Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StorageAssociationSetMapping.cs / 1305376 / StorageAssociationSetMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Linq; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Represents the Mapping metadata for an AssociationSet in CS space. /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityTypeMapping /// --TableMappingFragment /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// This class represents the metadata for the AssociationSetMapping elements in the /// above example. And it is possible to access the AssociationTypeMap underneath it. /// There will be only one TypeMap under AssociationSetMap. /// internal class StorageAssociationSetMapping : StorageSetMapping { #region Constructors ////// Construct a new AssociationSetMapping object /// /// Represents the Association Set Metadata object. Will /// change this to Extent instead of MemberMetadata. /// The entityContainerMapping mapping that contains this Set mapping internal StorageAssociationSetMapping(AssociationSet extent, StorageEntityContainerMapping entityContainerMapping) : base(extent, entityContainerMapping) { } #endregion #region Fields private StorageAssociationSetFunctionMapping m_functionMapping; #endregion #region Properties ////////// The RealtionshipSet Metadata object for which the mapping is represented. ///// //internal AssociationSet AssociationSet { // get { // return this.Set as AssociationSet; // } //} ////// Gets or sets function mapping information for this association set. May be null. /// internal StorageAssociationSetFunctionMapping FunctionMapping { get { return m_functionMapping; } set { m_functionMapping = value; } } internal EntitySetBase StoreEntitySet { get { if ((this.TypeMappings.Count != 0) && (this.TypeMappings.First().MappingFragments.Count != 0)) { return this.TypeMappings.First().MappingFragments.First().TableSet; } return null; } } #endregion #region Methods ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("AssociationSetMapping"); sb.Append(" "); sb.Append("Name:"); sb.Append(this.Set.Name); if (this.QueryView != null) { sb.Append(" "); sb.Append("Query View:"); sb.Append(this.QueryView); } Console.WriteLine(sb.ToString()); foreach (StorageTypeMapping typeMapping in TypeMappings) { typeMapping.Print(index + 5); } if(m_functionMapping != null) { m_functionMapping.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
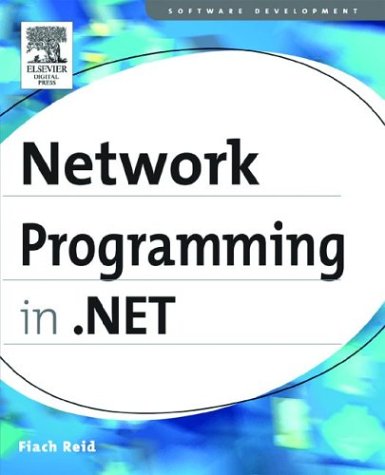
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RC2CryptoServiceProvider.cs
- CacheForPrimitiveTypes.cs
- NavigationCommands.cs
- TypeGeneratedEventArgs.cs
- PasswordTextContainer.cs
- InvalidFilterCriteriaException.cs
- Int16.cs
- NotifyParentPropertyAttribute.cs
- SymbolMethod.cs
- Stream.cs
- InputLanguage.cs
- TextStore.cs
- jithelpers.cs
- FastEncoder.cs
- IHttpResponseInternal.cs
- SQLGuidStorage.cs
- WinHttpWebProxyFinder.cs
- LocalBuilder.cs
- FileChangesMonitor.cs
- FormsAuthenticationUserCollection.cs
- AttachInfo.cs
- AdCreatedEventArgs.cs
- FigureParaClient.cs
- Soap.cs
- ZipIOModeEnforcingStream.cs
- wgx_sdk_version.cs
- Menu.cs
- MessageBox.cs
- ApplicationManager.cs
- GraphicsContainer.cs
- PageBuildProvider.cs
- HandledEventArgs.cs
- XPathNavigatorReader.cs
- AtomServiceDocumentSerializer.cs
- ButtonField.cs
- InlineCollection.cs
- DataGrid.cs
- AutomationElementCollection.cs
- Compiler.cs
- SystemDiagnosticsSection.cs
- Token.cs
- PathTooLongException.cs
- DbException.cs
- UrlMapping.cs
- DbModificationClause.cs
- InnerItemCollectionView.cs
- Int32Animation.cs
- ServiceMemoryGates.cs
- DPCustomTypeDescriptor.cs
- RuntimeArgumentHandle.cs
- FormsAuthenticationUser.cs
- TextOptionsInternal.cs
- RuleSettingsCollection.cs
- CopyOnWriteList.cs
- HeaderedItemsControl.cs
- TextDecorations.cs
- UriParserTemplates.cs
- Int16AnimationUsingKeyFrames.cs
- CssTextWriter.cs
- HtmlLink.cs
- Action.cs
- WebPartAuthorizationEventArgs.cs
- ReadWriteControlDesigner.cs
- SystemColors.cs
- PtsCache.cs
- AccessText.cs
- DefaultAsyncDataDispatcher.cs
- ContextMenuAutomationPeer.cs
- autovalidator.cs
- OpCellTreeNode.cs
- HandlerBase.cs
- PenContext.cs
- CacheForPrimitiveTypes.cs
- DataSourceSelectArguments.cs
- OrderByQueryOptionExpression.cs
- XmlTypeAttribute.cs
- WebPartsSection.cs
- TransactionInformation.cs
- Context.cs
- XmlAttributeCollection.cs
- CookieProtection.cs
- ParenthesizePropertyNameAttribute.cs
- LambdaCompiler.Logical.cs
- WindowsGraphics2.cs
- Wrapper.cs
- BindingMemberInfo.cs
- Group.cs
- ActivityInstanceReference.cs
- CacheDependency.cs
- _SecureChannel.cs
- SqlCachedBuffer.cs
- UnauthorizedAccessException.cs
- TextMetrics.cs
- ControlCollection.cs
- OdbcDataAdapter.cs
- TypeInitializationException.cs
- CallbackHandler.cs
- CorrelationActionMessageFilter.cs
- SafeFileMappingHandle.cs
- StdValidatorsAndConverters.cs