Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / MouseGesture.cs / 1305600 / MouseGesture.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The MouseGesture class is used by the developer to create Gestures for // Mouse Device // // See spec at : http://avalon/coreUI/Specs/Commanding%20--%20design.htm // // // History: // 03/26/2004 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.Windows.Markup; using System.ComponentModel; namespace System.Windows.Input { ////// MouseGesture - MouseAction and Modifier combination. /// Can be set on properties of MouseBinding and RoutedCommand. /// [TypeConverter(typeof(MouseGestureConverter))] [ValueSerializer(typeof(MouseGestureValueSerializer))] public class MouseGesture : InputGesture { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public MouseGesture() // Mouse action { } ////// constructor /// /// Mouse Action public MouseGesture(MouseAction mouseAction): this(mouseAction, ModifierKeys.None) { } ////// Constructor /// /// Mouse Action /// Modifiers public MouseGesture( MouseAction mouseAction,ModifierKeys modifiers) // acclerator action { if (!MouseGesture.IsDefinedMouseAction(mouseAction)) throw new InvalidEnumArgumentException("mouseAction", (int)mouseAction, typeof(MouseAction)); if (!ModifierKeysConverter.IsDefinedModifierKeys(modifiers)) throw new InvalidEnumArgumentException("modifiers", (int)modifiers, typeof(ModifierKeys)); _modifiers = modifiers; _mouseAction = mouseAction; //AttachClassListeners(); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Action /// public MouseAction MouseAction { get { return _mouseAction; } set { if (!MouseGesture.IsDefinedMouseAction((MouseAction)value)) throw new InvalidEnumArgumentException("value", (int)value, typeof(MouseAction)); if (_mouseAction != value) { _mouseAction = (MouseAction)value; OnPropertyChanged("MouseAction"); } } } ////// Modifiers /// public ModifierKeys Modifiers { get { return _modifiers; } set { if (!ModifierKeysConverter.IsDefinedModifierKeys((ModifierKeys)value)) throw new InvalidEnumArgumentException("value", (int)value, typeof(ModifierKeys)); if (_modifiers != value) { _modifiers = (ModifierKeys)value; OnPropertyChanged("Modifiers"); } } } ////// Compares InputEventArgs with current Input /// /// the element to receive the command /// inputEventArgs to compare to ///True - if matches, false otherwise. /// public override bool Matches(object targetElement, InputEventArgs inputEventArgs) { MouseAction mouseAction = GetMouseAction(inputEventArgs); if(mouseAction != MouseAction.None) { return ( ( (int)this.MouseAction == (int)mouseAction ) && ( this.Modifiers == Keyboard.Modifiers ) ); } return false; } // Helper like Enum.IsDefined, for MouseAction. internal static bool IsDefinedMouseAction(MouseAction mouseAction) { return (mouseAction >= MouseAction.None && mouseAction <= MouseAction.MiddleDoubleClick); } #endregion Public Methods #region Internal NotifyProperty changed ////// PropertyChanged event Handler /// internal event PropertyChangedEventHandler PropertyChanged; ////// PropertyChanged virtual /// internal virtual void OnPropertyChanged(string propertyName) { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } #endregion //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static MouseAction GetMouseAction(InputEventArgs inputArgs) { MouseAction MouseAction = MouseAction.None; MouseEventArgs mouseArgs = inputArgs as MouseEventArgs; if(mouseArgs != null) { if(inputArgs is MouseWheelEventArgs) { MouseAction = MouseAction.WheelClick; } else { MouseButtonEventArgs args = inputArgs as MouseButtonEventArgs; switch(args.ChangedButton) { case MouseButton.Left: { if(args.ClickCount == 2) MouseAction = MouseAction.LeftDoubleClick; else if(args.ClickCount == 1) MouseAction = MouseAction.LeftClick; } break; case MouseButton.Right: { if(args.ClickCount == 2) MouseAction = MouseAction.RightDoubleClick; else if(args.ClickCount == 1) MouseAction = MouseAction.RightClick; } break; case MouseButton.Middle: { if(args.ClickCount == 2) MouseAction = MouseAction.MiddleDoubleClick; else if(args.ClickCount == 1) MouseAction = MouseAction.MiddleClick; } break; } } } return MouseAction; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private MouseAction _mouseAction = MouseAction.None; private ModifierKeys _modifiers = ModifierKeys.None; // private static bool _classRegistered = false; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
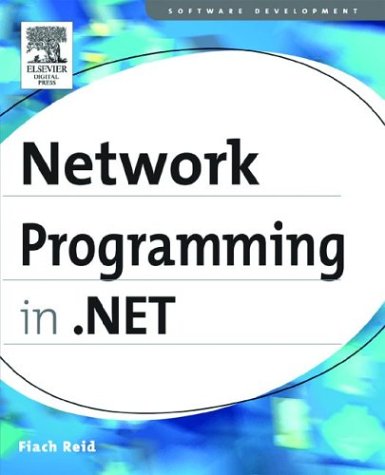
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlDataSource.cs
- SmtpTransport.cs
- TraceRecord.cs
- SchemaTableOptionalColumn.cs
- UpdatePanelControlTrigger.cs
- Point3DCollection.cs
- ActiveDesignSurfaceEvent.cs
- BoolExpression.cs
- CustomAttributeBuilder.cs
- BitmapEffectInput.cs
- AudioBase.cs
- NativeStructs.cs
- IndexedString.cs
- FormViewInsertedEventArgs.cs
- UInt32Storage.cs
- PlanCompiler.cs
- WriterOutput.cs
- PaintEvent.cs
- GridProviderWrapper.cs
- DataException.cs
- AnnouncementDispatcherAsyncResult.cs
- CharConverter.cs
- XmlSchemaInclude.cs
- X509Utils.cs
- ItemsControl.cs
- XmlAttributeAttribute.cs
- HTMLTagNameToTypeMapper.cs
- DbParameterCollection.cs
- TypedServiceChannelBuilder.cs
- AutomationPropertyInfo.cs
- PopOutPanel.cs
- DiagnosticsConfiguration.cs
- GridEntryCollection.cs
- CustomAttributeFormatException.cs
- TextElement.cs
- DefaultAsyncDataDispatcher.cs
- SqlExpander.cs
- DesignerLabelAdapter.cs
- DocComment.cs
- CodeIdentifiers.cs
- DateRangeEvent.cs
- AccessibleObject.cs
- PropertyStore.cs
- DataTemplateKey.cs
- SequenceFullException.cs
- LiteralTextParser.cs
- ImageKeyConverter.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- IisTraceWebEventProvider.cs
- RuleInfoComparer.cs
- Expander.cs
- TimerElapsedEvenArgs.cs
- XpsFontSubsetter.cs
- WebInvokeAttribute.cs
- RuleSet.cs
- RelativeSource.cs
- SoapAttributeAttribute.cs
- GiveFeedbackEvent.cs
- QueryStringParameter.cs
- SHA512Cng.cs
- TreeViewImageIndexConverter.cs
- ProfileGroupSettingsCollection.cs
- IisTraceWebEventProvider.cs
- DeviceSpecificChoiceCollection.cs
- CharacterHit.cs
- StyleXamlTreeBuilder.cs
- EastAsianLunisolarCalendar.cs
- ServiceDescriptionData.cs
- StylusButtonEventArgs.cs
- InfoCardRSACryptoProvider.cs
- DependentTransaction.cs
- EndpointConfigContainer.cs
- BulletedList.cs
- DrawingImage.cs
- SortQuery.cs
- TextServicesHost.cs
- CodeNamespaceCollection.cs
- ContextMenu.cs
- __Filters.cs
- Enumerable.cs
- TimeSpanStorage.cs
- DesignSurfaceManager.cs
- GridProviderWrapper.cs
- GPPOINT.cs
- CompressEmulationStream.cs
- typedescriptorpermissionattribute.cs
- DesignerAttribute.cs
- IdentityReference.cs
- VirtualizedCellInfoCollection.cs
- TouchDevice.cs
- AnimationTimeline.cs
- Annotation.cs
- UInt64Converter.cs
- AsyncPostBackTrigger.cs
- SamlAssertionKeyIdentifierClause.cs
- ObjectConverter.cs
- SafeNativeMethods.cs
- MexHttpsBindingElement.cs
- PageRanges.cs
- httpapplicationstate.cs