Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / WebException.cs / 1 / WebException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Runtime.Serialization; using System.Security.Permissions; /*++ Abstract: Contains the defintion for the WebException object. This is a subclass of Exception that contains a WebExceptionStatus and possible a reference to a WebResponse. --*/ ////// [Serializable] public class WebException : InvalidOperationException, ISerializable { private WebExceptionStatus m_Status = WebExceptionStatus.UnknownError; //Should be changed to GeneralFailure; private WebResponse m_Response; [NonSerialized] private WebExceptionInternalStatus m_InternalStatus = WebExceptionInternalStatus.RequestFatal; ////// Provides network communication exceptions to the application. /// /// This is the exception that is thrown by WebRequests when something untoward /// happens. It's a subclass of WebException that contains a WebExceptionStatus and possibly /// a reference to a WebResponse. The WebResponse is only present if we actually /// have a response from the remote server. /// ////// public WebException() { } ////// Creates a new instance of the ////// class with the default status /// from the /// values. /// /// public WebException(string message) : this(message, null) { } ////// Creates a new instance of the ///class with the specified error /// message. /// /// public WebException(string message, Exception innerException) : base(message, innerException) { } public WebException(string message, WebExceptionStatus status) : this(message, null, status, null) { } ////// Creates a new instance of the ///class with the specified error /// message and nested exception. /// /// Message - Message string for exception. /// InnerException - Exception that caused this exception. /// /// /// internal WebException(string message, WebExceptionStatus status, WebExceptionInternalStatus internalStatus, Exception innerException) : this(message, innerException, status, null, internalStatus) { } ////// Creates a new instance of the ///class with the specified error /// message and status. /// /// Message - Message string for exception. /// Status - Network status of exception /// /// public WebException(string message, Exception innerException, WebExceptionStatus status, WebResponse response) : this(message, null, innerException, status, response) { } internal WebException(string message, string data, Exception innerException, WebExceptionStatus status, WebResponse response) : base(message + (data != null ? ": '" + data + "'" : ""), innerException) { m_Status = status; m_Response = response; } internal WebException(string message, Exception innerException, WebExceptionStatus status, WebResponse response, WebExceptionInternalStatus internalStatus) : this(message, null, innerException, status, response, internalStatus) { } internal WebException(string message, string data, Exception innerException, WebExceptionStatus status, WebResponse response, WebExceptionInternalStatus internalStatus) : base(message + (data != null ? ": '" + data + "'" : ""), innerException) { m_Status = status; m_Response = response; m_InternalStatus = internalStatus; } protected WebException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { // m_Status = (WebExceptionStatus)serializationInfo.GetInt32("Status"); // m_InternalStatus = (WebExceptionInternalStatus)serializationInfo.GetInt32("InternalStatus"); } ////// Creates a new instance of the ///class with the specified error /// message, nested exception, status and response. /// /// Message - Message string for exception. /// InnerException - The exception that caused this one. /// Status - Network status of exception /// Response - The WebResponse we have. /// [SecurityPermission(SecurityAction.LinkDemand, Flags = SecurityPermissionFlag.SerializationFormatter)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext){ base.GetObjectData(serializationInfo, streamingContext); //serializationInfo.AddValue("Status", (int)m_Status, typeof(int)); //serializationInfo.AddValue("InternalStatus", (int)m_InternalStatus, typeof(int)); } /// /// public WebExceptionStatus Status { get { return m_Status; } } ////// Gets the status of the response. /// ////// public WebResponse Response { get { return m_Response; } } ////// Gets the error message returned from the remote host. /// ////// internal WebExceptionInternalStatus InternalStatus { get { return m_InternalStatus; } } }; // class WebException internal enum WebExceptionInternalStatus { RequestFatal = 0, ServicePointFatal = 1, Recoverable = 2, Isolated = 3, } } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Gets the error message returned from the remote host. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Runtime.Serialization; using System.Security.Permissions; /*++ Abstract: Contains the defintion for the WebException object. This is a subclass of Exception that contains a WebExceptionStatus and possible a reference to a WebResponse. --*/ ////// [Serializable] public class WebException : InvalidOperationException, ISerializable { private WebExceptionStatus m_Status = WebExceptionStatus.UnknownError; //Should be changed to GeneralFailure; private WebResponse m_Response; [NonSerialized] private WebExceptionInternalStatus m_InternalStatus = WebExceptionInternalStatus.RequestFatal; ////// Provides network communication exceptions to the application. /// /// This is the exception that is thrown by WebRequests when something untoward /// happens. It's a subclass of WebException that contains a WebExceptionStatus and possibly /// a reference to a WebResponse. The WebResponse is only present if we actually /// have a response from the remote server. /// ////// public WebException() { } ////// Creates a new instance of the ////// class with the default status /// from the /// values. /// /// public WebException(string message) : this(message, null) { } ////// Creates a new instance of the ///class with the specified error /// message. /// /// public WebException(string message, Exception innerException) : base(message, innerException) { } public WebException(string message, WebExceptionStatus status) : this(message, null, status, null) { } ////// Creates a new instance of the ///class with the specified error /// message and nested exception. /// /// Message - Message string for exception. /// InnerException - Exception that caused this exception. /// /// /// internal WebException(string message, WebExceptionStatus status, WebExceptionInternalStatus internalStatus, Exception innerException) : this(message, innerException, status, null, internalStatus) { } ////// Creates a new instance of the ///class with the specified error /// message and status. /// /// Message - Message string for exception. /// Status - Network status of exception /// /// public WebException(string message, Exception innerException, WebExceptionStatus status, WebResponse response) : this(message, null, innerException, status, response) { } internal WebException(string message, string data, Exception innerException, WebExceptionStatus status, WebResponse response) : base(message + (data != null ? ": '" + data + "'" : ""), innerException) { m_Status = status; m_Response = response; } internal WebException(string message, Exception innerException, WebExceptionStatus status, WebResponse response, WebExceptionInternalStatus internalStatus) : this(message, null, innerException, status, response, internalStatus) { } internal WebException(string message, string data, Exception innerException, WebExceptionStatus status, WebResponse response, WebExceptionInternalStatus internalStatus) : base(message + (data != null ? ": '" + data + "'" : ""), innerException) { m_Status = status; m_Response = response; m_InternalStatus = internalStatus; } protected WebException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { // m_Status = (WebExceptionStatus)serializationInfo.GetInt32("Status"); // m_InternalStatus = (WebExceptionInternalStatus)serializationInfo.GetInt32("InternalStatus"); } ////// Creates a new instance of the ///class with the specified error /// message, nested exception, status and response. /// /// Message - Message string for exception. /// InnerException - The exception that caused this one. /// Status - Network status of exception /// Response - The WebResponse we have. /// [SecurityPermission(SecurityAction.LinkDemand, Flags = SecurityPermissionFlag.SerializationFormatter)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext){ base.GetObjectData(serializationInfo, streamingContext); //serializationInfo.AddValue("Status", (int)m_Status, typeof(int)); //serializationInfo.AddValue("InternalStatus", (int)m_InternalStatus, typeof(int)); } /// /// public WebExceptionStatus Status { get { return m_Status; } } ////// Gets the status of the response. /// ////// public WebResponse Response { get { return m_Response; } } ////// Gets the error message returned from the remote host. /// ////// internal WebExceptionInternalStatus InternalStatus { get { return m_InternalStatus; } } }; // class WebException internal enum WebExceptionInternalStatus { RequestFatal = 0, ServicePointFatal = 1, Recoverable = 2, Isolated = 3, } } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets the error message returned from the remote host. /// ///
Link Menu
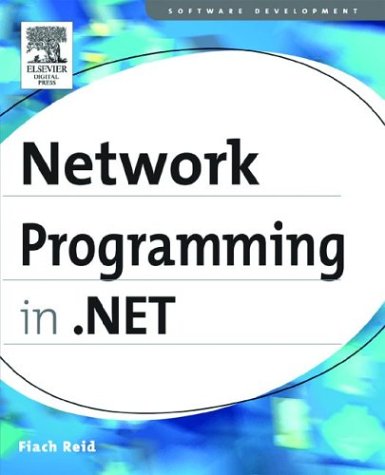
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InfiniteTimeSpanConverter.cs
- SQLSingle.cs
- ArrayElementGridEntry.cs
- XXXOnTypeBuilderInstantiation.cs
- ExceptionTrace.cs
- _ListenerAsyncResult.cs
- AccessDataSourceView.cs
- JsonWriter.cs
- MetadataProperty.cs
- XmlHierarchicalDataSourceView.cs
- BamlRecords.cs
- SoapAttributeOverrides.cs
- SafeReversePInvokeHandle.cs
- NamespaceEmitter.cs
- WarningException.cs
- XmlAnyAttributeAttribute.cs
- ErrorsHelper.cs
- StreamAsIStream.cs
- RecognizeCompletedEventArgs.cs
- UnionExpr.cs
- _Semaphore.cs
- HwndProxyElementProvider.cs
- OptimalBreakSession.cs
- QuerySelectOp.cs
- DataPagerFieldItem.cs
- OdbcRowUpdatingEvent.cs
- TextSelection.cs
- COM2ComponentEditor.cs
- HTTP_SERVICE_CONFIG_URLACL_KEY.cs
- SqlMethods.cs
- _CookieModule.cs
- FamilyMapCollection.cs
- shaperfactoryquerycacheentry.cs
- _ConnectionGroup.cs
- ReservationNotFoundException.cs
- WindowsEditBoxRange.cs
- RepeatBehavior.cs
- DataGridViewColumnEventArgs.cs
- PropertyGridCommands.cs
- XpsFilter.cs
- KeyToListMap.cs
- ActivityExecutor.cs
- SqlConnection.cs
- SelectionRange.cs
- InvariantComparer.cs
- TemplateBindingExtensionConverter.cs
- ColumnHeaderConverter.cs
- UrlRoutingModule.cs
- IgnoreSection.cs
- StorageMappingItemCollection.cs
- NetworkInterface.cs
- IsolationInterop.cs
- GatewayIPAddressInformationCollection.cs
- assemblycache.cs
- DesignerContextDescriptor.cs
- AutoCompleteStringCollection.cs
- Point3DAnimation.cs
- EntityDataSourceSelectedEventArgs.cs
- WebZoneDesigner.cs
- EventDescriptorCollection.cs
- ObjectDataSource.cs
- X509UI.cs
- CodeComment.cs
- XmlAttributeOverrides.cs
- LazyTextWriterCreator.cs
- Debugger.cs
- CompModHelpers.cs
- OracleInternalConnection.cs
- Visitor.cs
- SettingsPropertyIsReadOnlyException.cs
- AutomationElementIdentifiers.cs
- WmlCommandAdapter.cs
- EntityContainer.cs
- TdsParameterSetter.cs
- DefaultValueTypeConverter.cs
- FloaterBaseParaClient.cs
- ButtonBase.cs
- WindowsGrip.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- _ScatterGatherBuffers.cs
- RangeValidator.cs
- CaseInsensitiveOrdinalStringComparer.cs
- SiteMapProvider.cs
- FontCacheUtil.cs
- VerificationAttribute.cs
- RegexCode.cs
- RuntimeHelpers.cs
- SqlDataSourceQuery.cs
- TableLayoutPanel.cs
- ChangeDirector.cs
- DataSourceNameHandler.cs
- SafeEventLogWriteHandle.cs
- FrameworkTemplate.cs
- DataColumnMapping.cs
- SocketException.cs
- GeneratedCodeAttribute.cs
- XmlSchema.cs
- Rule.cs
- DataBoundControl.cs
- QueryStringParameter.cs