) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(String filename, long offset, long size) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", Justification = "Matches HttpResponse class")] public virtual void WriteFile(IntPtr fileHandle, long offset, long size) { throw new NotImplementedException(); } public virtual void WriteSubstitution(HttpResponseSubstitutionCallback callback) { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
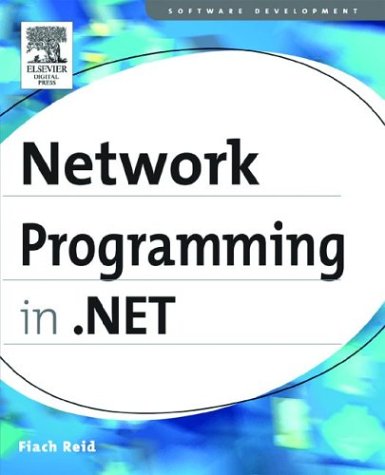
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationLocationCollection.cs
- TrackingLocationCollection.cs
- ErrorStyle.cs
- PageParser.cs
- SmiGettersStream.cs
- StorageAssociationSetMapping.cs
- DataGridRowHeaderAutomationPeer.cs
- PropertyInformationCollection.cs
- ActionNotSupportedException.cs
- SelectionHighlightInfo.cs
- PropertyIDSet.cs
- XmlSchemaFacet.cs
- OneWayBindingElement.cs
- ByteAnimationBase.cs
- GridViewRowCollection.cs
- SchemaImporterExtension.cs
- FormatConvertedBitmap.cs
- SystemWebCachingSectionGroup.cs
- PrePostDescendentsWalker.cs
- MiniAssembly.cs
- PersistenceContextEnlistment.cs
- Timer.cs
- XPathArrayIterator.cs
- SqlDataSourceQueryEditorForm.cs
- BitmapImage.cs
- NodeLabelEditEvent.cs
- UnsafeNetInfoNativeMethods.cs
- SchemaMerger.cs
- ControlBuilderAttribute.cs
- CounterSampleCalculator.cs
- SchemaImporterExtension.cs
- Win32MouseDevice.cs
- RefreshEventArgs.cs
- FlatButtonAppearance.cs
- cookiecollection.cs
- ListViewCommandEventArgs.cs
- InternalConfigConfigurationFactory.cs
- LocalizableResourceBuilder.cs
- UnitySerializationHolder.cs
- DataTemplate.cs
- XslTransform.cs
- FragmentQueryProcessor.cs
- PropertyDescriptorCollection.cs
- ParenExpr.cs
- ModuleBuilder.cs
- QilInvokeLateBound.cs
- ConnectionConsumerAttribute.cs
- KeyValueConfigurationCollection.cs
- XmlSchemaDatatype.cs
- OracleConnectionFactory.cs
- NumberAction.cs
- ParserHooks.cs
- Animatable.cs
- DrawingContext.cs
- DataSourceCache.cs
- KeyEventArgs.cs
- ContainerAction.cs
- DefinitionBase.cs
- ContractUtils.cs
- ModelVisual3D.cs
- ResolveResponse.cs
- Win32PrintDialog.cs
- GlobalProxySelection.cs
- PackagePartCollection.cs
- LoginName.cs
- ButtonChrome.cs
- ActivatableWorkflowsQueryResult.cs
- SQLDoubleStorage.cs
- PlanCompiler.cs
- LinkDescriptor.cs
- PageContent.cs
- ProfileInfo.cs
- VerificationAttribute.cs
- ObjectQueryExecutionPlan.cs
- SchemaEntity.cs
- TypeDelegator.cs
- HostedTransportConfigurationManager.cs
- BaseAppDomainProtocolHandler.cs
- CombinedGeometry.cs
- SamlAdvice.cs
- TreeViewHitTestInfo.cs
- ConnectionManagementElementCollection.cs
- WebGetAttribute.cs
- ObjectQuery_EntitySqlExtensions.cs
- Parser.cs
- AutomationEvent.cs
- TypeForwardedToAttribute.cs
- SkinBuilder.cs
- XmlQualifiedName.cs
- CompareInfo.cs
- ModuleElement.cs
- ValidatorCompatibilityHelper.cs
- ReadingWritingEntityEventArgs.cs
- WizardSideBarListControlItem.cs
- CompoundFileReference.cs
- SmiEventStream.cs
- HostUtils.cs
- DockPattern.cs
- Stacktrace.cs
- WebScriptMetadataMessage.cs