Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / cookiecollection.cs / 1 / cookiecollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.Globalization; using System.Runtime.Serialization; // // CookieCollection // // A list of cookies maintained in Sorted order. Only one cookie with matching Name/Domain/Path // ////// [Serializable] public class CookieCollection : ICollection { // fields internal enum Stamp { Check = 0, Set = 1, SetToUnused = 2, SetToMaxUsed = 3, } internal int m_version; ArrayList m_list = new ArrayList(); DateTime m_TimeStamp = DateTime.MinValue; bool m_has_other_versions; [OptionalField] bool m_IsReadOnly; // constructors ///[To be supplied.] ////// public CookieCollection() { m_IsReadOnly = true; } internal CookieCollection(bool IsReadOnly) { m_IsReadOnly = IsReadOnly; } // properties ///[To be supplied.] ////// public bool IsReadOnly { get { return m_IsReadOnly; } } ///[To be supplied.] ////// public Cookie this[int index] { get { if (index < 0 || index >= m_list.Count) { throw new ArgumentOutOfRangeException("index"); } return (Cookie)(m_list[index]); } } ///[To be supplied.] ////// public Cookie this[string name] { get { foreach (Cookie c in m_list) { if (string.Compare(c.Name, name, StringComparison.OrdinalIgnoreCase ) == 0) { return c; } } return null; } } // methods ///[To be supplied.] ////// public void Add(Cookie cookie) { if (cookie == null) { throw new ArgumentNullException("cookie"); } ++m_version; int idx = IndexOf(cookie); if (idx == -1) { m_list.Add(cookie); } else { m_list[idx] = cookie; } } ///[To be supplied.] ////// public void Add(CookieCollection cookies) { if (cookies == null) { throw new ArgumentNullException("cookies"); } //if (cookies == this) { // cookies = new CookieCollection(cookies); //} foreach (Cookie cookie in cookies) { Add(cookie); } } // ICollection interface ///[To be supplied.] ////// public int Count { get { return m_list.Count; } } ///[To be supplied.] ////// public bool IsSynchronized { get { return false; } } ///[To be supplied.] ////// public object SyncRoot { get { return this; } } ///[To be supplied.] ////// public void CopyTo(Array array, int index) { m_list.CopyTo(array, index); } ///[To be supplied.] ////// public void CopyTo(Cookie[] array, int index) { m_list.CopyTo(array, index); } internal DateTime TimeStamp(Stamp how) { switch (how) { case Stamp.Set: m_TimeStamp = DateTime.Now; break; case Stamp.SetToMaxUsed: m_TimeStamp = DateTime.MaxValue; break; case Stamp.SetToUnused: m_TimeStamp = DateTime.MinValue; break; case Stamp.Check: default: break; } return m_TimeStamp; } // This is for internal cookie container usage // For others not that m_has_other_versions gets changed ONLY in InternalAdd internal bool IsOtherVersionSeen{ get { return m_has_other_versions; } } // If isStrict == false, assumes that incoming cookie is unique // If isStrict == true, replace the cookie if found same with newest Variant // returns 1 if added, 0 if replaced or rejected internal int InternalAdd(Cookie cookie, bool isStrict) { int ret = 1; if (isStrict) { IComparer comp = Cookie.GetComparer(); int idx = 0; foreach (Cookie c in m_list) { if (comp.Compare(cookie, c) == 0) { ret = 0; //will replace or reject //Cookie2 spec requires that new Variant cookie overwrite the old one if (c.Variant <= cookie.Variant) { m_list[idx] = cookie; } break; } ++idx; } if (idx == m_list.Count) { m_list.Add(cookie); } } else { m_list.Add(cookie); } if (cookie.Version != Cookie.MaxSupportedVersion) { m_has_other_versions = true; } return ret; } internal int IndexOf(Cookie cookie) { IComparer comp = Cookie.GetComparer(); int idx = 0; foreach (Cookie c in m_list) { if (comp.Compare(cookie, c) == 0) { return idx; } ++idx; } return -1; } internal void RemoveAt(int idx) { m_list.RemoveAt(idx); } // IEnumerable interface ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return new CookieCollectionEnumerator(this); } #if DEBUG ///[To be supplied.] ////// internal void Dump() { GlobalLog.Print("CookieCollection:"); foreach (Cookie cookie in this) { cookie.Dump(); } } #endif //Not used anymore delete ? private class CookieCollectionEnumerator : IEnumerator { CookieCollection m_cookies; int m_count; int m_index = -1; int m_version; internal CookieCollectionEnumerator(CookieCollection cookies) { m_cookies = cookies; m_count = cookies.Count; m_version = cookies.m_version; } // IEnumerator interface object IEnumerator.Current { get { if (m_index < 0 || m_index >= m_count) { throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumOpCantHappen)); } if (m_version != m_cookies.m_version) { throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumFailedVersion)); } return m_cookies[m_index]; } } bool IEnumerator.MoveNext() { if (m_version != m_cookies.m_version) { throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumFailedVersion)); } if (++m_index < m_count) { return true; } m_index = m_count; return false; } void IEnumerator.Reset() { m_index = -1; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Collections; using System.Globalization; using System.Runtime.Serialization; // // CookieCollection // // A list of cookies maintained in Sorted order. Only one cookie with matching Name/Domain/Path // ////// [Serializable] public class CookieCollection : ICollection { // fields internal enum Stamp { Check = 0, Set = 1, SetToUnused = 2, SetToMaxUsed = 3, } internal int m_version; ArrayList m_list = new ArrayList(); DateTime m_TimeStamp = DateTime.MinValue; bool m_has_other_versions; [OptionalField] bool m_IsReadOnly; // constructors ///[To be supplied.] ////// public CookieCollection() { m_IsReadOnly = true; } internal CookieCollection(bool IsReadOnly) { m_IsReadOnly = IsReadOnly; } // properties ///[To be supplied.] ////// public bool IsReadOnly { get { return m_IsReadOnly; } } ///[To be supplied.] ////// public Cookie this[int index] { get { if (index < 0 || index >= m_list.Count) { throw new ArgumentOutOfRangeException("index"); } return (Cookie)(m_list[index]); } } ///[To be supplied.] ////// public Cookie this[string name] { get { foreach (Cookie c in m_list) { if (string.Compare(c.Name, name, StringComparison.OrdinalIgnoreCase ) == 0) { return c; } } return null; } } // methods ///[To be supplied.] ////// public void Add(Cookie cookie) { if (cookie == null) { throw new ArgumentNullException("cookie"); } ++m_version; int idx = IndexOf(cookie); if (idx == -1) { m_list.Add(cookie); } else { m_list[idx] = cookie; } } ///[To be supplied.] ////// public void Add(CookieCollection cookies) { if (cookies == null) { throw new ArgumentNullException("cookies"); } //if (cookies == this) { // cookies = new CookieCollection(cookies); //} foreach (Cookie cookie in cookies) { Add(cookie); } } // ICollection interface ///[To be supplied.] ////// public int Count { get { return m_list.Count; } } ///[To be supplied.] ////// public bool IsSynchronized { get { return false; } } ///[To be supplied.] ////// public object SyncRoot { get { return this; } } ///[To be supplied.] ////// public void CopyTo(Array array, int index) { m_list.CopyTo(array, index); } ///[To be supplied.] ////// public void CopyTo(Cookie[] array, int index) { m_list.CopyTo(array, index); } internal DateTime TimeStamp(Stamp how) { switch (how) { case Stamp.Set: m_TimeStamp = DateTime.Now; break; case Stamp.SetToMaxUsed: m_TimeStamp = DateTime.MaxValue; break; case Stamp.SetToUnused: m_TimeStamp = DateTime.MinValue; break; case Stamp.Check: default: break; } return m_TimeStamp; } // This is for internal cookie container usage // For others not that m_has_other_versions gets changed ONLY in InternalAdd internal bool IsOtherVersionSeen{ get { return m_has_other_versions; } } // If isStrict == false, assumes that incoming cookie is unique // If isStrict == true, replace the cookie if found same with newest Variant // returns 1 if added, 0 if replaced or rejected internal int InternalAdd(Cookie cookie, bool isStrict) { int ret = 1; if (isStrict) { IComparer comp = Cookie.GetComparer(); int idx = 0; foreach (Cookie c in m_list) { if (comp.Compare(cookie, c) == 0) { ret = 0; //will replace or reject //Cookie2 spec requires that new Variant cookie overwrite the old one if (c.Variant <= cookie.Variant) { m_list[idx] = cookie; } break; } ++idx; } if (idx == m_list.Count) { m_list.Add(cookie); } } else { m_list.Add(cookie); } if (cookie.Version != Cookie.MaxSupportedVersion) { m_has_other_versions = true; } return ret; } internal int IndexOf(Cookie cookie) { IComparer comp = Cookie.GetComparer(); int idx = 0; foreach (Cookie c in m_list) { if (comp.Compare(cookie, c) == 0) { return idx; } ++idx; } return -1; } internal void RemoveAt(int idx) { m_list.RemoveAt(idx); } // IEnumerable interface ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return new CookieCollectionEnumerator(this); } #if DEBUG ///[To be supplied.] ////// internal void Dump() { GlobalLog.Print("CookieCollection:"); foreach (Cookie cookie in this) { cookie.Dump(); } } #endif //Not used anymore delete ? private class CookieCollectionEnumerator : IEnumerator { CookieCollection m_cookies; int m_count; int m_index = -1; int m_version; internal CookieCollectionEnumerator(CookieCollection cookies) { m_cookies = cookies; m_count = cookies.Count; m_version = cookies.m_version; } // IEnumerator interface object IEnumerator.Current { get { if (m_index < 0 || m_index >= m_count) { throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumOpCantHappen)); } if (m_version != m_cookies.m_version) { throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumFailedVersion)); } return m_cookies[m_index]; } } bool IEnumerator.MoveNext() { if (m_version != m_cookies.m_version) { throw new InvalidOperationException(SR.GetString(SR.InvalidOperation_EnumFailedVersion)); } if (++m_index < m_count) { return true; } m_index = m_count; return false; } void IEnumerator.Reset() { m_index = -1; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
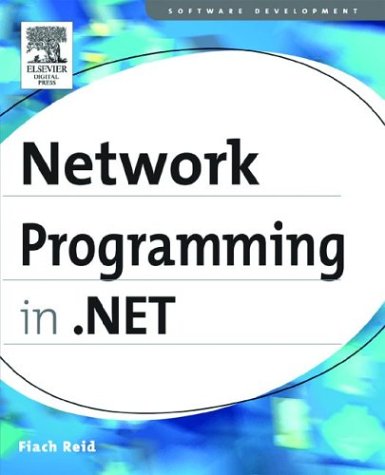
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextSelectionHelper.cs
- AbstractDataSvcMapFileLoader.cs
- AssemblyResolver.cs
- DashStyle.cs
- XPathExpr.cs
- RectAnimation.cs
- UnsafeNativeMethodsMilCoreApi.cs
- WebPartVerb.cs
- RoutedUICommand.cs
- SqlTopReducer.cs
- CompositeControl.cs
- BufferedGraphicsContext.cs
- FixedLineResult.cs
- Int64KeyFrameCollection.cs
- Rijndael.cs
- updatecommandorderer.cs
- ServiceBusyException.cs
- FileSystemWatcher.cs
- MatrixTransform.cs
- StreamWriter.cs
- ListenerElementsCollection.cs
- FreezableOperations.cs
- FileRecordSequence.cs
- DataTemplate.cs
- ComponentManagerBroker.cs
- Span.cs
- ToolbarAUtomationPeer.cs
- TextEmbeddedObject.cs
- Hyperlink.cs
- NumericPagerField.cs
- TabControlAutomationPeer.cs
- SqlServices.cs
- JournalEntryStack.cs
- SortExpressionBuilder.cs
- _OverlappedAsyncResult.cs
- Section.cs
- HtmlTable.cs
- GraphicsContainer.cs
- HMAC.cs
- _ConnectOverlappedAsyncResult.cs
- GridViewUpdatedEventArgs.cs
- StreamSecurityUpgradeAcceptorBase.cs
- COSERVERINFO.cs
- AttributeQuery.cs
- EntityViewGenerationAttribute.cs
- ListContractAdapter.cs
- SetterTriggerConditionValueConverter.cs
- ChangePasswordDesigner.cs
- InfoCardClaim.cs
- EntityRecordInfo.cs
- MsmqReceiveHelper.cs
- Int16Animation.cs
- clipboard.cs
- TextEditorLists.cs
- CryptographicAttribute.cs
- PassportPrincipal.cs
- PolyQuadraticBezierSegment.cs
- InfiniteTimeSpanConverter.cs
- XmlParser.cs
- XmlSerializer.cs
- XsdBuildProvider.cs
- MarkerProperties.cs
- HtmlDocument.cs
- Point.cs
- ItemAutomationPeer.cs
- TextParagraphCache.cs
- XmlSchemaGroup.cs
- AliasedSlot.cs
- XmlDocumentViewSchema.cs
- OperationAbortedException.cs
- rsa.cs
- GACIdentityPermission.cs
- SQLByte.cs
- SelectionList.cs
- MetadataPropertyvalue.cs
- IntPtr.cs
- InputBinder.cs
- ToolStripLocationCancelEventArgs.cs
- XmlUtf8RawTextWriter.cs
- FontStretchConverter.cs
- KeyEventArgs.cs
- SqlCrossApplyToCrossJoin.cs
- RectAnimationUsingKeyFrames.cs
- QilReference.cs
- UpdateTranslator.cs
- HashJoinQueryOperatorEnumerator.cs
- QilInvokeEarlyBound.cs
- IncrementalHitTester.cs
- HelpKeywordAttribute.cs
- AddInIpcChannel.cs
- Filter.cs
- WeakReferenceEnumerator.cs
- DependencyPropertyHelper.cs
- SmiRequestExecutor.cs
- GcHandle.cs
- SecurityDocument.cs
- Operators.cs
- ListControl.cs
- MethodAccessException.cs
- ContextItem.cs