Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewGroup.cs / 1 / ListViewGroup.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Collections; using System.ComponentModel; using System.Runtime.Serialization; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using System.Globalization; using System.Security.Permissions; namespace System.Windows.Forms { ////// /// [ TypeConverterAttribute(typeof(ListViewGroupConverter)), ToolboxItem(false), DesignTimeVisible(false), DefaultProperty("Header"), Serializable ] public sealed class ListViewGroup : ISerializable { private ListView listView; private int id; private string header; private HorizontalAlignment headerAlignment = HorizontalAlignment.Left; private ListView.ListViewItemCollection items; private static int nextID; private static int nextHeader = 1; private object userData; private string name; ////// Represents a group within a ListView. /// /// ////// /// Creates a ListViewGroup. /// public ListViewGroup() : this(SR.GetString(SR.ListViewGroupDefaultHeader, nextHeader++)) { } ////// /// Creates a ListViewItem object from an Stream. /// private ListViewGroup(SerializationInfo info, StreamingContext context) : this() { Deserialize(info, context); } ////// /// Creates a ListViewItem object from a Key and a Name /// public ListViewGroup(string key, string headerText) : this() { this.name = key; this.header = headerText; } ////// /// Creates a ListViewGroup. /// public ListViewGroup(string header) { this.header = header; this.id = nextID++; } ////// /// Creates a ListViewGroup. /// public ListViewGroup(string header, HorizontalAlignment headerAlignment) : this(header) { this.headerAlignment = headerAlignment; } ////// /// The text displayed in the group header. /// [SRCategory(SR.CatAppearance)] public string Header { get { return header == null ? "" : header; } set { if (header != value) { header = value; if (listView != null) { listView.RecreateHandleInternal(); } } } } ////// /// The alignment of the group header. /// [ DefaultValue(HorizontalAlignment.Left), SRCategory(SR.CatAppearance) ] public HorizontalAlignment HeaderAlignment { get { return headerAlignment; } set { // VSWhidbey 144699: Verify that the value is within the enum's range. //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)HorizontalAlignment.Left, (int)HorizontalAlignment.Center)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(HorizontalAlignment)); } if (headerAlignment != value) { headerAlignment = value; UpdateListView(); } } } internal int ID { get { return id; } } ////// /// The items that belong to this group. /// [Browsable(false)] public ListView.ListViewItemCollection Items { get { if (items == null) { items = new ListView.ListViewItemCollection(new ListViewGroupItemCollection(this)); } return items; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public ListView ListView { get { return listView; } } internal ListView ListViewInternal { get { return listView; } set { if (listView != value) { listView = value; } } } ///[ SRCategory(SR.CatBehavior), SRDescription(SR.ListViewGroupNameDescr), Browsable(true), DefaultValue("") ] public string Name { get { return this.name; } set { this.name = value; } } /// [ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// private void Deserialize(SerializationInfo info, StreamingContext context) { int count = 0; foreach (SerializationEntry entry in info) { if (entry.Name == "Header") { Header = (string)entry.Value; } else if (entry.Name == "HeaderAlignment") { HeaderAlignment = (HorizontalAlignment)entry.Value; } else if (entry.Name == "Tag") { Tag = entry.Value; } else if (entry.Name == "ItemsCount") { count = (int)entry.Value; } else if (entry.Name == "Name") { Name = (string) entry.Value; } } if (count > 0) { ListViewItem[] items = new ListViewItem[count]; for (int i = 0; i < count; i++) { // SEC items[i] = (ListViewItem)info.GetValue("Item" + i, typeof(ListViewItem)); } Items.AddRange(items); } } ///[To be supplied.] ///public override string ToString() { return Header; } private void UpdateListView() { if (listView != null && listView.IsHandleCreated) { listView.UpdateGroupNative(this); } } /// /// /// To be supplied. /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] void ISerializable.GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("Header", this.Header); info.AddValue("HeaderAlignment", this.HeaderAlignment); info.AddValue("Tag", this.Tag); if (!String.IsNullOrEmpty(this.Name)) { info.AddValue("Name", this.Name); } if (items != null && items.Count > 0) { info.AddValue("ItemsCount", this.Items.Count); for (int i = 0; i < Items.Count; i ++) { info.AddValue("Item" + i.ToString(CultureInfo.InvariantCulture), Items[i], typeof(ListViewItem)); } } } } ////// /// [ListBindable(false)] public class ListViewGroupCollection : IList { private ListView listView; private ArrayList list; internal ListViewGroupCollection(ListView listView) { this.listView = listView; } ////// A collection of listview groups. /// ////// /// To be supplied. /// public int Count { get { return this.List.Count; } } ////// object ICollection.SyncRoot { get { return this; } } /// /// bool ICollection.IsSynchronized { get { return true; } } /// /// bool IList.IsFixedSize { get { return false; } } /// /// bool IList.IsReadOnly { get { return false; } } private ArrayList List { get { if (list == null) { list = new ArrayList(); } return list; } } /// /// /// To be supplied. /// public ListViewGroup this[int index] { get { return (ListViewGroup)this.List[index]; } set { if (this.List.Contains(value)) { return; } this.List[index] = value; } } ////// /// To be supplied. /// public ListViewGroup this[string key] { get { if (list == null) { return null; } for (int i = 0; i < list.Count; i ++) { if (String.Compare(key, this[i].Name, false /*case insensitive*/, System.Globalization.CultureInfo.CurrentCulture) == 0) { return this[i]; } } return null; } set { int index = -1; if (this.list == null) { return; // nothing to do } for (int i = 0; i < this.list.Count; i ++) { if (String.Compare(key, this[i].Name, false /*case insensitive*/, System.Globalization.CultureInfo.CurrentCulture) ==0) { index = i; break; } } if (index != -1) { this.list[index] = value; } } } ////// object IList.this[int index] { get { return this[index]; } set { if (value is ListViewGroup) { this[index] = (ListViewGroup)value; } } } /// /// /// To be supplied. /// public int Add(ListViewGroup group) { if (this.Contains(group)) { return -1; } // Will throw InvalidOperationException if group contains items which are parented by another listView. CheckListViewItems(group); group.ListViewInternal = this.listView; int index = this.List.Add(group); if (listView.IsHandleCreated) { listView.InsertGroupInListView(this.List.Count, group); MoveGroupItems(group); } return index; } ////// /// To be supplied. /// public ListViewGroup Add(string key, string headerText) { ListViewGroup group = new ListViewGroup(key, headerText); this.Add(group); return group; } ////// [SuppressMessage("Microsoft.Usage", "CA2208:InstantiateArgumentExceptionsCorrectly")] [ SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters") // "value" is the name of the param passed in. // So we don't have to localize it. ] int IList.Add(object value) { if (value is ListViewGroup) { return Add((ListViewGroup)value); } throw new ArgumentException("value"); } /// /// /// To be supplied. /// public void AddRange(ListViewGroup[] groups) { for(int i=0; i < groups.Length; i++) { Add(groups[i]); } } ////// /// To be supplied. /// public void AddRange(ListViewGroupCollection groups) { for(int i=0; i < groups.Count; i++) { Add(groups[i]); } } private void CheckListViewItems(ListViewGroup group) { for (int i = 0; i < group.Items.Count; i ++) { ListViewItem item = group.Items[i]; if (item.ListView != null && item.ListView != this.listView) { throw new ArgumentException(SR.GetString(SR.OnlyOneControl, item.Text)); } } } ////// /// To be supplied. /// public void Clear() { if (listView.IsHandleCreated) { for(int i=0; i < Count; i++) { listView.RemoveGroupFromListView(this[i]); } } // Dissociate groups from the ListView // for(int i=0; i < Count; i++) { this[i].ListViewInternal = null; } this.List.Clear(); // we have to tell the listView that there are no more groups // so the list view knows to remove items from the default group this.listView.UpdateGroupView(); } ////// /// To be supplied. /// public bool Contains(ListViewGroup value) { return this.List.Contains(value); } ////// bool IList.Contains(object value) { if (value is ListViewGroup) { return Contains((ListViewGroup)value); } return false; } /// /// /// To be supplied. /// public void CopyTo(Array array, int index) { this.List.CopyTo(array, index); } ////// /// To be supplied. /// public IEnumerator GetEnumerator() { return this.List.GetEnumerator(); } ////// /// To be supplied. /// public int IndexOf(ListViewGroup value) { return this.List.IndexOf(value); } ////// int IList.IndexOf(object value) { if (value is ListViewGroup) { return IndexOf((ListViewGroup)value); } return -1; } /// /// /// To be supplied. /// public void Insert(int index, ListViewGroup group) { if (Contains(group)) { return; } group.ListViewInternal = this.listView; this.List.Insert(index, group); if (listView.IsHandleCreated) { listView.InsertGroupInListView(index, group); MoveGroupItems(group); } } ////// void IList.Insert(int index, object value) { if (value is ListViewGroup) { Insert(index, (ListViewGroup)value); } } private void MoveGroupItems(ListViewGroup group) { Debug.Assert(listView.IsHandleCreated, "MoveGroupItems pre-condition: listView handle must be created"); foreach(ListViewItem item in group.Items) { if (item.ListView == this.listView) { item.UpdateStateToListView(item.Index); } } } /// /// /// To be supplied. /// public void Remove(ListViewGroup group) { group.ListViewInternal = null; this.List.Remove(group); if (listView.IsHandleCreated) { listView.RemoveGroupFromListView(group); } } ////// void IList.Remove(object value) { if (value is ListViewGroup) { Remove((ListViewGroup)value); } } /// /// /// To be supplied. /// public void RemoveAt(int index) { Remove(this[index]); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
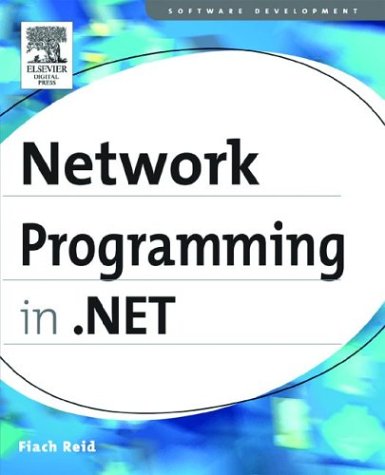
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StreamUpgradeBindingElement.cs
- PropertyManager.cs
- WindowsTreeView.cs
- DocumentPage.cs
- Int32RectConverter.cs
- ConnectionStringEditor.cs
- ValidationError.cs
- CodeVariableDeclarationStatement.cs
- DeviceContexts.cs
- OletxDependentTransaction.cs
- BindingContext.cs
- EnlistmentState.cs
- MimeObjectFactory.cs
- RepeaterItemEventArgs.cs
- CharEntityEncoderFallback.cs
- BoundPropertyEntry.cs
- DataSourceProvider.cs
- SafeSecurityHelper.cs
- TimeSpanConverter.cs
- FileDataSourceCache.cs
- OracleConnectionString.cs
- FolderLevelBuildProvider.cs
- PropertyGeneratedEventArgs.cs
- ToolStripItemImageRenderEventArgs.cs
- SubMenuStyleCollection.cs
- PropertyGridEditorPart.cs
- PageBuildProvider.cs
- XmlChoiceIdentifierAttribute.cs
- CustomTypeDescriptor.cs
- FileLoadException.cs
- MonitorWrapper.cs
- Int32.cs
- VerificationAttribute.cs
- SecureConversationServiceElement.cs
- LambdaCompiler.Unary.cs
- ScaleTransform3D.cs
- StylusDownEventArgs.cs
- ArraySortHelper.cs
- CompilerParameters.cs
- WebPartVerbCollection.cs
- SemaphoreSecurity.cs
- StyleSheetDesigner.cs
- TraceXPathNavigator.cs
- ListViewItem.cs
- SizeChangedInfo.cs
- TypeDelegator.cs
- ListBoxItem.cs
- SoapIgnoreAttribute.cs
- ModulesEntry.cs
- PackagingUtilities.cs
- DeadCharTextComposition.cs
- ColorAnimation.cs
- SByte.cs
- SerializationAttributes.cs
- IPGlobalProperties.cs
- HyperLink.cs
- BookmarkResumptionRecord.cs
- TextParentUndoUnit.cs
- AssemblyNameProxy.cs
- X509Utils.cs
- ResourceReferenceExpression.cs
- GAC.cs
- WebPartVerbCollection.cs
- StyleCollectionEditor.cs
- ProcessThread.cs
- InkCanvasSelectionAdorner.cs
- SplitterPanel.cs
- FileFormatException.cs
- DriveNotFoundException.cs
- CodeCompiler.cs
- DetailsViewUpdatedEventArgs.cs
- FormViewDesigner.cs
- DomNameTable.cs
- PersianCalendar.cs
- OdbcUtils.cs
- SerializableAttribute.cs
- MessageBodyMemberAttribute.cs
- XmlSubtreeReader.cs
- Timer.cs
- DynamicActionMessageFilter.cs
- MatrixTransform.cs
- WorkflowMarkupElementEventArgs.cs
- IPipelineRuntime.cs
- WebBrowserPermission.cs
- LogEntrySerializer.cs
- PerformanceCountersElement.cs
- TextWriterEngine.cs
- BitConverter.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- MethodRental.cs
- HtmlSelect.cs
- AsyncStreamReader.cs
- filewebrequest.cs
- ExpandableObjectConverter.cs
- AxWrapperGen.cs
- ReferentialConstraintRoleElement.cs
- ObjectListShowCommandsEventArgs.cs
- DiagnosticsConfiguration.cs
- DefaultValueAttribute.cs
- WebPartMovingEventArgs.cs