Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Dom / DomNameTable.cs / 1 / DomNameTable.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Schema; namespace System.Xml { internal class DomNameTable { XmlName[] entries; int count; int mask; XmlDocument ownerDocument; XmlNameTable nameTable; const int InitialSize = 64; // must be a power of two public DomNameTable( XmlDocument document ) { ownerDocument = document; nameTable = document.NameTable; entries = new XmlName[InitialSize]; mask = InitialSize - 1; Debug.Assert( ( entries.Length & mask ) == 0 ); // entries.Length must be a power of two } public XmlName GetName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } return null; } public XmlName AddName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } prefix = nameTable.Add(prefix); localName = nameTable.Add(localName); ns = nameTable.Add(ns); int index = hashCode & mask; XmlName name = XmlName.Create(prefix, localName, ns, hashCode, ownerDocument, entries[index], schemaInfo); entries[index] = name; if (count++ == mask) { Grow(); } return name; } private void Grow() { int newMask = mask * 2 + 1; XmlName[] oldEntries = entries; XmlName[] newEntries = new XmlName[newMask+1]; // use oldEntries.Length to eliminate the rangecheck for ( int i = 0; i < oldEntries.Length; i++ ) { XmlName name = oldEntries[i]; while ( name != null ) { int newIndex = name.HashCode & newMask; XmlName tmp = name.next; name.next = newEntries[newIndex]; newEntries[newIndex] = name; name = tmp; } } entries = newEntries; mask = newMask; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Schema; namespace System.Xml { internal class DomNameTable { XmlName[] entries; int count; int mask; XmlDocument ownerDocument; XmlNameTable nameTable; const int InitialSize = 64; // must be a power of two public DomNameTable( XmlDocument document ) { ownerDocument = document; nameTable = document.NameTable; entries = new XmlName[InitialSize]; mask = InitialSize - 1; Debug.Assert( ( entries.Length & mask ) == 0 ); // entries.Length must be a power of two } public XmlName GetName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } return null; } public XmlName AddName(string prefix, string localName, string ns, IXmlSchemaInfo schemaInfo) { if (prefix == null) { prefix = string.Empty; } if (ns == null) { ns = string.Empty; } int hashCode = XmlName.GetHashCode(localName); for (XmlName e = entries[hashCode & mask]; e != null; e = e.next) { if (e.HashCode == hashCode && ((object)e.LocalName == (object)localName || e.LocalName.Equals(localName)) && ((object)e.Prefix == (object)prefix || e.Prefix.Equals(prefix)) && ((object)e.NamespaceURI == (object)ns || e.NamespaceURI.Equals(ns)) && e.Equals(schemaInfo)) { return e; } } prefix = nameTable.Add(prefix); localName = nameTable.Add(localName); ns = nameTable.Add(ns); int index = hashCode & mask; XmlName name = XmlName.Create(prefix, localName, ns, hashCode, ownerDocument, entries[index], schemaInfo); entries[index] = name; if (count++ == mask) { Grow(); } return name; } private void Grow() { int newMask = mask * 2 + 1; XmlName[] oldEntries = entries; XmlName[] newEntries = new XmlName[newMask+1]; // use oldEntries.Length to eliminate the rangecheck for ( int i = 0; i < oldEntries.Length; i++ ) { XmlName name = oldEntries[i]; while ( name != null ) { int newIndex = name.HashCode & newMask; XmlName tmp = name.next; name.next = newEntries[newIndex]; newEntries[newIndex] = name; name = tmp; } } entries = newEntries; mask = newMask; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
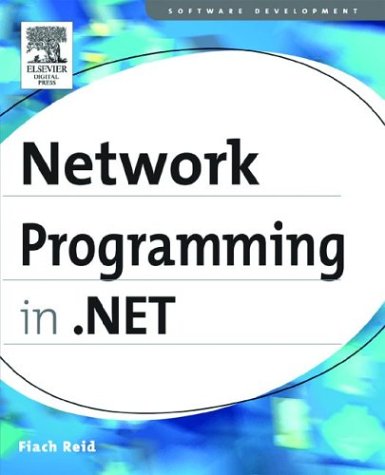
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityInstance.cs
- ListParaClient.cs
- ColumnResizeUndoUnit.cs
- XslException.cs
- Vector3DCollectionValueSerializer.cs
- TableLayoutPanelCellPosition.cs
- StickyNote.cs
- ProofTokenCryptoHandle.cs
- Utility.cs
- Size.cs
- FileUtil.cs
- OptimalTextSource.cs
- RuleRef.cs
- Stylesheet.cs
- BaseHashHelper.cs
- HasActivatableWorkflowEvent.cs
- PackageStore.cs
- SqlNode.cs
- ToolStripPanelSelectionGlyph.cs
- WebCategoryAttribute.cs
- ForeignKeyFactory.cs
- HtmlInputHidden.cs
- OperatingSystem.cs
- UrlMappingCollection.cs
- ValueHandle.cs
- ConcurrentStack.cs
- mansign.cs
- FrameDimension.cs
- IdnMapping.cs
- IntranetCredentialPolicy.cs
- XmlValueConverter.cs
- FindCriteria11.cs
- DesignerValidatorAdapter.cs
- EditCommandColumn.cs
- Single.cs
- ReadOnlyDataSource.cs
- DataControlFieldHeaderCell.cs
- DefaultHttpHandler.cs
- DynamicQueryableWrapper.cs
- GetPageNumberCompletedEventArgs.cs
- ProvidersHelper.cs
- BindingRestrictions.cs
- PeerObject.cs
- CommandSet.cs
- PropertyChangingEventArgs.cs
- DesignerActionService.cs
- EmptyEnumerable.cs
- ScrollEvent.cs
- VisualStates.cs
- InternalUserCancelledException.cs
- ConfigurationSectionGroup.cs
- Hash.cs
- ActiveXContainer.cs
- Assert.cs
- StateChangeEvent.cs
- ConfigurationStrings.cs
- PerformanceCounterPermission.cs
- EntityDataSourceConfigureObjectContext.cs
- Scene3D.cs
- HandledMouseEvent.cs
- TraceHandler.cs
- RichTextBoxConstants.cs
- HttpSocketManager.cs
- RoleServiceManager.cs
- SoapSchemaExporter.cs
- TextContainerChangedEventArgs.cs
- ManagedWndProcTracker.cs
- XmlSchemaObjectTable.cs
- WpfXamlLoader.cs
- FrugalMap.cs
- SiteMapDataSourceView.cs
- COM2EnumConverter.cs
- RegularExpressionValidator.cs
- ThreadPool.cs
- EventSetterHandlerConverter.cs
- AutomationFocusChangedEventArgs.cs
- XmlException.cs
- Aggregates.cs
- LogFlushAsyncResult.cs
- WebControlsSection.cs
- ScrollBarRenderer.cs
- ServiceMemoryGates.cs
- BookmarkInfo.cs
- SmtpTransport.cs
- DocumentSchemaValidator.cs
- UniqueID.cs
- RangeBase.cs
- TreeViewEvent.cs
- KeyTimeConverter.cs
- QuadraticEase.cs
- CollectionDataContract.cs
- ActivityCodeDomSerializationManager.cs
- DrawingCollection.cs
- CustomLineCap.cs
- KeyProperty.cs
- ByteStreamGeometryContext.cs
- ADConnectionHelper.cs
- OptimalTextSource.cs
- ChangeConflicts.cs
- Transform3DGroup.cs