Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Transform.cs / 1305600 / Transform.cs
/****************************************************************************\ * * File: Transform.cs * * Description: * Transform.cs defines the "Transform" object, translate, rotate and scale. * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using MS.Internal.PresentationCore; namespace System.Windows.Media { #region Transform ////// Transform provides a base for all types of transformations, including matrix and list type. /// [Localizability(LocalizationCategory.None, Readability=Readability.Unreadable)] public abstract partial class Transform : GeneralTransform { internal Transform() { } ////// Identity transformation. /// public static Transform Identity { get { return s_identity; } } private static Transform MakeIdentityTransform() { Transform identity = new MatrixTransform(Matrix.Identity); identity.Freeze(); return identity; } private static Transform s_identity = MakeIdentityTransform(); ////// Return the current transformation value. /// public abstract Matrix Value { get; } ////// Returns true if transformation if the transformation is definitely an identity. There are cases where it will /// return false because of computational error or presence of animations (And we're interpolating through a /// transient identity) -- this is intentional. This property is used internally only. If you need to check the /// current matrix value for identity, use Transform.Value.Identity. /// internal abstract bool IsIdentity {get;} internal virtual bool CanSerializeToString() { return false; } #region Perf Helpers internal virtual void TransformRect(ref Rect rect) { Matrix matrix = Value; MatrixUtil.TransformRect(ref rect, ref matrix); } ////// MultiplyValueByMatrix - result is set equal to "this" * matrixToMultiplyBy. /// /// The result is stored here. /// The multiplicand. internal virtual void MultiplyValueByMatrix(ref Matrix result, ref Matrix matrixToMultiplyBy) { result = Value; MatrixUtil.MultiplyMatrix(ref result, ref matrixToMultiplyBy); } ////// Critical -- references and writes out to memory addresses. The /// caller is safe if the pointer points to a D3DMATRIX /// value. /// [SecurityCritical] internal unsafe virtual void ConvertToD3DMATRIX(/* out */ D3DMATRIX* milMatrix) { Matrix matrix = Value; MILUtilities.ConvertToD3DMATRIX(&matrix, milMatrix); } #endregion ////// Consolidates the common logic of obtain the value of a /// Transform, after checking the transform for null. /// /// Transform to obtain value of. /// /// Current value of 'transform'. Matrix.Identity if /// the 'transform' parameter is null. /// internal static void GetTransformValue( Transform transform, out Matrix currentTransformValue ) { if (transform != null) { currentTransformValue = transform.Value; } else { currentTransformValue = Matrix.Identity; } } ////// Transforms a point /// /// Input point /// Output point ///True if the point was successfully transformed public override bool TryTransform(Point inPoint, out Point result) { Matrix m = Value; result = m.Transform(inPoint); return true; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Bounding box ///The transformed bounding box public override Rect TransformBounds(Rect rect) { TransformRect(ref rect); return rect; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform Inverse { get { ReadPreamble(); Matrix matrix = Value; if (!matrix.HasInverse) { return null; } matrix.Invert(); return new MatrixTransform(matrix); } } ////// Returns a best effort affine transform /// internal override Transform AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { return this; } } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: Transform.cs * * Description: * Transform.cs defines the "Transform" object, translate, rotate and scale. * * Copyright (C) 2002 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using MS.Internal.PresentationCore; namespace System.Windows.Media { #region Transform ////// Transform provides a base for all types of transformations, including matrix and list type. /// [Localizability(LocalizationCategory.None, Readability=Readability.Unreadable)] public abstract partial class Transform : GeneralTransform { internal Transform() { } ////// Identity transformation. /// public static Transform Identity { get { return s_identity; } } private static Transform MakeIdentityTransform() { Transform identity = new MatrixTransform(Matrix.Identity); identity.Freeze(); return identity; } private static Transform s_identity = MakeIdentityTransform(); ////// Return the current transformation value. /// public abstract Matrix Value { get; } ////// Returns true if transformation if the transformation is definitely an identity. There are cases where it will /// return false because of computational error or presence of animations (And we're interpolating through a /// transient identity) -- this is intentional. This property is used internally only. If you need to check the /// current matrix value for identity, use Transform.Value.Identity. /// internal abstract bool IsIdentity {get;} internal virtual bool CanSerializeToString() { return false; } #region Perf Helpers internal virtual void TransformRect(ref Rect rect) { Matrix matrix = Value; MatrixUtil.TransformRect(ref rect, ref matrix); } ////// MultiplyValueByMatrix - result is set equal to "this" * matrixToMultiplyBy. /// /// The result is stored here. /// The multiplicand. internal virtual void MultiplyValueByMatrix(ref Matrix result, ref Matrix matrixToMultiplyBy) { result = Value; MatrixUtil.MultiplyMatrix(ref result, ref matrixToMultiplyBy); } ////// Critical -- references and writes out to memory addresses. The /// caller is safe if the pointer points to a D3DMATRIX /// value. /// [SecurityCritical] internal unsafe virtual void ConvertToD3DMATRIX(/* out */ D3DMATRIX* milMatrix) { Matrix matrix = Value; MILUtilities.ConvertToD3DMATRIX(&matrix, milMatrix); } #endregion ////// Consolidates the common logic of obtain the value of a /// Transform, after checking the transform for null. /// /// Transform to obtain value of. /// /// Current value of 'transform'. Matrix.Identity if /// the 'transform' parameter is null. /// internal static void GetTransformValue( Transform transform, out Matrix currentTransformValue ) { if (transform != null) { currentTransformValue = transform.Value; } else { currentTransformValue = Matrix.Identity; } } ////// Transforms a point /// /// Input point /// Output point ///True if the point was successfully transformed public override bool TryTransform(Point inPoint, out Point result) { Matrix m = Value; result = m.Transform(inPoint); return true; } ////// Transforms the bounding box to the smallest axis aligned bounding box /// that contains all the points in the original bounding box /// /// Bounding box ///The transformed bounding box public override Rect TransformBounds(Rect rect) { TransformRect(ref rect); return rect; } ////// Returns the inverse transform if it has an inverse, null otherwise /// public override GeneralTransform Inverse { get { ReadPreamble(); Matrix matrix = Value; if (!matrix.HasInverse) { return null; } matrix.Invert(); return new MatrixTransform(matrix); } } ////// Returns a best effort affine transform /// internal override Transform AffineTransform { [FriendAccessAllowed] // Built into Core, also used by Framework. get { return this; } } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
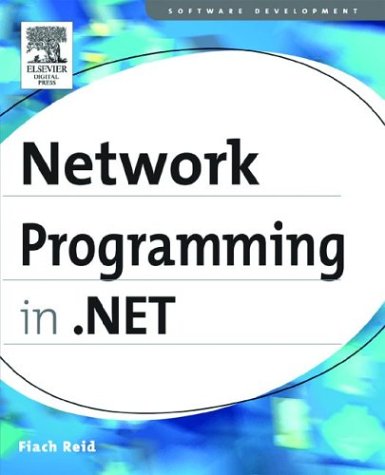
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeRemoveEventStatement.cs
- BigInt.cs
- VirtualizedContainerService.cs
- StringFreezingAttribute.cs
- DataGridPreparingCellForEditEventArgs.cs
- DbParameterHelper.cs
- DbDataAdapter.cs
- ImageAnimator.cs
- OleDbParameter.cs
- SchemaImporterExtensionElement.cs
- BrowserInteropHelper.cs
- ListBoxChrome.cs
- PointCollection.cs
- ContentElementAutomationPeer.cs
- MimeWriter.cs
- RuntimeHelpers.cs
- LocalBuilder.cs
- OuterGlowBitmapEffect.cs
- OletxVolatileEnlistment.cs
- EncoderBestFitFallback.cs
- ScrollViewer.cs
- ContainerUtilities.cs
- WebBrowserHelper.cs
- EmptyQuery.cs
- ClickablePoint.cs
- ConcurrencyMode.cs
- OdbcEnvironmentHandle.cs
- MediaPlayerState.cs
- TemplateField.cs
- XmlProcessingInstruction.cs
- RangeContentEnumerator.cs
- UIElement.cs
- UInt32Storage.cs
- InfoCardTraceRecord.cs
- ContentFileHelper.cs
- MetadataArtifactLoaderComposite.cs
- URLIdentityPermission.cs
- ModifyActivitiesPropertyDescriptor.cs
- Thickness.cs
- CheckoutException.cs
- Privilege.cs
- CompareInfo.cs
- ZipFileInfoCollection.cs
- SpellCheck.cs
- StylusSystemGestureEventArgs.cs
- MergePropertyDescriptor.cs
- ProviderSettings.cs
- ListBindingHelper.cs
- PassportIdentity.cs
- CommandEventArgs.cs
- IResourceProvider.cs
- ScriptMethodAttribute.cs
- SoapElementAttribute.cs
- NamedElement.cs
- CrossContextChannel.cs
- Internal.cs
- ResourceExpressionBuilder.cs
- AmbientLight.cs
- WindowsImpersonationContext.cs
- Animatable.cs
- OleCmdHelper.cs
- XmlAttributeCollection.cs
- ExtenderProvidedPropertyAttribute.cs
- TraceSection.cs
- TokenizerHelper.cs
- DataGridViewRowEventArgs.cs
- IsolatedStorageFileStream.cs
- TextComposition.cs
- PrintControllerWithStatusDialog.cs
- AdCreatedEventArgs.cs
- ResourceKey.cs
- DataServiceContext.cs
- DPTypeDescriptorContext.cs
- SingleAnimationBase.cs
- VisualStateManager.cs
- LinqDataSourceContextEventArgs.cs
- MatchAttribute.cs
- PeerNameResolver.cs
- PartialTrustVisibleAssembly.cs
- ToolStripOverflowButton.cs
- TokenBasedSet.cs
- ToolStripGripRenderEventArgs.cs
- ConstrainedGroup.cs
- SafeFileHandle.cs
- ZipIOBlockManager.cs
- BridgeDataReader.cs
- NonSerializedAttribute.cs
- BitmapCodecInfoInternal.cs
- SqlDataSourceStatusEventArgs.cs
- RtfNavigator.cs
- SqlDataSourceWizardForm.cs
- DbBuffer.cs
- WindowsRegion.cs
- ELinqQueryState.cs
- HttpListenerPrefixCollection.cs
- WebException.cs
- WeakReadOnlyCollection.cs
- StringExpressionSet.cs
- HtmlInputFile.cs
- validationstate.cs