Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / WindowCollection.cs / 1 / WindowCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WindowCollection can be used to interate over all the windows // that have been opened in the current application. // // History: // 08/10/04: kusumav Moved out of Application.cs to its own separate file. // //--------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; namespace System.Windows { #region WindowCollection class ////// WindowCollection can be used to interate over all the windows that have been /// opened in the current application. /// // public sealed class WindowCollection : ICollection { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Default Constructor /// public WindowCollection() { _list = new ArrayList(1); } internal WindowCollection(int count) { Debug.Assert(count >= 0, "count must not be less than zero"); _list = new ArrayList(count); } #endregion Public Methods //------------------------------------------------------ // // Operator overload // //----------------------------------------------------- #region Operator overload ////// Overloaded [] operator to access the WindowCollection list /// public Window this[int index] { get { return _list[index] as Window; } } #endregion Operator overload //------------------------------------------------------ // // IEnumerable implementation // //------------------------------------------------------ #region IEnumerable implementation ////// GetEnumerator /// ///public IEnumerator GetEnumerator() { return _list.GetEnumerator(); } #endregion IEnumerable implementation //------------------------------------------------------- // // ICollection implementation (derives from IEnumerable) // //-------------------------------------------------------- #region ICollection implementation /// /// CopyTo /// /// /// void ICollection.CopyTo(Array array, int index) { _list.CopyTo(array, index); } ////// CopyTo /// /// /// public void CopyTo(Window[] array, int index) { _list.CopyTo(array, index); } ////// Count property /// public int Count { get { return _list.Count; } } ////// IsSynchronized /// public bool IsSynchronized { get { return _list.IsSynchronized; } } ////// SyncRoot /// public Object SyncRoot { get { return _list.SyncRoot; } } #endregion ICollection implementation //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal WindowCollection Clone() { WindowCollection clone; lock (_list.SyncRoot) { clone = new WindowCollection(_list.Count); for (int i = 0; i < _list.Count; i++) { clone._list.Add(_list[i]); } } return clone; } internal void Remove(Window win) { lock (_list.SyncRoot) { _list.Remove(win); } } internal void RemoveAt(int index) { lock (_list.SyncRoot) { _list.Remove(index); } } internal int Add (Window win) { lock (_list.SyncRoot) { return _list.Add(win); } } internal bool HasItem(Window win) { lock (_list.SyncRoot) { for (int i = 0; i < _list.Count; i++) { if (_list[i] == win) { return true; } } } return false; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private ArrayList _list; #endregion Private Fields } #endregion WindowCollection class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // WindowCollection can be used to interate over all the windows // that have been opened in the current application. // // History: // 08/10/04: kusumav Moved out of Application.cs to its own separate file. // //--------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; namespace System.Windows { #region WindowCollection class ////// WindowCollection can be used to interate over all the windows that have been /// opened in the current application. /// // public sealed class WindowCollection : ICollection { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Default Constructor /// public WindowCollection() { _list = new ArrayList(1); } internal WindowCollection(int count) { Debug.Assert(count >= 0, "count must not be less than zero"); _list = new ArrayList(count); } #endregion Public Methods //------------------------------------------------------ // // Operator overload // //----------------------------------------------------- #region Operator overload ////// Overloaded [] operator to access the WindowCollection list /// public Window this[int index] { get { return _list[index] as Window; } } #endregion Operator overload //------------------------------------------------------ // // IEnumerable implementation // //------------------------------------------------------ #region IEnumerable implementation ////// GetEnumerator /// ///public IEnumerator GetEnumerator() { return _list.GetEnumerator(); } #endregion IEnumerable implementation //------------------------------------------------------- // // ICollection implementation (derives from IEnumerable) // //-------------------------------------------------------- #region ICollection implementation /// /// CopyTo /// /// /// void ICollection.CopyTo(Array array, int index) { _list.CopyTo(array, index); } ////// CopyTo /// /// /// public void CopyTo(Window[] array, int index) { _list.CopyTo(array, index); } ////// Count property /// public int Count { get { return _list.Count; } } ////// IsSynchronized /// public bool IsSynchronized { get { return _list.IsSynchronized; } } ////// SyncRoot /// public Object SyncRoot { get { return _list.SyncRoot; } } #endregion ICollection implementation //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal WindowCollection Clone() { WindowCollection clone; lock (_list.SyncRoot) { clone = new WindowCollection(_list.Count); for (int i = 0; i < _list.Count; i++) { clone._list.Add(_list[i]); } } return clone; } internal void Remove(Window win) { lock (_list.SyncRoot) { _list.Remove(win); } } internal void RemoveAt(int index) { lock (_list.SyncRoot) { _list.Remove(index); } } internal int Add (Window win) { lock (_list.SyncRoot) { return _list.Add(win); } } internal bool HasItem(Window win) { lock (_list.SyncRoot) { for (int i = 0; i < _list.Count; i++) { if (_list[i] == win) { return true; } } } return false; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private ArrayList _list; #endregion Private Fields } #endregion WindowCollection class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
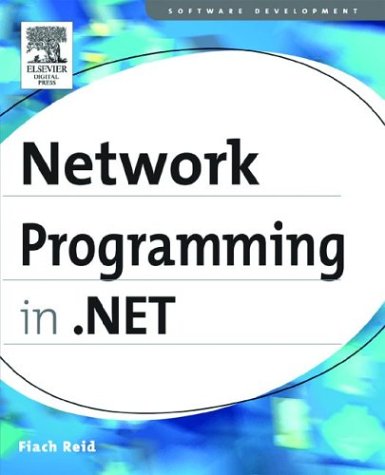
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmbeddedObject.cs
- TextModifier.cs
- ListItemCollection.cs
- UncommonField.cs
- EventArgs.cs
- SamlAssertionDirectKeyIdentifierClause.cs
- CngProvider.cs
- WinFormsUtils.cs
- SHA1.cs
- SettingsSavedEventArgs.cs
- AddInDeploymentState.cs
- ControlValuePropertyAttribute.cs
- AutoScrollExpandMessageFilter.cs
- Roles.cs
- APCustomTypeDescriptor.cs
- XmlByteStreamWriter.cs
- WorkflowValidationFailedException.cs
- EntityStoreSchemaGenerator.cs
- ElasticEase.cs
- XmlSortKeyAccumulator.cs
- ZipIOExtraField.cs
- DataGridViewControlCollection.cs
- TraceData.cs
- AdPostCacheSubstitution.cs
- IdentifierService.cs
- TextEndOfSegment.cs
- DesignerWebPartChrome.cs
- BackoffTimeoutHelper.cs
- FixedSOMTableRow.cs
- AppliedDeviceFiltersDialog.cs
- XmlSchemaSimpleType.cs
- TimelineGroup.cs
- PeerCustomResolverBindingElement.cs
- Accessors.cs
- DataGridViewComboBoxColumn.cs
- NotificationContext.cs
- NameSpaceEvent.cs
- DataStreams.cs
- NumericUpDown.cs
- StreamMarshaler.cs
- Workspace.cs
- DataSourceGeneratorException.cs
- Selection.cs
- BitmapEffectDrawingContextState.cs
- HtmlGenericControl.cs
- GenericParameterDataContract.cs
- FormViewActionList.cs
- ValidateNames.cs
- VirtualizedContainerService.cs
- DesignerSerializerAttribute.cs
- ColorAnimationBase.cs
- FilteredReadOnlyMetadataCollection.cs
- HttpVersion.cs
- BooleanExpr.cs
- NodeFunctions.cs
- ProtocolViolationException.cs
- DummyDataSource.cs
- StringSorter.cs
- UrlPropertyAttribute.cs
- SpotLight.cs
- InputScopeManager.cs
- HostedBindingBehavior.cs
- TextSpan.cs
- OuterGlowBitmapEffect.cs
- OutgoingWebRequestContext.cs
- Int32RectValueSerializer.cs
- SendKeys.cs
- PreviewPrintController.cs
- DirectoryObjectSecurity.cs
- ManagementException.cs
- XmlValidatingReader.cs
- PlatformNotSupportedException.cs
- Scene3D.cs
- PageSetupDialog.cs
- VariableQuery.cs
- TableRowCollection.cs
- GroupDescription.cs
- StringUtil.cs
- InternalBase.cs
- FormViewModeEventArgs.cs
- WeakReference.cs
- XmlChildEnumerator.cs
- WebScriptEnablingElement.cs
- TabControlCancelEvent.cs
- MulticastDelegate.cs
- DependencySource.cs
- WorkerRequest.cs
- OperationInfoBase.cs
- ReachDocumentPageSerializer.cs
- EndpointInstanceProvider.cs
- DataReaderContainer.cs
- EnumUnknown.cs
- DataBindingCollection.cs
- BitHelper.cs
- cache.cs
- OleDbEnumerator.cs
- TrackingRecord.cs
- _TLSstream.cs
- DeclarativeCatalogPart.cs
- ObjectQueryState.cs