Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OracleConnection.cs / 1 / OracleConnection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Threading; using System.Text; using System.IO; using System.Configuration; internal enum TransactionState { AutoCommit, // We're currently in autocommit mode LocalStarted, // Local transaction has been started, but not committed GlobalStarted, // We're in a distributed (MTS) transaction }; //--------------------------------------------------------------------- // OracleConnection // // Implements the Oracle Connection object, which connects // to the Oracle server // [ DefaultEvent("InfoMessage") ] sealed public partial class OracleConnection : DbConnection, ICloneable { static private readonly object EventInfoMessage = new object(); // Construct from a connection string public OracleConnection( String connectionString ) : this () { ConnectionString = connectionString; } // (internal) Construct from an existing Connection object (copy constructor) internal OracleConnection( OracleConnection connection ) : this() { CopyFrom(connection); } [ ResCategoryAttribute(Res.OracleCategory_Data), DefaultValue(""), RecommendedAsConfigurable(true), RefreshProperties(RefreshProperties.All), ResDescriptionAttribute(Res.OracleConnection_ConnectionString), Editor("Microsoft.VSDesigner.Data.Oracle.Design.OracleConnectionStringEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] override public string ConnectionString { get { return ConnectionString_Get(); } set { ConnectionString_Set(value); } } [ Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never) ] override public int ConnectionTimeout { // Oracle has no discernable or settable connection timeouts, so this // is being hidden from the user and we always return zero. (We have // to implement this because IDbConnection requires it) get { return 0; } } [ Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never) ] override public string Database { // Oracle has no notion of a "database", so this is being hidden from // the user and we always returns the empty string. (We have to implement // this because IDbConnection requires it) get { return String.Empty; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.OracleConnection_DataSource), ] override public string DataSource { get { OracleConnectionString connectionOptions = (OracleConnectionString)ConnectionOptions; string value = String.Empty; if (null != connectionOptions) value = connectionOptions.DataSource; return value; } } internal OciEnvironmentHandle EnvironmentHandle { get { return GetOpenInternalConnection().EnvironmentHandle; } } internal OciErrorHandle ErrorHandle { get { return GetOpenInternalConnection().ErrorHandle; } } internal bool HasTransaction { get { return GetOpenInternalConnection().HasTransaction; } } internal TimeSpan ServerTimeZoneAdjustmentToUTC { get { return GetOpenInternalConnection().GetServerTimeZoneAdjustmentToUTC(this); } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.OracleConnection_ServerVersion) ] override public string ServerVersion { get { return GetOpenInternalConnection().ServerVersion; } } internal bool ServerVersionAtLeastOracle8 { get { return GetOpenInternalConnection().ServerVersionAtLeastOracle8; } } internal bool ServerVersionAtLeastOracle9i { get { return GetOpenInternalConnection().ServerVersionAtLeastOracle9i; } } internal OciServiceContextHandle ServiceContextHandle { get { return GetOpenInternalConnection().ServiceContextHandle; } } internal OracleTransaction Transaction { get { return GetOpenInternalConnection().Transaction; } } internal TransactionState TransactionState { get { return GetOpenInternalConnection().TransactionState; } set { GetOpenInternalConnection().TransactionState = value; } } internal bool UnicodeEnabled { get { return GetOpenInternalConnection().UnicodeEnabled; } } new public OracleTransaction BeginTransaction() { return BeginTransaction(IsolationLevel.Unspecified); } new public OracleTransaction BeginTransaction(IsolationLevel il) { return (OracleTransaction)base.BeginTransaction(il); } override public void ChangeDatabase(String value) { IntPtr hscp; Bid.ScopeEnter(out hscp, "%d#, value='%ls'\n", ObjectID, value); try { // Oracle has no notion of a "database", so this is being hidden from // the user and we always throw. (We have to implement this because // IDbConnection requires it) throw ADP.ChangeDatabaseNotSupported(); } finally { Bid.ScopeLeave(ref hscp); } } internal void CheckError(OciErrorHandle errorHandle, int rc) { // Check the return code and perform the appropriate handling; either // throwing the exception or posting a warning message. switch ((OCI.RETURNCODE)rc) { case OCI.RETURNCODE.OCI_ERROR: // -1: Bad programming by the customer. case OCI.RETURNCODE.OCI_NO_DATA: //100: Bad programming by the customer/expected error. Exception exceptionToThrow = ADP.OracleError(errorHandle, rc); // If this connection is broken, make sure we note that fact and prevent it from being reused. if (null != errorHandle && errorHandle.ConnectionIsBroken) { OracleInternalConnection openConnection = GetOpenInternalConnection(); if (null != openConnection) { openConnection.ConnectionIsBroken(); // } } throw exceptionToThrow; case OCI.RETURNCODE.OCI_INVALID_HANDLE: // -2: Bad programming on my part. throw ADP.InvalidOperation(Res.GetString(Res.ADP_InternalError, rc)); case OCI.RETURNCODE.OCI_SUCCESS_WITH_INFO: // 1: Information Message OracleException infoMessage = OracleException.CreateException(errorHandle, rc); OracleInfoMessageEventArgs infoMessageEvent = new OracleInfoMessageEventArgs(infoMessage); OnInfoMessage(infoMessageEvent); break; default: if (rc < 0 || rc == (int)OCI.RETURNCODE.OCI_NEED_DATA) throw ADP.Simple(Res.GetString(Res.ADP_UnexpectedReturnCode, rc.ToString(CultureInfo.CurrentCulture))); Debug.Assert(false, "Unexpected return code: " + rc); // shouldn't be here for any reason. break; } } static public void ClearAllPools() { (new OraclePermission(System.Security.Permissions.PermissionState.Unrestricted)).Demand(); OracleConnectionFactory.SingletonInstance.ClearAllPools(); } static public void ClearPool(OracleConnection connection) { ADP.CheckArgumentNull(connection, "connection"); DbConnectionOptions connectionOptions = connection.UserConnectionOptions; if (null != connectionOptions) { connectionOptions.DemandPermission(); OracleConnectionFactory.SingletonInstance.ClearPool(connection); } } object ICloneable.Clone() { // We provide a clone that is closed; it would be very bad to hand out the // same handles again; instead they can do their own open and get their // own handles. OracleConnection clone = new OracleConnection(this); Bid.Trace(" %d#, clone=%d#\n", ObjectID, clone.ObjectID); return clone; } override public void Close() { InnerConnection.CloseConnection(this, ConnectionFactory); // does not require GC.KeepAlive(this) because of OnStateChange } internal void Commit() { GetOpenInternalConnection().Commit(); } new public OracleCommand CreateCommand() { OracleCommand cmd = new OracleCommand(); cmd.Connection = this; return cmd; } private void DisposeMe(bool disposing) { // MDAC 65459 } public void EnlistDistributedTransaction(System.EnterpriseServices.ITransaction distributedTransaction) { EnlistDistributedTransactionHelper(distributedTransaction); } internal byte[] GetBytes(string value, bool useNationalCharacterSet) { return GetOpenInternalConnection().GetBytes(value, useNationalCharacterSet); } internal OracleInternalConnection GetOpenInternalConnection() { DbConnectionInternal internalConnection = this.InnerConnection; if (internalConnection is OracleInternalConnection) return internalConnection as OracleInternalConnection; throw ADP.ClosedConnectionError(); } internal NativeBuffer GetScratchBuffer(int minSize) { return GetOpenInternalConnection().GetScratchBuffer(minSize); } internal string GetString(byte[] bytearray) { return GetOpenInternalConnection().GetString(bytearray); } internal string GetString(byte[] bytearray, bool useNationalCharacterSet) { return GetOpenInternalConnection().GetString(bytearray, useNationalCharacterSet); } override public void Open() { InnerConnection.OpenConnection(this, ConnectionFactory); OracleInternalConnection internalConnection = (InnerConnection as OracleInternalConnection); if (null != internalConnection) { internalConnection.FireDeferredInfoMessageEvents(this); } } internal void Rollback() { OracleInternalConnection internalConnection = InnerConnection as OracleInternalConnection; if (null != internalConnection) { internalConnection.Rollback(); } } internal void RollbackDeadTransaction() { GetOpenInternalConnection().RollbackDeadTransaction(); } [ ResCategoryAttribute(Res.OracleCategory_InfoMessage), ResDescriptionAttribute(Res.OracleConnection_InfoMessage) ] public event OracleInfoMessageEventHandler InfoMessage { add { Events.AddHandler(EventInfoMessage, value); } remove { Events.RemoveHandler(EventInfoMessage, value); } } internal void OnInfoMessage(OracleInfoMessageEventArgs infoMessageEvent) { OracleInfoMessageEventHandler handler = (OracleInfoMessageEventHandler) Events[EventInfoMessage]; if (null != handler) { handler(this, infoMessageEvent); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Security; using System.Threading; using System.Text; using System.IO; using System.Configuration; internal enum TransactionState { AutoCommit, // We're currently in autocommit mode LocalStarted, // Local transaction has been started, but not committed GlobalStarted, // We're in a distributed (MTS) transaction }; //--------------------------------------------------------------------- // OracleConnection // // Implements the Oracle Connection object, which connects // to the Oracle server // [ DefaultEvent("InfoMessage") ] sealed public partial class OracleConnection : DbConnection, ICloneable { static private readonly object EventInfoMessage = new object(); // Construct from a connection string public OracleConnection( String connectionString ) : this () { ConnectionString = connectionString; } // (internal) Construct from an existing Connection object (copy constructor) internal OracleConnection( OracleConnection connection ) : this() { CopyFrom(connection); } [ ResCategoryAttribute(Res.OracleCategory_Data), DefaultValue(""), RecommendedAsConfigurable(true), RefreshProperties(RefreshProperties.All), ResDescriptionAttribute(Res.OracleConnection_ConnectionString), Editor("Microsoft.VSDesigner.Data.Oracle.Design.OracleConnectionStringEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] override public string ConnectionString { get { return ConnectionString_Get(); } set { ConnectionString_Set(value); } } [ Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never) ] override public int ConnectionTimeout { // Oracle has no discernable or settable connection timeouts, so this // is being hidden from the user and we always return zero. (We have // to implement this because IDbConnection requires it) get { return 0; } } [ Browsable(false), EditorBrowsableAttribute(EditorBrowsableState.Never) ] override public string Database { // Oracle has no notion of a "database", so this is being hidden from // the user and we always returns the empty string. (We have to implement // this because IDbConnection requires it) get { return String.Empty; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.OracleConnection_DataSource), ] override public string DataSource { get { OracleConnectionString connectionOptions = (OracleConnectionString)ConnectionOptions; string value = String.Empty; if (null != connectionOptions) value = connectionOptions.DataSource; return value; } } internal OciEnvironmentHandle EnvironmentHandle { get { return GetOpenInternalConnection().EnvironmentHandle; } } internal OciErrorHandle ErrorHandle { get { return GetOpenInternalConnection().ErrorHandle; } } internal bool HasTransaction { get { return GetOpenInternalConnection().HasTransaction; } } internal TimeSpan ServerTimeZoneAdjustmentToUTC { get { return GetOpenInternalConnection().GetServerTimeZoneAdjustmentToUTC(this); } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ResDescriptionAttribute(Res.OracleConnection_ServerVersion) ] override public string ServerVersion { get { return GetOpenInternalConnection().ServerVersion; } } internal bool ServerVersionAtLeastOracle8 { get { return GetOpenInternalConnection().ServerVersionAtLeastOracle8; } } internal bool ServerVersionAtLeastOracle9i { get { return GetOpenInternalConnection().ServerVersionAtLeastOracle9i; } } internal OciServiceContextHandle ServiceContextHandle { get { return GetOpenInternalConnection().ServiceContextHandle; } } internal OracleTransaction Transaction { get { return GetOpenInternalConnection().Transaction; } } internal TransactionState TransactionState { get { return GetOpenInternalConnection().TransactionState; } set { GetOpenInternalConnection().TransactionState = value; } } internal bool UnicodeEnabled { get { return GetOpenInternalConnection().UnicodeEnabled; } } new public OracleTransaction BeginTransaction() { return BeginTransaction(IsolationLevel.Unspecified); } new public OracleTransaction BeginTransaction(IsolationLevel il) { return (OracleTransaction)base.BeginTransaction(il); } override public void ChangeDatabase(String value) { IntPtr hscp; Bid.ScopeEnter(out hscp, "%d#, value='%ls'\n", ObjectID, value); try { // Oracle has no notion of a "database", so this is being hidden from // the user and we always throw. (We have to implement this because // IDbConnection requires it) throw ADP.ChangeDatabaseNotSupported(); } finally { Bid.ScopeLeave(ref hscp); } } internal void CheckError(OciErrorHandle errorHandle, int rc) { // Check the return code and perform the appropriate handling; either // throwing the exception or posting a warning message. switch ((OCI.RETURNCODE)rc) { case OCI.RETURNCODE.OCI_ERROR: // -1: Bad programming by the customer. case OCI.RETURNCODE.OCI_NO_DATA: //100: Bad programming by the customer/expected error. Exception exceptionToThrow = ADP.OracleError(errorHandle, rc); // If this connection is broken, make sure we note that fact and prevent it from being reused. if (null != errorHandle && errorHandle.ConnectionIsBroken) { OracleInternalConnection openConnection = GetOpenInternalConnection(); if (null != openConnection) { openConnection.ConnectionIsBroken(); // } } throw exceptionToThrow; case OCI.RETURNCODE.OCI_INVALID_HANDLE: // -2: Bad programming on my part. throw ADP.InvalidOperation(Res.GetString(Res.ADP_InternalError, rc)); case OCI.RETURNCODE.OCI_SUCCESS_WITH_INFO: // 1: Information Message OracleException infoMessage = OracleException.CreateException(errorHandle, rc); OracleInfoMessageEventArgs infoMessageEvent = new OracleInfoMessageEventArgs(infoMessage); OnInfoMessage(infoMessageEvent); break; default: if (rc < 0 || rc == (int)OCI.RETURNCODE.OCI_NEED_DATA) throw ADP.Simple(Res.GetString(Res.ADP_UnexpectedReturnCode, rc.ToString(CultureInfo.CurrentCulture))); Debug.Assert(false, "Unexpected return code: " + rc); // shouldn't be here for any reason. break; } } static public void ClearAllPools() { (new OraclePermission(System.Security.Permissions.PermissionState.Unrestricted)).Demand(); OracleConnectionFactory.SingletonInstance.ClearAllPools(); } static public void ClearPool(OracleConnection connection) { ADP.CheckArgumentNull(connection, "connection"); DbConnectionOptions connectionOptions = connection.UserConnectionOptions; if (null != connectionOptions) { connectionOptions.DemandPermission(); OracleConnectionFactory.SingletonInstance.ClearPool(connection); } } object ICloneable.Clone() { // We provide a clone that is closed; it would be very bad to hand out the // same handles again; instead they can do their own open and get their // own handles. OracleConnection clone = new OracleConnection(this); Bid.Trace(" %d#, clone=%d#\n", ObjectID, clone.ObjectID); return clone; } override public void Close() { InnerConnection.CloseConnection(this, ConnectionFactory); // does not require GC.KeepAlive(this) because of OnStateChange } internal void Commit() { GetOpenInternalConnection().Commit(); } new public OracleCommand CreateCommand() { OracleCommand cmd = new OracleCommand(); cmd.Connection = this; return cmd; } private void DisposeMe(bool disposing) { // MDAC 65459 } public void EnlistDistributedTransaction(System.EnterpriseServices.ITransaction distributedTransaction) { EnlistDistributedTransactionHelper(distributedTransaction); } internal byte[] GetBytes(string value, bool useNationalCharacterSet) { return GetOpenInternalConnection().GetBytes(value, useNationalCharacterSet); } internal OracleInternalConnection GetOpenInternalConnection() { DbConnectionInternal internalConnection = this.InnerConnection; if (internalConnection is OracleInternalConnection) return internalConnection as OracleInternalConnection; throw ADP.ClosedConnectionError(); } internal NativeBuffer GetScratchBuffer(int minSize) { return GetOpenInternalConnection().GetScratchBuffer(minSize); } internal string GetString(byte[] bytearray) { return GetOpenInternalConnection().GetString(bytearray); } internal string GetString(byte[] bytearray, bool useNationalCharacterSet) { return GetOpenInternalConnection().GetString(bytearray, useNationalCharacterSet); } override public void Open() { InnerConnection.OpenConnection(this, ConnectionFactory); OracleInternalConnection internalConnection = (InnerConnection as OracleInternalConnection); if (null != internalConnection) { internalConnection.FireDeferredInfoMessageEvents(this); } } internal void Rollback() { OracleInternalConnection internalConnection = InnerConnection as OracleInternalConnection; if (null != internalConnection) { internalConnection.Rollback(); } } internal void RollbackDeadTransaction() { GetOpenInternalConnection().RollbackDeadTransaction(); } [ ResCategoryAttribute(Res.OracleCategory_InfoMessage), ResDescriptionAttribute(Res.OracleConnection_InfoMessage) ] public event OracleInfoMessageEventHandler InfoMessage { add { Events.AddHandler(EventInfoMessage, value); } remove { Events.RemoveHandler(EventInfoMessage, value); } } internal void OnInfoMessage(OracleInfoMessageEventArgs infoMessageEvent) { OracleInfoMessageEventHandler handler = (OracleInfoMessageEventHandler) Events[EventInfoMessage]; if (null != handler) { handler(this, infoMessageEvent); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
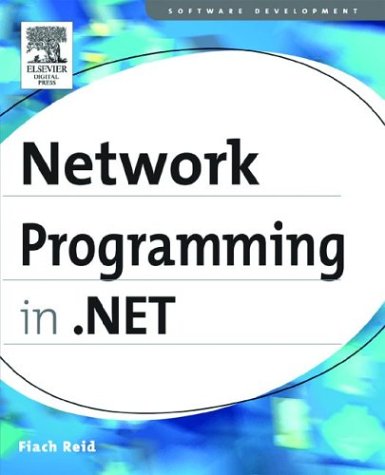
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeReversePInvokeHandle.cs
- IconEditor.cs
- CustomDictionarySources.cs
- EnterpriseServicesHelper.cs
- TemplateBamlTreeBuilder.cs
- RootProfilePropertySettingsCollection.cs
- GatewayIPAddressInformationCollection.cs
- GeometryDrawing.cs
- IdleTimeoutMonitor.cs
- AsymmetricKeyExchangeFormatter.cs
- AVElementHelper.cs
- coordinator.cs
- SafeUserTokenHandle.cs
- SqlProfileProvider.cs
- InlinedAggregationOperatorEnumerator.cs
- ProfileSettingsCollection.cs
- UpdateProgress.cs
- InkCanvasInnerCanvas.cs
- safemediahandle.cs
- SqlError.cs
- EmptyEnumerable.cs
- Evaluator.cs
- StreamWithDictionary.cs
- SemanticAnalyzer.cs
- PnrpPeerResolver.cs
- HwndSourceKeyboardInputSite.cs
- ActivityExecutionFilter.cs
- LowerCaseStringConverter.cs
- ItemsControl.cs
- BordersPage.cs
- CodeExpressionStatement.cs
- ListBoxItem.cs
- DateTimeOffset.cs
- FontDialog.cs
- cookieexception.cs
- DocumentOutline.cs
- PolygonHotSpot.cs
- ServerValidateEventArgs.cs
- RolePrincipal.cs
- ParagraphVisual.cs
- DictionaryBase.cs
- HitTestParameters3D.cs
- AddressHeader.cs
- TextEditorThreadLocalStore.cs
- SqlGatherProducedAliases.cs
- LinearQuaternionKeyFrame.cs
- CompilerGeneratedAttribute.cs
- LayoutTable.cs
- ConfigsHelper.cs
- EndpointFilterProvider.cs
- SettingsPropertyNotFoundException.cs
- InsufficientExecutionStackException.cs
- PenLineCapValidation.cs
- Listbox.cs
- WebPartConnection.cs
- _SslState.cs
- UInt32.cs
- PropertyPushdownHelper.cs
- DataGridPageChangedEventArgs.cs
- InstanceBehavior.cs
- TypeBuilderInstantiation.cs
- HelpProvider.cs
- _SecureChannel.cs
- TypeViewSchema.cs
- DataControlFieldHeaderCell.cs
- MD5CryptoServiceProvider.cs
- HttpNamespaceReservationInstallComponent.cs
- FormViewDeleteEventArgs.cs
- MenuAdapter.cs
- ListenerAdapterBase.cs
- MappingMetadataHelper.cs
- Intellisense.cs
- WeakReferenceList.cs
- SafeNativeMethodsMilCoreApi.cs
- DataTableReaderListener.cs
- ProfessionalColorTable.cs
- WebPartActionVerb.cs
- QueryExpr.cs
- CodeExpressionStatement.cs
- BypassElement.cs
- CodeDomLocalizationProvider.cs
- FindProgressChangedEventArgs.cs
- Int32Collection.cs
- GetWinFXPath.cs
- SessionParameter.cs
- OleDbDataReader.cs
- ButtonRenderer.cs
- EncodingTable.cs
- XmlSchemaSimpleTypeUnion.cs
- _AutoWebProxyScriptWrapper.cs
- RequestQueryProcessor.cs
- TypeConverter.cs
- _SSPIWrapper.cs
- ProviderUtil.cs
- RecipientInfo.cs
- ScriptResourceDefinition.cs
- ErrorHandler.cs
- DataGridViewToolTip.cs
- ArraySegment.cs
- StringCollection.cs