Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / ListenerElementsCollection.cs / 1 / ListenerElementsCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Configuration; using System; using System.Reflection; using System.Globalization; using System.Xml; using System.Collections.Specialized; using System.Collections; using System.Security; using System.Security.Permissions; namespace System.Diagnostics { [ConfigurationCollection(typeof(ListenerElement))] internal class ListenerElementsCollection : ConfigurationElementCollection { new public ListenerElement this[string name] { get { return (ListenerElement) BaseGet(name); } } public override ConfigurationElementCollectionType CollectionType { get { return ConfigurationElementCollectionType.AddRemoveClearMap; } } protected override ConfigurationElement CreateNewElement() { return new ListenerElement(true); } protected override Object GetElementKey(ConfigurationElement element) { return ((ListenerElement) element).Name; } public TraceListenerCollection GetRuntimeObject() { TraceListenerCollection listeners = new TraceListenerCollection(); bool _isDemanded = false; foreach(ListenerElement element in this) { // At some point, we need to pull out adding/removing the 'default' DefaultTraceListener // code from here in favor of adding/not-adding after we load the config (in TraceSource // and in static Trace) if (!_isDemanded && !element._isAddedByDefault) { // Do a full damand; This will disable partially trusted code from hooking up listeners new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); _isDemanded = true; } listeners.Add(element.GetRuntimeObject()); } return listeners; } protected override void InitializeDefault() { InitializeDefaultInternal(); } internal void InitializeDefaultInternal() { ListenerElement defaultListener = new ListenerElement(false); defaultListener.Name = "Default"; defaultListener.TypeName = typeof(DefaultTraceListener).FullName; defaultListener._isAddedByDefault = true; this.BaseAdd(defaultListener); } protected override void BaseAdd(ConfigurationElement element) { ListenerElement listenerElement = element as ListenerElement; Debug.Assert((listenerElement != null), "adding elements other than ListenerElement to ListenerElementsCollection?"); if (listenerElement.Name.Equals("Default") && listenerElement.TypeName.Equals(typeof(DefaultTraceListener).FullName)) BaseAdd(listenerElement, false); else BaseAdd(listenerElement, ThrowOnDuplicate); } } // This is the collection used by the sharedListener section. It is only slightly different from ListenerElementsCollection. // The differences are that it does not allow remove and clear, and that the ListenerElements it creates do not allow // references. [ConfigurationCollection(typeof(ListenerElement), AddItemName = "add", CollectionType = ConfigurationElementCollectionType.BasicMap)] internal class SharedListenerElementsCollection : ListenerElementsCollection { public override ConfigurationElementCollectionType CollectionType { get { return ConfigurationElementCollectionType.BasicMap; } } protected override ConfigurationElement CreateNewElement() { return new ListenerElement(false); } protected override string ElementName { get { return "add"; } } } internal class ListenerElement : TypedElement { private static readonly ConfigurationProperty _propFilter = new ConfigurationProperty("filter", typeof(FilterElement), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propOutputOpts = new ConfigurationProperty("traceOutputOptions", typeof(TraceOptions), TraceOptions.None, ConfigurationPropertyOptions.None); private ConfigurationProperty _propListenerTypeName; private bool _allowReferences; private Hashtable _attributes; internal bool _isAddedByDefault; static ListenerElement() { } public ListenerElement(bool allowReferences) : base(typeof(TraceListener)) { _allowReferences = allowReferences; ConfigurationPropertyOptions flags = ConfigurationPropertyOptions.None; if (!_allowReferences) flags |= ConfigurationPropertyOptions.IsRequired; _propListenerTypeName = new ConfigurationProperty("type", typeof(string), null, flags); _properties.Remove("type"); _properties.Add(_propListenerTypeName); _properties.Add(_propFilter); _properties.Add(_propName); _properties.Add(_propOutputOpts); } public Hashtable Attributes { get { if (_attributes == null) _attributes = new Hashtable(StringComparer.OrdinalIgnoreCase); return _attributes; } } [ConfigurationProperty("filter")] public FilterElement Filter { get { return (FilterElement) this[_propFilter]; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true)] public string Name { get { return (string) this[_propName]; } set { this[_propName] = value; } } [ConfigurationProperty("traceOutputOptions", DefaultValue = (TraceOptions) TraceOptions.None)] public TraceOptions TraceOutputOptions { get { return (TraceOptions) this[_propOutputOpts]; } // This is useful when the OM becomes public. In the meantime, this can be utilized via reflection set { this[_propOutputOpts] = value; } } [ConfigurationProperty("type")] public override string TypeName { get { return (string) this[_propListenerTypeName]; } set { this[_propListenerTypeName] = value; } } public override bool Equals(object compareTo) { if (this.Name.Equals("Default") && this.TypeName.Equals(typeof(DefaultTraceListener).FullName)) { // This is a workaround to treat all DefaultTraceListener named 'Default' the same. // This is needed for the Config.Save to work properly as otherwise config base layers // above us would run into duplicate 'Default' listener element and perceive it as // error. ListenerElement compareToElem = compareTo as ListenerElement; return (compareToElem != null) && compareToElem.Name.Equals("Default") && compareToElem.TypeName.Equals(typeof(DefaultTraceListener).FullName); } else return base.Equals(compareTo); } public override int GetHashCode() { return base.GetHashCode(); } public TraceListener GetRuntimeObject() { if (_runtimeObject != null) return (TraceListener) _runtimeObject; try { string className = TypeName; if (String.IsNullOrEmpty(className)) { // Look it up in SharedListeners Debug.Assert(_allowReferences, "_allowReferences must be true if type name is null"); if (_attributes != null || ElementInformation.Properties[_propFilter.Name].ValueOrigin == PropertyValueOrigin.SetHere || TraceOutputOptions != TraceOptions.None || !String.IsNullOrEmpty(InitData)) throw new ConfigurationErrorsException(SR.GetString(SR.Reference_listener_cant_have_properties, Name)); if (DiagnosticsConfiguration.SharedListeners == null) throw new ConfigurationErrorsException(SR.GetString(SR.Reference_to_nonexistent_listener, Name)); ListenerElement sharedListener = DiagnosticsConfiguration.SharedListeners[Name]; if (sharedListener == null) throw new ConfigurationErrorsException(SR.GetString(SR.Reference_to_nonexistent_listener, Name)); else { _runtimeObject = sharedListener.GetRuntimeObject(); return (TraceListener) _runtimeObject; } } else { // create a new one TraceListener newListener = (TraceListener) BaseGetRuntimeObject(); newListener.initializeData = InitData; newListener.Name = Name; newListener.SetAttributes(Attributes); newListener.TraceOutputOptions = TraceOutputOptions; if ((Filter != null) && (Filter.TypeName != null) && (Filter.TypeName.Length != 0)) newListener.Filter = Filter.GetRuntimeObject(); _runtimeObject = newListener; return newListener; } } catch (ArgumentException e) { throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_create_listener, Name), e); } } protected override bool OnDeserializeUnrecognizedAttribute(String name, String value) { ConfigurationProperty _propDynamic = new ConfigurationProperty(name, typeof(string), value); _properties.Add(_propDynamic); base[_propDynamic] = value; // Add them to the property bag Attributes.Add(name, value); return true; } internal void ResetProperties() { // blow away any UnrecognizedAttributes that we have deserialized earlier if (_attributes != null) { _attributes.Clear(); _properties.Clear(); _properties.Add(_propListenerTypeName); _properties.Add(_propFilter); _properties.Add(_propName); _properties.Add(_propOutputOpts); } } internal TraceListener RefreshRuntimeObject(TraceListener listener) { _runtimeObject = null; try { string className = TypeName; if (String.IsNullOrEmpty(className)) { // Look it up in SharedListeners and ask the sharedListener to refresh. Debug.Assert(_allowReferences, "_allowReferences must be true if type name is null"); if (_attributes != null || ElementInformation.Properties[_propFilter.Name].ValueOrigin == PropertyValueOrigin.SetHere || TraceOutputOptions != TraceOptions.None || !String.IsNullOrEmpty(InitData)) throw new ConfigurationErrorsException(SR.GetString(SR.Reference_listener_cant_have_properties, Name)); if (DiagnosticsConfiguration.SharedListeners == null) throw new ConfigurationErrorsException(SR.GetString(SR.Reference_to_nonexistent_listener, Name)); ListenerElement sharedListener = DiagnosticsConfiguration.SharedListeners[Name]; if (sharedListener == null) throw new ConfigurationErrorsException(SR.GetString(SR.Reference_to_nonexistent_listener, Name)); else { _runtimeObject = sharedListener.RefreshRuntimeObject(listener); return (TraceListener) _runtimeObject; } } else { // We're the element with the type and initializeData info. First see if those two are the same as they were. // If not, create a whole new object, otherwise, just update the other properties. if (Type.GetType(className) != listener.GetType() || InitData != listener.initializeData) { // type or initdata changed return GetRuntimeObject(); } else { listener.SetAttributes(Attributes); listener.TraceOutputOptions = TraceOutputOptions; if (ElementInformation.Properties[_propFilter.Name].ValueOrigin == PropertyValueOrigin.SetHere) listener.Filter = Filter.RefreshRuntimeObject(listener.Filter); else listener.Filter = null; _runtimeObject = listener; return listener; } } } catch (ArgumentException e) { throw new ConfigurationErrorsException(SR.GetString(SR.Could_not_create_listener, Name), e); } } } }
Link Menu
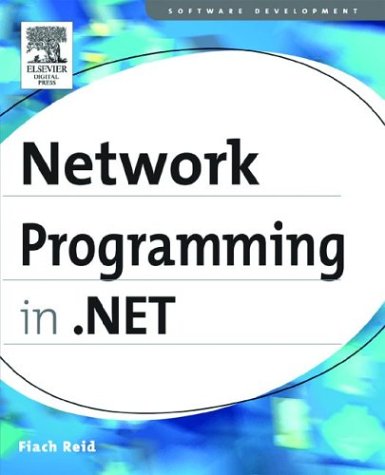
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Utils.cs
- EmissiveMaterial.cs
- StrokeSerializer.cs
- FormsAuthenticationCredentials.cs
- WebPartEditorCancelVerb.cs
- SplitterDesigner.cs
- XmlQueryRuntime.cs
- InlinedLocationReference.cs
- AlternateView.cs
- ToolStripItemEventArgs.cs
- RequestQueryProcessor.cs
- WebPartZone.cs
- DocumentOrderComparer.cs
- ActivationArguments.cs
- Operand.cs
- RepeaterItem.cs
- DataSourceProvider.cs
- ScriptControlDescriptor.cs
- OutputCacheSettings.cs
- FragmentNavigationEventArgs.cs
- TextEditorParagraphs.cs
- ListBindingHelper.cs
- PropertyEmitter.cs
- StringValidator.cs
- DataGridTextColumn.cs
- SelectorItemAutomationPeer.cs
- SID.cs
- WsatServiceCertificate.cs
- Missing.cs
- DispatcherObject.cs
- XmlHierarchicalEnumerable.cs
- XamlDebuggerXmlReader.cs
- XmlWrappingReader.cs
- XPathArrayIterator.cs
- InternalBufferManager.cs
- Utils.cs
- EntityViewContainer.cs
- TextRenderingModeValidation.cs
- InvalidProgramException.cs
- SchemaMerger.cs
- RegexCompilationInfo.cs
- ResourceExpressionBuilder.cs
- XmlFormatWriterGenerator.cs
- QilCloneVisitor.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- StorageAssociationSetMapping.cs
- ObjectViewListener.cs
- XmlSchemaSimpleContentRestriction.cs
- BookmarkScope.cs
- MessageEnumerator.cs
- UserControl.cs
- WebPartAuthorizationEventArgs.cs
- RegexRunner.cs
- GroupItemAutomationPeer.cs
- Image.cs
- MultipleViewProviderWrapper.cs
- ToolboxItemFilterAttribute.cs
- ViewStateModeByIdAttribute.cs
- List.cs
- TrueReadOnlyCollection.cs
- sitestring.cs
- TextHidden.cs
- ArgumentValidation.cs
- XmlNamespaceMapping.cs
- Preprocessor.cs
- ConstantExpression.cs
- TemplateKey.cs
- AnnotationComponentManager.cs
- IssuanceLicense.cs
- ItemCollection.cs
- TdsParameterSetter.cs
- UnicodeEncoding.cs
- odbcmetadatacolumnnames.cs
- ProjectionPlan.cs
- SchemaSetCompiler.cs
- ProtocolsConfigurationEntry.cs
- TagElement.cs
- HttpHandlersSection.cs
- BaseTemplateBuildProvider.cs
- EdmConstants.cs
- OdbcHandle.cs
- OracleNumber.cs
- MetadataReference.cs
- ImageMapEventArgs.cs
- ICspAsymmetricAlgorithm.cs
- WebPartZone.cs
- WebPartsSection.cs
- FolderBrowserDialog.cs
- Int32Rect.cs
- SyndicationDeserializer.cs
- EventArgs.cs
- Header.cs
- SafeNativeMethods.cs
- XsdDateTime.cs
- TextDecorationCollection.cs
- DataServiceContext.cs
- ConnectionPointCookie.cs
- TwoPhaseCommitProxy.cs
- ToolZone.cs
- ListView.cs