Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Stylus / Stylus.cs / 1 / Stylus.cs
using System; using System.Diagnostics; using System.Security; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The Stylus class represents all stylus devices /// public static class Stylus { ///////////////////////////////////////////////////////////////////// ////// PreviewStylusDown /// public static readonly RoutedEvent PreviewStylusDownEvent = EventManager.RegisterRoutedEvent("PreviewStylusDown", RoutingStrategy.Tunnel, typeof(StylusDownEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusDownHandler(DependencyObject element, StylusDownEventHandler handler) { UIElement.AddHandler(element, PreviewStylusDownEvent, handler); } ////// Removes a handler for the PreviewStylusDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusDownHandler(DependencyObject element, StylusDownEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusDownEvent, handler); } ////// StylusDown /// public static readonly RoutedEvent StylusDownEvent = EventManager.RegisterRoutedEvent("StylusDown", RoutingStrategy.Bubble, typeof(StylusDownEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusDownHandler(DependencyObject element, StylusDownEventHandler handler) { UIElement.AddHandler(element, StylusDownEvent, handler); } ////// Removes a handler for the StylusDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusDownHandler(DependencyObject element, StylusDownEventHandler handler) { UIElement.RemoveHandler(element, StylusDownEvent, handler); } ////// PreviewStylusUp /// public static readonly RoutedEvent PreviewStylusUpEvent = EventManager.RegisterRoutedEvent("PreviewStylusUp", RoutingStrategy.Tunnel, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusUpHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, PreviewStylusUpEvent, handler); } ////// Removes a handler for the PreviewStylusUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusUpHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusUpEvent, handler); } ////// StylusUp /// public static readonly RoutedEvent StylusUpEvent = EventManager.RegisterRoutedEvent("StylusUp", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusUpHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusUpEvent, handler); } ////// Removes a handler for the StylusUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusUpHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusUpEvent, handler); } ////// PreviewStylusMove /// public static readonly RoutedEvent PreviewStylusMoveEvent = EventManager.RegisterRoutedEvent("PreviewStylusMove", RoutingStrategy.Tunnel, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, PreviewStylusMoveEvent, handler); } ////// Removes a handler for the PreviewStylusMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusMoveEvent, handler); } ////// StylusMove /// public static readonly RoutedEvent StylusMoveEvent = EventManager.RegisterRoutedEvent("StylusMove", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusMoveEvent, handler); } ////// Removes a handler for the StylusMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusMoveEvent, handler); } ////// PreviewStylusInAirMove /// public static readonly RoutedEvent PreviewStylusInAirMoveEvent = EventManager.RegisterRoutedEvent("PreviewStylusInAirMove", RoutingStrategy.Tunnel, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusInAirMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusInAirMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, PreviewStylusInAirMoveEvent, handler); } ////// Removes a handler for the PreviewStylusInAirMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusInAirMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusInAirMoveEvent, handler); } ////// StylusInAirMove /// public static readonly RoutedEvent StylusInAirMoveEvent = EventManager.RegisterRoutedEvent("StylusInAirMove", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusInAirMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusInAirMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusInAirMoveEvent, handler); } ////// Removes a handler for the StylusInAirMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusInAirMoveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusInAirMoveEvent, handler); } ////// StylusEnter /// public static readonly RoutedEvent StylusEnterEvent = EventManager.RegisterRoutedEvent("StylusEnter", RoutingStrategy.Direct, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusEnter attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusEnterHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusEnterEvent, handler); } ////// Removes a handler for the StylusEnter attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusEnterHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusEnterEvent, handler); } ////// StylusLeave /// public static readonly RoutedEvent StylusLeaveEvent = EventManager.RegisterRoutedEvent("StylusLeave", RoutingStrategy.Direct, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusLeave attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusLeaveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusLeaveEvent, handler); } ////// Removes a handler for the StylusLeave attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusLeaveHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusLeaveEvent, handler); } ////// PreviewStylusInRange /// public static readonly RoutedEvent PreviewStylusInRangeEvent = EventManager.RegisterRoutedEvent("PreviewStylusInRange", RoutingStrategy.Tunnel, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusInRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusInRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, PreviewStylusInRangeEvent, handler); } ////// Removes a handler for the PreviewStylusInRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusInRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusInRangeEvent, handler); } ////// StylusInRange /// public static readonly RoutedEvent StylusInRangeEvent = EventManager.RegisterRoutedEvent("StylusInRange", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusInRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusInRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusInRangeEvent, handler); } ////// Removes a handler for the StylusInRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusInRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusInRangeEvent, handler); } ////// PreviewStylusOutOfRange /// public static readonly RoutedEvent PreviewStylusOutOfRangeEvent = EventManager.RegisterRoutedEvent("PreviewStylusOutOfRange", RoutingStrategy.Tunnel, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusOutOfRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusOutOfRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, PreviewStylusOutOfRangeEvent, handler); } ////// Removes a handler for the PreviewStylusOutOfRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusOutOfRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusOutOfRangeEvent, handler); } ////// StylusOutOfRange /// public static readonly RoutedEvent StylusOutOfRangeEvent = EventManager.RegisterRoutedEvent("StylusOutOfRange", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusOutOfRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusOutOfRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, StylusOutOfRangeEvent, handler); } ////// Removes a handler for the StylusOutOfRange attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusOutOfRangeHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, StylusOutOfRangeEvent, handler); } ////// PreviewStylusSystemGesture /// public static readonly RoutedEvent PreviewStylusSystemGestureEvent = EventManager.RegisterRoutedEvent("PreviewStylusSystemGesture", RoutingStrategy.Tunnel, typeof(StylusSystemGestureEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusSystemGesture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusSystemGestureHandler(DependencyObject element, StylusSystemGestureEventHandler handler) { UIElement.AddHandler(element, PreviewStylusSystemGestureEvent, handler); } ////// Removes a handler for the PreviewStylusSystemGesture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusSystemGestureHandler(DependencyObject element, StylusSystemGestureEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusSystemGestureEvent, handler); } ////// StylusSystemGesture /// public static readonly RoutedEvent StylusSystemGestureEvent = EventManager.RegisterRoutedEvent("StylusSystemGesture", RoutingStrategy.Bubble, typeof(StylusSystemGestureEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusSystemGesture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusSystemGestureHandler(DependencyObject element, StylusSystemGestureEventHandler handler) { UIElement.AddHandler(element, StylusSystemGestureEvent, handler); } ////// Removes a handler for the StylusSystemGesture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusSystemGestureHandler(DependencyObject element, StylusSystemGestureEventHandler handler) { UIElement.RemoveHandler(element, StylusSystemGestureEvent, handler); } ////// GotStylusCapture /// public static readonly RoutedEvent GotStylusCaptureEvent = EventManager.RegisterRoutedEvent("GotStylusCapture", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the GotStylusCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddGotStylusCaptureHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, GotStylusCaptureEvent, handler); } ////// Removes a handler for the GotStylusCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveGotStylusCaptureHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, GotStylusCaptureEvent, handler); } ////// LostStylusCapture /// public static readonly RoutedEvent LostStylusCaptureEvent = EventManager.RegisterRoutedEvent("LostStylusCapture", RoutingStrategy.Bubble, typeof(StylusEventHandler), typeof(Stylus)); ////// Adds a handler for the LostStylusCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddLostStylusCaptureHandler(DependencyObject element, StylusEventHandler handler) { UIElement.AddHandler(element, LostStylusCaptureEvent, handler); } ////// Removes a handler for the LostStylusCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveLostStylusCaptureHandler(DependencyObject element, StylusEventHandler handler) { UIElement.RemoveHandler(element, LostStylusCaptureEvent, handler); } ////// StylusButtonDown /// public static readonly RoutedEvent StylusButtonDownEvent = EventManager.RegisterRoutedEvent("StylusButtonDown", RoutingStrategy.Bubble, typeof(StylusButtonEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusButtonDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusButtonDownHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.AddHandler(element, StylusButtonDownEvent, handler); } ////// Removes a handler for the StylusButtonDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusButtonDownHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.RemoveHandler(element, StylusButtonDownEvent, handler); } ////// StylusButtonUp /// public static readonly RoutedEvent StylusButtonUpEvent = EventManager.RegisterRoutedEvent("StylusButtonUp", RoutingStrategy.Bubble, typeof(StylusButtonEventHandler), typeof(Stylus)); ////// Adds a handler for the StylusButtonUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddStylusButtonUpHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.AddHandler(element, StylusButtonUpEvent, handler); } ////// Removes a handler for the StylusButtonUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveStylusButtonUpHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.RemoveHandler(element, StylusButtonUpEvent, handler); } ////// PreviewStylusButtonDown /// public static readonly RoutedEvent PreviewStylusButtonDownEvent = EventManager.RegisterRoutedEvent("PreviewStylusButtonDown", RoutingStrategy.Tunnel, typeof(StylusButtonEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusButtonDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusButtonDownHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.AddHandler(element, PreviewStylusButtonDownEvent, handler); } ////// Removes a handler for the PreviewStylusButtonDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusButtonDownHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusButtonDownEvent, handler); } ////// PreviewStylusButtonUp /// public static readonly RoutedEvent PreviewStylusButtonUpEvent = EventManager.RegisterRoutedEvent("PreviewStylusButtonUp", RoutingStrategy.Tunnel, typeof(StylusButtonEventHandler), typeof(Stylus)); ////// Adds a handler for the PreviewStylusButtonUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewStylusButtonUpHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.AddHandler(element, PreviewStylusButtonUpEvent, handler); } ////// Removes a handler for the PreviewStylusButtonUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewStylusButtonUpHandler(DependencyObject element, StylusButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewStylusButtonUpEvent, handler); } ////// Reads the attached property IsPressAndHoldEnabled from the given element. /// /// The element from which to read the attached property. ///The property's value. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsPressAndHoldEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } object boolValue = element.GetValue(IsPressAndHoldEnabledProperty); if (boolValue == null) return false; // If we don't find property then return false. else return (bool)boolValue; } /// /// Writes the attached property IsPressAndHoldEnabled to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetIsPressAndHoldEnabled(DependencyObject element, bool enabled) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsPressAndHoldEnabledProperty, enabled); } /// /// DependencyProperty for IsPressAndHoldEnabled property. /// ////// public static readonly DependencyProperty IsPressAndHoldEnabledProperty = DependencyProperty.RegisterAttached("IsPressAndHoldEnabled", typeof(bool), typeof(Stylus), new PropertyMetadata(true)); // note we can't specify inherits in frameworkcore. new FrameworkPropertyMetadata(true, FrameworkPropertyMetadataOptions.Inherits) /// /// Reads the attached property IsFlicksEnabled from the given element. /// /// The element from which to read the attached property. ///The property's value. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsFlicksEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } object boolValue = element.GetValue(IsFlicksEnabledProperty); if (boolValue == null) return false; // If we don't find property then return false. else return (bool)boolValue; } /// /// Writes the attached property IsFlicksEnabled to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetIsFlicksEnabled(DependencyObject element, bool enabled) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsFlicksEnabledProperty, enabled); } /// /// DependencyProperty for IsFlicksEnabled property. /// ////// public static readonly DependencyProperty IsFlicksEnabledProperty = DependencyProperty.RegisterAttached("IsFlicksEnabled", typeof(bool), typeof(Stylus), new PropertyMetadata(true)); // note we can't specify inherits in frameworkcore. new FrameworkPropertyMetadata(true, FrameworkPropertyMetadataOptions.Inherits) /// /// Reads the attached property IsTapFeedbackEnabled from the given element. /// /// The element from which to read the attached property. ///The property's value. ///[AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsTapFeedbackEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } object boolValue = element.GetValue(IsTapFeedbackEnabledProperty); if (boolValue == null) return false; // If we don't find property then return false. else return (bool)boolValue; } /// /// Writes the attached property IsTapFeedbackEnabled to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetIsTapFeedbackEnabled(DependencyObject element, bool enabled) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsTapFeedbackEnabledProperty, enabled); } /// /// DependencyProperty for IsTapFeedbackEnabled property. /// ////// public static readonly DependencyProperty IsTapFeedbackEnabledProperty = DependencyProperty.RegisterAttached("IsTapFeedbackEnabled", typeof(bool), typeof(Stylus), new PropertyMetadata(true)); // note we can't specify inherits in frameworkcore. new FrameworkPropertyMetadata(true, FrameworkPropertyMetadataOptions.Inherits) /// /// Reads the attached property IsTouchFeedbackEnabled from the given element. /// /// The element from which to read the attached property. ///The property's value. ///public static bool GetIsTouchFeedbackEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } object boolValue = element.GetValue(IsTouchFeedbackEnabledProperty); if (boolValue == null) return false; // If we don't find property then return false. else return (bool)boolValue; } /// /// Writes the attached property IsTouchFeedbackEnabled to the given element. /// /// The element to which to write the attached property. /// The property value to set ///public static void SetIsTouchFeedbackEnabled(DependencyObject element, bool enabled) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsTouchFeedbackEnabledProperty, enabled); } /// /// DependencyProperty for IsTouchFeedbackEnabled property. /// ////// public static readonly DependencyProperty IsTouchFeedbackEnabledProperty = DependencyProperty.RegisterAttached("IsTouchFeedbackEnabled", typeof(bool), typeof(Stylus), new PropertyMetadata(true)); // note we can't specify inherits in frameworkcore. new FrameworkPropertyMetadata(true, FrameworkPropertyMetadataOptions.Inherits) ///////////////////////////////////////////////////////////////////// /// /// Returns the element that the stylus is over. /// public static IInputElement DirectlyOver { get { StylusDevice stylusDevice = Stylus.CurrentStylusDevice; if (stylusDevice == null) return null; return stylusDevice.DirectlyOver; } } ///////////////////////////////////////////////////////////////////// ////// Returns the element that has captured the stylus. /// public static IInputElement Captured { get { StylusDevice stylusDevice = Stylus.CurrentStylusDevice; if (stylusDevice == null) { return Mouse.Captured; } return stylusDevice.Captured; } } ///////////////////////////////////////////////////////////////////// ////// Captures the stylus to a particular element. /// /// /// The element to capture the stylus to. /// public static bool Capture(IInputElement element) { return Capture(element, CaptureMode.Element); } ///////////////////////////////////////////////////////////////////// ////// Captures the stylus to a particular element. /// /// /// The element to capture the stylus to. /// /// /// The kind of capture to acquire. /// public static bool Capture(IInputElement element, CaptureMode captureMode) { // The stylus code watches mouse capture changes and it will trigger us to // sync up all the stylusdevice capture settings to be the same as the mouse. // So we just call Mouse.Capture() here to trigger this all to happen. return Mouse.Capture(element, captureMode); } ////// Forces the sytlus to resynchronize. /// public static void Synchronize() { StylusDevice curDevice = Stylus.CurrentStylusDevice; if (null != curDevice) { curDevice.Synchronize(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] /// ////// Critical - calls SecurityCritical code StylusLogic.CurrentStylusLogic /// PublicOK: Used to get the currently active StylusDevice which is safe to expose. /// this is safe as: /// Takes no input and returns no security critical data. /// public static StylusDevice CurrentStylusDevice { [SecurityCritical] get { return StylusLogic.CurrentStylusLogic.CurrentStylusDevice; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
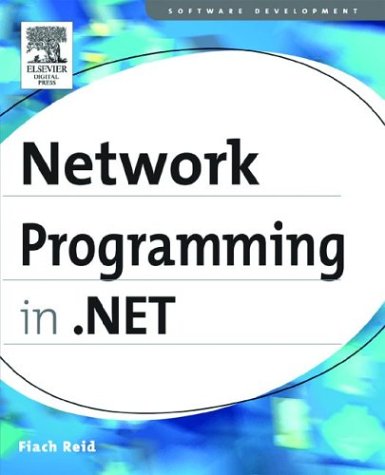
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerActionPropertyItem.cs
- EncryptedPackage.cs
- SingleTagSectionHandler.cs
- ByteStream.cs
- DragSelectionMessageFilter.cs
- ResourcePool.cs
- FactoryGenerator.cs
- AnnouncementService.cs
- NotCondition.cs
- CollectionsUtil.cs
- DependencyObjectType.cs
- UnionCodeGroup.cs
- TextServicesCompartment.cs
- AttributeTableBuilder.cs
- FrameworkContentElementAutomationPeer.cs
- ReferentialConstraint.cs
- XmlStringTable.cs
- SecurityException.cs
- WpfGeneratedKnownProperties.cs
- Types.cs
- RequestResizeEvent.cs
- PermissionListSet.cs
- RuntimeArgumentHandle.cs
- Action.cs
- FlowDocumentFormatter.cs
- WindowsToolbar.cs
- PointAnimation.cs
- TextSelectionHelper.cs
- FieldMetadata.cs
- QilStrConcat.cs
- SystemWebExtensionsSectionGroup.cs
- PolyLineSegment.cs
- TableItemStyle.cs
- CodeCommentStatement.cs
- SharedStatics.cs
- StreamGeometryContext.cs
- Mutex.cs
- XmlChoiceIdentifierAttribute.cs
- FrameworkName.cs
- _DigestClient.cs
- TextElement.cs
- SortableBindingList.cs
- SortAction.cs
- HtmlTernaryTree.cs
- XPathNodePointer.cs
- RtfControls.cs
- StringSource.cs
- PolicyValidator.cs
- AssertSection.cs
- XmlReflectionImporter.cs
- LineGeometry.cs
- RealProxy.cs
- HtmlControlPersistable.cs
- SecurityTokenValidationException.cs
- RotateTransform.cs
- UrlUtility.cs
- MarkupExtensionReturnTypeAttribute.cs
- CodeSnippetExpression.cs
- IRCollection.cs
- DiscoveryServerProtocol.cs
- ModelItemImpl.cs
- UrlMappingsModule.cs
- ContextMenuStrip.cs
- KeysConverter.cs
- ScrollBarRenderer.cs
- Variable.cs
- HttpChannelListener.cs
- ReservationCollection.cs
- CompleteWizardStep.cs
- ProtocolsSection.cs
- WsatRegistrationHeader.cs
- ShellProvider.cs
- Expressions.cs
- TreeViewBindingsEditorForm.cs
- InProcStateClientManager.cs
- HtmlTextViewAdapter.cs
- BitmapEffectDrawingContextState.cs
- SrgsElement.cs
- Helper.cs
- HtmlInputReset.cs
- ActivityLocationReferenceEnvironment.cs
- Classification.cs
- _SafeNetHandles.cs
- SQLDoubleStorage.cs
- VisualStateManager.cs
- FilterableAttribute.cs
- KnownBoxes.cs
- Util.cs
- MeasurementDCInfo.cs
- RepeaterCommandEventArgs.cs
- RawStylusSystemGestureInputReport.cs
- ObjectDisposedException.cs
- XmlDataLoader.cs
- TableColumnCollectionInternal.cs
- Inline.cs
- Synchronization.cs
- EnterpriseServicesHelper.cs
- CompositeDuplexBindingElementImporter.cs
- ExtractorMetadata.cs
- WindowsNonControl.cs