Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / StyleXamlTreeBuilder.cs / 1 / StyleXamlTreeBuilder.cs
/****************************************************************************\ * * File: StyleXamlTreeBuilder.cs * * Purpose: Class that builds a style object from XAML * * History: * 11/13/03: peterost Created * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; namespace System.Windows.Markup { ////// XamlTree builder is the TreeBuilder implementation that loads a Tree /// from XAML. /// internal class StyleXamlTreeBuilder : XamlTreeBuilder { #region Constructors ////// Constructor. Set up associated baml writer and xaml parser to /// create the triumvarate needed for parsing a style block. This is /// the main method to call to create the needed object graph for /// parsing. /// public StyleXamlTreeBuilder( ParserContext parserContext, XamlReaderHelper tokenReader, ReadWriteStreamManager streamManager, ParserStack bamlReaderStack, ArrayList rootList) { Debug.Assert(null != parserContext, "ParserContext is null"); Debug.Assert(null != tokenReader, "TokenReader is null"); Debug.Assert(null != parserContext.XamlTypeMapper, "ParserContext.XamlTypeMapper is null"); Debug.Assert(null != streamManager, "StreamManager is null"); XamlParseMode = tokenReader.XamlParseMode; // Fetch the previous BamlRecordReader XamlTreeBuilderBamlRecordWriter previousBamlRecordWriter = (XamlTreeBuilderBamlRecordWriter)tokenReader.ControllingXamlParser.BamlRecordWriter; BamlRecordReader previousBamlRecordReader = previousBamlRecordWriter.TreeBuilder.RecordReader; Parser = new StyleXamlParser(this, tokenReader, parserContext); Parser.StreamManager = streamManager; Parser.XamlParseMode = XamlParseMode; RecordWriter = new XamlTreeBuilderBamlRecordWriter(this, streamManager.WriterStream, parserContext, true /*isSerializer*/ ); // Give Writer to the Parser, since it can't be passed in constructor due to // circular dependency. Parser.BamlRecordWriter = RecordWriter; // The BamlRecordReader needs its own parser context ParserContext readerParserContext = previousBamlRecordReader.ParserContext; RecordReader = new StyleBamlRecordReader(streamManager.ReaderStream, null, null, readerParserContext, bamlReaderStack, rootList); } #endregion Constructors #region Overrides ////// Forward parsing directive onto xaml parser. /// ////// An array containing the root objects in the XAML stream /// public override object ParseFragment() { // Tell the parser the starting depth Parser.Parse(); // its okay for root to be null if its an empty file or the parse // was stopped. return GetRoot(); } #endregion Overrides ////// Forward the processing of a xaml node to the parser. This is done when there is /// an out-of-band record that needs to be added to the baml stream, such as the /// start of a Style tag. /// internal void ProcessXamlNode(XamlNode xamlNode) { bool cleanup = false; bool done = false; Parser.ProcessXamlNode(xamlNode, ref cleanup, ref done); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: StyleXamlTreeBuilder.cs * * Purpose: Class that builds a style object from XAML * * History: * 11/13/03: peterost Created * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Xml; using System.IO; using System.Windows; using System.Text; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; namespace System.Windows.Markup { ////// XamlTree builder is the TreeBuilder implementation that loads a Tree /// from XAML. /// internal class StyleXamlTreeBuilder : XamlTreeBuilder { #region Constructors ////// Constructor. Set up associated baml writer and xaml parser to /// create the triumvarate needed for parsing a style block. This is /// the main method to call to create the needed object graph for /// parsing. /// public StyleXamlTreeBuilder( ParserContext parserContext, XamlReaderHelper tokenReader, ReadWriteStreamManager streamManager, ParserStack bamlReaderStack, ArrayList rootList) { Debug.Assert(null != parserContext, "ParserContext is null"); Debug.Assert(null != tokenReader, "TokenReader is null"); Debug.Assert(null != parserContext.XamlTypeMapper, "ParserContext.XamlTypeMapper is null"); Debug.Assert(null != streamManager, "StreamManager is null"); XamlParseMode = tokenReader.XamlParseMode; // Fetch the previous BamlRecordReader XamlTreeBuilderBamlRecordWriter previousBamlRecordWriter = (XamlTreeBuilderBamlRecordWriter)tokenReader.ControllingXamlParser.BamlRecordWriter; BamlRecordReader previousBamlRecordReader = previousBamlRecordWriter.TreeBuilder.RecordReader; Parser = new StyleXamlParser(this, tokenReader, parserContext); Parser.StreamManager = streamManager; Parser.XamlParseMode = XamlParseMode; RecordWriter = new XamlTreeBuilderBamlRecordWriter(this, streamManager.WriterStream, parserContext, true /*isSerializer*/ ); // Give Writer to the Parser, since it can't be passed in constructor due to // circular dependency. Parser.BamlRecordWriter = RecordWriter; // The BamlRecordReader needs its own parser context ParserContext readerParserContext = previousBamlRecordReader.ParserContext; RecordReader = new StyleBamlRecordReader(streamManager.ReaderStream, null, null, readerParserContext, bamlReaderStack, rootList); } #endregion Constructors #region Overrides ////// Forward parsing directive onto xaml parser. /// ////// An array containing the root objects in the XAML stream /// public override object ParseFragment() { // Tell the parser the starting depth Parser.Parse(); // its okay for root to be null if its an empty file or the parse // was stopped. return GetRoot(); } #endregion Overrides ////// Forward the processing of a xaml node to the parser. This is done when there is /// an out-of-band record that needs to be added to the baml stream, such as the /// start of a Style tag. /// internal void ProcessXamlNode(XamlNode xamlNode) { bool cleanup = false; bool done = false; Parser.ProcessXamlNode(xamlNode, ref cleanup, ref done); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
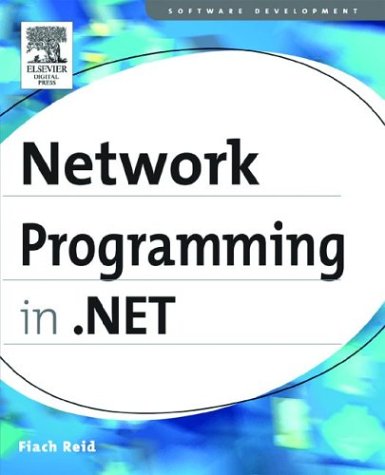
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssociationSetEnd.cs
- BitmapDownload.cs
- BooleanKeyFrameCollection.cs
- InputBindingCollection.cs
- HtmlLink.cs
- ConnectionOrientedTransportChannelFactory.cs
- Point4DValueSerializer.cs
- HtmlContainerControl.cs
- PropertyGridEditorPart.cs
- DbDataSourceEnumerator.cs
- EventSourceCreationData.cs
- SchemaTableColumn.cs
- RijndaelManagedTransform.cs
- CancellableEnumerable.cs
- Font.cs
- LinqExpressionNormalizer.cs
- ToolStripItem.cs
- Font.cs
- SoapExtensionTypeElement.cs
- backend.cs
- TaiwanCalendar.cs
- IPPacketInformation.cs
- BitVector32.cs
- ThemeDirectoryCompiler.cs
- MSAANativeProvider.cs
- ClientConvert.cs
- PolyBezierSegment.cs
- PartialArray.cs
- DataObjectFieldAttribute.cs
- UpdatePanelTrigger.cs
- PassportIdentity.cs
- ConnectionStringsExpressionBuilder.cs
- CopyOfAction.cs
- NumericExpr.cs
- MinimizableAttributeTypeConverter.cs
- ReturnEventArgs.cs
- PerformanceCounter.cs
- EmptyReadOnlyDictionaryInternal.cs
- DataServiceKeyAttribute.cs
- MDIClient.cs
- PlaceHolder.cs
- WebPartConnectionsCloseVerb.cs
- ViewSimplifier.cs
- ObjectToken.cs
- FormView.cs
- ImageCodecInfoPrivate.cs
- FontFamily.cs
- MemberMaps.cs
- CurrentTimeZone.cs
- ColumnMapTranslator.cs
- GetMemberBinder.cs
- ObjectDataSourceView.cs
- MulticastOption.cs
- BadImageFormatException.cs
- TextSegment.cs
- FormCollection.cs
- OracleConnection.cs
- OverloadGroupAttribute.cs
- ImmutableAssemblyCacheEntry.cs
- TemplateControlCodeDomTreeGenerator.cs
- SqlTypeSystemProvider.cs
- CodeTypeDeclarationCollection.cs
- TemplateBuilder.cs
- ResourceContainer.cs
- X509ChainPolicy.cs
- RuleSetBrowserDialog.cs
- WebRequestModuleElement.cs
- CngUIPolicy.cs
- ProfilePropertySettingsCollection.cs
- LinqDataSourceEditData.cs
- CngUIPolicy.cs
- WinInetCache.cs
- TextModifierScope.cs
- XpsFixedPageReaderWriter.cs
- DelayDesigner.cs
- IPAddressCollection.cs
- DateTimePickerDesigner.cs
- SqlDataSourceCommandEventArgs.cs
- RoleManagerEventArgs.cs
- COSERVERINFO.cs
- RijndaelManaged.cs
- SecureConversationSecurityTokenParameters.cs
- FontEditor.cs
- PrintDialogException.cs
- MetadataUtil.cs
- DetailsViewDeleteEventArgs.cs
- XmlAttributeCollection.cs
- ExternalDataExchangeService.cs
- Line.cs
- ResourcesGenerator.cs
- SettingsAttributeDictionary.cs
- InstanceKeyNotReadyException.cs
- FormViewModeEventArgs.cs
- Stylus.cs
- TimeSpan.cs
- ProxyFragment.cs
- COM2ComponentEditor.cs
- BinaryObjectWriter.cs
- CompositionCommandSet.cs
- XAMLParseException.cs