Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / Listbox.cs / 1305376 / Listbox.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI; ////// [ ValidationProperty("SelectedItem"), SupportsEventValidation ] public class ListBox : ListControl, IPostBackDataHandler { ///Constructs a list box and defines its /// properties. ////// public ListBox() { } ///Initializes a new instance of the ///class. /// [ Browsable(false) ] public override Color BorderColor { get { return base.BorderColor; } set { base.BorderColor = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override Unit BorderWidth { get { return base.BorderWidth; } set { base.BorderWidth = value; } } internal override bool IsMultiSelectInternal { get { return SelectionMode == ListSelectionMode.Multiple; } } ///[To be supplied.] ////// [ WebCategory("Appearance"), DefaultValue(4), WebSysDescription(SR.ListBox_Rows) ] public virtual int Rows { get { object n = ViewState["Rows"]; return((n == null) ? 4 : (int)n); } set { if (value < 1) { throw new ArgumentOutOfRangeException("value"); } ViewState["Rows"] = value; } } ///Gets or /// sets the display height (in rows) of the list box. ////// [ WebCategory("Behavior"), DefaultValue(ListSelectionMode.Single), WebSysDescription(SR.ListBox_SelectionMode) ] public virtual ListSelectionMode SelectionMode { get { object sm = ViewState["SelectionMode"]; return((sm == null) ? ListSelectionMode.Single : (ListSelectionMode)sm); } set { if (value < ListSelectionMode.Single || value > ListSelectionMode.Multiple) { throw new ArgumentOutOfRangeException("value"); } ViewState["SelectionMode"] = value; } } ///Gets or sets /// the selection behavior of the list box. ///protected override void AddAttributesToRender(HtmlTextWriter writer) { writer.AddAttribute(HtmlTextWriterAttribute.Size, Rows.ToString(NumberFormatInfo.InvariantInfo)); string uniqueID = UniqueID; if (uniqueID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } base.AddAttributesToRender(writer); } public virtual int[] GetSelectedIndices() { return (int[])SelectedIndicesInternal.ToArray(typeof(int)); } /// /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && SelectionMode == ListSelectionMode.Multiple && Enabled) { // ensure postback when no item is selected Page.RegisterRequiresPostBack(this); } } ////// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Loads the posted content of the list control if it is different from the last /// posting. ////// /// protected virtual bool LoadPostData(String postDataKey, NameValueCollection postCollection) { if (IsEnabled == false) { // When a ListBox is disabled, then there is no postback // data for it. Any checked state information has been loaded // via view state. return false; } string[] selectedItems = postCollection.GetValues(postDataKey); bool selectionChanged = false; EnsureDataBound(); if (selectedItems != null) { if (SelectionMode == ListSelectionMode.Single) { ValidateEvent(postDataKey, selectedItems[0]); int n = Items.FindByValueInternal(selectedItems[0], false); if (SelectedIndex != n) { SetPostDataSelection(n); selectionChanged = true; } } else { // multiple selection int count = selectedItems.Length; ArrayList oldSelectedIndices = SelectedIndicesInternal; ArrayList newSelectedIndices = new ArrayList(count); for (int i=0; i < count; i++) { ValidateEvent(postDataKey, selectedItems[i]); // create array of new indices from posted values newSelectedIndices.Add(Items.FindByValueInternal(selectedItems[i], false)); } int oldcount = 0; if (oldSelectedIndices != null) oldcount = oldSelectedIndices.Count; if (oldcount == count) { // check new indices against old indices // assumes selected values are posted in order for (int i=0; i < count; i++) { if (((int)newSelectedIndices[i]) != ((int)oldSelectedIndices[i])) { selectionChanged = true; break; } } } else { // indices must have changed if count is different selectionChanged = true; } if (selectionChanged) { // select new indices SelectInternal(newSelectedIndices); } } } else { // no items selected if (SelectedIndex != -1) { SetPostDataSelection(-1); selectionChanged = true; } } return selectionChanged; } ///Loads the posted content of the list control if it is different from the last /// posting. ////// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ///Invokes the OnSelectedIndexChanged method whenever posted data /// for the ///control has changed. /// /// protected virtual void RaisePostDataChangedEvent() { if (AutoPostBack && !Page.IsPostBackEventControlRegistered) { // VSWhidbey 204824 Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnSelectedIndexChanged(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Invokes the OnSelectedIndexChanged method whenever posted data /// for the ///control has changed. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI; ////// [ ValidationProperty("SelectedItem"), SupportsEventValidation ] public class ListBox : ListControl, IPostBackDataHandler { ///Constructs a list box and defines its /// properties. ////// public ListBox() { } ///Initializes a new instance of the ///class. /// [ Browsable(false) ] public override Color BorderColor { get { return base.BorderColor; } set { base.BorderColor = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override BorderStyle BorderStyle { get { return base.BorderStyle; } set { base.BorderStyle = value; } } ///[To be supplied.] ////// [ Browsable(false) ] public override Unit BorderWidth { get { return base.BorderWidth; } set { base.BorderWidth = value; } } internal override bool IsMultiSelectInternal { get { return SelectionMode == ListSelectionMode.Multiple; } } ///[To be supplied.] ////// [ WebCategory("Appearance"), DefaultValue(4), WebSysDescription(SR.ListBox_Rows) ] public virtual int Rows { get { object n = ViewState["Rows"]; return((n == null) ? 4 : (int)n); } set { if (value < 1) { throw new ArgumentOutOfRangeException("value"); } ViewState["Rows"] = value; } } ///Gets or /// sets the display height (in rows) of the list box. ////// [ WebCategory("Behavior"), DefaultValue(ListSelectionMode.Single), WebSysDescription(SR.ListBox_SelectionMode) ] public virtual ListSelectionMode SelectionMode { get { object sm = ViewState["SelectionMode"]; return((sm == null) ? ListSelectionMode.Single : (ListSelectionMode)sm); } set { if (value < ListSelectionMode.Single || value > ListSelectionMode.Multiple) { throw new ArgumentOutOfRangeException("value"); } ViewState["SelectionMode"] = value; } } ///Gets or sets /// the selection behavior of the list box. ///protected override void AddAttributesToRender(HtmlTextWriter writer) { writer.AddAttribute(HtmlTextWriterAttribute.Size, Rows.ToString(NumberFormatInfo.InvariantInfo)); string uniqueID = UniqueID; if (uniqueID != null) { writer.AddAttribute(HtmlTextWriterAttribute.Name, uniqueID); } base.AddAttributesToRender(writer); } public virtual int[] GetSelectedIndices() { return (int[])SelectedIndicesInternal.ToArray(typeof(int)); } /// /// /// protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && SelectionMode == ListSelectionMode.Multiple && Enabled) { // ensure postback when no item is selected Page.RegisterRequiresPostBack(this); } } ////// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Loads the posted content of the list control if it is different from the last /// posting. ////// /// protected virtual bool LoadPostData(String postDataKey, NameValueCollection postCollection) { if (IsEnabled == false) { // When a ListBox is disabled, then there is no postback // data for it. Any checked state information has been loaded // via view state. return false; } string[] selectedItems = postCollection.GetValues(postDataKey); bool selectionChanged = false; EnsureDataBound(); if (selectedItems != null) { if (SelectionMode == ListSelectionMode.Single) { ValidateEvent(postDataKey, selectedItems[0]); int n = Items.FindByValueInternal(selectedItems[0], false); if (SelectedIndex != n) { SetPostDataSelection(n); selectionChanged = true; } } else { // multiple selection int count = selectedItems.Length; ArrayList oldSelectedIndices = SelectedIndicesInternal; ArrayList newSelectedIndices = new ArrayList(count); for (int i=0; i < count; i++) { ValidateEvent(postDataKey, selectedItems[i]); // create array of new indices from posted values newSelectedIndices.Add(Items.FindByValueInternal(selectedItems[i], false)); } int oldcount = 0; if (oldSelectedIndices != null) oldcount = oldSelectedIndices.Count; if (oldcount == count) { // check new indices against old indices // assumes selected values are posted in order for (int i=0; i < count; i++) { if (((int)newSelectedIndices[i]) != ((int)oldSelectedIndices[i])) { selectionChanged = true; break; } } } else { // indices must have changed if count is different selectionChanged = true; } if (selectionChanged) { // select new indices SelectInternal(newSelectedIndices); } } } else { // no items selected if (SelectedIndex != -1) { SetPostDataSelection(-1); selectionChanged = true; } } return selectionChanged; } ///Loads the posted content of the list control if it is different from the last /// posting. ////// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ///Invokes the OnSelectedIndexChanged method whenever posted data /// for the ///control has changed. /// /// protected virtual void RaisePostDataChangedEvent() { if (AutoPostBack && !Page.IsPostBackEventControlRegistered) { // VSWhidbey 204824 Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnSelectedIndexChanged(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Invokes the OnSelectedIndexChanged method whenever posted data /// for the ///control has changed.
Link Menu
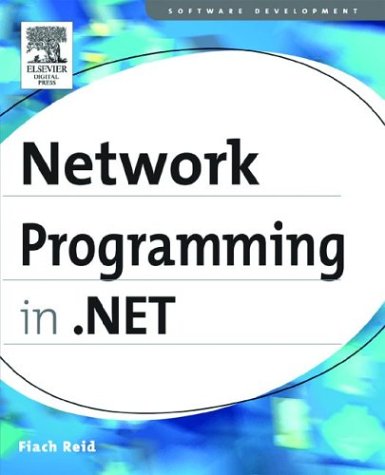
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTableMapping.cs
- GcHandle.cs
- Common.cs
- PageParser.cs
- BrowserInteropHelper.cs
- MaterialCollection.cs
- PackageDigitalSignature.cs
- SingletonConnectionReader.cs
- OleDbConnection.cs
- Transform3D.cs
- HttpProfileGroupBase.cs
- DataTableMappingCollection.cs
- SoapSchemaExporter.cs
- WebPartAuthorizationEventArgs.cs
- StringUtil.cs
- EndEvent.cs
- UnsafeNetInfoNativeMethods.cs
- SafeSerializationManager.cs
- RefreshPropertiesAttribute.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- IPHostEntry.cs
- ListViewHitTestInfo.cs
- Transform.cs
- DataGridViewRowStateChangedEventArgs.cs
- Encoder.cs
- HttpServerChannel.cs
- Label.cs
- HashSetDebugView.cs
- InvalidComObjectException.cs
- NotCondition.cs
- SQLMoneyStorage.cs
- EntityTypeEmitter.cs
- IdentityElement.cs
- Completion.cs
- CacheForPrimitiveTypes.cs
- ZipArchive.cs
- MarkupCompilePass1.cs
- XmlDataImplementation.cs
- MetadataArtifactLoaderResource.cs
- RegexWriter.cs
- SqlRewriteScalarSubqueries.cs
- EventListener.cs
- ReferentialConstraint.cs
- HttpCookieCollection.cs
- DockAndAnchorLayout.cs
- LinqDataView.cs
- OutOfMemoryException.cs
- OutputScope.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- ElementsClipboardData.cs
- ModelItemKeyValuePair.cs
- WebPart.cs
- Graphics.cs
- NativeObjectSecurity.cs
- ToolStripRenderEventArgs.cs
- FragmentQueryKB.cs
- AxisAngleRotation3D.cs
- ByteStack.cs
- GrammarBuilderRuleRef.cs
- CacheMemory.cs
- XpsFilter.cs
- WindowsToolbarAsMenu.cs
- EventPrivateKey.cs
- GenerateTemporaryAssemblyTask.cs
- WebFaultException.cs
- WorkflowView.cs
- TaskSchedulerException.cs
- CustomLineCap.cs
- Rotation3D.cs
- AlphaSortedEnumConverter.cs
- DynamicQueryableWrapper.cs
- OptimizedTemplateContentHelper.cs
- NamespaceCollection.cs
- DecimalAnimation.cs
- DbConnectionInternal.cs
- DataAdapter.cs
- CommandLibraryHelper.cs
- Operators.cs
- ObjectStateFormatter.cs
- SqlCacheDependencyDatabase.cs
- ManagementPath.cs
- ScriptRegistrationManager.cs
- SetIterators.cs
- ParameterCollection.cs
- ObservableCollection.cs
- CodeMemberProperty.cs
- EntityClassGenerator.cs
- BoundConstants.cs
- HtmlShim.cs
- DataGridSortCommandEventArgs.cs
- TextContainer.cs
- ProgressBar.cs
- Int32CollectionValueSerializer.cs
- Calendar.cs
- SchemaComplexType.cs
- CharAnimationUsingKeyFrames.cs
- Guid.cs
- SqlTypeConverter.cs
- RijndaelManagedTransform.cs
- XmlDataProvider.cs