Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / WinFormsIntegration / System / Windows / Integration / WindowsFormsHostPropertyMap.cs / 1 / WindowsFormsHostPropertyMap.cs
using System.Windows.Media; using System.Reflection; using SWF = System.Windows.Forms; using SD = System.Drawing; using SW = System.Windows; using SWM = System.Windows.Media; using SWI = System.Windows.Input; using SWC = System.Windows.Controls; namespace System.Windows.Forms.Integration { internal sealed class WindowsFormsHostPropertyMap : PropertyMap { public WindowsFormsHostPropertyMap(WindowsFormsHost host) : base(host) { InitializeDefaultTranslators(); ResetAll(); } ////// Initialize the list of things we translate by default, like /// Background. /// private void InitializeDefaultTranslators() { DefaultTranslators.Add("Background", BackgroundPropertyTranslator); DefaultTranslators.Add("FlowDirection", FlowDirectionPropertyTranslator); DefaultTranslators.Add("FontStyle", FontStylePropertyTranslator); DefaultTranslators.Add("FontWeight", FontWeightPropertyTranslator); DefaultTranslators.Add("FontFamily", FontFamilyPropertyTranslator); DefaultTranslators.Add("FontSize", FontSizePropertyTranslator); DefaultTranslators.Add("Foreground", ForegroundPropertyTranslator); DefaultTranslators.Add("IsEnabled", IsEnabledPropertyTranslator); DefaultTranslators.Add("Padding", PaddingPropertyTranslator); DefaultTranslators.Add("Visibility", VisibilityPropertyTranslator); //Note: there's no notification when the ambient cursor changes, so we //can't do a normal mapping for this and have it work. See the note in //WinFormsAdapter.Cursor. DefaultTranslators.Add("Cursor", EmptyPropertyTranslator); DefaultTranslators.Add("ForceCursor", EmptyPropertyTranslator); } ////// Translator for individual property /// private void BackgroundPropertyTranslator(object host, string propertyName, object value) { SWM.Brush brush = value as SWM.Brush; if (value == null) { //If they passed null (not to be confused with "passed us a non-null non-Brush"), //we should look up our parent chain. DependencyObject parent = host as WindowsFormsHost; do { brush = (Brush)parent.GetValue(SWC.Control.BackgroundProperty); parent = VisualTreeHelper.GetParent(parent); } while (parent != null && brush == null); } WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && brush != null) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost != null) { SWF.Control child = windowsFormsHost.Child; if (HostUtils.BrushIsSolidOpaque(brush)) { bool ignore; SD.Color wfColor = WindowsFormsHostPropertyMap.TranslateSolidOrGradientBrush(brush, out ignore); adapter.BackColor = wfColor; } else { SD.Bitmap backgroundImage = HostUtils.GetBitmapForWindowsFormsHost(windowsFormsHost, brush); HostUtils.SetBackgroundImage(adapter, child, backgroundImage); } } } } ////// Translator for individual property /// private void FlowDirectionPropertyTranslator(object host, string propertyName, object value) { SWF.Control childControl = GetChildControl(host, FlowDirectionPropertyTranslator, value); WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && childControl != null) { if (value is SW.FlowDirection) { const BindingFlags memberAccess = BindingFlags.Public | BindingFlags.Instance; //use reflection to see if this control supports the RightToLeftLayout property PropertyInfo propertyInfo = childControl.GetType().GetProperty("RightToLeftLayout", memberAccess); switch ((SW.FlowDirection)value) { case SW.FlowDirection.RightToLeft: adapter.RightToLeft = SWF.RightToLeft.Yes; if (propertyInfo != null) { propertyInfo.SetValue(childControl, true, null); } break; case SW.FlowDirection.LeftToRight: adapter.RightToLeft = SWF.RightToLeft.No; if (propertyInfo != null) { propertyInfo.SetValue(childControl, false, null); } break; } } } } ////// Translator for individual property /// private void FontFamilyPropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null) { SWM.FontFamily family = value as SWM.FontFamily; if (family != null) { string familySource = family.Source; adapter.Font = new SD.Font(familySource, adapter.Font.Size, adapter.Font.Style); } } } ////// Translator for individual property /// private void FontStylePropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null) { if (value is SW.FontStyle) { SD.FontStyle style; if ((SW.FontStyle)value == SW.FontStyles.Normal) { style = SD.FontStyle.Regular; } else { style = SD.FontStyle.Italic; } if (HostUtils.FontWeightIsBold(Host.FontWeight)) { style |= SD.FontStyle.Bold; } adapter.Font = new SD.Font(CurrentFontFamily, CurrentFontSize, style); } } } ////// Translator for individual property /// private void FontWeightPropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && value is SW.FontWeight) { SD.FontStyle style = CurrentFontStyle; if (HostUtils.FontWeightIsBold((SW.FontWeight)value)) { style |= SD.FontStyle.Bold; } adapter.Font = new SD.Font(CurrentFontFamily, CurrentFontSize, style); } } ////// Translator for individual property /// private void FontSizePropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && value is double) { double pointSize = Convert.FontSizeToSystemDrawing((double)value); adapter.Font = new SD.Font(CurrentFontFamily, (float)pointSize, CurrentFontStyle); } } ////// Translator for individual property /// private void ForegroundPropertyTranslator(object host, string propertyName, object value) { SWM.Brush brush = value as SWM.Brush; if (brush != null) { bool defined; SD.Color wfColor = WindowsFormsHostPropertyMap.TranslateSolidOrGradientBrush(brush, out defined); if (defined) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null) { adapter.ForeColor = wfColor; } } } } ////// Translator for individual property /// private void IsEnabledPropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && value is bool) { adapter.Enabled = (bool)value; } } ////// Translator for individual property /// private void PaddingPropertyTranslator(object host, string propertyName, object value) { SWF.Control childControl = GetChildControl(host, PaddingPropertyTranslator, value); WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && childControl != null) { if (value is SW.Thickness) { childControl.Padding = Convert.ToSystemWindowsFormsPadding((SW.Thickness)value); } } } ////// Translator for Visibility property /// private void VisibilityPropertyTranslator(object host, string propertyName, object value) { if (value is SW.Visibility) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost != null && windowsFormsHost.Child != null) { //Visible for Visible, not Visible for Hidden/Collapsed windowsFormsHost.Child.Visible = ((SW.Visibility)value == SW.Visibility.Visible); } } } ////// The WindowsFormsHost we're mapping /// private WindowsFormsHost Host { get { return (WindowsFormsHost)SourceObject; } } ////// Gets the child control if we have one. If we don't, cache the translator /// and property value so that we can restore them when the control is set. /// WindowsFormsHost /// The delegate to be called if this property is to be set later /// The value the property will have if it needs to be set later /// private SWF.Control GetChildControl(object host, PropertyTranslator translator, object value) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost == null || windowsFormsHost.Child == null) { return null; } return windowsFormsHost.Child; } ////// The HostContainer, if any. /// /// WindowsFormsHost internal static WinFormsAdapter GetAdapter(object host) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost == null) { return null; } return windowsFormsHost.HostContainerInternal; } ////// Translate the fontsize of this WFH to a font size equivalent to one /// that a SD.Font would use. /// private float CurrentFontSize { get { return (float)(Convert.FontSizeToSystemDrawing(Host.FontSize)); } } ////// Translate the FontStyle and FontWeight of this WFH to a SD.Font /// equivalent. /// private SD.FontStyle CurrentFontStyle { get { SD.FontStyle style; if (Host.FontStyle == SW.FontStyles.Normal) { style = SD.FontStyle.Regular; } else { style = SD.FontStyle.Italic; } if (HostUtils.FontWeightIsBold(Host.FontWeight)) { style |= SD.FontStyle.Bold; } return style; } } ////// The current FontFamily of this WFH /// private string CurrentFontFamily { get { return Host.FontFamily.Source; } } ////// This method translates Avalon SD.Brushes into /// WindowsForms color objects /// private static SD.Color TranslateSolidOrGradientBrush(SWM.Brush brush, out bool defined) { SWM.Color brushColor; SD.Color wfColor = SD.Color.Empty; defined = false; SWM.SolidColorBrush solidColorBrush = brush as SWM.SolidColorBrush; if (solidColorBrush != null) { brushColor = solidColorBrush.Color; defined = true; wfColor = Convert.ToSystemDrawingColor(brushColor); } else { SWM.GradientBrush gradientBrush = brush as SWM.GradientBrush; if (gradientBrush != null) { SWM.GradientStopCollection grads = gradientBrush.GradientStops; if (grads != null) { SWM.GradientStop firstStop = grads[0]; if (firstStop != null) { brushColor = firstStop.Color; defined = true; wfColor = Convert.ToSystemDrawingColor(brushColor); } } } } return wfColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Windows.Media; using System.Reflection; using SWF = System.Windows.Forms; using SD = System.Drawing; using SW = System.Windows; using SWM = System.Windows.Media; using SWI = System.Windows.Input; using SWC = System.Windows.Controls; namespace System.Windows.Forms.Integration { internal sealed class WindowsFormsHostPropertyMap : PropertyMap { public WindowsFormsHostPropertyMap(WindowsFormsHost host) : base(host) { InitializeDefaultTranslators(); ResetAll(); } ////// Initialize the list of things we translate by default, like /// Background. /// private void InitializeDefaultTranslators() { DefaultTranslators.Add("Background", BackgroundPropertyTranslator); DefaultTranslators.Add("FlowDirection", FlowDirectionPropertyTranslator); DefaultTranslators.Add("FontStyle", FontStylePropertyTranslator); DefaultTranslators.Add("FontWeight", FontWeightPropertyTranslator); DefaultTranslators.Add("FontFamily", FontFamilyPropertyTranslator); DefaultTranslators.Add("FontSize", FontSizePropertyTranslator); DefaultTranslators.Add("Foreground", ForegroundPropertyTranslator); DefaultTranslators.Add("IsEnabled", IsEnabledPropertyTranslator); DefaultTranslators.Add("Padding", PaddingPropertyTranslator); DefaultTranslators.Add("Visibility", VisibilityPropertyTranslator); //Note: there's no notification when the ambient cursor changes, so we //can't do a normal mapping for this and have it work. See the note in //WinFormsAdapter.Cursor. DefaultTranslators.Add("Cursor", EmptyPropertyTranslator); DefaultTranslators.Add("ForceCursor", EmptyPropertyTranslator); } ////// Translator for individual property /// private void BackgroundPropertyTranslator(object host, string propertyName, object value) { SWM.Brush brush = value as SWM.Brush; if (value == null) { //If they passed null (not to be confused with "passed us a non-null non-Brush"), //we should look up our parent chain. DependencyObject parent = host as WindowsFormsHost; do { brush = (Brush)parent.GetValue(SWC.Control.BackgroundProperty); parent = VisualTreeHelper.GetParent(parent); } while (parent != null && brush == null); } WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && brush != null) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost != null) { SWF.Control child = windowsFormsHost.Child; if (HostUtils.BrushIsSolidOpaque(brush)) { bool ignore; SD.Color wfColor = WindowsFormsHostPropertyMap.TranslateSolidOrGradientBrush(brush, out ignore); adapter.BackColor = wfColor; } else { SD.Bitmap backgroundImage = HostUtils.GetBitmapForWindowsFormsHost(windowsFormsHost, brush); HostUtils.SetBackgroundImage(adapter, child, backgroundImage); } } } } ////// Translator for individual property /// private void FlowDirectionPropertyTranslator(object host, string propertyName, object value) { SWF.Control childControl = GetChildControl(host, FlowDirectionPropertyTranslator, value); WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && childControl != null) { if (value is SW.FlowDirection) { const BindingFlags memberAccess = BindingFlags.Public | BindingFlags.Instance; //use reflection to see if this control supports the RightToLeftLayout property PropertyInfo propertyInfo = childControl.GetType().GetProperty("RightToLeftLayout", memberAccess); switch ((SW.FlowDirection)value) { case SW.FlowDirection.RightToLeft: adapter.RightToLeft = SWF.RightToLeft.Yes; if (propertyInfo != null) { propertyInfo.SetValue(childControl, true, null); } break; case SW.FlowDirection.LeftToRight: adapter.RightToLeft = SWF.RightToLeft.No; if (propertyInfo != null) { propertyInfo.SetValue(childControl, false, null); } break; } } } } ////// Translator for individual property /// private void FontFamilyPropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null) { SWM.FontFamily family = value as SWM.FontFamily; if (family != null) { string familySource = family.Source; adapter.Font = new SD.Font(familySource, adapter.Font.Size, adapter.Font.Style); } } } ////// Translator for individual property /// private void FontStylePropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null) { if (value is SW.FontStyle) { SD.FontStyle style; if ((SW.FontStyle)value == SW.FontStyles.Normal) { style = SD.FontStyle.Regular; } else { style = SD.FontStyle.Italic; } if (HostUtils.FontWeightIsBold(Host.FontWeight)) { style |= SD.FontStyle.Bold; } adapter.Font = new SD.Font(CurrentFontFamily, CurrentFontSize, style); } } } ////// Translator for individual property /// private void FontWeightPropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && value is SW.FontWeight) { SD.FontStyle style = CurrentFontStyle; if (HostUtils.FontWeightIsBold((SW.FontWeight)value)) { style |= SD.FontStyle.Bold; } adapter.Font = new SD.Font(CurrentFontFamily, CurrentFontSize, style); } } ////// Translator for individual property /// private void FontSizePropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && value is double) { double pointSize = Convert.FontSizeToSystemDrawing((double)value); adapter.Font = new SD.Font(CurrentFontFamily, (float)pointSize, CurrentFontStyle); } } ////// Translator for individual property /// private void ForegroundPropertyTranslator(object host, string propertyName, object value) { SWM.Brush brush = value as SWM.Brush; if (brush != null) { bool defined; SD.Color wfColor = WindowsFormsHostPropertyMap.TranslateSolidOrGradientBrush(brush, out defined); if (defined) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null) { adapter.ForeColor = wfColor; } } } } ////// Translator for individual property /// private void IsEnabledPropertyTranslator(object host, string propertyName, object value) { WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && value is bool) { adapter.Enabled = (bool)value; } } ////// Translator for individual property /// private void PaddingPropertyTranslator(object host, string propertyName, object value) { SWF.Control childControl = GetChildControl(host, PaddingPropertyTranslator, value); WinFormsAdapter adapter = GetAdapter(host); if (adapter != null && childControl != null) { if (value is SW.Thickness) { childControl.Padding = Convert.ToSystemWindowsFormsPadding((SW.Thickness)value); } } } ////// Translator for Visibility property /// private void VisibilityPropertyTranslator(object host, string propertyName, object value) { if (value is SW.Visibility) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost != null && windowsFormsHost.Child != null) { //Visible for Visible, not Visible for Hidden/Collapsed windowsFormsHost.Child.Visible = ((SW.Visibility)value == SW.Visibility.Visible); } } } ////// The WindowsFormsHost we're mapping /// private WindowsFormsHost Host { get { return (WindowsFormsHost)SourceObject; } } ////// Gets the child control if we have one. If we don't, cache the translator /// and property value so that we can restore them when the control is set. /// WindowsFormsHost /// The delegate to be called if this property is to be set later /// The value the property will have if it needs to be set later /// private SWF.Control GetChildControl(object host, PropertyTranslator translator, object value) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost == null || windowsFormsHost.Child == null) { return null; } return windowsFormsHost.Child; } ////// The HostContainer, if any. /// /// WindowsFormsHost internal static WinFormsAdapter GetAdapter(object host) { WindowsFormsHost windowsFormsHost = host as WindowsFormsHost; if (windowsFormsHost == null) { return null; } return windowsFormsHost.HostContainerInternal; } ////// Translate the fontsize of this WFH to a font size equivalent to one /// that a SD.Font would use. /// private float CurrentFontSize { get { return (float)(Convert.FontSizeToSystemDrawing(Host.FontSize)); } } ////// Translate the FontStyle and FontWeight of this WFH to a SD.Font /// equivalent. /// private SD.FontStyle CurrentFontStyle { get { SD.FontStyle style; if (Host.FontStyle == SW.FontStyles.Normal) { style = SD.FontStyle.Regular; } else { style = SD.FontStyle.Italic; } if (HostUtils.FontWeightIsBold(Host.FontWeight)) { style |= SD.FontStyle.Bold; } return style; } } ////// The current FontFamily of this WFH /// private string CurrentFontFamily { get { return Host.FontFamily.Source; } } ////// This method translates Avalon SD.Brushes into /// WindowsForms color objects /// private static SD.Color TranslateSolidOrGradientBrush(SWM.Brush brush, out bool defined) { SWM.Color brushColor; SD.Color wfColor = SD.Color.Empty; defined = false; SWM.SolidColorBrush solidColorBrush = brush as SWM.SolidColorBrush; if (solidColorBrush != null) { brushColor = solidColorBrush.Color; defined = true; wfColor = Convert.ToSystemDrawingColor(brushColor); } else { SWM.GradientBrush gradientBrush = brush as SWM.GradientBrush; if (gradientBrush != null) { SWM.GradientStopCollection grads = gradientBrush.GradientStops; if (grads != null) { SWM.GradientStop firstStop = grads[0]; if (firstStop != null) { brushColor = firstStop.Color; defined = true; wfColor = Convert.ToSystemDrawingColor(brushColor); } } } } return wfColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
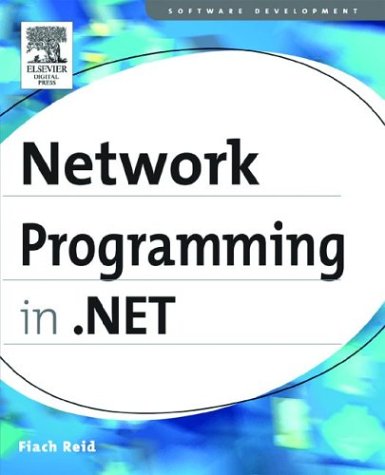
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripLocationCancelEventArgs.cs
- Range.cs
- GlyphTypeface.cs
- DbProviderServices.cs
- RoleGroupCollectionEditor.cs
- PropertyChangingEventArgs.cs
- DesignerFrame.cs
- HtmlElementErrorEventArgs.cs
- EllipseGeometry.cs
- TabControlAutomationPeer.cs
- KeyboardDevice.cs
- Scene3D.cs
- DbQueryCommandTree.cs
- Logging.cs
- DropSourceBehavior.cs
- EntitySqlQueryState.cs
- SortDescriptionCollection.cs
- MenuBase.cs
- BindingNavigator.cs
- InvariantComparer.cs
- Msec.cs
- DesignerToolStripControlHost.cs
- TypeConverterBase.cs
- LinearKeyFrames.cs
- XComponentModel.cs
- Base64Stream.cs
- ConfigurationCollectionAttribute.cs
- TimeIntervalCollection.cs
- WindowsFormsHost.cs
- ExpanderAutomationPeer.cs
- NamedObject.cs
- DebugHandleTracker.cs
- DateTimeUtil.cs
- ProviderConnectionPointCollection.cs
- FrameworkContentElementAutomationPeer.cs
- ListViewGroup.cs
- DbParameterHelper.cs
- SectionInput.cs
- TrustSection.cs
- TextPattern.cs
- MouseActionValueSerializer.cs
- GridEntryCollection.cs
- ConfigurationStrings.cs
- AutomationElementCollection.cs
- VectorCollectionValueSerializer.cs
- ComplexTypeEmitter.cs
- InputReferenceExpression.cs
- SymbolDocumentGenerator.cs
- RemotingConfigParser.cs
- SignedPkcs7.cs
- ScriptResourceInfo.cs
- HtmlHistory.cs
- ListView.cs
- SymbolType.cs
- SHA256CryptoServiceProvider.cs
- LocalizedNameDescriptionPair.cs
- AsymmetricSignatureDeformatter.cs
- InfoCardKeyedHashAlgorithm.cs
- XamlInterfaces.cs
- FileEnumerator.cs
- StaticResourceExtension.cs
- TypeConverterAttribute.cs
- SetStateDesigner.cs
- StaticSiteMapProvider.cs
- DbDataSourceEnumerator.cs
- StateInitialization.cs
- CodeRegionDirective.cs
- Policy.cs
- EntityDataSourceSelectingEventArgs.cs
- DesignTimeParseData.cs
- DataSet.cs
- TypeHelper.cs
- Win32Interop.cs
- KernelTypeValidation.cs
- ExceptionUtil.cs
- TransactionManager.cs
- PathTooLongException.cs
- Msec.cs
- CollectionBuilder.cs
- Viewport2DVisual3D.cs
- CalendarDay.cs
- XsltFunctions.cs
- Point3DAnimationUsingKeyFrames.cs
- DataGridViewLinkCell.cs
- PropertyValueUIItem.cs
- BrowserTree.cs
- TextServicesContext.cs
- VectorAnimationUsingKeyFrames.cs
- StoryFragments.cs
- MulticastOption.cs
- SafePEFileHandle.cs
- TileModeValidation.cs
- OperationContractAttribute.cs
- SettingsBindableAttribute.cs
- TableLayoutSettings.cs
- ListViewDataItem.cs
- GroupJoinQueryOperator.cs
- CryptoApi.cs
- TextEditor.cs
- ArrangedElementCollection.cs