Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / UnaryQueryOperator.cs / 1305376 / UnaryQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // UnaryQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// The base class from which all binary query operators derive, that is, those that /// have two child operators. This introduces some convenience methods for those /// classes, as well as any state common to all subclasses. /// ////// internal abstract class UnaryQueryOperator : QueryOperator { // The single child operator for the current node. private readonly QueryOperator m_child; // The state of the order index of the output of this operator. private OrdinalIndexState m_indexState = OrdinalIndexState.Shuffled; //---------------------------------------------------------------------------------------- // Constructors // internal UnaryQueryOperator(IEnumerable child) : this(QueryOperator .AsQueryOperator(child)) { } internal UnaryQueryOperator(IEnumerable child, bool outputOrdered) : this(QueryOperator .AsQueryOperator(child), outputOrdered) { } private UnaryQueryOperator(QueryOperator child) : this(child, child.OutputOrdered, child.SpecifiedQuerySettings) { } internal UnaryQueryOperator(QueryOperator child, bool outputOrdered) : this(child, outputOrdered, child.SpecifiedQuerySettings) { } private UnaryQueryOperator(QueryOperator child, bool outputOrdered, QuerySettings settings) : base(outputOrdered, settings) { m_child = child; } internal QueryOperator Child { get { return m_child; } } internal override sealed OrdinalIndexState OrdinalIndexState { get { return m_indexState; } } protected void SetOrdinalIndexState(OrdinalIndexState indexState) { m_indexState = indexState; } //--------------------------------------------------------------------------------------- // This method wraps each enumerator in inputStream with an enumerator performing this // operator's transformation. However, instead of returning the transformed partitioned // stream, we pass it to a recipient object by calling recipient.Give (..). That // way, we can "return" a partitioned stream that potentially uses a different order key // from the order key of the input stream. // internal abstract void WrapPartitionedStream ( PartitionedStream inputStream, IPartitionedStreamRecipient recipient, bool preferStriping, QuerySettings settings); //--------------------------------------------------------------------------------------- // Implementation of QueryResults for an unary operator. The results will not be indexible // unless a derived class provides that functionality. // internal class UnaryQueryOperatorResults : QueryResults { protected QueryResults m_childQueryResults; // Results of the child query private UnaryQueryOperator m_op; // Operator that generated these results private QuerySettings m_settings; // Settings collected from the query private bool m_preferStriping; // If the results are indexible, should we use striping when partitioning them internal UnaryQueryOperatorResults(QueryResults childQueryResults, UnaryQueryOperator op, QuerySettings settings, bool preferStriping) { m_childQueryResults = childQueryResults; m_op = op; m_settings = settings; m_preferStriping = preferStriping; } internal override void GivePartitionedStream(IPartitionedStreamRecipient recipient) { Contract.Assert(IsIndexible == (m_op.OrdinalIndexState == OrdinalIndexState.Indexible)); if (m_settings.ExecutionMode.Value == ParallelExecutionMode.Default && m_op.LimitsParallelism) { // We need to run the query sequentially, up to and including this operator IEnumerable opSequential = m_op.AsSequentialQuery(m_settings.CancellationState.ExternalCancellationToken); PartitionedStream result = ExchangeUtilities.PartitionDataSource( opSequential, m_settings.DegreeOfParallelism.Value, m_preferStriping); recipient.Receive (result); } else if (IsIndexible) { // The output of this operator is indexible. Pass the partitioned output into the IPartitionedStreamRecipient. PartitionedStream result = ExchangeUtilities.PartitionDataSource(this, m_settings.DegreeOfParallelism.Value, m_preferStriping); recipient.Receive (result); } else { // The common case: get partitions from the child and wrap each partition. m_childQueryResults.GivePartitionedStream(new ChildResultsRecipient(recipient, m_op, m_preferStriping, m_settings)); } } //--------------------------------------------------------------------------------------- // ChildResultsRecipient is a recipient of a partitioned stream. It receives a partitioned // stream from the child operator, wraps the enumerators with the transformation for this // operator, and passes the partitioned stream along to the next recipient (the parent // operator). // private class ChildResultsRecipient : IPartitionedStreamRecipient { IPartitionedStreamRecipient m_outputRecipient; UnaryQueryOperator m_op; bool m_preferStriping; QuerySettings m_settings; internal ChildResultsRecipient( IPartitionedStreamRecipient outputRecipient, UnaryQueryOperator op, bool preferStriping, QuerySettings settings) { m_outputRecipient = outputRecipient; m_op = op; m_preferStriping = preferStriping; m_settings = settings; } public void Receive (PartitionedStream inputStream) { // Call WrapPartitionedStream on our operator, which will wrap the input // partitioned stream, and pass the result along to m_outputRecipient. m_op.WrapPartitionedStream(inputStream, m_outputRecipient, m_preferStriping, m_settings); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // UnaryQueryOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; namespace System.Linq.Parallel { ////// The base class from which all binary query operators derive, that is, those that /// have two child operators. This introduces some convenience methods for those /// classes, as well as any state common to all subclasses. /// ////// internal abstract class UnaryQueryOperator : QueryOperator { // The single child operator for the current node. private readonly QueryOperator m_child; // The state of the order index of the output of this operator. private OrdinalIndexState m_indexState = OrdinalIndexState.Shuffled; //---------------------------------------------------------------------------------------- // Constructors // internal UnaryQueryOperator(IEnumerable child) : this(QueryOperator .AsQueryOperator(child)) { } internal UnaryQueryOperator(IEnumerable child, bool outputOrdered) : this(QueryOperator .AsQueryOperator(child), outputOrdered) { } private UnaryQueryOperator(QueryOperator child) : this(child, child.OutputOrdered, child.SpecifiedQuerySettings) { } internal UnaryQueryOperator(QueryOperator child, bool outputOrdered) : this(child, outputOrdered, child.SpecifiedQuerySettings) { } private UnaryQueryOperator(QueryOperator child, bool outputOrdered, QuerySettings settings) : base(outputOrdered, settings) { m_child = child; } internal QueryOperator Child { get { return m_child; } } internal override sealed OrdinalIndexState OrdinalIndexState { get { return m_indexState; } } protected void SetOrdinalIndexState(OrdinalIndexState indexState) { m_indexState = indexState; } //--------------------------------------------------------------------------------------- // This method wraps each enumerator in inputStream with an enumerator performing this // operator's transformation. However, instead of returning the transformed partitioned // stream, we pass it to a recipient object by calling recipient.Give (..). That // way, we can "return" a partitioned stream that potentially uses a different order key // from the order key of the input stream. // internal abstract void WrapPartitionedStream ( PartitionedStream inputStream, IPartitionedStreamRecipient recipient, bool preferStriping, QuerySettings settings); //--------------------------------------------------------------------------------------- // Implementation of QueryResults for an unary operator. The results will not be indexible // unless a derived class provides that functionality. // internal class UnaryQueryOperatorResults : QueryResults { protected QueryResults m_childQueryResults; // Results of the child query private UnaryQueryOperator m_op; // Operator that generated these results private QuerySettings m_settings; // Settings collected from the query private bool m_preferStriping; // If the results are indexible, should we use striping when partitioning them internal UnaryQueryOperatorResults(QueryResults childQueryResults, UnaryQueryOperator op, QuerySettings settings, bool preferStriping) { m_childQueryResults = childQueryResults; m_op = op; m_settings = settings; m_preferStriping = preferStriping; } internal override void GivePartitionedStream(IPartitionedStreamRecipient recipient) { Contract.Assert(IsIndexible == (m_op.OrdinalIndexState == OrdinalIndexState.Indexible)); if (m_settings.ExecutionMode.Value == ParallelExecutionMode.Default && m_op.LimitsParallelism) { // We need to run the query sequentially, up to and including this operator IEnumerable opSequential = m_op.AsSequentialQuery(m_settings.CancellationState.ExternalCancellationToken); PartitionedStream result = ExchangeUtilities.PartitionDataSource( opSequential, m_settings.DegreeOfParallelism.Value, m_preferStriping); recipient.Receive (result); } else if (IsIndexible) { // The output of this operator is indexible. Pass the partitioned output into the IPartitionedStreamRecipient. PartitionedStream result = ExchangeUtilities.PartitionDataSource(this, m_settings.DegreeOfParallelism.Value, m_preferStriping); recipient.Receive (result); } else { // The common case: get partitions from the child and wrap each partition. m_childQueryResults.GivePartitionedStream(new ChildResultsRecipient(recipient, m_op, m_preferStriping, m_settings)); } } //--------------------------------------------------------------------------------------- // ChildResultsRecipient is a recipient of a partitioned stream. It receives a partitioned // stream from the child operator, wraps the enumerators with the transformation for this // operator, and passes the partitioned stream along to the next recipient (the parent // operator). // private class ChildResultsRecipient : IPartitionedStreamRecipient { IPartitionedStreamRecipient m_outputRecipient; UnaryQueryOperator m_op; bool m_preferStriping; QuerySettings m_settings; internal ChildResultsRecipient( IPartitionedStreamRecipient outputRecipient, UnaryQueryOperator op, bool preferStriping, QuerySettings settings) { m_outputRecipient = outputRecipient; m_op = op; m_preferStriping = preferStriping; m_settings = settings; } public void Receive (PartitionedStream inputStream) { // Call WrapPartitionedStream on our operator, which will wrap the input // partitioned stream, and pass the result along to m_outputRecipient. m_op.WrapPartitionedStream(inputStream, m_outputRecipient, m_preferStriping, m_settings); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
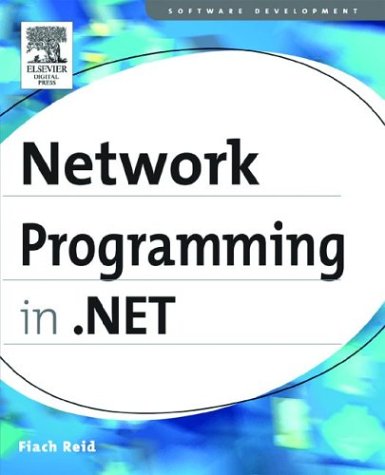
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextPattern.cs
- DbProviderFactories.cs
- PersonalizationStateInfoCollection.cs
- EditBehavior.cs
- RadioButton.cs
- XmlTextWriter.cs
- ConfigurationManagerHelper.cs
- URL.cs
- ReceiveCompletedEventArgs.cs
- CodeAccessSecurityEngine.cs
- HashMembershipCondition.cs
- Grant.cs
- Point3DCollectionConverter.cs
- EventlogProvider.cs
- Brushes.cs
- WebControlAdapter.cs
- SamlAttribute.cs
- SQLBinaryStorage.cs
- SqlInternalConnectionSmi.cs
- ControlCachePolicy.cs
- Point3DKeyFrameCollection.cs
- ApplicationProxyInternal.cs
- ConstructorBuilder.cs
- DataShape.cs
- FlowLayoutPanelDesigner.cs
- ValueConversionAttribute.cs
- SqlRowUpdatingEvent.cs
- TaskFormBase.cs
- HttpListenerPrefixCollection.cs
- DetailsViewInsertEventArgs.cs
- SynchronizationContext.cs
- TextEditorLists.cs
- HtmlToClrEventProxy.cs
- SecurityPolicySection.cs
- GetPageNumberCompletedEventArgs.cs
- ProjectionPathSegment.cs
- SliderAutomationPeer.cs
- MailHeaderInfo.cs
- QilXmlReader.cs
- BamlLocalizer.cs
- TableSectionStyle.cs
- OraclePermission.cs
- RtfControls.cs
- RegexBoyerMoore.cs
- FragmentNavigationEventArgs.cs
- ChannelSettingsElement.cs
- NavigatingCancelEventArgs.cs
- ConstructorArgumentAttribute.cs
- ExtenderControl.cs
- HttpModule.cs
- PropertyEmitterBase.cs
- BooleanKeyFrameCollection.cs
- Viewport2DVisual3D.cs
- OdbcPermission.cs
- XmlNullResolver.cs
- ExitEventArgs.cs
- TextMarkerSource.cs
- ScriptResourceHandler.cs
- EdmProviderManifest.cs
- WebBaseEventKeyComparer.cs
- TableLayoutStyle.cs
- DynamicILGenerator.cs
- ToolboxItemAttribute.cs
- TextPointerBase.cs
- RelationalExpressions.cs
- CompositeActivityTypeDescriptor.cs
- IndentedWriter.cs
- CharKeyFrameCollection.cs
- HighlightComponent.cs
- MobileControlsSectionHandler.cs
- SiteMapDataSourceDesigner.cs
- Image.cs
- WebPartMovingEventArgs.cs
- Delay.cs
- XmlWriterTraceListener.cs
- InternalControlCollection.cs
- ConnectionPoint.cs
- HttpHandlerActionCollection.cs
- ExpressionBuilder.cs
- ListSortDescriptionCollection.cs
- UnsafeMethods.cs
- StrongNameIdentityPermission.cs
- externdll.cs
- DataServiceClientException.cs
- DataPagerFieldCollection.cs
- RegionIterator.cs
- BaseAsyncResult.cs
- MouseActionConverter.cs
- CompilationRelaxations.cs
- Propagator.cs
- X509Chain.cs
- PermissionSetEnumerator.cs
- DataAccessor.cs
- FieldMetadata.cs
- FontCacheLogic.cs
- BooleanConverter.cs
- IdentityNotMappedException.cs
- ADConnectionHelper.cs
- TextSchema.cs
- ConfigUtil.cs