Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / InputScopeManager.cs / 1 / InputScopeManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class InputScopeManager { private InputScope scopeStack; private string defaultNS = string.Empty; private XPathNavigator navigator; // We need this nsvigator for document() function implementation public InputScopeManager(XPathNavigator navigator, InputScope rootScope) { this.navigator = navigator; this.scopeStack = rootScope; } internal InputScope CurrentScope { get { return this.scopeStack; } } internal InputScope VariableScope { get { Debug.Assert(this.scopeStack != null); Debug.Assert(this.scopeStack.Parent != null); return this.scopeStack.Parent; } } internal InputScopeManager Clone() { InputScopeManager manager = new InputScopeManager(this.navigator, null); manager.scopeStack = this.scopeStack; manager.defaultNS = this.defaultNS; return manager; } public XPathNavigator Navigator { get {return this.navigator;} } internal InputScope PushScope() { this.scopeStack = new InputScope(this.scopeStack); return this.scopeStack; } internal void PopScope() { Debug.Assert(this.scopeStack != null, "Push/Pop disbalance"); if (this.scopeStack == null) { return; } for (NamespaceDecl scope = this.scopeStack.Scopes; scope != null; scope = scope.Next) { this.defaultNS = scope.PrevDefaultNsUri; } this.scopeStack = this.scopeStack.Parent; } internal void PushNamespace(string prefix, string nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null); Debug.Assert(nspace != null); this.scopeStack.AddNamespace(prefix, nspace, this.defaultNS); if (prefix == null || prefix.Length == 0) { this.defaultNS = nspace; } } // CompileContext public string DefaultNamespace { get { return this.defaultNS; } } private string ResolveNonEmptyPrefix(string prefix) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null || prefix.Length != 0); if (prefix == Keywords.s_Xml) { return Keywords.s_XmlNamespace; } else if (prefix == Keywords.s_Xmlns) { return Keywords.s_XmlnsNamespace; } for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { string nspace = inputScope.ResolveNonAtom(prefix); if (nspace != null) { return nspace; } } throw XsltException.Create(Res.Xslt_InvalidPrefix, prefix); } public string ResolveXmlNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return this.defaultNS; } return ResolveNonEmptyPrefix(prefix); } public string ResolveXPathNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return string.Empty; } return ResolveNonEmptyPrefix(prefix); } internal void InsertExtensionNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExtensionNamespace(nsList[idx]); } } internal bool IsExtensionNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExtensionNamespace( nspace )) { return true; } } return false; } internal void InsertExcludedNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExcludedNamespace(nsList[idx]); } } internal bool IsExcludedNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExcludedNamespace( nspace )) { return true; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class InputScopeManager { private InputScope scopeStack; private string defaultNS = string.Empty; private XPathNavigator navigator; // We need this nsvigator for document() function implementation public InputScopeManager(XPathNavigator navigator, InputScope rootScope) { this.navigator = navigator; this.scopeStack = rootScope; } internal InputScope CurrentScope { get { return this.scopeStack; } } internal InputScope VariableScope { get { Debug.Assert(this.scopeStack != null); Debug.Assert(this.scopeStack.Parent != null); return this.scopeStack.Parent; } } internal InputScopeManager Clone() { InputScopeManager manager = new InputScopeManager(this.navigator, null); manager.scopeStack = this.scopeStack; manager.defaultNS = this.defaultNS; return manager; } public XPathNavigator Navigator { get {return this.navigator;} } internal InputScope PushScope() { this.scopeStack = new InputScope(this.scopeStack); return this.scopeStack; } internal void PopScope() { Debug.Assert(this.scopeStack != null, "Push/Pop disbalance"); if (this.scopeStack == null) { return; } for (NamespaceDecl scope = this.scopeStack.Scopes; scope != null; scope = scope.Next) { this.defaultNS = scope.PrevDefaultNsUri; } this.scopeStack = this.scopeStack.Parent; } internal void PushNamespace(string prefix, string nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null); Debug.Assert(nspace != null); this.scopeStack.AddNamespace(prefix, nspace, this.defaultNS); if (prefix == null || prefix.Length == 0) { this.defaultNS = nspace; } } // CompileContext public string DefaultNamespace { get { return this.defaultNS; } } private string ResolveNonEmptyPrefix(string prefix) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(prefix != null || prefix.Length != 0); if (prefix == Keywords.s_Xml) { return Keywords.s_XmlNamespace; } else if (prefix == Keywords.s_Xmlns) { return Keywords.s_XmlnsNamespace; } for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { string nspace = inputScope.ResolveNonAtom(prefix); if (nspace != null) { return nspace; } } throw XsltException.Create(Res.Xslt_InvalidPrefix, prefix); } public string ResolveXmlNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return this.defaultNS; } return ResolveNonEmptyPrefix(prefix); } public string ResolveXPathNamespace(string prefix) { Debug.Assert(prefix != null); if (prefix.Length == 0) { return string.Empty; } return ResolveNonEmptyPrefix(prefix); } internal void InsertExtensionNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExtensionNamespace(nsList[idx]); } } internal bool IsExtensionNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExtensionNamespace( nspace )) { return true; } } return false; } internal void InsertExcludedNamespaces(string[] nsList) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); Debug.Assert(nsList != null); for (int idx = 0; idx < nsList.Length; idx++) { this.scopeStack.InsertExcludedNamespace(nsList[idx]); } } internal bool IsExcludedNamespace(String nspace) { Debug.Assert(this.scopeStack != null, "PushScope wasn't called"); for (InputScope inputScope = this.scopeStack; inputScope != null; inputScope = inputScope.Parent) { if (inputScope.IsExcludedNamespace( nspace )) { return true; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
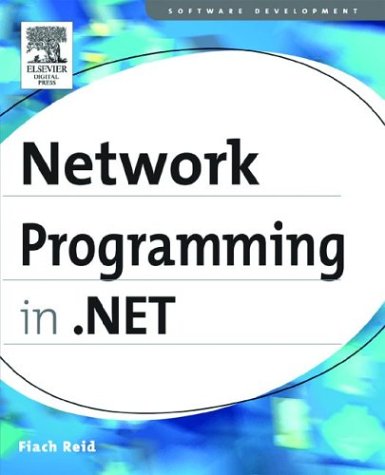
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreeText.cs
- EntityDesignerUtils.cs
- PageSettings.cs
- CursorConverter.cs
- DefaultAsyncDataDispatcher.cs
- XDRSchema.cs
- DataGridSortCommandEventArgs.cs
- FreezableCollection.cs
- FamilyCollection.cs
- XmlIgnoreAttribute.cs
- ComponentSerializationService.cs
- GeneralTransformGroup.cs
- FixedSOMLineRanges.cs
- HeaderedItemsControl.cs
- LinkedList.cs
- CharacterMetricsDictionary.cs
- MediaSystem.cs
- Menu.cs
- DbConnectionPoolIdentity.cs
- MetadataSource.cs
- ItemPager.cs
- _Rfc2616CacheValidators.cs
- XmlSchemaNotation.cs
- CodeStatementCollection.cs
- NetworkInterface.cs
- ReaderWriterLock.cs
- PropertyRef.cs
- UnlockInstanceAsyncResult.cs
- MouseGesture.cs
- LoginUtil.cs
- AppLevelCompilationSectionCache.cs
- RawStylusActions.cs
- JsonDeserializer.cs
- CredentialCache.cs
- RuleSettingsCollection.cs
- ObjectMemberMapping.cs
- IPAddressCollection.cs
- CalendarDay.cs
- ExecutorLocksHeldException.cs
- JsonFormatMapping.cs
- GroupBoxRenderer.cs
- SynchronizedInputProviderWrapper.cs
- CommandLibraryHelper.cs
- XmlDocumentFragment.cs
- ConnectionPoint.cs
- Constraint.cs
- RoutedCommand.cs
- ResourcesChangeInfo.cs
- GridViewColumn.cs
- DateTimeConverter.cs
- parserscommon.cs
- BinaryFormatter.cs
- LocalizedNameDescriptionPair.cs
- HtmlInputHidden.cs
- DetailsViewUpdatedEventArgs.cs
- Point3DKeyFrameCollection.cs
- DeploymentSectionCache.cs
- MoveSizeWinEventHandler.cs
- SystemResourceKey.cs
- Matrix3DStack.cs
- diagnosticsswitches.cs
- GifBitmapDecoder.cs
- FileEnumerator.cs
- Animatable.cs
- DisableDpiAwarenessAttribute.cs
- GZipDecoder.cs
- ZeroOpNode.cs
- DataException.cs
- WindowsStatic.cs
- XPathItem.cs
- WorkflowOwnershipException.cs
- ToolStripControlHost.cs
- DataViewManagerListItemTypeDescriptor.cs
- future.cs
- WebPermission.cs
- HMACSHA512.cs
- DebugTraceHelper.cs
- Facet.cs
- QilGenerator.cs
- SchemaLookupTable.cs
- DeclaredTypeElementCollection.cs
- ConfigXmlComment.cs
- DrawItemEvent.cs
- ErrorsHelper.cs
- ThreadAbortException.cs
- QEncodedStream.cs
- CodeCompiler.cs
- Parameter.cs
- DBSchemaTable.cs
- ModulesEntry.cs
- DesignerLoader.cs
- ExtenderHelpers.cs
- TextBlock.cs
- UIPermission.cs
- AsyncPostBackTrigger.cs
- DataColumnMapping.cs
- ErasingStroke.cs
- MsmqPoisonMessageException.cs
- DependencyObjectPropertyDescriptor.cs
- MaterialGroup.cs